How Can You Easily Print Column Names in SQL?
In the world of databases, understanding the structure of your data is crucial for effective management and analysis. One of the fundamental aspects of working with SQL is being able to identify and manipulate the various components of your tables, with column names being a key focus. Whether you are a seasoned database administrator or a budding data analyst, knowing how to print column names in SQL can enhance your ability to query and interpret data efficiently. This article will guide you through the essential techniques and commands that will empower you to extract and utilize column names in your SQL queries, paving the way for more sophisticated data operations.
When you dive into SQL, you quickly realize that column names serve as the identifiers for the data you are working with. They not only provide context but also play a significant role in how you construct your queries. Printing column names can be particularly useful for understanding the schema of a table, especially when dealing with large datasets or unfamiliar databases. This foundational skill allows you to verify the structure of your data before executing more complex queries, ensuring that you are working with the correct fields.
Moreover, mastering the ability to print column names opens up a world of possibilities for data exploration and manipulation. It sets the stage for more advanced SQL techniques, such as dynamic queries and automated reporting.
Using Information Schema
One of the most effective ways to retrieve column names in SQL is by querying the Information Schema. This schema provides metadata about all the objects in the database, including tables and their respective columns. The query to list column names is as follows:
“`sql
SELECT COLUMN_NAME
FROM INFORMATION_SCHEMA.COLUMNS
WHERE TABLE_NAME = ‘your_table_name’;
“`
Replace `your_table_name` with the actual name of the table you are interested in. This will return a list of all column names for that specific table.
Using SQL Server System Views
For SQL Server users, another approach is to use the system view `sys.columns`. The following SQL statement will list all column names for a specified table:
“`sql
SELECT name
FROM sys.columns
WHERE object_id = OBJECT_ID(‘your_table_name’);
“`
This query retrieves the column names directly from the system catalog, ensuring you have the most accurate information.
Retrieving Column Names in MySQL
In MySQL, you can utilize the `SHOW COLUMNS` command to obtain column names along with additional metadata such as data type and nullability. Here’s how you can use it:
“`sql
SHOW COLUMNS FROM your_table_name;
“`
The output will display a table with the following columns:
Field | Type | Null | Key | Default | Extra |
---|---|---|---|---|---|
column_name | data_type | YES/NO | Key info | default_value | additional_info |
This method is straightforward and provides a comprehensive overview of the table structure.
PostgreSQL Column Names Retrieval
In PostgreSQL, you can achieve similar results using the `pg_catalog` schema. The following query will return column names for a specified table:
“`sql
SELECT column_name
FROM pg_catalog.pg_columns
WHERE table_name = ‘your_table_name’;
“`
This query efficiently retrieves column names from the specified table, allowing for easy access to the schema details.
Using SQLite to Get Column Names
SQLite provides a unique way to list column names using the `PRAGMA table_info` command. Here’s how you can use it:
“`sql
PRAGMA table_info(your_table_name);
“`
This command will return a result set containing details about each column, including the name, type, and whether it can be null.
Dynamic SQL for Retrieving Column Names
In scenarios where you need to retrieve column names dynamically, you can construct a SQL query that can adapt to different table names. For instance, in SQL Server, you might use:
“`sql
DECLARE @tableName NVARCHAR(256) = ‘your_table_name’;
EXEC(‘SELECT COLUMN_NAME FROM INFORMATION_SCHEMA.COLUMNS WHERE TABLE_NAME = ”’ + @tableName + ””);
“`
This dynamic approach is useful in stored procedures or scripts where the table name may change frequently.
Each of these methods allows database administrators and developers to easily access and manage schema information, ensuring efficient database operations.
Using Information Schema to Retrieve Column Names
To obtain column names in SQL, one of the most common methods is to query the `INFORMATION_SCHEMA`. This schema provides access to database metadata, including details about tables and columns. The following SQL query retrieves the column names for a specific table:
“`sql
SELECT COLUMN_NAME
FROM INFORMATION_SCHEMA.COLUMNS
WHERE TABLE_NAME = ‘your_table_name’
AND TABLE_SCHEMA = ‘your_schema_name’;
“`
Parameters:
- TABLE_NAME: Replace `’your_table_name’` with the name of the table whose columns you want to list.
- TABLE_SCHEMA: Specify the schema name where the table resides, such as `’dbo’` for the default schema in SQL Server.
This query returns a list of column names for the specified table.
Using SQL Server System Views
In SQL Server, you can also use system views to get column names. The `sys.columns` and `sys.tables` views can be combined for this purpose. Here’s how you can execute this:
“`sql
SELECT c.name AS ColumnName
FROM sys.columns c
JOIN sys.tables t ON c.object_id = t.object_id
WHERE t.name = ‘your_table_name’;
“`
Key Components:
- sys.columns: Contains a row for each column in the database.
- sys.tables: Contains a row for each user-defined table.
Using this method allows for additional filtering options if needed.
Retrieving Column Names in MySQL
In MySQL, you can use the `SHOW COLUMNS` command to easily list the column names of a table. The syntax is straightforward:
“`sql
SHOW COLUMNS FROM your_table_name;
“`
Output:
The output will include:
- Field: Name of the column
- Type: Data type of the column
- Null: Whether the column can contain NULL values
- Key: Key information
- Default: Default value for the column
- Extra: Additional information (e.g., auto-increment)
This command provides a comprehensive view of the table structure along with column names.
