How Can You Print an ArrayList in Java Effectively?
In the world of Java programming, mastering data structures is essential for efficient coding and application development. One of the most versatile and widely used collections in Java is the ArrayList. This dynamic array not only allows for the storage of elements but also provides the flexibility to grow and shrink as needed. However, as you dive deeper into working with ArrayLists, you may find yourself asking: how can I effectively print the contents of my ArrayList? Whether you’re debugging your code or simply want to display data to users, understanding the various methods to print an ArrayList is a crucial skill for any Java developer.
Printing an ArrayList in Java might seem straightforward, but it encompasses several approaches that can enhance your coding efficiency and readability. From the basic methods that utilize loops to more advanced techniques that leverage Java’s built-in functions, each method serves a unique purpose depending on the context of your application. Moreover, knowing how to format the output can significantly improve the user experience, making your data not only accessible but also visually appealing.
As we explore the different ways to print an ArrayList, you’ll discover practical examples and best practices that can be applied in real-world scenarios. Whether you’re a novice programmer looking to grasp the fundamentals or an experienced developer seeking to refine your skills, this
Using the `toString()` Method
One of the simplest ways to print an ArrayList in Java is by utilizing the `toString()` method that is inherent to the Object class. The ArrayList class overrides this method to provide a string representation of the list elements.
Here’s how you can use it:
“`java
import java.util.ArrayList;
public class PrintArrayList {
public static void main(String[] args) {
ArrayList
list.add(“Apple”);
list.add(“Banana”);
list.add(“Cherry”);
System.out.println(list.toString());
}
}
“`
The output will be:
“`
[Apple, Banana, Cherry]
“`
This method is straightforward and provides a clean format for displaying the elements of the ArrayList.
Using a Loop to Print Elements
In cases where you require more control over the output format, you can iterate over the ArrayList using loops. Both the enhanced for-loop and the traditional for-loop can be employed.
Enhanced For-Loop Example:
“`java
for (String fruit : list) {
System.out.println(fruit);
}
“`
Traditional For-Loop Example:
“`java
for (int i = 0; i < list.size(); i++) {
System.out.println(list.get(i));
}
```
Both methods will output the list elements line by line:
```
Apple
Banana
Cherry
```
Using Streams for Printing
Java 8 introduced the Stream API, which provides a more functional approach to processing collections. You can leverage this API to print an ArrayList in a concise manner.
“`java
list.stream().forEach(System.out::println);
“`
This line effectively prints each element in the ArrayList, similar to the loop methods, but in a more elegant fashion.
Custom Formatting with String Join
If you need a specific format for the output, the `String.join()` method can be utilized. This allows you to concatenate the elements of the ArrayList into a single string with a specified delimiter.
“`java
String result = String.join(“, “, list);
System.out.println(result);
“`
The output will be:
“`
Apple, Banana, Cherry
“`
Comparative Overview of Printing Methods
The following table summarizes the different methods for printing an ArrayList:
Method | Description | Output Format |
---|---|---|
toString() | Default string representation of the list. | [Apple, Banana, Cherry] |
Enhanced For-Loop | Iterates through each element. | Apple Banana Cherry |
Traditional For-Loop | Accesses elements by index. | Apple Banana Cherry |
Streams | Functional approach to print elements. | Apple Banana Cherry |
String Join | Concatenates elements with a delimiter. | Apple, Banana, Cherry |
Each method has its unique advantages and can be selected based on the specific requirements of your application.
Using the `toString()` Method
The simplest way to print an `ArrayList` in Java is by utilizing its built-in `toString()` method. This method returns a string representation of the list, which includes all elements in a readable format.
“`java
ArrayList
list.add(“Apple”);
list.add(“Banana”);
list.add(“Cherry”);
System.out.println(list.toString());
“`
This will produce the output:
“`
[Apple, Banana, Cherry]
“`
Iterating with a Loop
For more control over the output format, you can iterate through the `ArrayList` using a loop. This method allows for custom formatting or additional operations during the printing process.
- Using a For-Each Loop:
“`java
for (String fruit : list) {
System.out.println(fruit);
}
“`
- Using a Traditional For Loop:
“`java
for (int i = 0; i < list.size(); i++) {
System.out.println(list.get(i));
}
```
Using Java Streams
Java 8 introduced the Stream API, which allows for a more functional approach to manipulating collections. You can use streams to print an `ArrayList` efficiently.
“`java
list.stream().forEach(System.out::println);
“`
This will output each element on a new line, similar to the loop methods described earlier.
