How Can You Replace a Letter in a String Using Python?
In the world of programming, strings are one of the most fundamental data types, serving as the backbone for text manipulation and representation. Whether you’re developing a simple application or diving into complex data processing, knowing how to effectively manipulate strings is essential. One common task that programmers often encounter is the need to replace a specific letter or character within a string. This seemingly straightforward operation can unlock a plethora of possibilities, from correcting typos to transforming data formats. In this article, we will explore various methods to replace letters in strings using Python, a versatile and powerful programming language.
When it comes to replacing a letter in a string, Python offers a variety of techniques that cater to different needs and scenarios. From built-in string methods to more advanced approaches using regular expressions, each method has its own advantages and use cases. Understanding these options not only enhances your coding skills but also empowers you to write cleaner, more efficient code. As we delve into the intricacies of string manipulation, you’ll discover how to seamlessly integrate these techniques into your projects.
Whether you’re a beginner eager to grasp the basics or an experienced developer looking to refine your skills, this article will guide you through the essential concepts and practical applications of letter replacement in strings. Get ready to unlock the potential of Python’s string handling
Using the `replace()` Method
The simplest way to replace a letter in a string in Python is by utilizing the built-in `replace()` method. This method returns a new string with all occurrences of a specified substring replaced with another substring.
“`python
original_string = “Hello World”
new_string = original_string.replace(“o”, “a”)
print(new_string) Hella Warld
“`
Key features of the `replace()` method include:
- Case Sensitivity: The replacement is case-sensitive. For example, replacing “o” will not affect “O”.
- Count Parameter: You can specify the maximum number of occurrences to replace.
Example with count parameter:
“`python
original_string = “banana”
new_string = original_string.replace(“a”, “o”, 1)
print(new_string) bonana
“`
Using List Comprehension
List comprehension offers a more flexible approach to replace characters in a string. This method can be particularly useful when you need to apply complex conditions for replacements.
“`python
original_string = “Hello World”
new_string = ”.join([‘a’ if char == ‘o’ else char for char in original_string])
print(new_string) Hella Warld
“`
This technique involves iterating through each character in the original string, applying a condition to determine whether to replace the character or retain it.
Using Regular Expressions
For more advanced string manipulation, Python’s `re` module provides powerful tools for searching and replacing patterns in strings.
“`python
import re
original_string = “Hello World”
new_string = re.sub(r’o’, ‘e’, original_string)
print(new_string) Helle Werld
“`
The `re.sub()` function takes the following parameters:
- Pattern: The substring to search for.
- Replacement: The string to replace with.
- String: The original string to perform the replacement on.
Replacement Summary Table
Method | Use Case | Example Code |
---|---|---|
replace() | Simple character replacement | original_string.replace(“o”, “a”) |
List Comprehension | Conditional replacements | ”.join([‘a’ if char == ‘o’ else char for char in original_string]) |
Regular Expressions | Pattern-based replacements | re.sub(r’o’, ‘e’, original_string) |
By selecting the appropriate method for replacing letters in a string, you can achieve efficient and effective string manipulation in Python, tailored to your specific needs.
Methods to Replace a Letter in a String in Python
In Python, there are several methods to replace a letter in a string. Each method has its own use cases and advantages depending on the requirements of the task.
Using the `str.replace()` Method
The `replace()` method is a straightforward way to replace occurrences of a specified substring with another substring.
“`python
original_string = “hello world”
modified_string = original_string.replace(“o”, “a”)
print(modified_string) Output: “hella warld”
“`
- Syntax: `str.replace(old, new, count)`
- `old`: The substring to be replaced.
- `new`: The substring to replace with.
- `count` (optional): The number of occurrences to replace.
This method returns a new string and does not modify the original string.
Using String Slicing
String slicing allows for more control over the replacement process, especially when you need to replace a letter at a specific position.
“`python
original_string = “hello”
index_to_replace = 1
new_letter = “a”
modified_string = original_string[:index_to_replace] + new_letter + original_string[index_to_replace + 1:]
print(modified_string) Output: “hallo”
“`
- Explanation:
- `original_string[:index_to_replace]`: Gets the substring before the specified index.
- `new_letter`: The new letter to insert.
- `original_string[index_to_replace + 1:]`: Gets the substring after the specified index.
This method is useful for single character replacements at known positions.
Using List Comprehension
List comprehension provides a flexible way to replace letters while iterating through the string. This method is particularly effective if you want to apply more complex conditions.
“`python
original_string = “hello”
modified_string = ”.join([‘a’ if char == ‘o’ else char for char in original_string])
print(modified_string) Output: “hella”
“`
- Explanation:
- The list comprehension iterates through each character in the string.
