How Can You Effectively Manage Events in Kubernetes Using a Dynamic Informer?
In the ever-evolving landscape of cloud-native applications, Kubernetes has emerged as the backbone for orchestrating containerized workloads. As organizations increasingly rely on Kubernetes to manage their applications, the need for efficient event management becomes paramount. Enter the dynamic informer—a powerful tool designed to streamline the process of monitoring and responding to changes within your Kubernetes cluster. Whether you’re a seasoned developer or a newcomer to the Kubernetes ecosystem, understanding how to leverage dynamic informers can significantly enhance your operational capabilities and improve the responsiveness of your applications.
Dynamic informers serve as a bridge between your application and the Kubernetes API, providing a real-time view of resource changes. By efficiently watching for events such as creation, updates, or deletions of resources, these informers enable developers to build reactive systems that can adapt to the dynamic nature of cloud environments. This capability is crucial for maintaining the health and performance of applications, especially in scenarios where rapid scaling or configuration changes are required.
As we delve deeper into the intricacies of managing events with a dynamic informer, you’ll discover the underlying principles that govern their operation, the benefits they offer, and best practices for implementation. Whether you’re looking to enhance your monitoring strategies or automate response actions, mastering dynamic informers will empower you to harness the full potential of Kubernetes, ensuring your applications remain
Understanding Dynamic Informers
Dynamic Informers in Kubernetes provide a flexible way to monitor and manage events for various resources in a cluster. They allow developers to build applications that react to changes in the state of resources such as Pods, Services, and Deployments. By utilizing Dynamic Informers, you can dynamically adapt to the types of resources you want to watch without hardcoding specific types into your application.
The key components of Dynamic Informers include:
- Client-Go Library: This is the official Go client for interacting with Kubernetes. It provides functionalities to work with Dynamic Informers.
- Dynamic Client: This allows you to interact with Kubernetes resources in a generic way, enabling you to work with different resource types without needing to define each one beforehand.
- Event Handlers: These are functions that you can define to respond to events such as creation, updating, or deletion of resources.
Setting Up a Dynamic Informer
To set up a Dynamic Informer, follow these steps:
- Initialize the Kubernetes Client: Create a dynamic Kubernetes client using the client-go library.
- Create a Dynamic Informer: Use the dynamic client to set up an informer that watches a specific resource type.
- Define Event Handlers: Implement event handlers that will process the events emitted by the informer.
Here’s a sample code snippet to illustrate this setup:
“`go
import (
“context”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
“k8s.io/client-go/dynamic”
“k8s.io/client-go/tools/cache”
metav1 “k8s.io/apimachinery/pkg/apis/meta/v1”
)
func main() {
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfig)
if err != nil {
panic(err.Error())
}
dynamicClient, err := dynamic.NewForConfig(config)
if err != nil {
panic(err.Error())
}
gvr := schema.GroupVersionResource{Group: “”, Version: “v1”, Resource: “pods”}
informer := cache.NewSharedIndexInformer(
&cache.ListWatch{
ListFunc: func(options metav1.ListOptions) (runtime.Object, error) {
return dynamicClient.Resource(gvr).Namespace(namespace).List(context.TODO(), options)
},
WatchFunc: func(options metav1.ListOptions) (watch.Interface, error) {
return dynamicClient.Resource(gvr).Namespace(namespace).Watch(context.TODO(), options)
},
},
&unstructured.Unstructured{},
0, // No resync period
cache.Indexers{},
)
informer.AddEventHandler(cache.ResourceEventHandlerFuncs{
AddFunc: func(obj interface{}) {
// Handle add event
},
UpdateFunc: func(oldObj, newObj interface{}) {
// Handle update event
},
DeleteFunc: func(obj interface{}) {
// Handle delete event
},
})
stopCh := make(chan struct{})
defer close(stopCh)
go informer.Run(stopCh)
}
“`
Best Practices for Managing Events
When managing events with Dynamic Informers, consider the following best practices:
- Resource Efficiency: Limit the scope of the resources you watch to reduce the load on your cluster.
- Error Handling: Implement robust error handling in your event handlers to avoid crashes due to unexpected data.
- Concurrency: Handle events concurrently when possible to improve performance but ensure thread safety.
- Resource Cleanup: Ensure that you unregister event handlers and clean up resources when they are no longer needed.
Event Handler Patterns
Employing different patterns in your event handlers can improve the responsiveness and maintainability of your applications. Here are common patterns:
Pattern | Description |
---|---|
Logging | Log event details for audit and debugging purposes. |
State Management | Update application state based on resource changes. |
Notifications | Trigger alerts or notifications based on specific events. |
Aggregation | Combine events from multiple sources to produce a unified response. |
By adhering to these practices and patterns, you can effectively manage events in Kubernetes using Dynamic Informers, creating a responsive and adaptive environment for your applications.
Understanding the Dynamic Informer
A dynamic informer in Kubernetes is a crucial component that simplifies the process of managing and responding to changes in resource states within the cluster. It leverages informers to monitor resources and react to events such as creation, updates, and deletions.
Key features of dynamic informers include:
- Event Notification: They provide notifications for various events, allowing applications to respond in real-time.
- Caching Mechanism: Dynamic informers maintain an internal cache of resources, reducing the load on the Kubernetes API server.
- Custom Resource Support: They can be used with both built-in and custom resources, enabling flexibility in resource management.
Setting Up a Dynamic Informer
To set up a dynamic informer, follow these steps:
- Create a Clientset: Use the Kubernetes client-go library to create a clientset that interacts with the Kubernetes API.
