How Can You Effectively Use Repetition in Python Programming?
In the world of programming, repetition is a fundamental concept that can streamline processes, enhance efficiency, and reduce the likelihood of errors. Whether you’re automating tasks, iterating through data, or simply performing a set of operations multiple times, knowing how to repeat actions effectively is crucial. Python, renowned for its simplicity and readability, offers a variety of tools and techniques to help you master the art of repetition. In this article, we will explore the various methods Python provides for repeating tasks, empowering you to write cleaner and more efficient code.
Repetition in Python can be achieved through several constructs, each tailored for different scenarios and use cases. From loops that allow you to iterate over sequences to built-in functions that can execute tasks multiple times, Python’s versatility ensures that you have the right tool for the job. Understanding these mechanisms not only enhances your programming skills but also opens the door to more complex problem-solving strategies.
As we delve into this topic, we will uncover the nuances of using loops, such as `for` and `while`, alongside the power of list comprehensions and built-in functions like `map` and `filter`. By the end of this exploration, you will have a comprehensive understanding of how to effectively implement repetition in your Python programs, making your
Using Loops to Repeat Actions
In Python, the most common way to repeat actions is through loops, primarily the `for` loop and the `while` loop. Each of these constructs provides a method to execute a block of code multiple times based on a specified condition or sequence.
The `for` loop is typically used to iterate over a sequence, such as a list or a string. Its structure allows you to easily access each item in the sequence, enabling straightforward and readable code. Here is a basic example:
“`python
for item in [‘apple’, ‘banana’, ‘cherry’]:
print(item)
“`
This code will output each fruit on a new line. The loop runs for each element in the list, performing the action defined in the loop body.
On the other hand, the `while` loop continues to execute as long as a specified condition is true. This is useful when the number of iterations is not predetermined. Here’s an example:
“`python
count = 0
while count < 5:
print(count)
count += 1
```
This will print numbers from 0 to 4. The loop checks the condition (`count < 5`) before each iteration, ensuring that the loop only runs while the condition holds true.
Using the `range()` Function
The `range()` function is particularly useful when you need to repeat an action a specific number of times. It generates a sequence of numbers that can be iterated over in a loop. The syntax is as follows:
“`python
range(start, stop, step)
“`
- start: The starting value of the sequence (default is 0).
- stop: The stopping value (exclusive).
- step: The increment (default is 1).
Here’s an example of using `range()` with a `for` loop:
“`python
for i in range(5):
print(i)
“`
This will output numbers from 0 to 4. You can also customize the start and step values:
“`python
for i in range(1, 10, 2):
print(i)
“`
This code will print odd numbers from 1 to 9.
Repeating Code with Functions
Another effective way to repeat code in Python is through functions. By defining a function, you can encapsulate code into a reusable block that can be called multiple times throughout your program.
Here’s an example of a simple function that prints a message:
“`python
def repeat_message(message, times):
for _ in range(times):
print(message)
repeat_message(“Hello, World!”, 3)
“`
This will print “Hello, World!” three times. Functions provide not only code repetition but also better organization and clarity in your code.
Table of Loop Types
Loop Type | Use Case | Example |
---|---|---|
for loop | Iterate over a sequence (list, string, etc.) | for item in [1, 2, 3]: print(item) |
while loop | Repeat until a condition is | while x < 10: x += 1 |
range() | Generate a sequence of numbers for iteration | for i in range(5): print(i) |
Using Loops for Repetition
In Python, loops are a fundamental way to repeat actions. The two primary types of loops are `for` loops and `while` loops.
For Loops
A `for` loop iterates over a sequence, such as a list or a string. It is particularly useful when the number of iterations is known ahead of time.
“`python
Example of a for loop
fruits = [“apple”, “banana”, “cherry”]
for fruit in fruits:
print(fruit)
“`
- Structure:
- `for variable in iterable:`
- The `variable` takes the value of each item in the iterable on each iteration.
While Loops
A `while` loop continues to execute as long as a specified condition is `True`. This is useful when the number of iterations is not predetermined.
“`python
Example of a while loop
count = 0
while count < 5:
print(count)
count += 1
```
- Structure:
- `while condition:`
- The loop will terminate when the condition evaluates to “.
Using List Comprehensions
List comprehensions provide a concise way to create lists and can be seen as a shorthand for creating loops.
“`python
Example of a list comprehension
squares = [x**2 for x in range(10)]
print(squares)
“`
- Structure:
- `[expression for item in iterable if condition]`
- This creates a new list based on the expression for each item in the iterable.
