How Can You Effectively Print Out an Array in Java?
When diving into the world of Java programming, one of the fundamental skills every developer must master is the ability to manipulate and display data effectively. Arrays, which serve as a powerful structure for storing multiple values in a single variable, are a cornerstone of Java’s data handling capabilities. However, simply creating an array is just the beginning; knowing how to print out an array can significantly enhance your ability to debug, visualize, and present data in your applications. Whether you’re a novice coder or a seasoned programmer looking to brush up on your skills, understanding the nuances of array printing in Java is essential for effective coding.
In Java, printing an array is not as straightforward as it might seem at first glance. While it may be tempting to use a simple print statement, arrays require a more nuanced approach to display their contents in a readable format. From using loops to iterate through the elements, to employing utility methods that simplify the process, there are various techniques at your disposal. Each method has its own advantages and can be chosen based on the specific needs of your project.
Moreover, the way you present array data can greatly influence the clarity of your output. Whether you’re working on a console application or preparing data for a graphical user interface, knowing how to format your array output can make a significant difference
Using Arrays.toString()
The simplest way to print an array in Java is by utilizing the `Arrays.toString()` method from the `java.util.Arrays` class. This method provides a straightforward means of converting the array into a human-readable format, which is particularly useful for one-dimensional arrays.
java
import java.util.Arrays;
public class PrintArrayExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(numbers));
}
}
This code snippet will output:
[1, 2, 3, 4, 5]
Printing Multi-Dimensional Arrays
To print multi-dimensional arrays, such as two-dimensional arrays, `Arrays.deepToString()` is the appropriate method to use. It recursively converts the array into a string representation.
java
import java.util.Arrays;
public class PrintMultiDimensionalArray {
public static void main(String[] args) {
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
System.out.println(Arrays.deepToString(matrix));
}
}
The output will be:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Using a Loop to Print Elements
For more control over formatting, you can manually iterate through the array using loops. This method allows for customized output but requires additional coding.
java
public class PrintArrayWithLoop {
public static void main(String[] args) {
String[] fruits = {“Apple”, “Banana”, “Cherry”};
for (String fruit : fruits) {
System.out.print(fruit + ” “);
}
}
}
The output will be:
Apple Banana Cherry
Formatting Output with StringBuilder
For complex formatting, consider using `StringBuilder`. This approach enables you to build the string representation of the array incrementally.
java
public class PrintArrayWithStringBuilder {
public static void main(String[] args) {
double[] scores = {92.5, 85.0, 76.5};
StringBuilder sb = new StringBuilder(“[“);
for (int i = 0; i < scores.length; i++) {
sb.append(scores[i]);
if (i < scores.length - 1) {
sb.append(", ");
}
}
sb.append("]");
System.out.println(sb.toString());
}
}
The output will be:
[92.5, 85.0, 76.5]
Choosing the Right Method
When deciding how to print an array, consider the following factors:
Method | Best Use Case |
---|---|
Arrays.toString() | One-dimensional arrays |
Arrays.deepToString() | Multi-dimensional arrays |
Looping | Custom formatting or complex structures |
StringBuilder | Performance-sensitive applications requiring formatted output |
These methods provide a variety of options depending on the complexity of the array and the desired output format. Select the method that best fits your specific requirements for clarity and maintainability.
Using Arrays.toString() for One-Dimensional Arrays
To print a one-dimensional array in Java, the most straightforward method is to use the `Arrays.toString()` method from the `java.util.Arrays` class. This method provides a clean and readable format for the output.
Example code snippet:
java
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(numbers));
}
}
Output:
[1, 2, 3, 4, 5]
Using Arrays.deepToString() for Multi-Dimensional Arrays
For multi-dimensional arrays, `Arrays.deepToString()` is the recommended approach. This method can handle nested arrays and provides a readable representation.
Example code snippet:
java
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[][] matrix = {{1, 2, 3}, {4, 5, 6}};
System.out.println(Arrays.deepToString(matrix));
}
}
Output:
[[1, 2, 3], [4, 5, 6]]
Using a Loop for Custom Formatting
In cases where more control over the formatting is required, a simple loop can be used. This approach allows for custom separators or formatting styles.
Example code snippet for a one-dimensional array:
java
public class Main {
public static void main(String[] args) {
String[] fruits = {“Apple”, “Banana”, “Cherry”};
for (String fruit : fruits) {
System.out.print(fruit + ” “);
}
}
}
Output:
Apple Banana Cherry
Example code snippet for a multi-dimensional array:
java
public class Main {
public static void main(String[] args) {
String[][] grid = {{“A”, “B”}, {“C”, “D”}};
for (String[] row : grid) {
for (String item : row) {
System.out.print(item + ” “);
}
System.out.println();
}
}
}
Output:
A B
C D
Using Streams for Modern Java (Java 8 and Later)
Java 8 introduced Streams, which can be leveraged to print arrays in a more functional programming style. This approach is particularly useful for collections.
