How Can You Run a Laravel App in Docker Effectively?
In the ever-evolving landscape of web development, the need for efficient and scalable solutions has never been more pronounced. Laravel, a powerful PHP framework, has emerged as a favorite among developers for building robust web applications. However, as projects grow in complexity, managing dependencies and ensuring a consistent environment can become a daunting task. Enter Docker—a revolutionary tool that simplifies the process of deploying applications by encapsulating them in lightweight containers. In this article, we will explore how to run a Laravel app in Docker, unlocking a world of streamlined development and deployment.
By leveraging Docker, developers can create isolated environments that mirror production settings, eliminating the classic “it works on my machine” dilemma. This approach not only enhances collaboration among team members but also ensures that applications run seamlessly across different systems. In the following sections, we will delve into the essential steps for setting up a Laravel application within a Docker container, covering everything from configuration to deployment. Whether you’re a seasoned developer or just starting your journey with Laravel, this guide will equip you with the knowledge to harness the power of Docker and elevate your development workflow.
As we navigate through the intricacies of containerization, you’ll discover best practices for managing your Laravel app’s dependencies, optimizing performance, and ensuring a smooth development experience. Get ready
Setting Up the Docker Environment
To run a Laravel application in Docker, you first need to set up the Docker environment properly. This involves creating a Dockerfile and a `docker-compose.yml` file that will define the services, networks, and volumes required for your application.
The Dockerfile is used to build a custom image for your Laravel application. Here’s a basic example of what your Dockerfile might look like:
“`Dockerfile
FROM php:8.0-fpm
Set working directory
WORKDIR /var/www
Install dependencies
RUN apt-get update && apt-get install -y libpng-dev libjpeg-dev libfreetype6-dev libzip-dev unzip git
Install PHP extensions
RUN docker-php-ext-configure gd –with-freetype –with-jpeg \
&& docker-php-ext-install gd zip
Copy application files
COPY . .
Install Composer
RUN curl -sS https://getcomposer.org/installer | php — –install-dir=/usr/local/bin –filename=composer
Install application dependencies
RUN composer install
“`
The docker-compose.yml file will define the services needed for your Laravel application, including the web server and database. Below is a sample configuration:
“`yaml
version: ‘3.8’
services:
app:
build:
context: .
dockerfile: Dockerfile
image: laravel-app
container_name: laravel-app
ports:
- “8000:80”
volumes:
- .:/var/www
networks:
- app-network
db:
image: mysql:5.7
container_name: mysql
restart: always
environment:
MYSQL_ROOT_PASSWORD: secret
MYSQL_DATABASE: laravel
MYSQL_USER: user
MYSQL_PASSWORD: password
ports:
- “3306:3306”
volumes:
- db_data:/var/lib/mysql
networks:
- app-network
networks:
app-network:
driver: bridge
volumes:
db_data:
“`
Building and Running the Docker Containers
Once you have your `Dockerfile` and `docker-compose.yml` ready, you can build and run your containers. Use the following command in your terminal:
“`bash
docker-compose up –build
“`
This command will build the Docker image as per your Dockerfile and start your Laravel application along with the MySQL database defined in your `docker-compose.yml`.
You can also run the containers in detached mode by adding the `-d` flag:
“`bash
docker-compose up –build -d
“`
To view the logs of the running containers, you can use:
“`bash
docker-compose logs -f
“`
Accessing the Laravel Application
After successfully running the containers, your Laravel application should be accessible through your browser at `http://localhost:8000`. Ensure that your `.env` file in the Laravel application is configured to connect to the MySQL database with the correct credentials.
The `.env` database configuration might look like this:
“`
DB_CONNECTION=mysql
DB_HOST=db
DB_PORT=3306
DB_DATABASE=laravel
DB_USERNAME=user
DB_PASSWORD=password
“`
Managing the Containers
You can manage your Docker containers with several commands. Below is a summary of useful commands for managing your Laravel application in Docker:
Command | Description |
---|---|
`docker-compose up` | Start the containers defined in `docker-compose.yml`. |
`docker-compose down` | Stop and remove the containers. |
`docker-compose exec app bash` | Access the shell of the Laravel app container. |
`docker-compose ps` | List all the containers defined in the project. |
`docker-compose logs` | View logs from the containers. |
By following these steps, you can run a Laravel application efficiently within a Docker environment, ensuring your development process is streamlined and consistent.
Setting Up the Docker Environment
To run a Laravel application in Docker, the first step is to create a suitable Docker environment. This involves defining the services needed for your application, primarily the web server and the database.
- Create a Dockerfile: This file contains the instructions for building your application’s Docker image.
“`dockerfile
FROM php:8.1-fpm
Install system dependencies
RUN apt-get update && apt-get install -y \
libpng-dev \
libjpeg-dev \
libfreetype6-dev \
&& docker-php-ext-configure gd –with-freetype –with-jpeg \
&& docker-php-ext-install gd \
&& apt-get clean \
&& rm -rf /var/lib/apt/lists/*
Set working directory
WORKDIR /var/www
Copy application files
COPY . .
Install Composer
COPY –from=composer:latest /usr/bin/composer /usr/bin/composer
Install Laravel dependencies
RUN composer install
“`
- Create a docker-compose.yml file: This file defines and runs multi-container Docker applications.
