How Can You Save a File in Python? A Step-by-Step Guide
In the world of programming, the ability to save files is a fundamental skill that every developer must master. Whether you’re working on a simple script or a complex application, knowing how to save data efficiently can make all the difference in your project’s success. Python, renowned for its simplicity and versatility, offers a variety of methods to handle file operations seamlessly. This article will guide you through the essential techniques for saving files in Python, empowering you to manage data like a pro.
Saving a file in Python is not just about writing data to disk; it’s about understanding the different file formats, modes, and libraries that can enhance your workflow. From text files to more structured formats like JSON and CSV, Python provides robust tools to cater to your needs. You’ll learn how to open files, write data, and ensure that your information is stored securely and efficiently.
As we delve deeper into the intricacies of file handling in Python, you’ll discover best practices that can help you avoid common pitfalls and improve your coding skills. Whether you’re a beginner eager to learn the ropes or an experienced programmer looking to refine your techniques, this exploration of file saving in Python will equip you with the knowledge to elevate your programming projects. Get ready to unlock the power of file management in Python!
Saving Text Files
To save a text file in Python, the built-in `open()` function is utilized, which can create a new file or overwrite an existing one. The function requires two parameters: the file name and the mode of operation. The most common modes for saving files are:
- `’w’`: Write mode, which overwrites the file if it exists or creates a new one if it does not.
- `’a’`: Append mode, which adds new data to the end of the file if it exists.
Here’s a basic example of saving a text file:
“`python
with open(‘example.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
“`
Using the `with` statement ensures that the file is properly closed after its suite finishes, even if an exception is raised.
Saving CSV Files
CSV (Comma-Separated Values) files are commonly used for data storage and exchange. Python’s `csv` module makes it straightforward to save data in this format.
A typical example of saving a list of dictionaries to a CSV file looks like this:
“`python
import csv
data = [
{‘Name’: ‘Alice’, ‘Age’: 30},
{‘Name’: ‘Bob’, ‘Age’: 25}
]
with open(‘people.csv’, ‘w’, newline=”) as file:
writer = csv.DictWriter(file, fieldnames=data[0].keys())
writer.writeheader()
writer.writerows(data)
“`
This code creates a CSV file called `people.csv`, writes the headers from the dictionary keys, and then adds the rows of data.
Saving JSON Files
JSON (JavaScript Object Notation) is a popular format for data interchange. Python provides the `json` module to facilitate saving and loading JSON data easily.
Here is how to save a Python dictionary as a JSON file:
“`python
import json
data = {
‘name’: ‘Alice’,
‘age’: 30,
‘city’: ‘New York’
}
with open(‘data.json’, ‘w’) as file:
json.dump(data, file)
“`
This example creates a file named `data.json` containing the serialized version of the dictionary.
Saving Binary Files
For saving binary data, such as images or executable files, Python allows the use of binary modes. The `’wb’` mode is used for writing binary files.
Here’s a simple example of saving an image:
“`python
image_data = b’\x89PNG\r\n\x1a\n…’ Example binary data
with open(‘image.png’, ‘wb’) as file:
file.write(image_data)
“`
This code snippet writes raw binary data to a file named `image.png`.
File Writing Modes
A summary of common file writing modes in Python can be found in the table below:
Mode | Description |
---|---|
‘w’ | Write mode; creates a new file or overwrites an existing one. |
‘a’ | Append mode; adds new data to the end of the file. |
‘wb’ | Write binary mode; used for binary files. |
‘r’ | Read mode; opens a file for reading only. |
These modes provide flexibility for various file operations, ensuring that developers can handle different types of data efficiently.
Saving Text Files
To save data in a text file using Python, you can employ the built-in `open()` function. This function allows you to specify the mode in which the file will be opened. For writing to a file, you typically use the `’w’` mode, which truncates the file if it already exists, or the `’a’` mode to append data to an existing file.
“`python
Example of saving a text file
data = “Hello, World!”
with open(‘example.txt’, ‘w’) as file:
file.write(data)
“`
- Modes:
- `’w’`: Write mode (overwrites existing file)
- `’a’`: Append mode (adds to existing file)
- `’r’`: Read mode (default)
Saving JSON Files
Python provides the `json` module to work with JSON data. To save a Python dictionary as a JSON file, use the `json.dump()` method.
“`python
import json
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file)
“`
- Key functions:
- `json.dump(obj, file)`: Writes `obj` to the `file`.
- `json.dumps(obj)`: Returns a JSON string of `obj`.
Saving CSV Files
For saving data in a CSV format, the `csv` module is utilized. This is particularly useful for tabular data.
