How Can You Read a File Line by Line in Python?
Reading files is a fundamental skill for anyone venturing into the world of programming, and Python makes this task remarkably straightforward. Whether you’re analyzing data, processing logs, or simply extracting information from text files, knowing how to read a file line by line can significantly enhance your coding efficiency and effectiveness. This technique not only allows for better memory management but also provides a more granular control over file contents, making it easier to manipulate and analyze data as needed.
In Python, reading a file line by line can be accomplished using various methods, each with its own advantages. For instance, using a loop to iterate through each line can help you handle large files without overwhelming your system’s memory. Additionally, Python’s built-in functions and context management features streamline the process, ensuring that files are opened and closed properly. This is particularly useful in preventing data loss or corruption, which can occur if files are not handled correctly.
As we delve deeper into the specifics of reading files in Python, you’ll discover practical examples and best practices that will empower you to tackle file handling tasks with confidence. From understanding file modes to exploring different reading techniques, this guide will equip you with the tools you need to master file input and output in your Python projects. Whether you’re a beginner or looking to refine your skills, the ability
Using the `for` Loop
One of the most straightforward ways to read a file line by line in Python is by utilizing a `for` loop. This method automatically handles the iteration over each line, making your code cleaner and more readable. Here’s how it works:
“`python
with open(‘file.txt’, ‘r’) as file:
for line in file:
print(line.strip())
“`
In this example, the `with` statement ensures that the file is properly closed after its suite finishes, even if an exception is raised. The `strip()` function is used to remove any leading or trailing whitespace, including newline characters.
Using `readline()` Method
Another method is to use the `readline()` function, which reads one line at a time. This approach gives you more control over the reading process, allowing you to implement logic that can manipulate how lines are processed:
“`python
with open(‘file.txt’, ‘r’) as file:
while True:
line = file.readline()
if not line: Break the loop if line is empty
break
print(line.strip())
“`
In this snippet, the loop continues until `readline()` returns an empty string, indicating that the end of the file has been reached.
Using `readlines()` Method
The `readlines()` method reads all the lines in a file and returns them as a list. This can be useful if you need to process the lines collectively. Here’s an example:
“`python
with open(‘file.txt’, ‘r’) as file:
lines = file.readlines()
for line in lines:
print(line.strip())
“`
This method is beneficial when you want to manipulate the lines as a batch, but keep in mind that it loads the entire file into memory, which may not be optimal for large files.
Performance Considerations
When reading files, consider the following performance aspects:
- Memory Usage: Using `for` or `readline()` is memory efficient since lines are read one at a time.
- File Size: For very large files, prefer `for` or `readline()` to avoid excessive memory consumption.
- Processing Speed: Batch processing with `readlines()` might be faster for small files, but it can slow down significantly with larger files due to memory overhead.
Method | Memory Efficiency | Speed | Use Case |
---|---|---|---|
`for` Loop | High | Moderate | General line processing |
`readline()` | High | Moderate | Conditional reading |
`readlines()` | Low | Fast (small) | Small files, batch processing |
Understanding these methods allows for efficient file handling in Python, enabling developers to choose the best approach based on their specific needs and constraints. Whether your focus is on performance, memory management, or simplicity, Python provides flexible options for reading files line by line.
Using the `with` Statement
Utilizing the `with` statement is a preferred method for reading files in Python. This approach ensures that files are properly closed after their suite finishes, preventing resource leaks and potential file corruption. The syntax is straightforward and promotes cleaner code.
“`python
with open(‘file.txt’, ‘r’) as file:
for line in file:
print(line.strip())
“`
This snippet reads a file named `file.txt` line by line. The `strip()` method removes any leading or trailing whitespace, including the newline character.
Reading All Lines into a List
If you need to process all lines at once, you can read the entire file into a list. This method can be useful for applications where you need to access lines multiple times.
“`python
with open(‘file.txt’, ‘r’) as file:
lines = file.readlines()
for line in lines:
print(line.strip())
“`
Here, `readlines()` reads all lines into a list, allowing you to iterate through them later.
Using a Loop with `readline()`
The `readline()` method reads one line at a time from the file, which can be useful when working with large files to minimize memory usage.
“`python
with open(‘file.txt’, ‘r’) as file:
while True:
line = file.readline()
if not line:
break
print(line.strip())
“`
This code continues reading lines until there are no more lines left, effectively processing each line individually.
