How Can You Effectively Prevent Circular Imports in Python?
In the world of Python programming, the elegance of your code can sometimes be overshadowed by the complexities of its structure. One common pitfall that developers encounter is the dreaded circular import. This issue arises when two or more modules attempt to import each other, leading to confusion and errors that can halt your project in its tracks. Understanding how to prevent circular imports is essential for maintaining clean, efficient, and functional code. In this article, we will explore the nature of circular imports, their implications, and effective strategies to avoid them, ensuring your Python projects remain robust and error-free.
Circular imports can create a tangled web of dependencies that not only complicate your code but also introduce runtime errors that can be difficult to diagnose. When two modules rely on each other, Python may struggle to resolve the order of imports, leading to incomplete initialization and unexpected behavior. This challenge is particularly common in larger projects where modules are interdependent, making it crucial for developers to adopt best practices in module design and organization.
To tackle the issue of circular imports, developers must first recognize the signs and understand the underlying mechanics of Python’s import system. By employing strategies such as restructuring code, utilizing import statements wisely, and embracing design patterns that promote modularity, you can significantly reduce the likelihood of
Understanding Circular Imports
Circular imports occur when two or more modules attempt to import each other directly or indirectly. This can lead to issues where the Python interpreter is unable to resolve the dependencies, resulting in `ImportError`. Understanding the underlying causes of circular imports can help in preventing them effectively.
The primary reasons for circular imports include:
- Interdependent modules: When two modules require each other for functionality.
- Single-file imports: Importing classes or functions from the same file in a way that creates a loop.
- Misplaced imports: Import statements placed in the wrong scope, such as within a function.
Strategies to Prevent Circular Imports
To avoid circular imports in Python, consider the following strategies:
- Reorganize Code Structure: Place related classes and functions in a single module or create a new module to house shared functionality.
- Use Import Statements Wisely: Import modules only when necessary, preferably within functions or methods to limit their scope and avoid premature execution.
- Refactor Code: Break down large modules into smaller, more cohesive components that can operate independently, thereby reducing interdependencies.
Utilizing Lazy Imports
Lazy imports can help mitigate circular dependencies by delaying the import of modules until they are absolutely necessary. This can be achieved by placing the import statement inside a function rather than at the top of the module.
Example:
“`python
def my_function():
from mymodule import my_class
return my_class()
“`
By doing this, you ensure that the import only occurs when `my_function` is called, thus preventing circular import errors during module loading.
Refactoring Code with a Shared Module
Creating a shared module can effectively eliminate circular dependencies. By extracting common functionalities into a separate module, you can reduce the direct dependencies between the original modules.
Consider the following structure:
“`
/project
/module_a.py
/module_b.py
/common.py Shared functionality
“`
In this case, both `module_a` and `module_b` can import from `common.py` instead of importing each other.
Example: Refactoring Circular Imports
Here is a simple table illustrating how to refactor code to avoid circular imports:
Before Refactoring | After Refactoring |
---|---|
module_a.py from module_b import function_b def function_a(): function_b() |
module_a.py from common import shared_function def function_a(): shared_function() |
module_b.py from module_a import function_a def function_b(): function_a() |
module_b.py from common import shared_function def function_b(): shared_function() |
By following these strategies, developers can effectively prevent circular imports, ensuring a smoother and more maintainable codebase.
Understanding Circular Imports
Circular imports occur when two or more modules depend on each other, creating a loop that can lead to import errors. This situation arises when:
- Module A imports Module B.
- Module B imports Module A.
The Python interpreter struggles to resolve the dependencies, often resulting in an `ImportError`.
Identifying Circular Imports
To spot circular imports in your code, consider the following approaches:
- Error Messages: Pay attention to the traceback information provided by Python when an import fails.
- Code Structure Review: Analyze your module dependencies and look for cycles.
- Static Code Analysis Tools: Use tools like `pylint` or `flake8` that can help identify circular dependencies automatically.
Preventing Circular Imports
Several strategies can effectively prevent circular imports in your Python projects:
1. Refactor Code Structure
- Combine Related Modules: If two modules are tightly coupled, consider merging them into a single module.
- Use Submodules: Organize related functions or classes into submodules to reduce interdependencies.
2. Lazy Imports
- Import Inside Functions: Delay the import of a module until it is needed, placing the import statement inside the function where the module is used.
