How Can You Run Old Laravel Apps on Different PHP Versions?
How To Run Old Laravel Apps With Diff PHP
In the fast-paced world of web development, keeping up with the latest technologies can often feel like a race against time. Laravel, one of the most popular PHP frameworks, has seen numerous updates that introduce new features, improvements, and optimizations. However, many developers find themselves in a situation where they need to maintain or run older Laravel applications that were built on previous versions of PHP. This can pose significant challenges, particularly when the PHP version required by the application differs from the environment in which it is currently being executed.
Navigating the complexities of running old Laravel apps with different PHP versions requires a clear understanding of both the framework and the underlying PHP environment. From compatibility issues to potential security vulnerabilities, there are several factors to consider to ensure that your application runs smoothly and efficiently. Moreover, the process involves more than just switching PHP versions; it often necessitates adjustments to dependencies, configuration files, and even the codebase itself to align with the requirements of the older framework.
In this article, we will explore effective strategies and best practices for running legacy Laravel applications in environments with different PHP versions. Whether you’re a seasoned developer or just starting out, you’ll gain insights into the steps necessary to breathe new life into your old projects while
Understanding Compatibility Issues
Running an old Laravel application on a different PHP version can introduce several compatibility issues. Laravel applications are often tightly coupled with specific versions of PHP due to dependencies and language features. The following are common issues you may encounter:
- Deprecated Functions: Functions used in older PHP versions may no longer exist or function differently in newer versions.
- Changed Error Handling: PHP’s error handling mechanisms may vary, affecting how errors are reported and handled in your application.
- Library and Package Support: Third-party libraries may not support the newer PHP version, leading to potential breaks in functionality.
Steps to Run Old Laravel Apps with Different PHP Versions
To successfully run an old Laravel application on a different PHP version, follow these steps:
- **Set Up a Version Management Tool**: Utilize tools like PHP Version Manager (phpenv) or Composer to manage multiple PHP versions on your server.
- **Check Laravel Version**: Confirm the Laravel version your application is using. Different Laravel versions have specific PHP version requirements. Refer to the official Laravel documentation for compatibility:
Laravel Version | Required PHP Version |
---|---|
5.1 | >=5.5.9 |
5.2 | >=5.5.9 |
5.3 | >=5.6.4 |
5.4 | >=7.0 |
5.5 | >=7.0 |
6.x | >=7.2.5 |
7.x | >=7.2.5 |
8.x | >=7.3 |
9.x | >=8.0 |
- Adjust Composer Dependencies: Update your `composer.json` file to reflect the PHP version changes. Run `composer update` to ensure all dependencies are compatible with the new PHP version.
- Modify Configuration Files: Review your `.env` and configuration files for any settings that may require updates due to the new PHP version.
- Test Your Application: After making the necessary changes, run your application in a local or staging environment to identify any errors or issues that arise from the version change.
Using Docker for Isolation
Docker provides an effective way to isolate your Laravel application with the required PHP version without affecting your local environment. By creating a Docker container, you can specify the exact PHP version needed.
- Create a Dockerfile: Define the PHP version and install necessary extensions.
- docker-compose.yml: Set up your services for Laravel, including the database and web server.
Example Dockerfile:
“`Dockerfile
FROM php:7.4-fpm
Install dependencies
RUN docker-php-ext-install pdo pdo_mysql
Set working directory
WORKDIR /var/www
Copy application files
COPY . .
Install Composer
COPY –from=composer:latest /usr/bin/composer /usr/bin/composer
“`
Final Testing and Deployment
Once you’ve configured your application, conduct thorough testing to ensure that all functionalities work as expected. Address any errors or deprecations that arise during this process. After successful testing, you can deploy your application to the production environment with confidence.
- Use Version Control: Ensure all changes are tracked in a version control system like Git to facilitate rollback if necessary.
- Monitoring: Implement logging and monitoring to catch any issues in real-time post-deployment.
By following these steps, you can effectively manage running old Laravel applications on different PHP versions while minimizing compatibility issues.
Understanding PHP Version Compatibility
Running old Laravel applications on different PHP versions requires careful consideration of compatibility issues. Each Laravel version has a minimum supported PHP version, which must be adhered to in order to ensure the application functions correctly.
- Laravel 5.5: Requires PHP 7.0.0 or higher.
- Laravel 5.6: Requires PHP 7.1.3 or higher.
- Laravel 5.7: Requires PHP 7.1.3 or higher.
- Laravel 5.8: Requires PHP 7.1.3 or higher.
- Laravel 6.x: Requires PHP 7.2.5 or higher.
- Laravel 7.x: Requires PHP 7.2.5 or higher.
- Laravel 8.x: Requires PHP 7.3 or higher.
- Laravel 9.x: Requires PHP 8.0 or higher.
Ensure that your PHP version aligns with the version required by your Laravel application.
Setting Up Multiple PHP Versions
To run old Laravel applications with different PHP versions on the same machine, you can use tools like Docker, Homestead, or PHP version managers such as `phpbrew` or `phpenv`.
- Docker: Create containers for each PHP version.
- Laravel Homestead: Use the pre-packaged Vagrant box that allows you to specify PHP versions.
- phpbrew: Manage multiple PHP installations on your local environment.
Here’s a brief example of how to set up PHP versions using Docker:
“`dockerfile
FROM php:7.2-fpm
COPY . /var/www
WORKDIR /var/www
RUN composer install
“`
Updating Dependencies
When switching PHP versions, it is crucial to review and update dependencies in your `composer.json` file. You may need to:
- Update Laravel and other package versions to be compatible with the new PHP version.
