How Can You Run a JavaScript File in Terminal?
In the world of web development, JavaScript has emerged as a powerhouse, not just for creating dynamic web pages but also for powering server-side applications. While many developers are accustomed to running their scripts directly in a browser, there’s a whole new realm of possibilities when you take your JavaScript files to the terminal. Whether you’re looking to streamline your development process, automate tasks, or simply explore the capabilities of JavaScript beyond the browser, knowing how to run a JavaScript file in the terminal is an invaluable skill.
In this article, we will delve into the straightforward yet powerful method of executing JavaScript files directly from your command line interface. This approach opens up a world of efficiency, allowing you to test snippets, run scripts, and even manage complex applications with ease. By harnessing the terminal, you can enhance your workflow and gain greater control over your JavaScript projects.
We’ll explore the tools and environments that make running JavaScript in the terminal not only possible but also enjoyable. From setting up the necessary software to understanding the commands that will bring your scripts to life, this guide will equip you with the knowledge you need to elevate your JavaScript development experience. Whether you’re a seasoned developer or just starting out, the terminal is a powerful ally in your coding journey
Prerequisites for Running JavaScript Files
Before executing JavaScript files in the terminal, it is essential to have Node.js installed on your system. Node.js is a JavaScript runtime that enables you to run JavaScript code outside of a web browser. Here are the steps to check if Node.js is installed and how to install it if necessary:
- Open your terminal.
- Type the command `node -v` to check the installed version of Node.js.
- If Node.js is not installed, download it from the official [Node.js website](https://nodejs.org/) and follow the installation instructions relevant to your operating system.
Creating a JavaScript File
To run a JavaScript file, you must first create one. Here’s how to create a simple JavaScript file:
- Open your terminal.
- Navigate to the directory where you want to create your JavaScript file using the `cd` command.
- Use a text editor to create a new file. For instance, you can use `nano`:
“`
nano myscript.js
“`
- Write your JavaScript code in the file. For example:
“`javascript
console.log(“Hello, World!”);
“`
- Save the file and exit the editor (in `nano`, press `CTRL + X`, then `Y`, and hit `Enter`).
Running the JavaScript File
Once you have your JavaScript file ready, you can execute it in the terminal using Node.js. Follow these steps:
- In your terminal, navigate to the directory containing your JavaScript file (if not already there).
- Run the following command:
“`
node myscript.js
“`
If your code contains a simple `console.log` statement, you should see the output in the terminal:
“`
Hello, World!
“`
Common Errors and Troubleshooting
When running JavaScript files in the terminal, you might encounter some common errors. Here are a few and their solutions:
Error Message | Description | Solution |
---|---|---|
`command not found: node` | Node.js is not installed or not added to your PATH. | Install Node.js or check your PATH settings. |
`SyntaxError: Unexpected token` | There is a syntax error in your JavaScript code. | Review the code for typos or incorrect syntax. |
`TypeError: is not a function` | You are trying to call a function that does not exist. | Verify that the function is defined correctly. |
By understanding these prerequisites, file creation steps, and potential errors, you can effectively run JavaScript files in the terminal environment, enhancing your development workflow.
Prerequisites for Running JavaScript in the Terminal
Before executing a JavaScript file in the terminal, ensure the following prerequisites are met:
- Node.js Installed: Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. To check if it’s installed:
“`bash
node -v
“`
If Node.js is not installed, download it from the [official Node.js website](https://nodejs.org/) and follow the installation instructions for your operating system.
- JavaScript File: Have a JavaScript file ready for execution. This file should have a `.js` extension. Example: `script.js`.
Running a JavaScript File
To run a JavaScript file, follow these steps:
- Open Terminal: Launch your terminal application.
- Navigate to the File Directory:
Use the `cd` command to change directories to where your JavaScript file is located. For example:
“`bash
cd path/to/your/directory
“`
- Execute the File: Run the JavaScript file using the Node.js command:
“`bash
node script.js
“`
- View Output: Any output generated by the JavaScript file will be displayed in the terminal.
