How Can You Effectively Run a TypeScript File?
In the ever-evolving landscape of web development, TypeScript has emerged as a powerful tool that enhances JavaScript with static typing, making code more robust and maintainable. Whether you are a seasoned developer or just starting your coding journey, understanding how to run a TypeScript file is essential for harnessing the full potential of this versatile language. This article will guide you through the fundamental steps and best practices for executing TypeScript code, ensuring you can seamlessly integrate it into your projects and workflows.
Running a TypeScript file may seem daunting at first, especially for those accustomed to traditional JavaScript. However, with the right tools and a bit of guidance, you can unlock the benefits of TypeScript in no time. From setting up your development environment to compiling TypeScript into JavaScript, this process is not only straightforward but also rewarding. You’ll discover how TypeScript’s features can lead to cleaner, more efficient code, and how running your TypeScript files can enhance your development experience.
As we delve deeper into the specifics of executing TypeScript files, we will explore various methods and tools available to streamline the process. Whether you prefer using the command line, integrating with popular text editors, or leveraging build tools, there are multiple pathways to get your TypeScript code up
Setting Up Your Environment
To run a TypeScript file, you first need to ensure that your development environment is properly set up. This involves installing Node.js and TypeScript. Follow these steps:
- Install Node.js: Node.js is a JavaScript runtime that enables you to execute JavaScript code outside of a browser. You can download it from the official website and follow the installation instructions for your operating system.
- Install TypeScript: After Node.js is installed, you can install TypeScript globally using npm (Node Package Manager). Open your terminal or command prompt and run the following command:
“`
npm install -g typescript
“`
- Verify Installation: To confirm that TypeScript is installed correctly, run:
“`
tsc -v
“`
This command should display the version of TypeScript installed on your system.
Compiling TypeScript to JavaScript
TypeScript files have a `.ts` extension and must be compiled into JavaScript before they can be executed. You can compile a TypeScript file using the TypeScript compiler (`tsc`). Here’s how to do it:
- Navigate to the directory where your TypeScript file is located.
- Use the following command to compile the file:
“`
tsc yourfile.ts
“`
This will generate a `yourfile.js` JavaScript file in the same directory.
Command | Description |
---|---|
tsc yourfile.ts | Compiles the TypeScript file into JavaScript. |
tsc -w | Watches for changes in TypeScript files and re-compiles automatically. |
Running the Compiled JavaScript File
Once you have compiled your TypeScript file into JavaScript, you can run it using Node.js. Execute the following command in your terminal:
“`
node yourfile.js
“`
This will execute the JavaScript code generated from your TypeScript file.
Using ts-node for Direct Execution
An alternative approach to running TypeScript files directly without the need for manual compilation is to use `ts-node`. This utility allows you to run TypeScript files directly. To set it up:
- Install ts-node: You can install `ts-node` globally with npm:
“`
npm install -g ts-node
“`
- Run Your TypeScript File: Now you can execute your TypeScript file directly with:
“`
ts-node yourfile.ts
“`
This method is particularly useful for development and testing, as it eliminates the need for separate compilation steps.
Common Issues and Troubleshooting
When working with TypeScript, you may encounter a few common issues. Here are some troubleshooting tips:
- Error: Cannot find module: Ensure that all required modules are installed. Use npm to install any missing packages.
- Type Errors: Make sure that your TypeScript code adheres to the type definitions. TypeScript is strict about types, so refer to the TypeScript documentation for guidance.
- Configuration Issues: If you have a `tsconfig.json` file, ensure that it is correctly configured to reflect your project’s structure and requirements.
By following these steps and troubleshooting tips, you can efficiently run and manage your TypeScript files in a Node.js environment.
Setting Up the Environment
To run a TypeScript file, you need to ensure that your development environment is properly configured. This includes having Node.js and TypeScript installed. Follow these steps:
- Install Node.js: Download and install Node.js from the [official website](https://nodejs.org/). This will also install `npm`, the Node package manager, which is required for managing TypeScript.
- Install TypeScript: Open your command line interface (CLI) and run the following command:
“`bash
npm install -g typescript
“`
This command installs TypeScript globally on your machine, making it accessible from any directory.
Creating a TypeScript File
Once your environment is set up, you can create a TypeScript file. Here’s how:
- Open your text editor or integrated development environment (IDE).
- Create a new file and save it with a `.ts` extension, for example, `app.ts`.
- Write your TypeScript code in this file.
Example content for `app.ts`:
“`typescript
let message: string = “Hello, TypeScript!”;
console.log(message);
“`
Compiling TypeScript to JavaScript
TypeScript code must be compiled into JavaScript before it can be executed. Use the TypeScript compiler (tsc) for this purpose:
- Open your CLI.
- Navigate to the directory where your TypeScript file is located.