“`yaml
version: ‘3.8’
services:
app:
build:
context: .
dockerfile: Dockerfile
image: laravel-app
container_name: laravel_app
volumes:
- .:/var/www
ports:
- “8000:80”
depends_on:
- db
db:
image: mysql:5.7
container_name: mysql_db
restart: always
environment:
MYSQL_ROOT_PASSWORD: root
MYSQL_DATABASE: laravel
MYSQL_USER: user
MYSQL_PASSWORD: password
ports:
- “3306:3306”
volumes:
- db_data:/var/lib/mysql
volumes:
db_data:
“`
Building and Running the Docker Containers
Once the necessary configuration files are in place, you can build and run the Docker containers.
- Build the Docker images: Run the following command in the terminal:
“`bash
docker-compose build
“`
- Start the containers: Use the command below to start your Laravel application along with the database:
“`bash
docker-compose up -d
“`
- Check the running containers: You can verify that everything is running smoothly by executing:
“`bash
docker-compose ps
“`
Accessing the Laravel Application
After starting the containers, you can access your Laravel application. By default, the application will be available at:
- Local Development URL: `http://localhost:8000`
Ensure that your `.env` file is configured correctly to connect to the MySQL database. The database connection settings should look like this:
“`plaintext
DB_CONNECTION=mysql
DB_HOST=db
DB_PORT=3306
DB_DATABASE=laravel
DB_USERNAME=user
DB_PASSWORD=password
“`
Managing Artisan Commands
To run Artisan commands, you can execute the following command, which allows you to run commands inside the application container:
“`bash
docker-compose exec app php artisan migrate
“`
This command runs the migrations for your Laravel application. You can replace `migrate` with any other Artisan command as needed.
Stopping and Removing Containers
When you need to stop the application, you can do so using:
“`bash
docker-compose down
“`
This command stops and removes all containers defined in your `docker-compose.yml` file. If you want to remove the associated volumes as well, use:
“`bash
docker-compose down -v
“`
This comprehensive approach ensures that your Laravel application runs seamlessly within a Docker environment, leveraging containers for both development and production setups.
Expert Insights on Running Laravel Apps in Docker
Dr. Emily Chen (Senior Software Engineer, Cloud Solutions Inc.). “Utilizing Docker for Laravel applications streamlines the development process significantly. By encapsulating the application and its dependencies in a container, developers can ensure consistency across different environments, which is crucial for avoiding deployment issues.”
Michael Thompson (DevOps Consultant, Tech Innovations Group). “One of the key advantages of running Laravel apps in Docker is the ability to leverage container orchestration tools like Kubernetes. This not only simplifies scaling but also enhances the management of microservices, which is increasingly becoming the norm in modern web applications.”
Sarah Patel (Full Stack Developer, CodeCraft Agency). “When setting up a Laravel app in Docker, it is vital to configure the environment variables correctly. This ensures that the application behaves as expected in both development and production environments, thereby reducing the risk of runtime errors.”
Frequently Asked Questions (FAQs)
How do I set up a Docker environment for my Laravel application?
To set up a Docker environment for a Laravel application, create a `Dockerfile` and a `docker-compose.yml` file in your project root. The `Dockerfile` should define the base image, dependencies, and configurations, while the `docker-compose.yml` file manages services like PHP, MySQL, and Nginx.
What Docker images are recommended for running a Laravel app?
Commonly used Docker images for Laravel include `php:8.0-fpm` for PHP processing, `nginx:latest` for serving the application, and `mysql:latest` for the database. You can also use `laravelphp/php-fpm` for a pre-configured PHP environment tailored for Laravel.
How can I connect my Laravel application to a MySQL database in Docker?
In your `docker-compose.yml`, define a MySQL service with the necessary environment variables such as `MYSQL_ROOT_PASSWORD`, `MYSQL_DATABASE`, `MYSQL_USER`, and `MYSQL_PASSWORD`. In your Laravel `.env` file, set the database connection parameters to match the MySQL service configuration.
What commands should I run to start my Laravel application in Docker?
To start your Laravel application in Docker, navigate to your project directory and run `docker-compose up -d`. This command builds the containers and starts them in detached mode. You can then access your application via the defined port in the `docker-compose.yml`.
How do I run migrations and seed the database in a Dockerized Laravel app?
To run migrations and seed your database, use the command `docker-compose exec app php artisan migrate –seed`, where `app` is the name of your Laravel service defined in `docker-compose.yml`. This command executes the migration and seeding process within the container.
How can I access my Laravel application logs when running in Docker?
You can access your Laravel application logs by executing `docker-compose logs app`, where `app` is the service name for your Laravel application. Alternatively, you can check the logs directly in the container by running `docker-compose exec app tail -f storage/logs/laravel.log`.
Running a Laravel application in Docker provides a robust and efficient environment for development and deployment. By utilizing Docker, developers can create isolated environments that ensure consistency across different stages of the application lifecycle. The process typically involves creating a Dockerfile to define the application’s environment, setting up a docker-compose.yml file to manage services, and configuring necessary dependencies such as PHP, MySQL, and Nginx.
One of the key advantages of using Docker with Laravel is the ability to easily replicate the production environment locally. This minimizes the “works on my machine” syndrome, as developers can ensure that their application behaves identically in both development and production settings. Additionally, Docker’s containerization allows for simplified dependency management and version control, which can significantly streamline the development workflow.
Moreover, leveraging Docker for a Laravel application enhances scalability and facilitates continuous integration and deployment (CI/CD) practices. By containerizing the application, teams can deploy updates quickly and reliably, ensuring that new features and fixes are delivered to users without downtime. Overall, adopting Docker for Laravel development not only improves efficiency but also fosters a more collaborative and agile development process.
Author Profile
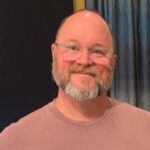
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?