How Can You Create a Stunning Website Using Python?
Creating a website can seem like a daunting task, especially if you’re new to programming. However, with the rise of powerful frameworks and libraries, Python has emerged as a popular choice for web development. Whether you’re looking to build a personal blog, an e-commerce platform, or a portfolio site, Python offers a variety of tools that make the process not only manageable but also enjoyable. In this article, we’ll explore how to harness the power of Python to bring your web development ideas to life, regardless of your experience level.
At its core, building a website with Python involves understanding the essential components of web development, including server-side programming, database management, and user interface design. Python’s versatility allows developers to choose from a range of frameworks, such as Django and Flask, each catering to different needs and preferences. These frameworks simplify the development process by providing built-in functionalities that streamline common tasks, allowing you to focus on creating a unique and engaging user experience.
Moreover, Python’s extensive libraries and supportive community make it an ideal choice for both beginners and seasoned developers. With resources readily available, you can quickly learn how to implement features like user authentication, data storage, and responsive design. As we delve deeper into the specifics of creating a website with Python, you’ll discover how to leverage these tools
Choosing a Web Framework
When developing a website with Python, selecting the appropriate web framework is crucial, as it dictates the structure and capabilities of your project. The most popular frameworks include Flask, Django, and FastAPI, each catering to different project requirements.
- Flask: A micro-framework that is lightweight and easy to get started with. Ideal for small applications or when you want more control over components.
- Django: A high-level framework that follows the “batteries-included” philosophy. It is suitable for larger applications that require more built-in features like authentication, ORM, and admin interfaces.
- FastAPI: A modern framework that is built on top of Starlette and Pydantic, known for its speed and support for asynchronous programming. It is particularly useful for APIs.
Consider the following table to compare these frameworks:
Framework | Type | Best For | Key Features |
---|---|---|---|
Flask | Micro-framework | Small to medium apps | Lightweight, flexible, easy to learn |
Django | Full-stack | Large applications | Built-in admin, ORM, security features |
FastAPI | Modern | API development | Asynchronous support, automatic validation |
Setting Up the Development Environment
To begin building your website, establish a proper development environment. This typically involves installing Python, a web framework, and other necessary tools.
- Install Python: Download the latest version from the official Python website and ensure that it’s added to your system PATH.
- Create a Virtual Environment: This helps manage dependencies specific to your project without affecting the global Python installation. Use the following command:
“`bash
python -m venv myprojectenv
“`
- Activate the Virtual Environment:
- On Windows:
“`bash
myprojectenv\Scripts\activate
“`
- On macOS/Linux:
“`bash
source myprojectenv/bin/activate
“`
- Install Required Packages: Use pip to install your chosen web framework and any other dependencies. For example, to install Flask:
“`bash
pip install Flask
“`
Creating Your First Web Application
Once your environment is set up, you can create your first web application. Below is a simple example using Flask:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Welcome to My Website!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- Explanation:
- The `Flask` class is instantiated to create an application object.
- The `@app.route` decorator is used to bind a function to a URL endpoint.
- The `home()` function returns a simple string when the root URL is accessed.
- `app.run()` starts the development server.
You can run this application by executing the script. Navigate to `http://127.0.0.1:5000/` in your web browser, and you will see the welcome message.
Adding Functionality
To expand your application, consider adding features such as templates, static files, and database integration. Flask supports Jinja2 for templating, which allows you to separate HTML from Python code.
- Templates: Store HTML files in a `templates` directory and render them using Flask’s `render_template()` function.
- Static Files: Place CSS, JavaScript, and images in a `static` directory.
- Database: Use SQLAlchemy for ORM or connect to databases like SQLite, PostgreSQL, or MySQL.
By integrating these functionalities, you can create a robust and dynamic web application tailored to user needs.
Choosing the Right Framework
Selecting an appropriate framework for your web application is crucial. Python offers several frameworks, each suited for different types of projects. Here are some popular options:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. Ideal for larger applications requiring robustness and scalability.
- Flask: A micro-framework that is lightweight and easy to use, perfect for smaller applications or APIs. It allows developers more flexibility in choosing components.
- FastAPI: Known for its speed and performance, this framework is excellent for building APIs with asynchronous capabilities.
Framework | Use Case | Pros | Cons |
---|---|---|---|
Django | Large applications | Batteries-included, ORM support | Can be heavy for simple apps |
Flask | Small to medium apps | Simple, flexible | Requires more setup for larger projects |
FastAPI | API development | High performance, async support | Less mature than Django |
Setting Up Your Development Environment
To develop a website with Python, you need to set up your development environment correctly. Follow these steps:
- Install Python: Ensure that you have the latest version of Python installed. You can download it from the official Python website.
- Create a Virtual Environment: Use `venv` to create a virtual environment to manage dependencies.
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use: myenv\Scripts\activate
“`
- Install the Framework: Use pip to install your chosen framework. For example, for Django:
“`bash
pip install django
“`
Creating Your First Application
Once your environment is set up, you can create your first web application. Here’s how to do it using Django as an example:
- Start a New Project: Use the command line to create a new Django project.
