How Can You Execute Specific Lines of Code in Python?
In the world of programming, efficiency and precision are paramount, especially when working with languages like Python. Whether you’re debugging a complex script or experimenting with new code snippets, the ability to run only certain lines of code can save time and enhance your workflow. Imagine being able to isolate specific functions or test individual components without executing the entire program—this not only streamlines your development process but also helps in identifying issues more effectively. In this article, we will explore various methods and techniques that empower you to selectively run lines of Python code, allowing you to harness the true potential of this versatile language.
When working with Python, it’s common to find yourself in situations where executing the entire script is unnecessary or even counterproductive. By learning how to run specific lines, you can focus on the parts of your code that matter most at any given moment. This approach not only aids in debugging but also fosters a more interactive coding experience, especially in environments like Jupyter notebooks or interactive shells.
Moreover, mastering the art of executing selective code can significantly boost your productivity, enabling you to iterate quickly on your ideas. Whether you’re a seasoned developer or a novice just starting out, understanding how to manipulate your code execution can lead to more efficient problem-solving and a deeper comprehension of Python’s capabilities
Using the Interactive Python Shell
The Interactive Python Shell, also known as REPL (Read-Eval-Print Loop), is a powerful tool that allows you to execute Python code line by line. This is especially useful for testing small code snippets or debugging specific parts of a larger program.
To access the Interactive Shell, simply open your terminal and type `python` or `python3`, depending on your installation. Once inside, you can enter any line of code, and it will be executed immediately.
Key features of the Interactive Shell include:
- Immediate feedback on execution.
- Ability to test small code snippets without creating a file.
- Easy inspection of variables and their values.
Utilizing Jupyter Notebooks
Jupyter Notebooks provide an interactive computing environment that supports executing individual code cells. This feature makes it easy to run specific lines of code without executing the entire script. Each code cell can be run independently, allowing for iterative development and testing.
To create a Jupyter Notebook:
- Install Jupyter using pip:
“`
pip install notebook
“`
- Launch Jupyter Notebook:
“`
jupyter notebook
“`
In a notebook, you can run code cells by selecting the cell and pressing `Shift + Enter`. This runs only the code in that cell, making it a great option for testing.
Using Conditional Statements for Debugging
When developing larger scripts, you may want to run only certain sections based on specific conditions. Conditional statements allow you to control the execution flow of your program.
For example, you can encapsulate code blocks within `if` statements:
“`python
debug_mode = True
if debug_mode:
Code to run only in debug mode
print(“Debugging information”)
“`
This method allows you to toggle the execution of certain lines of code without removing them from the script.
Leveraging Functions for Modular Execution
Functions in Python provide a way to encapsulate code that you might want to execute independently. By defining functions for specific tasks, you can call them as needed without running the entire script.
Here’s a basic example:
“`python
def run_specific_task():
print(“This task is executed.”)
Call the function when needed
run_specific_task()
“`
This approach not only organizes your code but also allows you to run sections as required.
Creating a Control Flow Table
Using a table can help visualize which lines or functions to execute under different conditions.
Condition | Action |
---|---|
Debug Mode On | Run debugging code |
Debug Mode Off | Run main application code |
Special Feature Active | Run additional feature code |
This structured approach enables efficient code execution and debugging, allowing for a clearer understanding of which sections to run under varying circumstances.
Using the Python Interactive Shell
The Python interactive shell, often referred to as the REPL (Read-Eval-Print Loop), allows for executing specific lines of code in an interactive manner. This is particularly useful for testing snippets or debugging.
– **Accessing the Shell**:
- Open a terminal or command prompt.
- Type `python` or `python3` to start the interactive session.
– **Executing Code**:
- Type your Python code directly into the shell and press Enter.
- Each line of code executes immediately, providing instant feedback.
Example:
“`python
>>> x = 10
>>> print(x)
10
“`
Using Jupyter Notebooks
Jupyter Notebooks provide an intuitive environment for running selected lines of Python code. Users can create cells, execute them individually, and see results immediately.
- Creating and Running Cells:
- Install Jupyter with `pip install notebook`.
- Launch Jupyter Notebook with the command `jupyter notebook`.
- Create a new Python notebook and enter code in separate cells.
- Executing Cells:
- Run a cell by selecting it and pressing `Shift + Enter`.
- Modify and rerun cells as needed without executing the entire script.
Using Scripts with Conditional Execution
Python scripts can include conditional statements that allow specific sections of code to run based on certain criteria.
- Example Structure:
“`python
if __name__ == “__main__”:
Code to execute when script is run directly
print(“This runs only when executed directly.”)
“`
- Using Flags:
- Define flags to control which code blocks execute.
