How Can You Effectively Mod in Python? A Comprehensive Guide!
In the ever-evolving world of software development, the ability to modify existing code—commonly known as “modding”—has become an invaluable skill for programmers and enthusiasts alike. Python, with its simplicity and versatility, stands out as a prime candidate for those looking to dive into the art of modding. Whether you’re a seasoned developer aiming to customize a game, enhance a web application, or simply tinker with a script, understanding how to mod in Python opens up a realm of possibilities. This article will guide you through the essentials of modding in Python, empowering you to transform and elevate your projects to new heights.
Modding in Python involves altering or extending existing codebases to achieve new functionalities or improve performance. This can range from creating plugins for popular applications to modifying game mechanics in your favorite titles. The beauty of Python lies in its rich ecosystem of libraries and frameworks, which provide a solid foundation for developers to build upon. By leveraging these tools, you can not only create unique modifications but also contribute to the open-source community, sharing your innovations with others.
As you embark on your journey into Python modding, you’ll discover the importance of understanding the underlying architecture of the code you wish to modify. Familiarity with Python’s syntax and structure will be
Understanding Modding Basics
Modding, or modifying, in Python typically involves altering existing code or creating new functionalities within a software environment. This can take various forms, such as enhancing gameplay in video games, adding features to existing applications, or customizing libraries. To successfully mod in Python, it is essential to understand the following core concepts:
- Python Environment: Setting up a proper environment using tools like virtualenv or conda can help manage dependencies and avoid conflicts.
- Source Code Access: Ensure you have access to the source code of the software you wish to modify. This may involve downloading it from repositories or extracting it from installed applications.
- Familiarity with the Codebase: Understanding the structure and flow of the original code will facilitate smoother modifications. Utilize comments and documentation within the code to guide your understanding.
Tools and Libraries for Modding
Several tools and libraries can enhance your modding experience in Python. Here are some essential ones:
- IDEs and Text Editors: Use Integrated Development Environments (IDEs) like PyCharm, or text editors like Visual Studio Code, to write and debug your code efficiently.
- Version Control Systems: Tools like Git allow you to track changes in your codebase, making it easier to revert back if needed.
- Python Libraries: Depending on your modding goals, various libraries may be beneficial. For example, `pygame` is excellent for game modifications, while `Flask` can be used for web application enhancements.
Modding Techniques
Modding techniques can vary significantly based on the application being modified. Here are some common approaches:
- Script Injection: This involves adding new scripts or modifying existing ones to change behavior without altering the core codebase.
- Patching: Modifying existing binaries or scripts to fix bugs or introduce new features.
- Creating Plugins: Developing separate modules or plugins that can be integrated with the main application to extend functionality.
Technique | Description | Use Case |
---|---|---|
Script Injection | Adding or modifying scripts to change application behavior. | Game mods where new features are added without altering the original game files. |
Patching | Directly modifying the existing code or binary files. | Fixing bugs in open-source applications. |
Creating Plugins | Developing additional modules that interface with the main application. | Enhancing web applications with new features. |
Best Practices for Modding
When engaging in modding, adhere to best practices to ensure a smooth and efficient process:
- Backup Original Files: Always create backups of the original files before making any modifications. This precaution allows you to restore the original state if necessary.
- Document Changes: Keep a record of the changes you make, including the reasons for these alterations. This documentation can be invaluable for future reference.
- Test Thoroughly: After making modifications, rigorously test the application to ensure that your changes work as intended and do not introduce new issues.
By following these guidelines and utilizing the appropriate tools and techniques, you can successfully mod in Python, enhancing your projects and applications in meaningful ways.
Understanding Python Modules
In Python, a module is a file containing Python code that can define functions, classes, and variables. Modules help in organizing code logically and are reusable across different projects. Here are key aspects to understand:
- File Structure: A module is simply a `.py` file. For example, `mymodule.py` can be imported using `import mymodule`.
- Namespace: Each module has its own namespace, which helps avoid naming conflicts.
- Built-in Modules: Python comes with a standard library of modules, such as `os`, `sys`, and `math`, which can be utilized directly.
Creating Your Own Module
To create a module, follow these steps:
- Create a Python file: Name it `mymodule.py`.
- Define functions or classes: For example:
“`python
def greet(name):
return f”Hello, {name}!”
“`
- Import the module: In another Python file, you can use:
“`python
import mymodule
print(mymodule.greet(“Alice”))
“`
Using `__init__.py` for Packages
When organizing modules into packages, include an `__init__.py` file in the directory. This file can be empty or execute initialization code for the package.
