How Can You Remove a Character from a String in Python?
When working with strings in Python, you may find yourself needing to manipulate text data for various reasons—whether it’s cleaning up user input, formatting output, or processing data for analysis. One common task is removing unwanted characters from strings, which can seem daunting at first but is quite manageable with the right techniques. In this article, we will explore the different methods available in Python to effectively remove characters from strings, empowering you to refine your text data with ease and precision.
Python offers a rich set of tools for string manipulation, making it a versatile choice for developers and data scientists alike. From built-in string methods to powerful regular expressions, there are numerous ways to approach the task of character removal. Depending on your specific needs, you can choose from straightforward techniques that are perfect for quick fixes or more complex solutions that allow for advanced pattern matching and character filtering.
Understanding how to efficiently remove characters from strings not only enhances your coding skills but also improves the quality of your data processing tasks. Whether you’re cleaning up a messy dataset or simply trying to format a string for display, mastering these techniques will enable you to handle strings like a pro. So, let’s dive into the various methods and discover how you can manipulate strings in Python to achieve your desired results!
Using the Replace Method
The `replace()` method in Python is one of the most straightforward ways to remove specific characters from a string. This method allows you to specify the character you want to remove and replaces it with an empty string.
python
original_string = “Hello, World!”
modified_string = original_string.replace(“,”, “”)
print(modified_string) # Output: Hello World!
In this example, the comma is removed from the original string. You can use this method to remove any character by specifying it in the first argument.
Using String Comprehension
String comprehension offers a flexible way to filter out unwanted characters. This approach is particularly useful when you want to remove multiple characters or create more complex conditions.
python
original_string = “Hello, World!”
characters_to_remove = “,!”
modified_string = ”.join(char for char in original_string if char not in characters_to_remove)
print(modified_string) # Output: Hello World
In this code snippet, a new string is built by including only those characters that are not in the `characters_to_remove` list.
Using Regular Expressions
For more advanced string manipulation, the `re` module provides powerful tools to remove characters using regular expressions. This is especially useful when you need to remove characters based on patterns rather than exact matches.
python
import re
original_string = “Hello, World!”
modified_string = re.sub(r'[,!]’, ”, original_string)
print(modified_string) # Output: Hello World
This method utilizes a regular expression to identify and remove commas and exclamation marks from the original string.
Using the Translate Method
The `translate()` method, combined with the `str.maketrans()` function, allows for efficient removal of multiple characters in a single operation. This method is particularly fast and optimal for larger strings.
python
original_string = “Hello, World!”
remove_chars = “,!”
translation_table = str.maketrans(”, ”, remove_chars)
modified_string = original_string.translate(translation_table)
print(modified_string) # Output: Hello World
In this method, the `str.maketrans()` function creates a translation table where characters to be removed are specified.
Comparison of Methods
The table below summarizes the different methods for removing characters from strings, highlighting their advantages and use cases.
Method | Advantages | Use Case |
---|---|---|
replace() | Simple and straightforward | Removing known single characters |
String Comprehension | Flexible and customizable | Removing multiple characters based on conditions |
Regular Expressions | Powerful pattern matching | Complex removal based on patterns |
translate() | Efficient for large strings | Removing multiple characters quickly |
By understanding these methods, you can choose the most appropriate technique for your specific string manipulation needs in Python.
Methods to Remove Characters from a String in Python
In Python, there are several efficient methods to remove specific characters or groups of characters from a string. Each method serves different use cases, allowing flexibility depending on your needs. Below are the most common methods.
Using the `replace()` Method
The `replace()` method is straightforward and is used to replace occurrences of a specified substring with another substring. To remove a character, you can replace it with an empty string.
python
original_string = “Hello, World!”
modified_string = original_string.replace(“o”, “”)
print(modified_string) # Output: Hell, Wrld!
Using the `str.translate()` Method
The `translate()` method combined with `str.maketrans()` is powerful for removing multiple characters efficiently. This method is particularly useful when you want to remove several characters at once.
python
original_string = “Hello, World!”
remove_chars = “lo”
translation_table = str.maketrans(”, ”, remove_chars)
modified_string = original_string.translate(translation_table)
print(modified_string) # Output: He, Wr!
Using List Comprehension
List comprehension provides a concise way to create a new string by filtering out unwanted characters. This method offers high readability.
python
original_string = “Hello, World!”
modified_string = ”.join([char for char in original_string if char not in “lo”])
print(modified_string) # Output: He, Wr!