Example:
“`python
DEBUG_MODE = True
if DEBUG_MODE:
print(“Debugging information…”)
“`
Using Functions to Isolate Code Execution
Defining functions allows for better control over which lines of code are executed when called.
- Defining Functions:
“`python
def run_specific_lines():
print(“This code runs only when the function is called.”)
run_specific_lines() Call the function to execute its code
“`
- Benefits:
- Code is organized and reusable.
- You can easily comment out function calls to skip execution.
Using the `exec()` Function
The `exec()` function can execute dynamically generated Python code as a string. This can be useful for running specific lines conditionally.
- Example:
“`python
code_to_run = “””
x = 5
print(x)
“””
exec(code_to_run)
“`
- Considerations:
- Be cautious with `exec()` as it can execute arbitrary code, potentially leading to security risks.
Using a Debugger
Utilizing a debugger allows for stepping through code line by line, thus executing only the lines you choose.
- Using PDB:
- Start the debugger by inserting `import pdb; pdb.set_trace()` in your code.
- Use commands like:
- `n`: to execute the next line.
- `c`: to continue execution until the next breakpoint.
Example:
“`python
import pdb
def main():
x = 10
pdb.set_trace() Execution will pause here
y = x + 5
print(y)
main()
“`
Each of these methods provides powerful tools for controlling code execution, allowing you to run only the lines you need at any given time.
Expert Insights on Running Specific Lines in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To run specific lines in Python, utilizing the interactive Python shell or Jupyter Notebook can be highly effective. These environments allow you to execute individual lines or blocks of code, facilitating quick testing and debugging without needing to run the entire script.”
Michael Chen (Software Engineer, CodeCraft Solutions). “Using the built-in `exec()` function provides a flexible way to run specific lines of code. By passing a string containing the code you wish to execute, you can dynamically control which parts of your script are run, which is particularly useful for iterative development.”
Sarah Thompson (Python Educator, LearnPython Academy). “For those new to Python, I recommend using debugging tools like `pdb`. This allows you to set breakpoints and step through your code line by line, giving you precise control over which lines to execute, thus enhancing your understanding of the code flow.”
Frequently Asked Questions (FAQs)
How can I run specific lines of code in a Python script?
You can run specific lines of code in a Python script using an interactive environment like Jupyter Notebook or IPython. These environments allow you to execute selected lines or blocks of code independently.
Is it possible to execute certain lines in an IDE like PyCharm or VSCode?
Yes, both PyCharm and VSCode allow you to select specific lines of code and run them using built-in features. In PyCharm, you can use the “Run Selection” option, while in VSCode, you can use the integrated terminal or the Python extension to run selected lines.
What is the purpose of using the Python REPL for running specific lines?
The Python REPL (Read-Eval-Print Loop) provides an interactive shell where you can enter and execute individual lines of Python code. This is useful for testing snippets and debugging specific parts of your code.
Can I use comments to skip certain lines when running a Python script?
Yes, you can comment out lines of code using the “ symbol. This effectively prevents those lines from being executed when you run the script, allowing you to focus on the remaining lines.
Are there any tools or libraries that facilitate running specific lines in Python?
Yes, tools like `IPython` and libraries such as `pdb` (Python Debugger) allow you to set breakpoints and execute code line by line, facilitating the execution of specific lines during debugging sessions.
How can I use functions to isolate and run specific lines of code?
By encapsulating lines of code within functions, you can call those functions independently to execute only the desired code. This modular approach enhances code organization and allows for targeted execution.
In Python, executing specific lines of code rather than running an entire script can be achieved through various methods. One common approach is to utilize the interactive Python shell or Jupyter Notebook, where users can execute individual lines or blocks of code independently. This allows for immediate feedback and testing, which is particularly useful during the development and debugging phases of programming.
Another effective technique is to leverage functions and modular programming. By encapsulating code within functions, developers can call and execute only the desired sections of their code, facilitating a more organized and efficient workflow. Additionally, using conditional statements can help control the execution flow, allowing certain lines to run based on specific conditions.
Moreover, employing integrated development environments (IDEs) or code editors with debugging capabilities can significantly enhance the ability to run selected lines of code. These tools often provide features such as breakpoints and step execution, enabling developers to pause execution and inspect the state of the program at various points. This not only aids in pinpointing errors but also allows for targeted testing of specific code segments.
In summary, selectively running lines of code in Python can be accomplished through interactive environments, modular programming practices, and the use of advanced IDE features. By adopting these strategies, developers can
Author Profile
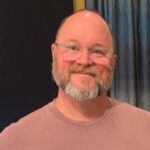
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?