How Can You Easily Replace an Item in a List Using Python?
In the world of Python programming, lists are one of the most versatile and widely used data structures. They allow you to store collections of items, from simple numbers to complex objects, and manipulate them with ease. However, as your data evolves, you may find yourself needing to modify these lists—whether it’s updating an item, replacing a value, or even changing the order of elements. Understanding how to effectively replace something in a list is a fundamental skill that can enhance your coding efficiency and flexibility.
In this article, we will explore the various methods available in Python for replacing elements within a list. From basic indexing techniques to more advanced methods using built-in functions, we’ll guide you through the process step-by-step. You’ll learn not only how to perform replacements but also when to choose one method over another, ensuring that your code remains clean and efficient.
Whether you’re a beginner looking to grasp the basics or an experienced programmer seeking to refine your skills, this guide will provide you with the insights you need to confidently manipulate lists in Python. Get ready to dive into the world of list replacement, where we’ll unlock the potential of this powerful data structure together!
Using the `list[index]` Method
One of the simplest ways to replace an item in a Python list is by directly accessing the item using its index. This method allows you to specify the exact position of the item you want to change.
For instance, consider the following example:
“`python
fruits = [‘apple’, ‘banana’, ‘cherry’]
fruits[1] = ‘orange’
print(fruits) Output: [‘apple’, ‘orange’, ‘cherry’]
“`
In this example, the item at index 1 (which is ‘banana’) is replaced with ‘orange’. This approach is straightforward but requires you to know the index of the item you wish to replace.
Using the `list.replace()` Method
Although lists do not have a built-in `replace()` method like strings, you can achieve similar functionality using list comprehensions or the `map()` function. This method is useful when you want to replace all occurrences of a specific item.
Here’s how you can implement it:
“`python
fruits = [‘apple’, ‘banana’, ‘banana’, ‘cherry’]
fruits = [‘orange’ if fruit == ‘banana’ else fruit for fruit in fruits]
print(fruits) Output: [‘apple’, ‘orange’, ‘orange’, ‘cherry’]
“`
This list comprehension iterates through each item in the list and replaces it with ‘orange’ if it is ‘banana’.
Using the `list.index()` Method
If you don’t know the index of the item you want to replace, you can find it using the `index()` method. This method returns the first index of the specified value.
Example:
“`python
fruits = [‘apple’, ‘banana’, ‘cherry’]
index = fruits.index(‘banana’)
fruits[index] = ‘kiwi’
print(fruits) Output: [‘apple’, ‘kiwi’, ‘cherry’]
“`
Keep in mind that if the item does not exist in the list, the `index()` method will raise a `ValueError`.
Replacing Multiple Items
If you need to replace multiple items, you can utilize a loop or a more advanced technique like a dictionary mapping. Here’s an example using a loop:
“`python
fruits = [‘apple’, ‘banana’, ‘cherry’, ‘banana’]
to_replace = {‘banana’: ‘kiwi’, ‘cherry’: ‘mango’}
for i in range(len(fruits)):
if fruits[i] in to_replace:
fruits[i] = to_replace[fruits[i]]
print(fruits) Output: [‘apple’, ‘kiwi’, ‘mango’, ‘kiwi’]
“`
Alternatively, a dictionary can allow for more complex replacements in a single pass.
Summary Table of Replacement Methods
Method | Description | Example |
---|---|---|
list[index] | Directly replace an item at a known index. | fruits[1] = ‘orange’ |
list comprehension | Replace all occurrences of a specific item. | [‘orange’ if fruit == ‘banana’ else fruit for fruit in fruits] |
list.index() | Find the index of an item and replace it. | fruits.index(‘banana’) |
Loop with dictionary | Replace multiple items efficiently. | for i in range(len(fruits)) |
Replacing Items in a List Using Indexing
In Python, lists are mutable, meaning you can change their contents after creation. One of the simplest methods to replace an item in a list is by using its index. The syntax is straightforward: `list[index] = new_value`.
For example:
“`python
my_list = [10, 20, 30, 40]
my_list[1] = 25 Replaces the value at index 1
print(my_list) Output: [10, 25, 30, 40]
“`
Using the `list` Method `replace()`
While lists in Python do not have a built-in `replace()` method, you can achieve similar results using a combination of list comprehension and the `enumerate()` function. This allows you to replace all occurrences of a specific value.
Example:
“`python
my_list = [‘apple’, ‘banana’, ‘apple’, ‘orange’]
my_list = [item if item != ‘apple’ else ‘grape’ for item in my_list]
print(my_list) Output: [‘grape’, ‘banana’, ‘grape’, ‘orange’]
“`
Using the `index()` Method for First Occurrence Replacement
To replace only the first occurrence of a value, you can utilize the `index()` method along with indexing. This method finds the index of the first occurrence of the specified value.
