How Can You Effectively Print to the Console in JavaScript?
In the vast world of web development, understanding how to effectively communicate with your code is essential. One of the most fundamental yet powerful tools at your disposal is the console. Whether you’re debugging, testing, or simply trying to understand how your code behaves, knowing how to print to the console in JavaScript can significantly enhance your coding experience. This article will guide you through the nuances of console output, empowering you to harness this tool to its fullest potential.
Printing to the console in JavaScript is not just about displaying messages; it’s about gaining insights into your code’s execution and state. From simple log statements that help track the flow of your program to more advanced techniques that allow for structured data visualization, the console serves as a versatile companion in your development journey. As you delve deeper, you’ll discover how to leverage various console methods to improve your debugging skills and streamline your workflow.
Moreover, understanding how to print to the console can also enhance collaboration with other developers. By effectively communicating the status of your code through well-placed console outputs, you can make your intentions clear, making it easier for others to follow your logic. In this article, we will explore the different ways to interact with the console, providing you with the tools you need to elevate your JavaScript programming and
Using console.log()
The primary method for printing output to the console in JavaScript is through the `console.log()` function. This method allows developers to display information, which is particularly useful for debugging purposes. The syntax is straightforward:
“`javascript
console.log(value);
“`
You can pass any value to `console.log()`, including:
- Strings
- Numbers
- Arrays
- Objects
- Boolean values
For example:
“`javascript
console.log(“Hello, World!”);
console.log(42);
console.log([1, 2, 3]);
console.log({ name: “Alice”, age: 30 });
“`
Each of these commands will print the respective data type to the console.
Other Console Methods
In addition to `console.log()`, JavaScript provides several other methods for different logging purposes. These include:
- `console.error()`: Displays error messages.
- `console.warn()`: Issues warning messages.
- `console.info()`: Provides informational messages.
- `console.table()`: Displays tabular data in a readable format.
Here is a quick comparison of these methods:
Method | Description | Example |
---|---|---|
console.log() | General logging | console.log(“Log message”); |
console.error() | Log an error | console.error(“Error message”); |
console.warn() | Log a warning | console.warn(“Warning message”); |
console.info() | Log informational messages | console.info(“Info message”); |
console.table() | Display data in a table format | console.table([{name: “Alice”, age: 30}, {name: “Bob”, age: 25}]); |
Formatting Console Output
JavaScript allows for formatted output in the console, making it easier to present complex data or highlight specific information. You can use placeholders in your strings, similar to other programming languages. The syntax for formatted output is:
“`javascript
console.log(“My name is %s and I am %d years old.”, “Alice”, 30);
“`
In this example:
- `%s` is a placeholder for a string.
- `%d` is a placeholder for a number.
JavaScript supports several formatting specifiers, including:
- `%s`: String
- `%d`: Number (integer)
- `%f`: Floating point number
- `%o`: Object
- `%c`: CSS styling for the output
Example:
“`javascript
console.log(“%cThis is a styled message”, “color: blue; font-size: 16px;”);
“`
This command will print the message in blue with a specified font size.
Logging Objects and Arrays
When logging objects and arrays, the `console.log()` method will display them in a structured format, allowing for easy inspection of their properties. Additionally, `console.table()` is a powerful method that presents arrays or objects in a tabular format, enhancing readability.
To log an array:
“`javascript
const fruits = [“Apple”, “Banana”, “Cherry”];
console.table(fruits);
“`
For an array of objects:
“`javascript
const users = [
{ name: “Alice”, age: 30 },
{ name: “Bob”, age: 25 }
];
console.table(users);
“`
This will provide a clear, organized view of the data, making it easier to analyze.
By employing these methods effectively, developers can enhance their debugging process and improve the overall development experience in JavaScript.
Understanding the Console Object
The console object in JavaScript provides access to the browser’s debugging console. It is primarily used for logging information, which can help during development and debugging processes.
Key methods of the console object include:
- `console.log()`: Outputs a message to the console.
- `console.error()`: Outputs an error message to the console.
- `console.warn()`: Outputs a warning message to the console.
- `console.info()`: Outputs an informational message to the console.
- `console.debug()`: Outputs a debug message to the console, typically useful for development.
Using console.log() to Print Messages
The most common method for printing to the console is `console.log()`. This method can take multiple arguments, which can be of any type.
Example usage:
“`javascript
console.log(“Hello, World!”);
console.log(“The value of x is:”, x);
“`
This will print “Hello, World!” and the value of `x` to the console.
Formatting Console Output
You can format the output in the console using string interpolation and template literals. This is particularly useful for creating readable logs.