- If the character matches the target letter (`’o’`), it replaces it with `’a’`; otherwise, it keeps the original character.
- `join()` method combines the list back into a string.
Using Regular Expressions
For more advanced replacements, especially when patterns need to be matched, the `re` module can be utilized.
“`python
import re
original_string = “hello world”
modified_string = re.sub(r’o’, ‘a’, original_string)
print(modified_string) Output: “hella warld”
“`
- Explanation:
- `re.sub(pattern, repl, string)` replaces occurrences of `pattern` with `repl` in the `string`.
- This is particularly useful for replacing all instances of a letter or letters that match a specific pattern.
Comparison of Methods
Method | In-Place Modification | Complexity | Use Case |
---|---|---|---|
`str.replace()` | No | O(n) | Simple replacements |
String Slicing | No | O(n) | Specific index replacements |
List Comprehension | No | O(n) | Condition-based replacements |
Regular Expressions | No | O(n) | Pattern matching and complex replacements |
Choosing the appropriate method depends on the specific requirements of your task, such as the need for simple replacements versus more complex pattern matching. Each method offers flexibility and efficiency suited for different scenarios.
Expert Insights on Replacing Letters in Strings with Python
Dr. Emily Carter (Senior Software Engineer, Code Innovators Inc.). “Replacing a letter in a string using Python can be efficiently achieved with the `str.replace()` method. This built-in function not only simplifies the process but also enhances code readability, making it a preferred choice for developers.”
James Lin (Python Developer and Educator, Tech Academy). “While `str.replace()` is straightforward, I often recommend using list comprehensions for more complex scenarios. This approach allows for greater control over the replacement conditions, especially when dealing with multiple replacements or specific character cases.”
Sarah Thompson (Data Scientist, Analytics Hub). “In data manipulation tasks, understanding how to replace letters in strings is crucial. I suggest combining the `replace()` method with regular expressions for advanced string operations, which can handle patterns and multiple occurrences seamlessly.”
Frequently Asked Questions (FAQs)
How can I replace a specific letter in a string in Python?
You can use the `str.replace()` method to replace a specific letter in a string. For example, `my_string.replace(‘old_letter’, ‘new_letter’)` will replace all occurrences of ‘old_letter’ with ‘new_letter’.
Is it possible to replace only the first occurrence of a letter in a string?
Yes, you can limit the replacement to the first occurrence by using the `str.replace()` method with a third argument. For instance, `my_string.replace(‘old_letter’, ‘new_letter’, 1)` replaces only the first occurrence.
Can I replace multiple letters in a string at once in Python?
Yes, you can replace multiple letters by chaining the `replace()` method or using a loop. For example: `my_string.replace(‘a’, ‘b’).replace(‘c’, ‘d’)` replaces ‘a’ with ‘b’ and ‘c’ with ‘d’.
What if I want to replace a letter based on a condition?
You can use a list comprehension or the `str.join()` method along with a generator expression. For example: `”.join(‘new_letter’ if char == ‘old_letter’ else char for char in my_string)` replaces ‘old_letter’ based on the specified condition.
Are there any built-in libraries that can help with string manipulation in Python?
Yes, the `re` module provides powerful regular expression capabilities for advanced string manipulation, including conditional replacements. You can use `re.sub(pattern, replacement, string)` for more complex scenarios.
What should I do if I need to replace letters in a case-insensitive manner?
You can use the `re` module with the `re.IGNORECASE` flag. For example: `re.sub(‘old_letter’, ‘new_letter’, my_string, flags=re.IGNORECASE)` replaces ‘old_letter’ regardless of its case.
In Python, replacing a letter in a string is a straightforward process that can be accomplished using the built-in string method called `replace()`. This method allows you to specify the target character you wish to replace and the new character that will take its place. The syntax is simple: `string.replace(old, new)`, where `old` is the character to be replaced and `new` is the character that will replace it. This method returns a new string with all occurrences of the specified character replaced, leaving the original string unchanged.
Another approach to replace a letter in a string is by using string slicing and concatenation. This method is particularly useful when you want to replace a letter at a specific index. By slicing the string before and after the target index, you can concatenate the segments with the new character in between. This method provides greater control over the exact position of the replacement but requires more lines of code compared to the `replace()` method.
In summary, Python offers multiple methods to replace letters in strings, catering to different needs and preferences. The `replace()` method is the most efficient for replacing all occurrences of a character, while string slicing provides flexibility for targeted replacements. Understanding these techniques enhances your ability to manipulate strings
Author Profile
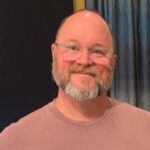
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?