PostgreSQL Approach
In PostgreSQL, you can use the following SQL query to retrieve column names:
“`sql
SELECT column_name
FROM information_schema.columns
WHERE table_name = ‘your_table_name’;
“`
Additional Filtering:
- You can also filter by schema or database if necessary.
- Use `table_schema` to specify the schema.
Oracle SQL Column Name Retrieval
For Oracle databases, the `USER_TAB_COLUMNS` view can be queried to get column names:
“`sql
SELECT COLUMN_NAME
FROM USER_TAB_COLUMNS
WHERE TABLE_NAME = ‘YOUR_TABLE_NAME’;
“`
Important Note:
- Ensure that the table name is in uppercase, as Oracle stores object names in uppercase by default unless specified otherwise.
This method efficiently retrieves the list of column names for the specified table.
Summary of Commands Across Databases
Database | Command |
---|---|
SQL Server | `SELECT COLUMN_NAME FROM INFORMATION_SCHEMA.COLUMNS WHERE TABLE_NAME = ‘your_table_name’;` |
MySQL | `SHOW COLUMNS FROM your_table_name;` |
PostgreSQL | `SELECT column_name FROM information_schema.columns WHERE table_name = ‘your_table_name’;` |
Oracle | `SELECT COLUMN_NAME FROM USER_TAB_COLUMNS WHERE TABLE_NAME = ‘YOUR_TABLE_NAME’;` |
These commands provide a quick reference for retrieving column names in various SQL databases.
Expert Insights on Printing Column Names in SQL
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “Printing column names in SQL is a fundamental task that can significantly enhance data readability. Utilizing commands like ‘SELECT column_name FROM information_schema.columns’ allows developers to dynamically retrieve column names, which is particularly useful in large databases.”
James Liu (Senior SQL Developer, Data Solutions Group). “Understanding how to print column names is crucial for database management. Using the ‘SHOW COLUMNS FROM table_name’ command in MySQL, for instance, provides a straightforward approach to list all columns, ensuring that developers can efficiently navigate their database schemas.”
Maria Gonzalez (Data Analyst, Insights Analytics). “For those working with SQL Server, the ‘SELECT COLUMN_NAME FROM INFORMATION_SCHEMA.COLUMNS WHERE TABLE_NAME = ‘your_table_name” query is invaluable. It not only returns the column names but also allows for filtering based on specific tables, which is essential for maintaining organized data structures.”
Frequently Asked Questions (FAQs)
How can I print column names in SQL Server?
You can print column names in SQL Server using the `INFORMATION_SCHEMA.COLUMNS` view. Execute the query:
“`sql
SELECT COLUMN_NAME FROM INFORMATION_SCHEMA.COLUMNS WHERE TABLE_NAME = ‘your_table_name’;
“`
What SQL command retrieves column names for a specific table?
To retrieve column names for a specific table, use the following SQL command:
“`sql
SELECT COLUMN_NAME FROM INFORMATION_SCHEMA.COLUMNS WHERE TABLE_NAME = ‘your_table_name’;
“`
Is there a way to print column names in MySQL?
Yes, in MySQL, you can print column names using the `SHOW COLUMNS` command:
“`sql
SHOW COLUMNS FROM your_table_name;
“`
How do I list column names in PostgreSQL?
In PostgreSQL, you can list column names using the `information_schema` or `pg_catalog`:
“`sql
SELECT column_name FROM information_schema.columns WHERE table_name = ‘your_table_name’;
“`
Can I get column names using a stored procedure?
Yes, you can create a stored procedure that queries `INFORMATION_SCHEMA.COLUMNS` or equivalent system tables to return column names dynamically.
What is the difference between using INFORMATION_SCHEMA and system catalogs?
`INFORMATION_SCHEMA` provides a standardized way to access metadata across different SQL databases, while system catalogs are specific to each database system and may offer more detailed information.
In SQL, printing column names is an essential task that can aid in understanding the structure of a database table. Various methods exist to achieve this, depending on the SQL database management system in use. Common approaches include querying system catalogs or information schema views, which provide metadata about the database objects, including tables and their respective columns. For instance, in MySQL, one can use the `SHOW COLUMNS FROM table_name` command, while in PostgreSQL, the query `SELECT column_name FROM information_schema.columns WHERE table_name = ‘your_table_name’` serves the same purpose.
Additionally, leveraging programming languages that interface with SQL, such as Python or R, can also be effective for retrieving and displaying column names. Libraries like Pandas in Python allow users to easily extract and manipulate data frames, including accessing column names directly. This flexibility can enhance productivity, especially when dealing with large datasets or complex queries.
Ultimately, understanding how to print column names in SQL not only aids in database navigation but also enhances data management practices. By utilizing the appropriate commands or programming techniques, users can efficiently gather necessary information about their database schema, facilitating better data analysis and reporting. This knowledge is particularly valuable for database administrators, analysts, and developers working with
Author Profile
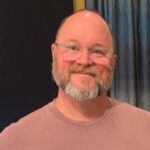
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?