How Can You Effectively Make API Calls in Python?
In today’s interconnected digital landscape, the ability to communicate with various web services through Application Programming Interfaces (APIs) is a crucial skill for developers and data enthusiasts alike. Whether you’re building a dynamic web application, automating tasks, or integrating third-party services, knowing how to make API calls in Python can unlock a world of possibilities. Python, with its simplicity and robust libraries, makes it easier than ever to interact with APIs, allowing you to fetch data, send requests, and manipulate responses seamlessly.
Making API calls in Python involves understanding the fundamental concepts of HTTP requests, such as GET, POST, PUT, and DELETE. With libraries like `requests`, developers can easily craft requests to retrieve or send data to a server, handle authentication, and manage response data. This process not only enables access to a plethora of online resources but also empowers you to build applications that can respond dynamically to user needs by leveraging external data sources.
As you delve deeper into the world of API calls in Python, you’ll discover best practices for error handling, response parsing, and optimizing your requests for better performance. Whether you’re a beginner looking to get started or an experienced developer aiming to refine your skills, understanding how to make API calls effectively will enhance your programming toolkit and open the door to innovative project possibilities. Get
Understanding HTTP Methods
When making API calls, understanding the different HTTP methods is crucial as they dictate the type of interaction you will have with the server. The most commonly used methods include:
- GET: Retrieves data from the server. It is the most common method and is idempotent, meaning multiple identical requests will yield the same result.
- POST: Sends data to the server to create a resource. This method is not idempotent, as subsequent requests may lead to different outcomes.
- PUT: Updates a resource or creates it if it does not exist. It is idempotent.
- DELETE: Removes a resource from the server. Like GET and PUT, it is also idempotent.
Using the Requests Library
Python’s `requests` library simplifies the process of making HTTP requests. To get started, ensure you have the library installed:
“`bash
pip install requests
“`
Here is a basic example of how to perform various types of API calls using this library:
“`python
import requests
GET request
response = requests.get(‘https://api.example.com/data’)
print(response.json())
POST request
data = {‘key’: ‘value’}
response = requests.post(‘https://api.example.com/data’, json=data)
print(response.json())
PUT request
update_data = {‘key’: ‘new_value’}
response = requests.put(‘https://api.example.com/data/1’, json=update_data)
print(response.json())
DELETE request
response = requests.delete(‘https://api.example.com/data/1’)
print(response.status_code)
“`
Handling Response Data
When you make a request, the server responds with a status code and data. The `requests` library provides methods to handle these responses effectively.
Status Code | Description |
---|---|
200 | OK |
201 | Created |
204 | No Content |
400 | Bad Request |
401 | Unauthorized |
404 | Not Found |
500 | Internal Server Error |
You can check the status code and parse the response data as follows:
“`python
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f”Error: {response.status_code}”)
“`
Sending Parameters and Headers
APIs often require parameters and custom headers for requests. You can include these in your calls with the `params` and `headers` arguments.
“`python
url = ‘https://api.example.com/search’
params = {‘query’: ‘example’}
headers = {‘Authorization’: ‘Bearer your_token’}
response = requests.get(url, params=params, headers=headers)
print(response.json())
“`
Error Handling in API Calls
Robust error handling is essential when working with APIs. You can implement try-except blocks to catch exceptions and handle unexpected responses gracefully.
“`python
try:
response = requests.get(‘https://api.example.com/data’)
response.raise_for_status() Raises an error for bad responses
data = response.json()
except requests.exceptions.HTTPError as err:
print(f”HTTP error occurred: {err}”)
except Exception as err:
print(f”An error occurred: {err}”)
“`
This ensures that your application can handle errors without crashing, providing a better user experience.
Understanding API Calls
API (Application Programming Interface) calls are a way for applications to communicate with each other. In Python, these calls can be made using various libraries, the most popular being `requests`.
Setting Up Your Environment
Before making API calls in Python, you need to ensure that your environment is set up correctly. Follow these steps:
- Install Python if it is not already installed. You can download it from [python.org](https://www.python.org/).
- Install the `requests` library using pip:
“`bash
pip install requests
“`
Making a Basic GET Request
A GET request is used to retrieve data from a specified resource. The following is a simple example of how to make a GET request.
“`python
import requests
response = requests.get(‘https://api.example.com/data’)
if response.status_code == 200:
data = response.json() Convert the response to JSON
print(data)
else:
print(f”Error: {response.status_code}”)
“`
Making a POST Request
POST requests are used to send data to a server. This is often used when submitting forms or uploading files. Below is an example of how to execute a POST request.
“`python
import requests
url = ‘https://api.example.com/data’
payload = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
response = requests.post(url, json=payload)
if response.status_code == 201: 201 Created
print(“Data submitted successfully.”)
else:
print(f”Error: {response.status_code}”)
“`
Handling Query Parameters
Query parameters can be included in a GET request by appending them to the URL. You can also use the `params` argument in the `requests.get()` method.