“`go
import “k8s.io/client-go/kubernetes”
import “k8s.io/client-go/tools/clientcmd”
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfigPath)
clientset, err := kubernetes.NewForConfig(config)
“`
- Initialize the Informer: Create an informer for the specific resource type you want to monitor.
“`go
import “k8s.io/client-go/tools/cache”
informer := cache.NewSharedInformer(
cache.NewListWatchFromClient(clientset.CoreV1().RESTClient(), “pods”, metav1.NamespaceAll, fields.Everything()),
&corev1.Pod{},
0,
)
“`
- Define Event Handlers: Set up event handlers to define actions on resource events.
“`go
informer.AddEventHandler(cache.ResourceEventHandlerFuncs{
AddFunc: func(obj interface{}) { fmt.Println(“Pod added:”, obj) },
UpdateFunc: func(oldObj, newObj interface{}) { fmt.Println(“Pod updated:”, newObj) },
DeleteFunc: func(obj interface{}) { fmt.Println(“Pod deleted:”, obj) },
})
“`
- Start the Informer: Begin the informer to start receiving events.
“`go
stopCh := make(chan struct{})
defer close(stopCh)
go informer.Run(stopCh)
“`
Handling Events with Dynamic Informers
To effectively handle events, consider implementing the following best practices:
- Concurrency Management: Ensure that your event handler logic is thread-safe, especially if it modifies shared state.
- Rate Limiting: Implement rate limiting for event processing to avoid overwhelming your application during high-load scenarios.
- Error Handling: Incorporate robust error handling in event handlers to prevent failures from propagating.
Performance Considerations
When using dynamic informers, keep the following performance considerations in mind:
Aspect | Recommendation |
---|---|
Resource Selection | Limit the watch scope to necessary namespaces or labels. |
Caching | Regularly monitor and tune cache sizes to balance memory usage. |
Event Processing | Leverage goroutines for non-blocking event processing. |
By adhering to these guidelines and utilizing dynamic informers effectively, you can optimize your Kubernetes event management processes and enhance overall application responsiveness.
Expert Insights on Managing Events with Dynamic Informers in Kubernetes
Dr. Emily Chen (Kubernetes Architect, Cloud Innovations Inc.). “Dynamic informers are essential for managing events in Kubernetes effectively. They allow developers to listen for changes in real-time, enabling responsive applications that can adapt to the ever-changing state of the cluster.”
Michael Thompson (DevOps Engineer, Tech Solutions Group). “Utilizing dynamic informers not only streamlines event management but also enhances resource efficiency. By dynamically adjusting to the needs of the application, teams can reduce overhead and improve system performance.”
Sarah Patel (Cloud Native Consultant, Agile Cloud Services). “The integration of dynamic informers into event management workflows is a game-changer. It empowers teams to implement more sophisticated event-driven architectures, leading to increased scalability and resilience in Kubernetes deployments.”
Frequently Asked Questions (FAQs)
What is a dynamic informer in Kubernetes?
A dynamic informer in Kubernetes is a component that watches for changes in resources and provides notifications about those changes. It allows developers to dynamically manage and respond to events related to Kubernetes objects without having to manually poll for updates.
How do I set up a dynamic informer in my Kubernetes application?
To set up a dynamic informer, you need to create a client for the Kubernetes API, specify the resource type you want to watch, and then register event handlers for add, update, and delete events. This typically involves using client-go libraries in Go or similar client libraries in other programming languages.
What are the benefits of using a dynamic informer?
Dynamic informers provide real-time updates on resource changes, reduce the need for constant polling, and improve application responsiveness. They also help manage resource state effectively by allowing applications to react to changes immediately.
Can I use dynamic informers for custom resources?
Yes, dynamic informers can be used for custom resources defined in Kubernetes. You need to ensure that your custom resource definitions (CRDs) are properly set up, and then you can create informers to watch these resources just like built-in Kubernetes resources.
What are some common use cases for dynamic informers?
Common use cases include monitoring the state of pods, services, and deployments, implementing custom controllers, and managing configurations that change frequently. Dynamic informers are essential for building responsive and efficient Kubernetes operators.
How do I handle errors when using dynamic informers?
Error handling in dynamic informers involves implementing appropriate error handling logic in your event handlers. You should log errors, implement retries for transient errors, and ensure that your application can recover gracefully from failures.
Managing events with a dynamic informer in Kubernetes is a crucial aspect of ensuring efficient resource monitoring and response within a cluster. Dynamic informers allow developers to watch for changes in resources, such as Pods, Services, or ConfigMaps, and react accordingly. By leveraging the Kubernetes client-go library, users can create informers that dynamically adapt to changes, thereby enhancing the responsiveness of applications and services in a cloud-native environment.
One of the key insights from the discussion is the importance of understanding the lifecycle of informers. Properly managing the start, stop, and synchronization of informers is essential to prevent resource leaks and ensure that the application remains in sync with the state of the cluster. Additionally, implementing appropriate event handlers allows for tailored responses to specific events, further optimizing the management of resources.
Another significant takeaway is the flexibility offered by dynamic informers in handling multiple resource types. This capability enables developers to create more complex applications that can respond to a variety of resource changes, facilitating better orchestration and automation within Kubernetes. Overall, mastering the use of dynamic informers is vital for developers seeking to build robust, responsive applications in a Kubernetes environment.
Author Profile
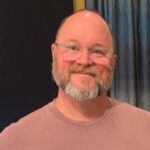
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?