How Can You Create Applications Using Python Effectively?
### How To Make Applications With Python
In the ever-evolving landscape of technology, Python has emerged as a powerhouse for developers and hobbyists alike. Renowned for its simplicity and versatility, this dynamic programming language empowers individuals to create a wide array of applications, from web platforms to data analysis tools and even artificial intelligence systems. Whether you’re a seasoned programmer or just stepping into the world of coding, learning how to make applications with Python can open up a realm of possibilities, allowing you to bring your ideas to life with efficiency and elegance.
As you embark on this journey of application development, you’ll discover that Python’s rich ecosystem of libraries and frameworks provides the essential building blocks for your projects. These tools not only streamline the development process but also enhance functionality, enabling you to focus on creativity and innovation. From crafting user-friendly interfaces to implementing complex algorithms, Python’s capabilities are vast and accessible, making it an ideal choice for diverse applications.
In this article, we will explore the fundamental principles of application development with Python, guiding you through the essential concepts and best practices. Whether you’re aiming to build a simple script or a full-fledged application, you’ll gain insights into the methodologies and resources that can help transform your vision into reality. Get ready to unlock your potential and dive into the exciting world
Choosing the Right Framework
When developing applications with Python, selecting the appropriate framework is crucial for streamlining the development process. The choice of framework can significantly affect the performance, scalability, and maintainability of your application. Below are some popular frameworks, each tailored for different types of applications:
- Web Development:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It is equipped with built-in features like an ORM, authentication, and an admin panel.
- Flask: A lightweight WSGI web application framework that is easy to get started with. It is flexible and allows developers to choose the components they want to integrate.
- GUI Applications:
- Tkinter: The standard GUI toolkit for Python, suitable for building simple desktop applications.
- PyQt: A set of Python bindings for the Qt libraries, allowing for complex and feature-rich applications.
- Data Science and Machine Learning:
- Streamlit: An open-source framework for quickly building web applications for data science projects.
- Dash: A productive framework for building web applications with Python, especially useful for data visualization.
Setting Up Your Development Environment
Establishing a proper development environment is essential for efficient coding and debugging. The following steps outline how to set up your environment:
- Install Python: Ensure you have the latest version of Python installed. You can download it from the official [Python website](https://www.python.org/downloads/).
- Choose an Integrated Development Environment (IDE): Select an IDE that suits your style. Popular choices include:
- PyCharm
- Visual Studio Code
- Jupyter Notebook (for data-focused projects)
- Manage Dependencies: Use `pip` or `conda` to manage your project dependencies. Creating a virtual environment for each project helps maintain a clean workspace.
bash
# Create a virtual environment
python -m venv myenv
# Activate the environment (Windows)
myenv\Scripts\activate
# Activate the environment (Linux/Mac)
source myenv/bin/activate
Building Your First Application
Once your environment is set up, you can begin building your application. Here’s a simple example of a web application using Flask.
python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def hello_world():
return ‘Hello, World!’
if __name__ == ‘__main__’:
app.run(debug=True)
To run this application, save it as `app.py` and execute the following command in your terminal:
bash
python app.py
This will start a local server, and you can view your application by navigating to `http://127.0.0.1:5000/` in your web browser.
Best Practices for Development
Adhering to best practices in software development will enhance the quality and maintainability of your applications. Consider the following guidelines:
- Code Organization: Structure your code into modules and packages for better readability and reusability.
- Version Control: Use Git for version control to track changes and collaborate effectively.
- Documentation: Maintain clear and concise documentation for your code to assist future developers and users.
- Testing: Implement unit tests and integration tests to ensure your application functions as expected.
Best Practice | Description |
---|---|
Modular Design | Break down your application into smaller, manageable components. |
Consistent Naming Conventions | Use clear and descriptive names for functions and variables. |
Code Reviews | Encourage peer reviews to catch bugs and improve code quality. |
Choosing the Right Framework
When developing applications with Python, selecting the appropriate framework is crucial. Different frameworks cater to various types of applications, including web, desktop, and mobile.
- Web Development Frameworks:
- Django: A high-level framework promoting rapid development and clean, pragmatic design. Ideal for complex applications requiring a robust backend.
- Flask: A micro-framework that is lightweight and easy to extend, suitable for simpler applications or APIs.
- FastAPI: Designed for building APIs quickly with automatic data validation and documentation.
- Desktop Application Frameworks:
- Tkinter: The standard GUI toolkit for Python, perfect for simple desktop applications.
- PyQt/PySide: Comprehensive frameworks for developing cross-platform applications with advanced features.
- Kivy: Suitable for applications that require multi-touch support and are intended for both desktop and mobile.
- Mobile Application Frameworks:
- Kivy: Again, versatile for mobile, allowing for multi-platform deployment.
- BeeWare: A collection of tools for building native user interfaces across platforms.
Setting Up Your Development Environment
A well-configured development environment enhances productivity. Follow these steps to set up your Python application development environment:
- Install Python: Download the latest version from the official Python website. Ensure that you add Python to your system PATH during installation.