“`python
def use_module_a():
from module_a import function_a
function_a()
“`
3. Dependency Injection
- Pass Dependencies as Arguments: Instead of importing a module directly, pass the required functions or classes as parameters to other functions or classes.
4. Use Python’s Import System Wisely
- Utilize Absolute Imports: Always prefer absolute imports over relative imports to avoid confusion and unintended circular dependencies.
5. Create a Central Module
- Centralize Common Functions: Create a new module that holds shared functions or classes, and have other modules import from this central module instead.
Example Scenario
Consider the following example that demonstrates a circular import:
module_a.py:
“`python
from module_b import function_b
def function_a():
print(“Function A”)
function_b()
“`
module_b.py:
“`python
from module_a import function_a
def function_b():
print(“Function B”)
function_a()
“`
This structure leads to an `ImportError`. Refactoring might involve creating a `module_common.py`:
module_common.py:
“`python
def shared_function():
print(“Shared Function”)
“`
Then adjust `module_a.py` and `module_b.py`:
module_a.py:
“`python
from module_common import shared_function
def function_a():
print(“Function A”)
shared_function()
“`
module_b.py:
“`python
from module_common import shared_function
def function_b():
print(“Function B”)
shared_function()
“`
By separating the shared functionality, both modules can operate independently without circular dependencies.
Implementing these strategies will enhance code maintainability and reduce the likelihood of circular imports in Python applications. Regular code reviews and employing static analysis tools can further assist in managing module dependencies effectively.
Strategies to Avoid Circular Imports in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively prevent circular imports in Python, it is crucial to structure your codebase with a clear separation of concerns. By organizing related functionalities into distinct modules and avoiding interdependencies, you can minimize the risk of circular imports occurring.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “Utilizing lazy imports can be a game changer in preventing circular imports. By importing modules only when they are needed, rather than at the top of your files, you can break the cycle of dependencies that often leads to these issues.”
Sarah Patel (Python Software Architect, DevMasters). “Refactoring your code to use a more modular approach can significantly reduce circular import problems. Consider consolidating shared functionality into a common module that both original modules can import, thus eliminating direct dependencies between them.”
Frequently Asked Questions (FAQs)
What is a circular import in Python?
A circular import occurs when two or more modules depend on each other directly or indirectly, creating a loop that can lead to ImportError or unexpected behavior.
How can I identify circular imports in my Python project?
You can identify circular imports by examining the import statements in your modules. Tools like `pylint` or `flake8` can also help detect circular dependencies.
What strategies can I use to prevent circular imports?
To prevent circular imports, consider restructuring your code by consolidating related functions into a single module, using import statements within functions instead of at the top of the file, or utilizing dependency injection.
Is it advisable to use relative imports to avoid circular imports?
While relative imports can help manage module dependencies, they may not always resolve circular imports. It is generally better to refactor the code structure for clarity and maintainability.
Can I use importlib to dynamically import modules and avoid circular imports?
Yes, using `importlib` allows for dynamic imports, which can help avoid circular imports by importing modules only when needed, thus breaking the dependency cycle.
What are the potential consequences of ignoring circular imports?
Ignoring circular imports can lead to runtime errors, unexpected behavior, and maintenance challenges, making it difficult to debug and understand the codebase.
Preventing circular imports in Python is essential for maintaining a clean and efficient codebase. Circular imports occur when two or more modules depend on each other, leading to import errors and complications in the execution of the program. To avoid this issue, developers can adopt several strategies, such as restructuring the code to minimize interdependencies, utilizing local imports within functions, and implementing a clear module hierarchy. These practices not only mitigate the risk of circular imports but also enhance the overall organization of the code.
One effective approach to prevent circular imports is to refactor code into smaller, more focused modules. By ensuring that each module has a single responsibility, developers can reduce the likelihood of interdependencies that lead to circular imports. Additionally, using local imports allows for the import of a module only when it is needed, which can help avoid circular dependencies during the initial loading of modules.
Another important consideration is to establish a clear module hierarchy. Organizing modules in a way that reflects their relationships can help clarify dependencies and reduce the chances of circular imports. Utilizing design patterns, such as dependency injection, can further assist in managing dependencies effectively. By implementing these strategies, developers can create a more robust and maintainable codebase, ultimately leading to improved software quality.
Author Profile
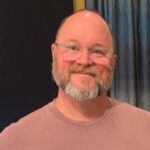
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?