Custom Formatting with `String.join()`
If you prefer a custom separator between elements, the `String.join()` method can be particularly useful. This method joins the elements of the list into a single string with a specified delimiter.
“`java
String result = String.join(“, “, list);
System.out.println(result);
“`
Output:
“`
Apple, Banana, Cherry
“`
Using a Formatter
Java’s `Formatter` class can also be used to achieve formatted output. This method is particularly useful when formatting complex data types.
“`java
Formatter formatter = new Formatter();
for (String fruit : list) {
formatter.format(“%s%n”, fruit);
}
System.out.print(formatter.toString());
formatter.close();
“`
This approach provides flexibility in formatting, such as alignment and padding.
Displaying in a Table Format
For a more structured output, especially with larger datasets, presenting an `ArrayList` in a table format can be beneficial. You can achieve this by manually creating a table structure.
“`java
System.out.printf(“%-10s%n”, “Fruits”);
System.out.println(“—————-“);
for (String fruit : list) {
System.out.printf(“%-10s%n”, fruit);
}
“`
This will create a simple table-like structure in the console. The `%-10s` format specifier ensures that each fruit is left-aligned within a field of width 10.
Printing an `ArrayList` in Java can be done in various ways, each suited to different needs. Whether leveraging built-in methods, loops, or custom formatting, the approach chosen can enhance clarity and usability in your applications.
Expert Insights on Printing ArrayLists in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Printing an ArrayList in Java can be done efficiently using the `System.out.println()` method, which automatically calls the `toString()` method of the ArrayList. This is a straightforward approach, but for more complex data structures, implementing a custom `toString()` method can enhance readability and provide better insights into the object’s state.”
Michael Chen (Java Development Consultant, CodeCraft Solutions). “When printing an ArrayList, consider using the enhanced for loop to iterate through the elements. This not only makes the code cleaner but also improves performance when dealing with large datasets. Additionally, using `String.join()` can help format the output more elegantly if you need a specific delimiter between elements.”
Sarah Patel (Lead Java Instructor, Code Academy). “For beginners, understanding how to print an ArrayList is crucial. I always recommend starting with the basic `System.out.println()` method, then progressing to more advanced techniques like using streams for filtering and formatting. This progression helps solidify their understanding of Java collections and enhances their programming skills.”
Frequently Asked Questions (FAQs)
How do I print an ArrayList in Java?
To print an ArrayList in Java, you can use the `System.out.println()` method directly on the ArrayList instance. This will invoke the `toString()` method of the ArrayList, displaying its elements in a readable format.
Can I print the elements of an ArrayList one by one?
Yes, you can print the elements of an ArrayList one by one using a loop. For example, you can use a for-each loop to iterate through the ArrayList and print each element individually.
What is the output format when printing an ArrayList?
The output format of an ArrayList when printed is a comma-separated list of its elements enclosed in square brackets. For example, printing an ArrayList containing integers will look like `[1, 2, 3, 4]`.
Is there a way to format the output when printing an ArrayList?
Yes, you can format the output by iterating through the ArrayList and using `String.format()` or `StringBuilder` to customize how each element is displayed before printing.
Can I print an ArrayList of objects?
Yes, you can print an ArrayList of objects. However, ensure that the objects in the ArrayList override the `toString()` method to provide meaningful output. Otherwise, the default implementation will print the class name and hash code.
What happens if the ArrayList is empty when printed?
If an empty ArrayList is printed, the output will be `[]`, indicating that there are no elements within the ArrayList.
In summary, printing an ArrayList in Java can be accomplished through several methods, each serving different needs and preferences. The most straightforward approach is to use the built-in `System.out.println()` method, which automatically invokes the `toString()` method of the ArrayList, providing a readable string representation of its elements. Additionally, developers can utilize loops, such as the enhanced for-loop or traditional for-loop, to iterate through the elements and print them individually, allowing for more control over the output format.
Another effective technique involves using Java Streams, which can simplify the process of printing elements while also enabling the application of additional operations, such as filtering or mapping. This modern approach not only enhances code readability but also aligns with functional programming principles, making it a preferred choice for many developers. Furthermore, utilizing the `forEach()` method of the ArrayList can provide a clean and concise way to print elements while leveraging lambda expressions for added flexibility.
Ultimately, the choice of method for printing an ArrayList in Java depends on the specific requirements of the task at hand and the developer’s coding style. Understanding these various techniques allows developers to select the most appropriate and efficient method for their needs, thereby improving code quality and maintainability. Mastery of
Author Profile
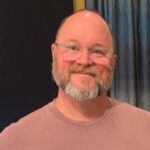
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?