How Can You Create a Python Script from Scratch?
In today’s digital age, the ability to automate tasks and harness the power of programming can significantly enhance productivity and creativity. Python, known for its simplicity and versatility, stands out as a preferred language for both beginners and seasoned developers alike. Whether you’re looking to streamline mundane tasks, analyze data, or even develop complex applications, learning how to make a Python script is an invaluable skill that opens up a world of possibilities. This article will guide you through the essentials of scripting in Python, empowering you to create efficient, functional code that can tackle a variety of challenges.
Creating a Python script is more than just typing lines of code; it’s about understanding the logic and structure that underpin programming. From setting up your environment to writing your first lines of code, the process is both accessible and rewarding. You’ll discover how to utilize Python’s extensive libraries and frameworks, which can help you build scripts that are not only effective but also elegant. As you delve deeper, you’ll learn about best practices and tips that can elevate your coding skills, making your scripts not only functional but also efficient and maintainable.
Whether you’re a complete novice or someone with a bit of coding experience, this article will provide you with the foundational knowledge needed to embark on your Python scripting journey. By the end, you’ll be
Choosing a Python Environment
When creating a Python script, selecting the right environment is crucial for effective development. Python can be executed in various environments, such as local installations, virtual environments, and cloud-based platforms. Each option has its benefits:
- Local Installation: Ideal for development and testing on your own machine. Install Python from the official website and use an IDE (Integrated Development Environment) like PyCharm, Visual Studio Code, or Jupyter Notebook for enhanced coding capabilities.
- Virtual Environments: Useful for managing dependencies. You can create isolated environments using `venv` or `virtualenv`, allowing projects to have their own libraries without conflicts.
- Cloud-Based Platforms: Services like Google Colab or JupyterHub allow for collaborative coding and easy sharing of scripts, especially beneficial for data science projects.
Writing Your First Python Script
A simple Python script can be written using any text editor or IDE. Follow these steps to create a basic script:
- Open your text editor or IDE.
- Create a new file and save it with a `.py` extension, for example, `hello.py`.
- Write your code. A simple script that prints “Hello, World!” would look like this:
“`python
print(“Hello, World!”)
“`
- Save the file.
To execute the script, navigate to the directory where your file is saved using the command line, and run:
“`bash
python hello.py
“`
Structuring Your Script
Properly structuring your Python script enhances readability and maintainability. Here are some best practices:
- Use Functions: Break your code into reusable functions. This promotes modularity and clarity.
- Commenting: Include comments to explain the purpose of complex code sections. Use “ for single-line comments and triple quotes for multi-line comments.
- Consistent Naming Conventions: Follow naming conventions for variables and functions. Use `snake_case` for variable names and `CamelCase` for class names.
Element | Recommendation |
---|---|
File Naming | Use descriptive names with underscores (e.g., my_script.py) |
Function Naming | Use verbs in present tense (e.g., calculate_sum) |
Variable Naming | Be descriptive (e.g., total_price instead of tp) |
Testing Your Script
Testing is an essential part of the development process. Python provides several built-in modules for testing, such as `unittest` and `pytest`.
- Unit Testing: Create tests to check individual functions. An example using `unittest`:
“`python
import unittest
def add(a, b):
return a + b
class TestMathFunctions(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
if __name__ == ‘__main__’:
unittest.main()
“`
Run your tests to ensure that each function behaves as expected. This practice helps in identifying bugs early in the development cycle.
Debugging and Error Handling
Debugging is crucial for identifying issues in your script. Python provides several tools for debugging, including:
- Print Statements: Use `print()` to output variable values at different stages.
- PDB: Python’s built-in debugger allows you to set breakpoints and step through your code.
For error handling, use `try` and `except` blocks to catch and manage exceptions gracefully:
“`python
try:
result = 10 / 0
except ZeroDivisionError:
print(“You cannot divide by zero.”)
“`
Implementing robust error handling ensures your script can handle unexpected situations without crashing.
Setting Up Your Environment
To create a Python script, you must first ensure that your environment is properly set up. This involves installing Python and selecting an appropriate code editor or Integrated Development Environment (IDE).
- Install Python:
- Download the latest version of Python from the official website: [python.org](https://www.python.org/downloads/).
- Follow the installation instructions for your operating system. Ensure you check the box to add Python to your PATH.
- Choose a Code Editor or IDE:
- Popular options include:
- Visual Studio Code: Lightweight, customizable, and supports numerous extensions.
- PyCharm: A powerful IDE specifically designed for Python development.
- Jupyter Notebook: Ideal for data analysis and visualization.
Creating Your First Python Script
Once your environment is set up, you can create your first Python script. Follow these steps:
- Open your chosen code editor or IDE.
- Create a new file with the `.py` extension, for example, `hello_world.py`.
- Write your Python code. Here’s a simple example:
“`python
print(“Hello, World!”)
“`
- Save the file.
Running Your Python Script
After creating your script, you need to run it. The method depends on your environment:
- Using the Command Line:
- Open your command prompt or terminal.
- Navigate to the directory where your script is saved using the `cd` command.