Example:
“`javascript
let name = “John”;
let age = 30;
console.log(`Name: ${name}, Age: ${age}`);
“`
Additionally, you can use `%` placeholders for string substitution:
“`javascript
console.log(“Name: %s, Age: %d”, name, age);
“`
Logging Different Data Types
The console can handle various data types, which can be crucial when debugging complex applications. Here’s how to log different types:
Data Type | Example |
---|---|
String | `console.log(“This is a string”);` |
Number | `console.log(42);` |
Boolean | `console.log(true);` |
Object | `console.log({ key: “value” });` |
Array | `console.log([1, 2, 3]);` |
Advanced Console Methods
Beyond basic logging, the console object includes advanced methods for enhanced debugging capabilities.
- console.table(): Displays tabular data as a table.
Example:
“`javascript
const people = [
{ name: “Alice”, age: 25 },
{ name: “Bob”, age: 30 }
];
console.table(people);
“`
- console.group(): Starts a new group in the console, allowing for better organization of logs.
Example:
“`javascript
console.group(“User Info”);
console.log(“Name: Alice”);
console.log(“Age: 25”);
console.groupEnd();
“`
- console.time() and console.timeEnd(): Used to measure the time taken to execute a block of code.
Example:
“`javascript
console.time(“MyTimer”);
// Code to measure
console.timeEnd(“MyTimer”);
“`
Conditional Logging
Sometimes you may want to log messages based on certain conditions. This can be achieved using simple if statements.
Example:
“`javascript
let debugMode = true;
if (debugMode) {
console.log(“Debugging is enabled.”);
}
“`
This will print the debug message only if `debugMode` is true.
Expert Insights on Printing to Console in JavaScript
Jane Thompson (Senior JavaScript Developer, CodeCraft Inc.). “Using console.log() is fundamental for debugging in JavaScript. It allows developers to output messages, variables, and objects to the console, making it easier to track the flow of data and identify issues in real-time.”
Michael Chen (Lead Software Engineer, Tech Innovations). “Beyond just printing simple messages, developers can utilize console.table() to display arrays and objects in a more readable tabular format. This enhances the debugging process, especially when dealing with complex data structures.”
Emily Rodriguez (JavaScript Educator, LearnCode Academy). “It is crucial to remember that console methods can vary in their output. For instance, console.warn() and console.error() provide different visual cues in the console, which can be beneficial for highlighting issues versus standard log messages.”
Frequently Asked Questions (FAQs)
How do I print a message to the console in JavaScript?
You can print a message to the console in JavaScript using the `console.log()` method. For example, `console.log(“Hello, World!”);` will output “Hello, World!” to the console.
What are the different console methods available in JavaScript?
JavaScript provides several console methods, including `console.log()`, `console.error()`, `console.warn()`, `console.info()`, and `console.table()`. Each method serves a specific purpose, such as logging errors or displaying tabular data.
Can I print variables to the console in JavaScript?
Yes, you can print variables to the console by passing them as arguments to `console.log()`. For instance, `let x = 10; console.log(x);` will display the value of `x`, which is 10.
Is it possible to format output in the console?
Yes, you can format output in the console using placeholders. For example, `console.log(“My name is %s and I am %d years old.”, “Alice”, 25);` will format the string with the specified values.
How can I clear the console in JavaScript?
You can clear the console by using the `console.clear()` method. This will remove all previous messages from the console, providing a clean slate for new logs.
What is the difference between console.log() and console.error()?
`console.log()` is used for general output, while `console.error()` is specifically for logging error messages. The latter typically displays the message in red, making it easier to identify issues during debugging.
printing to the console in JavaScript is a fundamental skill that enhances debugging and logging capabilities for developers. The primary method for outputting data to the console is through the `console.log()` function, which can accept multiple arguments and various data types, including strings, numbers, objects, and arrays. This versatility allows developers to effectively monitor the flow of their code and inspect variable states at different execution points.
Additionally, JavaScript offers several other console methods, such as `console.error()`, `console.warn()`, and `console.info()`, each serving specific purposes for logging different types of messages. These methods help categorize console output, making it easier to identify issues or warnings during development. Understanding how to use these functions can significantly improve the efficiency of the debugging process.
Moreover, leveraging the console’s features, such as grouping messages with `console.group()` and timing code execution with `console.time()`, can provide deeper insights into application performance and behavior. By mastering these techniques, developers can create a more organized and informative console output, ultimately leading to better code quality and more efficient troubleshooting.
Author Profile
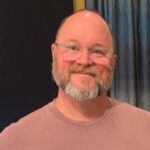
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?