Using Regular Expressions
The `re` module allows for more complex character removal, especially useful when dealing with patterns.
python
import re
original_string = “Hello, World!”
modified_string = re.sub(r”[lo]”, “”, original_string)
print(modified_string) # Output: He, Wr!
Using Slicing
If you need to remove characters from specific positions in the string, slicing can be a practical solution. However, this method is less flexible for arbitrary character removal.
python
original_string = “Hello, World!”
modified_string = original_string[:2] + original_string[3:] # Remove character at index 2
print(modified_string) # Output: Helo, World!
Comparison Table of Methods
Method | Use Case | Example |
---|---|---|
replace() | Single character removal | original_string.replace(“o”, “”) |
translate() | Multiple character removal | original_string.translate(str.maketrans(”, ”, ‘lo’)) |
List Comprehension | Readability and filtering | ”.join([c for c in original_string if c not in ‘lo’]) |
Regular Expressions | Pattern-based removal | re.sub(r”[lo]”, “”, original_string) |
Slicing | Removing at specific positions | original_string[:2] + original_string[3:] |
Expert Insights on Removing Characters from Strings in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “When removing characters from strings in Python, utilizing the `str.replace()` method is often the most straightforward approach. It allows for the replacement of specified characters with an empty string, effectively removing them from the original string.”
Mark Thompson (Data Scientist, Analytics Innovators). “For more complex scenarios, such as removing multiple different characters, using regular expressions with the `re.sub()` function can be highly effective. This method provides flexibility and precision in character removal.”
Linda Nguyen (Python Developer, Tech Solutions Inc.). “Another efficient way to remove characters is through list comprehensions. By iterating over the string and selectively including characters that do not match the ones to be removed, developers can create a new string without the unwanted characters.”
Frequently Asked Questions (FAQs)
How can I remove a specific character from a string in Python?
You can remove a specific character from a string using the `replace()` method. For example, to remove the character ‘a’, you would use `string.replace(‘a’, ”)`.
Is there a way to remove multiple characters from a string in Python?
Yes, you can use the `str.translate()` method along with `str.maketrans()`. For example, to remove characters ‘a’ and ‘b’, you can use `string.translate(str.maketrans(”, ”, ‘ab’))`.
Can I remove characters by their index in a string?
Yes, you can remove characters by their index using slicing. For instance, to remove the character at index 2, you can concatenate the parts of the string before and after that index: `string[:2] + string[3:]`.
What is the difference between `replace()` and `translate()` for removing characters?
The `replace()` method is straightforward for removing specific characters, while `translate()` is more efficient for removing multiple characters at once, as it processes the string in a single pass.
Are there any built-in functions in Python for character removal?
Python does not have a dedicated built-in function solely for character removal, but methods like `replace()`, `translate()`, and slicing can effectively achieve this task.
Can I remove whitespace characters from a string in Python?
Yes, you can remove whitespace characters using the `str.replace()` method or `str.strip()` to remove leading and trailing whitespace. To remove all whitespace, use `string.replace(‘ ‘, ”)` or `”.join(string.split())`.
In Python, removing characters from a string can be accomplished through various methods, each suited to different use cases. The most common techniques include using the `replace()` method, list comprehensions, and regular expressions. The `replace()` method is straightforward for removing specific characters, while list comprehensions provide a more flexible approach for filtering out multiple characters based on conditions. Regular expressions, on the other hand, offer powerful pattern matching capabilities for more complex string manipulations.
When choosing a method for character removal, it is essential to consider the context and requirements of the task. For simple replacements, `replace()` is efficient and easy to implement. However, if the goal is to remove multiple characters or apply specific filtering criteria, list comprehensions or the `filter()` function may yield better results. Regular expressions are ideal for more intricate patterns, allowing for advanced string processing that can handle a variety of scenarios.
In summary, Python provides a robust set of tools for removing characters from strings, enabling developers to select the most appropriate method based on their specific needs. Understanding the strengths and limitations of each approach will enhance string manipulation capabilities and improve code efficiency. By leveraging these techniques effectively, programmers can ensure cleaner and more manageable string data in their applications.
Author Profile
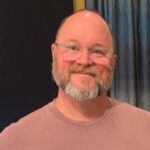
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?