- Run the script with the following command:
“`
python hello_world.py
“`
- Using an IDE:
- Most IDEs have a run button or command. Look for a play icon or an option in the menu labeled “Run” or “Execute.”
Understanding Basic Python Syntax
Familiarizing yourself with Python syntax is crucial for writing effective scripts. Here are some fundamental concepts:
Concept | Description | Example |
---|---|---|
Variables | Store data values | `x = 5` |
Data Types | Different types of data (integers, strings, etc.) | `name = “Alice”` |
Control Flow | Conditional statements and loops | `if x > 0: print(x)` |
Functions | Reusable blocks of code | `def greet(): print(“Hello”)` |
Using Libraries and Modules
Python’s strength lies in its extensive libraries. To leverage them, you can import modules into your scripts.
- Importing a Module:
- Use the `import` statement. For example, to use the `math` library:
“`python
import math
print(math.sqrt(16)) Outputs: 4.0
“`
- Installing External Libraries:
- Use `pip`, Python’s package installer. For instance, to install the `requests` library, run:
“`
pip install requests
“`
Debugging Your Script
Debugging is an essential skill for any programmer. Here are techniques to identify and fix issues in your script:
- Print Statements: Use print statements to track variable values and program flow.
- Error Messages: Pay attention to error messages. They often provide clues about what went wrong.
- Debugging Tools: Utilize debugging features available in IDEs, such as breakpoints and step execution.
Best Practices for Writing Python Scripts
To ensure your scripts are efficient and maintainable, consider the following best practices:
- Code Readability:
- Use descriptive variable names.
- Maintain consistent indentation.
- Commenting:
- Add comments to explain complex logic.
- Version Control:
- Use Git for version control, allowing you to track changes and collaborate effectively.
- Testing:
- Write unit tests to validate your code’s functionality. Use frameworks like `unittest` or `pytest`.
By following these steps and guidelines, you can effectively create and manage Python scripts tailored to various tasks and applications.
Expert Insights on Crafting Python Scripts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When creating a Python script, it is crucial to start with a clear understanding of the problem you are solving. This involves outlining the requirements and breaking down the task into manageable components. A well-structured approach can significantly enhance the efficiency and maintainability of your code.”
Michael Chen (Lead Data Scientist, Data Solutions Group). “Utilizing libraries and frameworks effectively can elevate your Python scripting capabilities. Familiarizing yourself with popular libraries such as NumPy and Pandas not only saves time but also allows you to leverage powerful tools that can handle complex data manipulations effortlessly.”
Sarah Thompson (Python Instructor, Code Academy). “Testing and debugging are integral parts of the scripting process. Implementing unit tests and using debugging tools can help identify issues early on, ensuring that your Python script runs smoothly and meets the intended functionality before deployment.”
Frequently Asked Questions (FAQs)
How do I start writing a Python script?
To start writing a Python script, open a text editor or an Integrated Development Environment (IDE) such as PyCharm or Visual Studio Code. Create a new file with a `.py` extension and begin coding using Python syntax.
What are the basic components of a Python script?
The basic components of a Python script include variables, data types, control structures (like loops and conditionals), functions, and modules. These elements work together to perform tasks and execute logic.
How do I run a Python script?
To run a Python script, open a command line or terminal, navigate to the directory containing the script, and execute the command `python script_name.py`, replacing `script_name.py` with your actual file name.
How can I debug a Python script?
You can debug a Python script using built-in debugging tools like `pdb` or by utilizing IDE features such as breakpoints and step-through execution. Print statements can also help identify issues in the code.
What libraries should I consider for a Python script?
The libraries you might consider depend on your project requirements. Common libraries include NumPy for numerical computations, pandas for data manipulation, requests for HTTP requests, and Flask or Django for web applications.
How can I make my Python script more efficient?
To enhance the efficiency of your Python script, optimize algorithms, minimize the use of global variables, utilize list comprehensions, and leverage built-in functions. Profiling tools can also help identify bottlenecks in performance.
creating a Python script involves several essential steps that can be easily followed by both beginners and experienced programmers. The process begins with defining the problem you want to solve or the task you want to automate. Once you have a clear understanding of the objective, you can start writing the script using a text editor or an Integrated Development Environment (IDE) that supports Python. It is crucial to follow best practices in coding, such as using meaningful variable names, adding comments, and structuring your code for readability and maintainability.
Additionally, testing and debugging are vital components of the scripting process. After writing the initial code, running tests helps identify any errors or unexpected behavior. Debugging tools and techniques can assist in resolving issues, ensuring that the script functions as intended. Furthermore, leveraging Python’s extensive libraries and frameworks can enhance the script’s capabilities and efficiency, allowing for more complex functionalities with less effort.
Finally, once the script is developed and tested, sharing it with others or deploying it in a production environment can maximize its utility. Documenting the script with clear instructions and usage examples will facilitate its adoption by other users. Overall, mastering the process of creating Python scripts opens up numerous opportunities for automation, data analysis, and software development
Author Profile
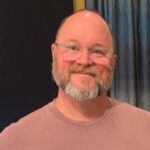
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?