“`python
import csv
data = [[‘Name’, ‘Age’], [‘Alice’, 30], [‘Bob’, 25]]
with open(‘data.csv’, ‘w’, newline=”) as csv_file:
writer = csv.writer(csv_file)
writer.writerows(data)
“`
- Methods:
- `csv.writer(file)`: Creates a writer object.
- `writerow(row)`: Writes a single row.
- `writerows(rows)`: Writes multiple rows.
Saving Binary Files
When dealing with binary data, the `wb` mode is used. This is common for images, audio files, and other non-text data.
“`python
data = b’This is binary data.’
with open(‘binary_file.bin’, ‘wb’) as binary_file:
binary_file.write(data)
“`
- Important considerations:
- Always use binary mode (`’wb’`) when writing binary files to avoid data corruption.
Saving Pickled Objects
The `pickle` module allows for serialization of Python objects, enabling you to save complex data structures.
“`python
import pickle
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.pkl’, ‘wb’) as pkl_file:
pickle.dump(data, pkl_file)
“`
- Key functions:
- `pickle.dump(obj, file)`: Serializes `obj` to `file`.
- `pickle.load(file)`: Deserializes `file` back into a Python object.
Saving with Context Managers
Using context managers (the `with` statement) is recommended when saving files in Python. This ensures that files are properly closed after their suite finishes, even if an error occurs.
- Benefits of using `with`:
- Automatic resource management.
- Reduces the risk of file corruption.
- Simplifies exception handling.
By following these methods and best practices, you can efficiently save various types of files in Python, ensuring data integrity and ease of access for future use.
Expert Insights on Saving Files in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When saving files in Python, it is crucial to understand the various modes available in the open() function. Using ‘w’ for writing, ‘a’ for appending, and ‘r’ for reading can significantly impact how data is stored and retrieved.”
Michael Thompson (Lead Python Developer, CodeCrafters). “Utilizing the with statement when opening files is a best practice. It ensures that files are properly closed after their suite finishes, which helps prevent data loss and resource leaks.”
Sarah Kim (Data Scientist, Analytics Hub). “For saving data in formats like CSV or JSON, leveraging libraries such as pandas or json is highly effective. These libraries provide built-in functions that simplify the process and enhance data integrity.”
Frequently Asked Questions (FAQs)
How do I save a text file in Python?
To save a text file in Python, use the built-in `open()` function with the mode set to `’w’` for writing. After opening the file, write your content using the `write()` method, and finally, close the file using the `close()` method or utilize a `with` statement for automatic closing.
What is the difference between ‘w’ and ‘a’ modes in file handling?
The `’w’` mode opens a file for writing and truncates the file to zero length if it already exists, effectively erasing its content. The `’a’` mode opens a file for appending, allowing new data to be added at the end of the file without deleting existing content.
How can I save a file in a specific directory using Python?
To save a file in a specific directory, provide the full path in the `open()` function. For example, use `open(‘/path/to/directory/filename.txt’, ‘w’)` to create or overwrite a file in the specified directory.
Can I save binary files in Python? If so, how?
Yes, you can save binary files in Python by opening the file in binary mode using `’wb’`. Use the `write()` method to save binary data, such as images or audio files, ensuring that the data is in bytes format.
What should I do if I encounter a permission error while saving a file?
If you encounter a permission error, check the file path and ensure that you have the necessary write permissions for the directory. You may need to change the directory permissions or choose a different location where you have write access.
How can I handle exceptions when saving files in Python?
To handle exceptions when saving files, use a `try` and `except` block around your file operations. This allows you to catch and manage errors, such as `IOError` or `FileNotFoundError`, providing a way to inform the user or log the error appropriately.
In summary, saving a file in Python is a straightforward process that can be accomplished using built-in functions and methods. The most common approach involves utilizing the `open()` function to create or open a file, followed by the `write()` method to store data within that file. It is essential to specify the correct mode when opening a file, such as ‘w’ for writing, ‘a’ for appending, or ‘r’ for reading, to ensure the desired operation is performed correctly.
Additionally, it is crucial to handle file operations with care to prevent data loss or corruption. Implementing context managers using the `with` statement is highly recommended, as this approach automatically manages file closing, even in the event of an error. This practice enhances code reliability and simplifies resource management.
Moreover, understanding file formats and encoding is vital for effective data storage and retrieval. Depending on the requirements, one may choose to save files in various formats, such as text, CSV, JSON, or binary. Each format serves different purposes and may require specific libraries or methods for proper handling.
mastering file-saving techniques in Python not only improves programming efficiency but also enhances data management capabilities. By following best practices and leveraging
Author Profile
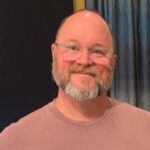
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?