- Directory Structure:
“`
mypackage/
__init__.py
module1.py
module2.py
“`
- Importing from Packages: You can import like this:
“`python
from mypackage import module1
“`
Modifying Existing Modules
Modifying a module involves editing its code directly. To ensure changes take effect without restarting your application, use the `importlib` module:
- Reloading a Module:
“`python
import importlib
import mymodule
importlib.reload(mymodule)
“`
- Best Practices:
- Maintain a clear structure and documentation for your modules.
- Use version control to track changes effectively.
Extending Functionality with Third-Party Modules
Python has a rich ecosystem of third-party modules available via the Python Package Index (PyPI). You can use `pip` to install these packages.
- Installation Command:
“`bash
pip install package_name
“`
- Commonly Used Packages:
Package | Purpose |
---|---|
NumPy | Numerical computing |
Pandas | Data manipulation and analysis |
Requests | HTTP requests handling |
Creating Custom Modifications
To modify or extend the functionality of an existing module without changing its source code, consider subclassing or composing:
- Subclassing:
“`python
import existing_module
class CustomClass(existing_module.ExistingClass):
def new_method(self):
Implement new functionality
pass
“`
- Composition:
“`python
import existing_module
class NewClass:
def __init__(self):
self.instance = existing_module.ExistingClass()
def additional_functionality(self):
Use self.instance to access existing functionality
pass
“`
This approach allows you to enhance or modify behavior while keeping the original module intact.
Expert Insights on Modding in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Modding in Python requires a deep understanding of the language’s object-oriented features. By leveraging these capabilities, developers can create custom modifications that enhance functionality while maintaining code integrity.”
James Liu (Lead Software Engineer, Open Source Community). “The key to successful modding in Python lies in utilizing existing libraries and frameworks. This approach not only accelerates development but also ensures compatibility with various systems, making your mods more robust and widely usable.”
Sarah Thompson (Python Educator and Author). “Understanding the principles of modular programming is essential for anyone looking to mod in Python. By structuring your code into reusable components, you can easily adapt and expand your modifications without compromising the overall architecture.”
Frequently Asked Questions (FAQs)
What is modding in Python?
Modding in Python refers to the process of modifying existing Python programs or libraries to enhance functionality, fix bugs, or customize behavior according to specific needs.
How do I get started with modding in Python?
To begin modding in Python, familiarize yourself with the Python programming language, understand the codebase you wish to modify, and set up a suitable development environment with necessary tools such as a code editor and version control system.
Are there specific libraries or frameworks for modding in Python?
Yes, several libraries and frameworks facilitate modding in Python, including Pygame for game development, Flask for web applications, and Django for more extensive web projects. Each provides unique functionalities that can be extended or modified.
What are some common practices for modding in Python?
Common practices include maintaining clear documentation, adhering to coding standards, using version control systems like Git, and writing unit tests to ensure modifications do not introduce new issues.
Can I mod third-party libraries in Python?
Yes, you can mod third-party libraries in Python, but it is essential to respect the licensing agreements and consider contributing back to the community by submitting your modifications.
What tools can assist in the modding process in Python?
Tools such as PyCharm, VSCode, and Jupyter Notebook can assist in the modding process by providing features like debugging, code completion, and integrated terminal access, which streamline development and testing.
modding in Python offers a versatile approach to enhancing and customizing applications, games, and software. By leveraging Python’s extensive libraries and frameworks, developers can create modifications that improve functionality, add new features, or alter existing behaviors. Understanding the underlying principles of Python programming is essential for effective modding, as it allows developers to manipulate code and integrate their modifications seamlessly.
Key takeaways from the discussion on modding in Python include the importance of familiarity with the Python environment and the specific application being modified. Developers should also consider the ethical implications of modding, ensuring that their modifications do not infringe on copyright or violate terms of service. Additionally, engaging with the community through forums and open-source projects can provide valuable insights and support for those looking to enhance their modding skills.
Ultimately, successful modding in Python requires a blend of technical knowledge, creativity, and community engagement. As developers continue to explore the possibilities within Python, they will find that modding not only enhances their projects but also contributes to their growth as programmers. Embracing this practice can lead to innovative solutions and a deeper understanding of the programming landscape.
Author Profile
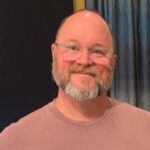
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?