“`python
import requests
params = {‘param1’: ‘value1’, ‘param2’: ‘value2’}
response = requests.get(‘https://api.example.com/data’, params=params)
if response.status_code == 200:
data = response.json()
print(data)
“`
Working with Headers
Sometimes, APIs require specific headers, such as authentication tokens. Here’s how to add headers to your requests.
“`python
import requests
url = ‘https://api.example.com/data’
headers = {‘Authorization’: ‘Bearer your_token_here’}
response = requests.get(url, headers=headers)
if response.status_code == 200:
data = response.json()
print(data)
“`
Handling Errors
Error handling is crucial when making API calls. The following table outlines common HTTP status codes and their meanings:
Status Code | Meaning |
---|---|
200 | OK |
201 | Created |
400 | Bad Request |
401 | Unauthorized |
404 | Not Found |
500 | Internal Server Error |
To handle errors effectively, you can check the status code and take appropriate action:
“`python
response = requests.get(‘https://api.example.com/data’)
if response.ok:
data = response.json()
else:
print(f”Error: {response.status_code} – {response.text}”)
“`
Making Asynchronous API Calls
For performance optimization, particularly in applications that require multiple API calls, asynchronous programming can be beneficial. The `aiohttp` library allows for non-blocking API requests.
“`python
import aiohttp
import asyncio
async def fetch_data(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.json()
async def main():
url = ‘https://api.example.com/data’
data = await fetch_data(url)
print(data)
asyncio.run(main())
“`
Conclusion of API Call Techniques
Utilizing the `requests` and `aiohttp` libraries, along with proper error handling and parameter management, allows you to effectively interact with APIs in Python.
Expert Insights on Making API Calls in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When making API calls in Python, it is crucial to understand the requests library as it simplifies the process significantly. Utilizing this library allows developers to send HTTP requests with minimal code, making it an essential tool for efficient API interaction.”
Michael Chen (Lead Data Scientist, Data Insights Group). “Incorporating error handling when making API calls in Python cannot be overstated. Implementing try-except blocks ensures that your application can gracefully handle unexpected issues, such as network errors or invalid responses, thereby enhancing the robustness of your code.”
Lisa Patel (API Integration Specialist, Cloud Solutions Ltd.). “Understanding the API documentation is fundamental before initiating calls in Python. Each API has unique requirements for authentication, parameters, and response formats. Familiarizing yourself with these details will lead to more successful integrations and fewer runtime errors.”
Frequently Asked Questions (FAQs)
How do I make a simple API call in Python?
You can make a simple API call in Python using the `requests` library. First, install the library using `pip install requests`. Then, use `requests.get(‘API_URL’)` to send a GET request and retrieve data.
What is the difference between GET and POST requests?
GET requests retrieve data from a server, while POST requests send data to a server for processing. GET requests include parameters in the URL, whereas POST requests include data in the request body.
How can I handle JSON responses from an API in Python?
You can handle JSON responses by calling the `.json()` method on the response object returned by the `requests` library. This method converts the JSON response into a Python dictionary for easy manipulation.
What should I do if I encounter an error while making an API call?
If you encounter an error, first check the status code of the response using `response.status_code`. Common codes include 404 for not found or 500 for server errors. You can also implement exception handling using `try` and `except` blocks to manage errors gracefully.
How can I pass headers in an API request?
You can pass headers by using the `headers` parameter in your request. For example, `requests.get(‘API_URL’, headers={‘Authorization’: ‘Bearer TOKEN’})` allows you to include authentication tokens or other necessary headers.
Is it possible to make asynchronous API calls in Python?
Yes, you can make asynchronous API calls using the `aiohttp` library alongside Python’s `asyncio`. This allows you to perform non-blocking requests, improving the efficiency of your application when handling multiple API calls.
making API calls in Python is a fundamental skill for developers who wish to interact with web services and retrieve or send data. The process typically involves using libraries such as `requests`, which simplifies the task of sending HTTP requests and handling responses. Understanding the structure of the API, including endpoints, request methods (GET, POST, PUT, DELETE), and authentication mechanisms, is crucial for successful integration.
Key takeaways include the importance of reading the API documentation thoroughly, as it provides essential information about how to format requests and handle responses. Additionally, error handling is a critical aspect of API calls; developers should implement proper exception handling to manage potential issues such as connection errors or unexpected response formats. Moreover, leveraging libraries like `json` for parsing JSON responses can streamline data manipulation and enhance the overall effectiveness of the API interaction.
Lastly, practicing with real-world APIs can significantly improve one’s proficiency in making API calls. This hands-on experience allows developers to familiarize themselves with various scenarios, such as rate limiting and pagination, which are common in API usage. By mastering these concepts, developers can build robust applications that effectively utilize external data sources and services.
Author Profile
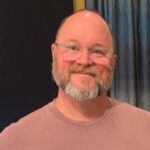
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?