“`bash
django-admin startproject myproject
“`
- Navigate to Project Directory: Change into the project directory.
“`bash
cd myproject
“`
- Run the Development Server: Start the server to see your application in action.
“`bash
python manage.py runserver
“`
- Create an App: Within your project, create an application.
“`bash
python manage.py startapp myapp
“`
- Configure Settings: Add the new app to the `INSTALLED_APPS` section of `settings.py`.
Building Your Web Pages
With your application set up, you can start building web pages. In Django, this involves defining views and templates:
- Define Views: In `views.py`, create functions that handle requests and return responses.
“`python
from django.http import HttpResponse
def home(request):
return HttpResponse(“Hello, World!”)
“`
- Create Templates: Use HTML files to define the structure of your web pages. Store them in a folder named `templates` inside your app directory.
“`html
Welcome to My Website
“`
- Map URLs: In `urls.py`, map URLs to your views.
“`python
from django.urls import path
from .views import home
urlpatterns = [
path(”, home, name=’home’),
]
“`
Deploying Your Website
Once your website is ready, you will want to deploy it. Common deployment options include:
- Heroku: A cloud platform that allows easy deployment of applications. Use the Heroku CLI to deploy your app.
- DigitalOcean: Provides cloud infrastructure to run your web application on a VPS.
- AWS: Offers a scalable and flexible cloud computing environment.
Steps for deploying on Heroku:
- Install the Heroku CLI: Download and install from the Heroku website.
- Create a Heroku App: Use the CLI to create a new app.
“`bash
heroku create myapp
“`
- Deploy Your Code: Push your code to Heroku.
“`bash
git push heroku main
“`
- Open the App: Launch your application in the browser.
“`bash
heroku open
“`
Expert Insights on Building Websites with Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Python offers a versatile framework for web development, particularly with tools like Django and Flask. These frameworks not only simplify the process of building robust applications but also enhance scalability and maintainability, making them ideal for both startups and established enterprises.”
Mark Thompson (Lead Developer, Web Solutions Group). “When considering how to make a website with Python, it is essential to leverage its rich ecosystem of libraries. Utilizing libraries such as SQLAlchemy for database management or Jinja2 for templating can significantly streamline development, allowing for cleaner code and faster deployment.”
Lisa Chen (Web Development Instructor, Coding Academy). “For beginners, starting with Python to create a website can be an enriching experience. I recommend focusing on Flask for small projects due to its simplicity. As you progress, transitioning to Django can provide a more comprehensive understanding of full-stack development.”
Frequently Asked Questions (FAQs)
What are the basic requirements to make a website with Python?
To create a website with Python, you need a basic understanding of Python programming, a web framework such as Flask or Django, and knowledge of HTML, CSS, and JavaScript for front-end development. Additionally, a code editor and a local server environment are essential for testing.
Which Python web frameworks are best for beginners?
Flask is often recommended for beginners due to its simplicity and lightweight nature, allowing for quick development. Django is also a great choice, providing a more structured framework with built-in features for larger applications.
Can I use Python for both front-end and back-end development?
Python is primarily used for back-end development. However, for front-end development, you typically use HTML, CSS, and JavaScript. Tools like Brython allow you to run Python in the browser, but they are not widely adopted for production environments.
How do I deploy a Python web application?
To deploy a Python web application, you can use platforms such as Heroku, AWS, or DigitalOcean. The process typically involves setting up a server, configuring the environment, and transferring your application files, followed by running the application on the server.
What are some common challenges faced when making a website with Python?
Common challenges include managing dependencies, ensuring security, handling database connections, and optimizing performance. Additionally, debugging and testing can be more complex due to the dynamic nature of web applications.
Is it necessary to learn JavaScript when making a website with Python?
While it is not strictly necessary to learn JavaScript, having a good grasp of it is highly beneficial. JavaScript is essential for creating interactive and dynamic user interfaces, which enhances the overall user experience on your website.
In summary, creating a website with Python involves several key steps and considerations. First, selecting the right web framework is crucial, as it dictates the structure and functionality of the website. Popular frameworks such as Django and Flask offer different advantages, with Django being suited for larger applications and Flask providing simplicity for smaller projects. Understanding the framework’s features and capabilities will significantly impact the development process.
Additionally, setting up a development environment is essential for efficient coding and testing. Utilizing tools like virtual environments and package managers can help manage dependencies and ensure that the project runs smoothly. Furthermore, incorporating front-end technologies such as HTML, CSS, and JavaScript will enhance the user interface and overall user experience of the website.
Finally, deploying the website is the last step, which requires consideration of hosting options and server configurations. Platforms like Heroku, AWS, or DigitalOcean provide various services to host Python applications. It is important to ensure that the website is secure, scalable, and performs well under user traffic. By following these steps, one can successfully create a robust and functional website using Python.
Author Profile
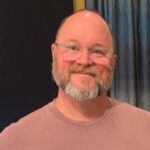
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?