Example:
“`python
my_list = [‘apple’, ‘banana’, ‘apple’, ‘orange’]
first_index = my_list.index(‘apple’) Finds the index of the first ‘apple’
my_list[first_index] = ‘grape’ Replaces it with ‘grape’
print(my_list) Output: [‘grape’, ‘banana’, ‘apple’, ‘orange’]
“`
Replacing Multiple Items Conditionally
You can also replace items in a list based on certain conditions. This is often accomplished using list comprehensions.
Example:
“`python
my_list = [1, 2, 3, 4, 5]
my_list = [x * 2 if x % 2 == 0 else x for x in my_list]
print(my_list) Output: [1, 4, 3, 8, 5]
“`
Utilizing the `map()` Function
The `map()` function can also be used for replacing items in a list. This function applies a specified function to every item in the iterable.
Example:
“`python
def replace_even(x):
return x * 2 if x % 2 == 0 else x
my_list = [1, 2, 3, 4, 5]
my_list = list(map(replace_even, my_list))
print(my_list) Output: [1, 4, 3, 8, 5]
“`
Using a Loop for Custom Replacements
In some cases, you may want to use a standard loop for more complex replacement logic. This allows for greater flexibility.
Example:
“`python
my_list = [1, 2, 3, 4, 5]
for i in range(len(my_list)):
if my_list[i] % 2 == 0:
my_list[i] = my_list[i] * 2
print(my_list) Output: [1, 4, 3, 8, 5]
“`
Summary of Methods to Replace Items in a List
Method | Description |
---|---|
Indexing | Direct replacement using index |
List Comprehension | Replace all occurrences or based on conditions |
`index()` Method | Replace the first occurrence of a value |
`map()` Function | Apply a function to all items for replacement |
Loop | Custom logic for replacing items |
Expert Insights on Replacing Elements in Python Lists
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Replacing elements in a list in Python is straightforward using indexing. One can simply assign a new value to a specific index, but it is essential to ensure that the index is within the bounds of the list to avoid an IndexError.”
James Liu (Software Engineer, CodeCraft Solutions). “For more complex replacements, such as replacing all occurrences of a specific value, utilizing list comprehensions or the `map` function can be very efficient. This approach not only enhances readability but also maintains the immutability of the original list.”
Linda Patel (Python Educator, LearnPython Academy). “It is crucial for beginners to understand that lists in Python are mutable. Therefore, when replacing an item, one should always consider the implications on the list’s structure and ensure that the logic for replacement is sound to maintain data integrity.”
Frequently Asked Questions (FAQs)
How do I replace an element in a list by its index in Python?
To replace an element in a list by its index, use the assignment operator. For example, if you have a list `my_list` and want to replace the element at index `2`, you can do so with `my_list[2] = new_value`.
Can I replace multiple elements in a list at once in Python?
Yes, you can replace multiple elements in a list by using slicing. For example, `my_list[start:end] = [new_value1, new_value2]` will replace the elements from index `start` to `end – 1` with `new_value1` and `new_value2`.
What happens if I try to replace an index that is out of range?
If you attempt to replace an index that is out of range, Python will raise an `IndexError`. Ensure that the index you are trying to access exists within the bounds of the list.
Is there a method to replace all occurrences of a specific value in a list?
Yes, you can replace all occurrences of a specific value using a list comprehension. For example, `my_list = [new_value if x == old_value else x for x in my_list]` will replace all instances of `old_value` with `new_value`.
Can I use the `replace()` method on a list in Python?
No, the `replace()` method is not available for lists. It is a string method. To replace elements in a list, you must use indexing or list comprehensions as described above.
What is the best practice for replacing elements in a large list?
For large lists, consider using list comprehensions or the `map()` function for efficiency. These methods can provide better performance compared to iterative approaches, especially when dealing with large datasets.
In Python, replacing an item in a list is a straightforward process that can be accomplished using several methods. The most common approach involves directly accessing the list element by its index and assigning a new value to it. This method is efficient and allows for quick modifications. Additionally, Python provides list methods such as `list.replace()` for strings within lists, which can also be useful when dealing with specific scenarios involving string elements.
Another effective technique for replacing items is through list comprehensions, which can create a new list based on conditions applied to the original list. This method is particularly beneficial when you need to replace multiple occurrences of an item or when the replacement depends on certain criteria. Understanding these various methods allows for greater flexibility and efficiency when manipulating lists in Python.
In summary, knowing how to replace elements in a list is an essential skill for any Python programmer. By leveraging direct indexing, built-in methods, and list comprehensions, developers can efficiently manage and modify list contents. This knowledge not only enhances coding capabilities but also contributes to writing cleaner and more maintainable code.
Author Profile
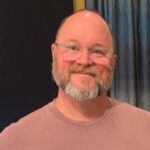
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?