How Can You Effectively Parse XML in Python?
In an increasingly data-driven world, the ability to efficiently handle and manipulate data formats is more crucial than ever. XML, or eXtensible Markup Language, is one of the most widely used formats for data interchange, especially in web services and configuration files. Whether you’re working on a small project or a large-scale application, knowing how to parse XML in Python can empower you to extract meaningful information and integrate it seamlessly into your workflows. This article will guide you through the essentials of XML parsing in Python, equipping you with the tools and techniques needed to tackle XML data with confidence.
Parsing XML in Python is a straightforward yet powerful skill that opens up a myriad of possibilities for developers and data scientists alike. Python’s rich ecosystem offers several libraries designed to simplify the process of reading, navigating, and manipulating XML files. From the built-in `xml.etree.ElementTree` module to more advanced libraries like `lxml`, each option provides unique features that cater to different parsing needs. Understanding these tools will help you choose the right one for your specific use case, whether it involves simple data extraction or complex document manipulation.
As we delve deeper into the world of XML parsing, you’ll discover the fundamental concepts and techniques that will enable you to transform raw XML data into structured
Parsing XML with ElementTree
The ElementTree module is part of the Python standard library and provides a simple way to process XML data. It allows you to navigate and modify the XML tree structure easily. To start using ElementTree, you first need to import it.
“`python
import xml.etree.ElementTree as ET
“`
To parse an XML file, you can use the `parse()` method, which reads an XML file and returns an ElementTree object. Here’s an example of how to parse a file:
“`python
tree = ET.parse(‘example.xml’)
root = tree.getroot()
“`
After parsing, you can access elements in the XML structure using various methods:
- Finding elements: Use `find()` to get the first matching child element.
- Finding all elements: Use `findall()` to get a list of all matching child elements.
- Accessing attributes: Use the `attrib` property to get the attributes of an element.
Here’s a sample code snippet demonstrating these methods:
“`python
for child in root:
print(child.tag, child.attrib)
Finding specific elements
specific_element = root.find(‘specific_tag’)
print(specific_element.text)
“`
Parsing XML with lxml
The `lxml` library is a powerful and feature-rich library for XML and HTML parsing. It supports XPath and XSLT, making it suitable for complex XML processing tasks. To use `lxml`, you need to install it first:
“`bash
pip install lxml
“`
Once installed, you can parse XML similarly to ElementTree, but with added capabilities. Here’s an example:
“`python
from lxml import etree
Parse the XML file
tree = etree.parse(‘example.xml’)
root = tree.getroot()
“`
With `lxml`, you can perform XPath queries to locate elements more efficiently:
“`python
Using XPath to find elements
elements = root.xpath(‘//specific_tag’)
for element in elements:
print(element.text)
“`
Comparative Overview of XML Parsing Libraries
When choosing a library for parsing XML in Python, consider the following aspects:
Feature | ElementTree | lxml |
---|---|---|
Standard Library | Yes | No |
Ease of Use | Simple | More Complex |
Performance | Good | Better |
XPath Support | Limited | Full |
XSLT Support | No | Yes |
Choosing between `ElementTree` and `lxml` depends on your specific needs. If you require simplicity and do not need advanced features, ElementTree may suffice. However, for complex XML processing that requires XPath or XSLT, `lxml` is the better choice.
Understanding XML Parsing Libraries in Python
Python offers several libraries for parsing XML, each suited for different use cases. The most commonly used libraries include:
- ElementTree: Part of the Python standard library, it provides a simple and efficient way to parse and create XML.
- lxml: A powerful library that extends ElementTree with additional features, including support for XPath and XSLT.
- minidom: A part of the xml.dom module, it implements a Document Object Model (DOM) interface for XML.
Library | Pros | Cons |
---|---|---|
ElementTree | Simple, included in the standard library | Limited to basic parsing capabilities |
lxml | Fast, supports advanced XML features | Requires installation, not built-in |
minidom | Easy to use, supports DOM manipulation | Can be memory-intensive for large XML |
Parsing XML with ElementTree
ElementTree is the easiest way to parse XML in Python. Here’s how to use it:
- Import the library:
“`python
import xml.etree.ElementTree as ET
“`
- Load and parse an XML file:
“`python
tree = ET.parse(‘file.xml’)
root = tree.getroot()
“`
- Accessing elements:
You can navigate through the XML tree using various methods:
- `root.tag` retrieves the root element’s tag.
- `root.attrib` returns the attributes of the root element.
- `root[0]` accesses the first child element.