- Choose an Integrated Development Environment (IDE): Popular IDEs include:
- PyCharm: Feature-rich with strong support for web frameworks.
- Visual Studio Code: Lightweight, customizable, and supports numerous extensions.
- Jupyter Notebook: Ideal for data science applications and prototyping.
- Package Management: Utilize `pip` for package management. Consider using `virtualenv` or `conda` to create isolated environments for your projects.
Understanding Application Structure
A well-organized application structure is vital for maintainability and scalability. A typical Python application might include the following components:
Component | Description |
---|---|
`main.py` | The entry point of the application. |
`requirements.txt` | A file listing all project dependencies. |
`app/` | A directory for application logic and modules. |
`tests/` | A directory dedicated to unit and integration tests. |
`static/` | For static files like images, CSS, and JavaScript. |
`templates/` | For HTML templates, especially in web applications. |
Implementing Core Functionality
To develop your application, focus on the core functionalities required by the end-user. This involves:
- Defining Requirements: Clearly outline what features your application must have.
- Designing the Architecture: Use design patterns such as MVC (Model-View-Controller) for web applications to separate concerns effectively.
- Database Integration: Choose a database (e.g., SQLite, PostgreSQL) and utilize ORMs like SQLAlchemy or Django ORM for data handling.
- User Interface Development: For web apps, use HTML/CSS frameworks like Bootstrap or Tailwind CSS to enhance the UI.
Testing and Debugging
Testing is an integral part of application development. Implement the following strategies:
- Unit Testing: Use the `unittest` or `pytest` frameworks to write tests for individual components.
- Integration Testing: Test how different parts of your application work together.
- Debugging Tools: Utilize built-in tools in IDEs or libraries like `pdb` for debugging purposes.
By carefully planning and executing each step, developers can create robust applications using Python tailored to user needs and industry standards.
Expert Insights on Developing Applications with Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Python’s simplicity and readability make it an ideal choice for both beginners and experienced developers. When creating applications, leveraging frameworks like Flask or Django can significantly accelerate development while ensuring robust functionality.”
Michael Chen (Lead Data Scientist, Data Solutions Group). “For applications that require data analysis or machine learning, Python provides a rich ecosystem of libraries such as Pandas and TensorFlow. Integrating these tools into your application can enhance its capabilities and provide valuable insights to users.”
Sarah Johnson (Full-Stack Developer, CodeCraft Studios). “Understanding the principles of object-oriented programming in Python is crucial for building scalable applications. By structuring your code effectively and utilizing design patterns, you can create maintainable and efficient applications that stand the test of time.”
Frequently Asked Questions (FAQs)
What libraries are essential for making applications with Python?
Key libraries include Flask and Django for web applications, Tkinter and PyQt for desktop applications, and Kivy for mobile applications. These libraries provide frameworks and tools to streamline the development process.
Can I create a mobile application using Python?
Yes, Python can be used to create mobile applications using frameworks like Kivy, BeeWare, or PyQt. These tools allow for cross-platform development, enabling applications to run on both iOS and Android devices.
What are the best practices for structuring a Python application?
Best practices include organizing code into modules and packages, following the Model-View-Controller (MVC) pattern, maintaining a clear directory structure, and using version control systems like Git for collaboration and tracking changes.
How do I handle databases in Python applications?
You can use Object-Relational Mapping (ORM) libraries such as SQLAlchemy or Django ORM to interact with databases. These libraries simplify database operations and allow for easier management of database schemas and queries.
Is it possible to create a GUI application with Python?
Yes, Python supports GUI development through libraries such as Tkinter, PyQt, and wxPython. These libraries provide tools to design user interfaces and handle user interactions effectively.
What resources are available for learning Python application development?
Numerous resources are available, including online courses on platforms like Coursera and Udemy, documentation for specific libraries, and community forums like Stack Overflow. Additionally, books focused on Python programming and application development can be highly beneficial.
creating applications with Python offers a versatile and powerful approach for developers of all skill levels. The language’s simplicity and readability make it an excellent choice for both beginners and experienced programmers. By leveraging Python’s extensive libraries and frameworks, such as Flask for web applications or Tkinter for desktop applications, developers can efficiently build robust and scalable solutions tailored to specific needs.
Moreover, Python’s strong community support and wealth of resources, including documentation and tutorials, significantly enhance the learning curve. Developers can easily find help and collaborate with others, which fosters innovation and problem-solving. Additionally, the language’s cross-platform compatibility ensures that applications can run on various operating systems, broadening their accessibility and usability.
Ultimately, the key takeaway is that Python empowers developers to create a wide range of applications, from simple scripts to complex systems. By mastering the fundamentals of Python programming and exploring its rich ecosystem, individuals can unlock new opportunities in software development and contribute to the ever-evolving tech landscape.
Author Profile
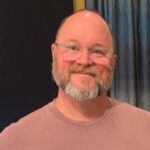
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?