Common Errors and Troubleshooting
While running JavaScript files in the terminal, you may encounter some common errors. Here are a few and their solutions:
Error Message | Description | Solution |
---|---|---|
`command not found: node` | Node.js is not installed or not found in PATH. | Install Node.js and ensure it’s in your PATH. |
`Error: Cannot find module` | The specified file cannot be found. | Check the file name and path for correctness. |
`SyntaxError: Unexpected token` | There is a syntax error in your JavaScript code. | Review your code for syntax issues. |
Additional Options for Running JavaScript
There are other ways to execute JavaScript files in the terminal, including:
- Using npm scripts: If your project has a `package.json` file, you can add scripts to run your JavaScript files. In your `package.json`, add:
“`json
“scripts”: {
“start”: “node script.js”
}
“`
Then execute the script using:
“`bash
npm run start
“`
- Using npx: For running a package without installing it globally, you can use `npx`:
“`bash
npx your-package
“`
- Interactive Node.js Shell: You can start an interactive Node.js shell by simply typing `node` in the terminal. This allows you to execute JavaScript statements directly.
To efficiently run JavaScript files in the terminal, ensure Node.js is installed, navigate to the directory containing your files, and execute them using the Node command. Familiarize yourself with common errors and alternative methods for a smoother experience.
Expert Insights on Running JavaScript Files in the Terminal
Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “To run a JavaScript file in the terminal, ensure you have Node.js installed. Simply navigate to the directory containing your file and execute the command ‘node yourfile.js’. This method is efficient and widely used in the industry for executing server-side scripts.”
James Patel (Lead Developer Advocate, CodeMaster Solutions). “Utilizing the terminal to run JavaScript files is a fundamental skill for developers. It allows for quick testing and debugging. I recommend familiarizing yourself with the Node.js REPL for interactive execution, which can enhance your coding workflow significantly.”
Sarah Thompson (Technical Writer, JavaScript Weekly). “Running JavaScript files in the terminal is not only straightforward but also crucial for modern web development. By mastering this process, developers can streamline their development cycles and improve productivity. Always check for the latest Node.js version to leverage new features and improvements.”
Frequently Asked Questions (FAQs)
How do I run a JavaScript file in the terminal?
To run a JavaScript file in the terminal, use Node.js. First, ensure Node.js is installed on your system. Then, navigate to the directory containing your JavaScript file and execute the command `node filename.js`, replacing `filename.js` with the name of your file.
What do I need to install to run JavaScript in the terminal?
You need to install Node.js, which includes the Node Package Manager (npm). This allows you to run JavaScript files directly in the terminal.
Can I run JavaScript files without Node.js?
Yes, you can run JavaScript files using a web browser’s developer console or by embedding the JavaScript code in an HTML file and opening it in a browser. However, for terminal execution, Node.js is required.
What is the difference between running JavaScript in a browser and in the terminal?
Running JavaScript in a browser executes the code in a web environment, allowing access to the Document Object Model (DOM) and browser-specific APIs. In contrast, running JavaScript in the terminal with Node.js executes server-side code, providing access to file systems and other backend functionalities.
Can I pass arguments to my JavaScript file when running it in the terminal?
Yes, you can pass arguments to your JavaScript file in the terminal. Use the command `node filename.js arg1 arg2`, and access the arguments in your code using `process.argv`.
What should I do if my JavaScript file doesn’t run in the terminal?
Ensure that Node.js is correctly installed and that you are in the correct directory where the JavaScript file is located. Additionally, check for syntax errors in your JavaScript code that may prevent execution.
In summary, running a JavaScript file in the terminal is a straightforward process that can be accomplished with the right tools and commands. The most common method involves using Node.js, a JavaScript runtime that allows developers to execute JavaScript code outside of a web browser. By installing Node.js and utilizing the command line interface, users can easily run their JavaScript files by navigating to the file’s directory and executing the command `node filename.js`.
Additionally, it is important to ensure that Node.js is correctly installed and that the terminal is configured to recognize the `node` command. Users should also be aware of potential issues such as file path errors or syntax errors in their JavaScript code, which can prevent successful execution. Familiarity with the terminal and basic command line operations will further streamline this process.
Ultimately, mastering the ability to run JavaScript files in the terminal not only enhances a developer’s workflow but also opens up opportunities for server-side programming and the creation of command-line tools. By leveraging Node.js, developers can harness the full power of JavaScript beyond traditional web applications, making it a valuable skill in today’s programming landscape.
Author Profile
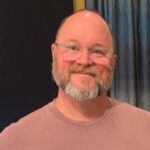
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?