- Iterating through elements:
“`python
for child in root:
print(child.tag, child.attrib)
“`
Using lxml for Advanced Parsing
To leverage lxml for more complex XML tasks, you need to install it via pip:
“`bash
pip install lxml
“`
Here’s a basic example of using lxml:
- Import the library:
“`python
from lxml import etree
“`
- Parse an XML string or file:
“`python
tree = etree.parse(‘file.xml’)
root = tree.getroot()
“`
- Using XPath:
lxml allows you to perform XPath queries for more precise searches:
“`python
results = root.xpath(‘//tagname[@attribute=”value”]’)
for result in results:
print(result.text)
“`
Creating XML Documents
Both ElementTree and lxml can be used to create XML documents. Here’s how to do it with ElementTree:
- Create a new XML structure:
“`python
import xml.etree.ElementTree as ET
root = ET.Element(“root”)
child = ET.SubElement(root, “child”, attrib={“name”: “example”})
child.text = “This is a child element.”
tree = ET.ElementTree(root)
tree.write(“output.xml”)
“`
- Creating XML with lxml:
“`python
from lxml import etree
root = etree.Element(“root”)
child = etree.SubElement(root, “child”, name=”example”)
child.text = “This is a child element.”
tree = etree.ElementTree(root)
tree.write(“output.xml”, pretty_print=True, xml_declaration=True, encoding=’UTF-8′)
“`
Error Handling in XML Parsing
Error handling is crucial when dealing with XML. Consider the following common exceptions:
- ParseError: Raised when the XML is not well-formed.
- FileNotFoundError: Raised if the specified XML file does not exist.
Example of handling errors:
“`python
try:
tree = ET.parse(‘file.xml’)
except ET.ParseError as e:
print(f”Parse error: {e}”)
except FileNotFoundError:
print(“File not found.”)
“`
Using these libraries and techniques allows for effective XML parsing and manipulation in Python, catering to both simple and advanced needs.
Expert Insights on Parsing XML in Python
Dr. Emily Carter (Senior Software Engineer, DataTech Solutions). “When parsing XML in Python, leveraging libraries such as ElementTree or lxml is essential for efficient data manipulation. These libraries provide robust methods for navigating and modifying XML trees, which can significantly streamline data extraction processes.”
Johnathan Lee (Lead Data Scientist, Insight Analytics). “Understanding the structure of the XML document is crucial before parsing. Using XPath expressions in conjunction with libraries like lxml can enhance your ability to query specific data points, allowing for more precise data analysis and transformation.”
Sarah Thompson (XML Data Specialist, Tech Innovations Inc.). “Error handling is often overlooked when parsing XML. Implementing try-except blocks in your Python code can prevent crashes and ensure that your application gracefully handles malformed XML, which is a common issue in real-world data.”
Frequently Asked Questions (FAQs)
What libraries can I use to parse XML in Python?
You can use several libraries to parse XML in Python, including `xml.etree.ElementTree`, `lxml`, and `xml.dom.minidom`. Each library has its own strengths, with `ElementTree` being part of the standard library and `lxml` offering advanced features and better performance.
How do I parse XML using the ElementTree library?
To parse XML using the ElementTree library, you can use the `ElementTree.parse()` method to read an XML file or `ElementTree.fromstring()` for XML strings. After parsing, you can navigate the XML tree using methods like `.find()`, `.findall()`, and `.iter()`.
Can I parse large XML files in Python?
Yes, you can parse large XML files in Python using the `iterparse()` method from the ElementTree library. This method allows you to process the XML incrementally, which helps manage memory usage effectively for large files.
What is the difference between SAX and DOM parsing in Python?
SAX (Simple API for XML) is an event-driven, streaming approach that reads XML data sequentially and is memory efficient, while DOM (Document Object Model) loads the entire XML document into memory, allowing for easier manipulation but requiring more memory. Choose SAX for large files and DOM for smaller, manageable documents.
How can I handle namespaces when parsing XML in Python?
To handle namespaces in XML, you can use the `namespace` parameter in methods like `.find()` or `.findall()`. You need to define a dictionary mapping prefixes to their corresponding URIs and pass it when querying elements.
Is it possible to convert XML data to JSON in Python?
Yes, you can convert XML data to JSON in Python by first parsing the XML into a Python dictionary using libraries like `xmltodict`, and then using the `json` module to convert the dictionary to a JSON string. This process allows for easier manipulation and data interchange.
Parsing XML in Python is a fundamental skill for developers working with data interchange formats. Python offers several libraries for XML parsing, including the built-in `xml.etree.ElementTree`, `minidom`, and third-party libraries like `lxml`. Each of these libraries provides unique features and capabilities, allowing developers to choose the one that best fits their specific use case. The choice of library often depends on factors such as performance requirements, ease of use, and the complexity of the XML data being processed.
Using `xml.etree.ElementTree` is typically recommended for its simplicity and ease of integration into Python applications. This library allows for straightforward navigation of XML trees, making it easier to extract and manipulate data. For more complex XML documents, `lxml` provides robust support for XPath and XSLT, enhancing the ability to query and transform XML data efficiently. Understanding how to utilize these libraries effectively is essential for anyone looking to work with XML in Python.
mastering XML parsing in Python not only enhances a developer’s toolkit but also facilitates better data handling and interoperability between systems. By leveraging the appropriate libraries and understanding their functionalities, developers can efficiently parse, manipulate, and utilize XML data in their applications. As XML continues to
Author Profile
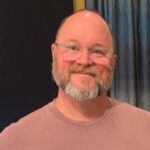
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?