- Run the following command:
“`bash
tsc app.ts
“`
This command will generate a JavaScript file named `app.js` in the same directory.
Running the Compiled JavaScript File
After compiling your TypeScript code, you can run the resulting JavaScript file using Node.js:
- In the CLI, execute the following command:
“`bash
node app.js
“`
This will execute the JavaScript file, and you should see the output in the console.
Using ts-node for Simplicity
For a more streamlined process, you can use `ts-node`, which allows you to run TypeScript files directly without manual compilation:
- Install ts-node:
“`bash
npm install -g ts-node
“`
- Run your TypeScript file:
“`bash
ts-node app.ts
“`
This command eliminates the need for a separate compilation step, making development faster.
Common Issues and Troubleshooting
When working with TypeScript, you might encounter some common issues. Here are a few resolutions:
Issue | Solution |
---|---|
TypeScript not recognized | Ensure TypeScript is installed globally. Check your PATH environment variable. |
Compilation errors | Review the error messages for specific syntax errors or type mismatches. |
Node.js not found | Confirm that Node.js is installed and accessible via the command line. |
By following these guidelines, you can efficiently run TypeScript files and enhance your development workflow.
Expert Insights on Running TypeScript Files
Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “To run a TypeScript file, it is essential to first ensure that you have Node.js and TypeScript installed on your machine. Once installed, you can compile the TypeScript file using the command `tsc yourFile.ts`, which generates a corresponding JavaScript file. Finally, execute the JavaScript file using `node yourFile.js` to see the output.”
Michael Thompson (Lead Developer, CodeCraft Solutions). “Running a TypeScript file can be streamlined by utilizing the `ts-node` package, which allows you to execute TypeScript files directly without the need for a separate compilation step. Simply install `ts-node` globally with `npm install -g ts-node`, and then run your TypeScript file using `ts-node yourFile.ts` for immediate execution.”
Sara Patel (Technical Writer, DevInsights). “For those who prefer an integrated development environment (IDE), tools like Visual Studio Code provide built-in support for TypeScript. You can run your TypeScript files directly from the terminal in the IDE, making it convenient to test and debug your code as you develop. Ensure that the TypeScript compiler is properly configured in your project settings for optimal results.”
Frequently Asked Questions (FAQs)
How do I run a TypeScript file from the command line?
To run a TypeScript file from the command line, first ensure you have TypeScript installed globally using `npm install -g typescript`. Then, compile the TypeScript file using `tsc filename.ts`, which generates a JavaScript file. Finally, execute the JavaScript file using `node filename.js`.
Do I need to install Node.js to run TypeScript files?
Yes, Node.js is required to execute the compiled JavaScript files generated from TypeScript. Ensure you have Node.js installed on your machine to run TypeScript effectively.
Can I run TypeScript files directly without compiling?
Yes, you can run TypeScript files directly using a tool like `ts-node`. Install it via `npm install -g ts-node`, and then execute your TypeScript file with `ts-node filename.ts` without the need for prior compilation.
What are the prerequisites for running TypeScript files?
You need to have Node.js and TypeScript installed on your system. Additionally, ensure that your TypeScript file is free of syntax errors before attempting to run it.
Is there an integrated development environment (IDE) that supports running TypeScript files?
Yes, several IDEs such as Visual Studio Code, WebStorm, and Atom support running TypeScript files directly. They often include built-in terminals and extensions that facilitate seamless execution of TypeScript code.
How can I set up a TypeScript project to run multiple files?
To set up a TypeScript project, create a `tsconfig.json` file in your project directory. This file allows you to configure compiler options and specify the files to include. After setup, you can compile the entire project using `tsc` and run the generated JavaScript files with Node.js.
running a TypeScript file involves several key steps that ensure a smooth execution of your code. First, it is essential to have TypeScript installed on your system, either globally or locally within your project. This can be achieved using npm, the Node.js package manager, with the command `npm install -g typescript` for a global installation. After installation, you can compile TypeScript files into JavaScript using the TypeScript compiler (tsc), which is a crucial step since browsers do not natively understand TypeScript.
Once the TypeScript file is compiled, the resulting JavaScript file can be executed in a Node.js environment or served in a browser. For Node.js, you can simply run the command `node filename.js`, where `filename.js` is the compiled output of your TypeScript code. This process highlights the importance of understanding both the TypeScript compilation process and the environment in which your code will run.
Key takeaways from this discussion include the necessity of having a proper development setup that includes TypeScript and Node.js, as well as the importance of compiling TypeScript files to JavaScript before execution. Additionally, familiarizing oneself with the command line interface is beneficial for efficiently running and managing TypeScript
Author Profile
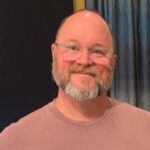
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?