Repeating Actions with Functions
Functions can encapsulate repetitive tasks and can be called multiple times throughout your code.
“`python
Example of a function
def repeat_message(message, times):
for _ in range(times):
print(message)
repeat_message(“Hello, World!”, 3)
“`
- Structure:
- `def function_name(parameters):`
- The function can be invoked with different arguments to repeat specific actions.
Using the `itertools` Module
The `itertools` module provides functions that create iterators for efficient looping. The `cycle()` and `repeat()` functions are particularly useful for repetition.
“`python
import itertools
Example of itertools.cycle
colors = itertools.cycle([‘red’, ‘green’, ‘blue’])
for _ in range(6):
print(next(colors))
Example of itertools.repeat
for num in itertools.repeat(5, 3):
print(num)
“`
- Functionality:
- `itertools.cycle(iterable)`: Repeats the elements of the iterable indefinitely.
- `itertools.repeat(object, times)`: Repeats the specified object a given number of times.
Using the `time.sleep()` Function
When repetition includes waiting periods, the `time` module can be utilized to pause execution.
“`python
import time
for i in range(5):
print(i)
time.sleep(1) Pauses for 1 second
“`
- Structure:
- `time.sleep(seconds)`: Pauses the program for the specified number of seconds, allowing for timed repetition.
Expert Insights on Repeating Actions in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, repeating actions can be efficiently achieved using loops such as ‘for’ and ‘while’. These constructs allow developers to execute a block of code multiple times based on a defined condition or over a sequence, making them fundamental to effective programming in Python.”
Michael Zhang (Python Developer Advocate, OpenSource Community). “Understanding how to repeat tasks in Python is crucial for automation and data processing. Utilizing functions in combination with loops not only enhances code readability but also promotes reusability, which is a best practice in software development.”
Lisa Tran (Data Scientist, Analytics Hub). “When dealing with large datasets, Python’s ability to repeat operations through list comprehensions and generator expressions can significantly improve performance. These methods allow for concise and efficient iteration, which is essential for data manipulation and analysis.”
Frequently Asked Questions (FAQs)
How can I repeat a string multiple times in Python?
You can repeat a string in Python using the multiplication operator `*`. For example, `repeated_string = “Hello” * 3` results in `repeated_string` being `”HelloHelloHello”`.
What is the purpose of the `for` loop in repeating actions in Python?
The `for` loop allows you to execute a block of code multiple times. For instance, `for i in range(5): print(“Hello”)` will print “Hello” five times.
Can I use the `while` loop to repeat actions in Python?
Yes, the `while` loop can be used to repeat actions until a certain condition is met. For example, `count = 0; while count < 5: print("Hello"); count += 1` will print "Hello" five times.
How do I repeat a list of items in Python?
You can repeat a list using the multiplication operator as well. For instance, `repeated_list = [1, 2, 3] * 2` results in `repeated_list` being `[1, 2, 3, 1, 2, 3]`.
Is there a built-in function to repeat elements in Python?
Python does not have a specific built-in function for repeating elements, but you can use list comprehensions or the `itertools` module’s `cycle` function for more complex repetition patterns.
What is the difference between `*` and `itertools.repeat()` for repetition?
The `*` operator creates a new list or string with repeated elements, while `itertools.repeat()` returns an iterator that generates repeated elements on demand, which can be more memory efficient for large repetitions.
In Python, repeating elements or sequences can be accomplished through various methods, each suited to different contexts and requirements. The most common approaches include using loops, list comprehensions, and the `*` operator for lists and strings. For instance, a `for` loop can iterate over a range or a collection, allowing for repeated execution of a block of code. List comprehensions provide a concise way to generate lists by applying an expression to each item in an iterable, effectively repeating an operation across multiple elements.
Additionally, the `*` operator offers a straightforward technique for repeating sequences. By multiplying a list or string by an integer, you can create a new sequence that contains the original repeated multiple times. This method is particularly useful for creating repeated patterns or initializing lists with default values. Understanding these various methods enables Python developers to choose the most efficient and readable approach for their specific use cases.
Overall, mastering the techniques for repeating elements in Python enhances code efficiency and readability. By leveraging loops, list comprehensions, and the `*` operator, developers can effectively manage repetitive tasks and streamline their coding processes. As Python continues to evolve, staying informed about these fundamental concepts will remain essential for both novice and experienced programmers alike.
Author Profile
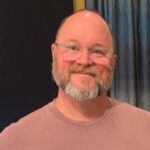
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?