File Iterators
Python’s file objects are iterable, allowing for a concise and efficient way to read lines without explicitly calling a method. This method is both memory efficient and easy to read.
“`python
with open(‘file.txt’, ‘r’) as file:
for line in file:
print(line.strip())
“`
This approach leverages the inherent iterable nature of file objects, making it an elegant solution for line-by-line reading.
Handling Exceptions
When working with files, it’s crucial to handle potential exceptions that may arise, such as file not found errors or permission issues. Using a try-except block can help manage these situations effectively.
“`python
try:
with open(‘file.txt’, ‘r’) as file:
for line in file:
print(line.strip())
except FileNotFoundError:
print(“The file was not found.”)
except IOError:
print(“An error occurred while reading the file.”)
“`
This example provides a robust way to handle errors, ensuring that the program can continue running smoothly even when encountering issues.
Performance Considerations
When reading large files, consider the following performance tips:
- Buffered I/O: Python’s default file I/O is buffered, which optimizes read performance.
- Memory Usage: Use `readline()` or iterate over the file object to minimize memory overhead.
- File Size: For very large files, consider processing lines in chunks or using more advanced libraries like `pandas` for data manipulation.
By understanding these strategies, you can effectively read files line by line in Python while optimizing for performance and resource management.
Expert Insights on Reading Files Line by Line in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Reading files line by line in Python is a fundamental skill for any developer. Using the built-in `open()` function with a context manager ensures that files are properly handled and closed, which is crucial for resource management.”
Mark Thompson (Python Educator and Author, CodeMaster Publications). “A common approach to reading files line by line is utilizing the `for` loop directly on the file object. This not only simplifies the code but also enhances readability, making it easier for beginners to grasp file handling in Python.”
Lisa Chang (Data Scientist, Analytics Solutions Group). “When dealing with large files, it is advisable to read files line by line to conserve memory. The `readline()` method can be particularly useful in such scenarios, allowing for efficient processing of each line without loading the entire file into memory.”
Frequently Asked Questions (FAQs)
How can I read a file line by line in Python?
You can read a file line by line in Python using the `with` statement and the `open()` function. For example:
“`python
with open(‘file.txt’, ‘r’) as file:
for line in file:
print(line.strip())
“`
What is the advantage of using the `with` statement when reading files?
The `with` statement ensures that the file is properly closed after its suite finishes, even if an error occurs. This helps prevent resource leaks and ensures better file handling.
Can I read a file line by line without loading the entire file into memory?
Yes, reading a file line by line inherently avoids loading the entire file into memory. Python reads one line at a time, making it efficient for large files.
What method can I use to read all lines at once?
You can use the `readlines()` method to read all lines at once into a list. For example:
“`python
with open(‘file.txt’, ‘r’) as file:
lines = file.readlines()
“`
Is there a way to read specific lines from a file in Python?
Yes, you can read specific lines by iterating through the file and using a counter to track the current line number. Alternatively, you can read all lines into a list and access the desired indices.
What should I do if the file does not exist?
You should handle the `FileNotFoundError` exception using a `try` and `except` block. This allows your program to gracefully manage the error without crashing. For example:
“`python
try:
with open(‘file.txt’, ‘r’) as file:
for line in file:
print(line.strip())
except FileNotFoundError:
print(“The file does not exist.”)
“`
Reading a file line by line in Python is a fundamental skill that enhances a programmer’s ability to handle data efficiently. The most common methods for achieving this include using the built-in `open()` function in conjunction with a for loop, the `readline()` method, and the `readlines()` method. Each of these methods serves specific use cases, allowing for flexibility depending on the size of the file and the requirements of the program.
One of the most efficient and Pythonic ways to read a file line by line is by utilizing a for loop directly on the file object. This method automatically handles the opening and closing of the file, making it both concise and safe. Additionally, using the `with` statement is highly recommended, as it ensures that resources are managed properly and that the file is closed automatically after its block of code has been executed.
Another important takeaway is the consideration of memory usage when dealing with large files. Reading a file line by line prevents loading the entire file into memory at once, which can be crucial for performance. Understanding these methods and their implications allows developers to write more efficient and scalable Python applications, particularly when processing large datasets or logs.
Author Profile
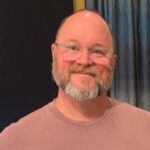
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?