Example code snippet for a one-dimensional array:
java
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
double[] values = {1.1, 2.2, 3.3};
Arrays.stream(values).forEach(value -> System.out.print(value + ” “));
}
}
Output:
1.1 2.2 3.3
Example code snippet for a multi-dimensional array:
java
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
String[][] words = {{“Hello”, “World”}, {“Java”, “Streams”}};
Arrays.stream(words)
.flatMap(Arrays::stream)
.forEach(word -> System.out.print(word + ” “));
}
}
Output:
Hello World Java Streams
Using StringBuilder for Efficient Concatenation
When concatenating large arrays, using `StringBuilder` can enhance performance by reducing the number of temporary string objects created.
Example code snippet:
java
public class Main {
public static void main(String[] args) {
char[] letters = {‘J’, ‘a’, ‘v’, ‘a’};
StringBuilder sb = new StringBuilder();
for (char letter : letters) {
sb.append(letter);
}
System.out.println(sb.toString());
}
}
Output:
Java
Expert Perspectives on Printing Arrays in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Printing an array in Java can be efficiently accomplished using the Arrays class. Utilizing the Arrays.toString() method provides a straightforward way to output the contents of one-dimensional arrays, while Arrays.deepToString() is ideal for multi-dimensional arrays.”
Michael Chen (Java Developer Advocate, CodeCraft Solutions). “For developers looking to customize their output, implementing a loop to iterate through the array elements offers flexibility. This allows for formatting the output according to specific requirements, such as aligning numbers or adding delimiters.”
Sarah Thompson (Lead Instructor, Java Programming Academy). “Understanding the nuances of printing arrays is crucial for effective debugging. Using System.out.println() in conjunction with a loop can help visualize the data structure, making it easier to identify issues during development.”
Frequently Asked Questions (FAQs)
How do I print an array in Java?
To print an array in Java, you can use the `Arrays.toString()` method for one-dimensional arrays or `Arrays.deepToString()` for multi-dimensional arrays. For example:
java
int[] array = {1, 2, 3};
System.out.println(Arrays.toString(array));
What is the best way to print each element of an array in a loop?
You can iterate through the array using a `for` loop or an enhanced `for` loop. For example:
java
for (int element : array) {
System.out.println(element);
}
Can I print an array without using loops?
Yes, you can print an array without explicit loops by utilizing `Arrays.toString()` or `Arrays.deepToString()`, which handle the iteration internally.
What happens if I try to print an uninitialized array?
Attempting to print an uninitialized array will result in a `NullPointerException`. Ensure the array is initialized before printing.
Is there a way to format the output when printing an array?
Yes, you can format the output using `String.format()` or `System.out.printf()`. For instance:
java
for (int i = 0; i < array.length; i++) {
System.out.printf("Element %d: %d%n", i, array[i]);
}
How can I print a 2D array in Java?
To print a 2D array, use `Arrays.deepToString()` for a concise representation. Example:
java
int[][] matrix = {{1, 2}, {3, 4}};
System.out.println(Arrays.deepToString(matrix));
printing out an array in Java is a fundamental skill that every Java programmer should master. The process can be accomplished through various methods, each suited to different scenarios. The most common approaches include using loops, such as for-loops and enhanced for-loops, as well as utilizing built-in utility methods from the Java Standard Library, like `Arrays.toString()` for one-dimensional arrays and `Arrays.deepToString()` for multi-dimensional arrays. Understanding these methods allows developers to efficiently display array contents for debugging and data presentation purposes.
Moreover, the choice of method depends on the specific requirements of the task at hand. For instance, when dealing with simple one-dimensional arrays, using a loop may provide more flexibility for formatting output. In contrast, the `Arrays.toString()` method offers a quick and concise way to print array contents without the need for manual iteration. Additionally, recognizing the difference between one-dimensional and multi-dimensional arrays is crucial, as it influences the choice of printing method.
Ultimately, mastering the techniques for printing arrays not only enhances a programmer’s ability to visualize data but also contributes to more effective debugging practices. By leveraging the appropriate methods, developers can ensure their code is both efficient and easy to read, which is essential
Author Profile
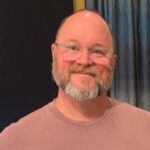
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?