- Use `composer update` to refresh the package lock and ensure all dependencies are compatible.
Check for deprecated packages or those that have not been maintained. You can use the following command to check for outdated packages:
“`bash
composer outdated
“`
Handling Deprecated Functions
PHP’s evolution may lead to deprecated functions or changes in behavior. When updating your application:
- Review the PHP migration guide for deprecated functions.
- Refactor your code to replace deprecated functions with their modern alternatives.
Example of a deprecated function:
“`php
// Deprecated
mysql_connect(‘localhost’, ‘user’, ‘pass’);
// Use PDO or MySQLi instead
$pdo = new PDO(‘mysql:host=localhost;dbname=test’, ‘user’, ‘pass’);
“`
Testing and Debugging
After setting up the environment and updating dependencies, thorough testing is essential. Follow these steps:
- Unit Testing: Ensure unit tests are passing with the new PHP version.
- Integration Testing: Test the application’s interaction with databases and other services.
- Manual Testing: Perform manual tests on critical paths of the application.
Utilize tools like PHPUnit for automated tests and Xdebug for debugging to identify any issues that arise during testing.
Environment Configuration
When running an application on different PHP versions, ensure your `.env` file is properly configured. Key areas to verify include:
- Database connections
- Cache and session drivers
- Mail service configurations
Consider using version-specific environment files if necessary, such as `.env.php72`, for clarity and organization.
This structured approach facilitates running old Laravel applications on different PHP versions efficiently while minimizing potential issues.
Strategies for Running Legacy Laravel Applications on Different PHP Versions
Dr. Emily Chen (Senior Software Architect, Tech Innovations Inc.). “When dealing with legacy Laravel applications, it is crucial to first assess the compatibility of your application with the desired PHP version. Utilizing tools like Laravel Shift can help automate the upgrade process, but manual adjustments may still be necessary to address deprecated functions and libraries.”
Michael Thompson (PHP Development Specialist, CodeCraft Solutions). “To successfully run old Laravel applications on different PHP versions, consider using Docker containers. This allows you to create isolated environments tailored to each application’s specific requirements, ensuring that dependencies and configurations do not conflict.”
Lisa Patel (Lead Laravel Developer, WebMasters Co.). “Maintaining backward compatibility is essential when upgrading PHP for legacy Laravel apps. Implementing feature flags can allow you to gradually introduce new PHP features while ensuring that existing functionality remains intact, thus minimizing the risk of breaking changes.”
Frequently Asked Questions (FAQs)
How can I run an old Laravel application with a different PHP version?
To run an old Laravel application with a different PHP version, you can use tools like Docker or PHP version managers such as PHPbrew or Homebrew. These tools allow you to specify the PHP version for your application environment without affecting the global PHP installation.
What are the common compatibility issues when running old Laravel apps on newer PHP versions?
Common compatibility issues include deprecated functions, changes in error handling, and differences in library dependencies. It’s essential to review the Laravel upgrade guide and PHP migration documentation to address these issues systematically.
Is it possible to use multiple PHP versions on the same server for different Laravel applications?
Yes, it is possible to use multiple PHP versions on the same server. You can configure your web server (e.g., Apache or Nginx) to point to different PHP versions based on the application directory or use a version manager to switch between them.
What steps should I take to upgrade an old Laravel app to support a newer PHP version?
To upgrade an old Laravel app, start by reviewing the Laravel upgrade guide for the specific version you are targeting. Update your dependencies in `composer.json`, run `composer update`, and test your application thoroughly to identify and fix any compatibility issues.
Are there specific PHP extensions required for older Laravel versions?
Yes, older Laravel versions may require specific PHP extensions like OpenSSL, PDO, Mbstring, and Tokenizer. Ensure that these extensions are enabled in your PHP configuration to avoid runtime errors.
What tools can assist in testing compatibility when running an old Laravel app with a different PHP version?
Tools like PHPStan, Psalm, and Laravel Shift can help identify compatibility issues. Additionally, using PHPUnit for unit testing can ensure that your application’s functionality remains intact after changing PHP versions.
Running old Laravel applications with different versions of PHP can present various challenges, primarily due to the framework’s evolving dependencies and features. To successfully manage these applications, it is essential to understand the compatibility requirements of both Laravel and PHP. Each Laravel version has specific PHP version requirements, and using an incompatible PHP version can lead to runtime errors or unexpected behavior. Therefore, it is crucial to verify the Laravel version in use and ensure that the PHP version aligns with the framework’s compatibility guidelines.
One effective approach to running old Laravel applications with different PHP versions is to utilize tools such as Docker or Homestead. These tools allow developers to create isolated environments tailored to specific application requirements, including the desired PHP version. By setting up a containerized environment, developers can easily switch between PHP versions without affecting the host system or other applications. This flexibility not only streamlines the development process but also minimizes potential conflicts that may arise from version discrepancies.
Additionally, it is advisable to review and update the application’s dependencies, especially if the Laravel app is significantly outdated. Utilizing Composer to manage dependencies can help identify any outdated packages that may not be compatible with the newer PHP version. Furthermore, testing the application thoroughly after upgrading PHP is essential to ensure that all functionalities work as intended
Author Profile
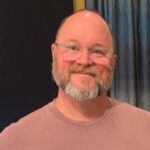
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?