How Can You Create a List of Lists in Python?
In the world of programming, particularly in Python, the ability to organize and manage data efficiently is crucial for developing robust applications. One powerful way to achieve this is by creating a list of lists, a versatile data structure that allows you to group related items together in a hierarchical manner. Whether you’re handling complex datasets, representing matrices, or simply organizing information in a structured format, mastering the art of lists within lists can significantly enhance your coding prowess.
This article will guide you through the fundamentals of making a list of lists in Python, presenting a clear and practical approach to this essential concept. You’ll discover how to create, manipulate, and access nested lists, enabling you to tackle a variety of programming challenges with ease. We’ll also explore some common use cases where lists of lists shine, providing you with a solid foundation to apply this technique in your own projects.
As we delve deeper, you’ll learn about the nuances of list comprehension, iteration, and how to leverage Python’s built-in functions to work with these nested structures effectively. By the end of this article, you’ll not only understand how to create a list of lists but also appreciate the flexibility and power they bring to your coding toolkit. Get ready to unlock new possibilities in your Python programming journey!
Creating a List of Lists
To create a list of lists in Python, you can simply define a list where each element is itself a list. This structure allows you to store multiple collections of items, making it ideal for representing matrices, grids, or any nested data structure. The syntax is straightforward, and you can initialize your list of lists in several ways.
“`python
Example of a list of lists
list_of_lists = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
“`
In this example, `list_of_lists` contains three sublists, each representing a row of numbers. You can access elements using double indexing.
Accessing Elements in a List of Lists
To access an element within a list of lists, use two indices: the first for the outer list and the second for the inner list. This allows for precise access to any item within the nested structure.
“`python
Accessing elements
element = list_of_lists[1][2] Accesses the number 6
“`
You can also loop through the list of lists to process each sublist or element:
“`python
for sublist in list_of_lists:
for item in sublist:
print(item)
“`
Modifying a List of Lists
You can modify elements in a list of lists just like any regular list. By accessing an element via its indices, you can assign a new value:
“`python
Modifying an element
list_of_lists[0][1] = 20 Changes 2 to 20
“`
This flexibility allows you to update your data structure efficiently. Additionally, you can append new sublists or elements to the existing structure:
“`python
Appending a new sublist
list_of_lists.append([10, 11, 12])
“`
Common Operations on Lists of Lists
Here are some common operations you can perform on a list of lists:
- Length of a List of Lists: Use the `len()` function to find the number of sublists.
- Flattening a List of Lists: Use list comprehension to create a single list from all elements.
- Slicing: Access a portion of the list of lists.
Operation | Example | Result |
---|---|---|
Length | len(list_of_lists) | 3 |
Flatten | [item for sublist in list_of_lists for item in sublist] | [1, 20, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12] |
Slicing | list_of_lists[1:3] | [[4, 5, 6], [7, 8, 9]] |
These operations enhance your ability to manage and manipulate nested lists effectively in Python, enabling you to handle complex data structures with ease.
Creating a List of Lists
In Python, a list of lists can be created by appending lists to a parent list. This structure allows for a two-dimensional array-like format, which is useful for representing grids, matrices, or collections of related items.
To create a list of lists, you can follow these steps:
- Initialize the Parent List: Start by defining an empty list.
- Append Inner Lists: Use the `append()` method to add inner lists to the parent list.
Here is an example illustrating these steps:
“`python
Initialize the parent list
list_of_lists = []
Append inner lists
list_of_lists.append([1, 2, 3])
list_of_lists.append([4, 5, 6])
list_of_lists.append([7, 8, 9])
“`
After executing the above code, `list_of_lists` will contain: `[[1, 2, 3], [4, 5, 6], [7, 8, 9]]`.
Accessing Elements
Accessing elements in a list of lists is straightforward. You can access an inner list by its index and then access individual elements from that inner list.
For example:
“`python
Access the second inner list
second_inner_list = list_of_lists[1] Returns [4, 5, 6]
Access an element from the second inner list
element = list_of_lists[1][2] Returns 6
“`
Modifying Elements
To modify elements within a list of lists, you can specify the index of the inner list and the index of the element you wish to change.
Example:
“`python
Modify an element
list_of_lists[0][1] = 20 Changes the second element of the first inner list to 20
“`
After this modification, `list_of_lists` will look like: `[[1, 20, 3], [4, 5, 6], [7, 8, 9]]`.
Iterating Through a List of Lists
Iterating through a list of lists can be accomplished using nested loops. This approach allows you to access each element in the sublists.
Here is a simple example:
“`python
for inner_list in list_of_lists:
for item in inner_list:
print(item)
“`
This will print each item in the list of lists sequentially.
Example of a List of Lists
To further illustrate the concept, consider the following table representing students and their grades:
Student | Grades |
---|---|
Alice | [85, 90, 78] |
Bob | [82, 88, 91] |
Charlie | [90, 95, 92] |
In Python, this can be represented as:
“`python
grades = [
[“Alice”, [85, 90, 78]],
[“Bob”, [82, 88, 91]],
[“Charlie”, [90, 95, 92]]
]
“`
This structure allows for easy access to both student names and their respective grades.
Using List Comprehensions
List comprehensions can be utilized to create a list of lists concisely. For example, if you wanted to create a list of squares of numbers from 1 to 3 for each inner list:
“`python
list_of_squares = [[x**2 for x in range(1, 4)] for _ in range(3)]
“`
This results in `[[1, 4, 9], [1, 4, 9], [1, 4, 9]]`. Each inner list contains the squares of the numbers 1, 2, and 3.
The flexibility of lists of lists in Python allows for organized and efficient data management. By following the outlined methods for creating, accessing, modifying, and iterating over these structures, you can effectively manage multi-dimensional data in your applications.
Expert Insights on Creating Lists of Lists in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Creating a list of lists in Python is a fundamental skill for any data scientist. It allows for efficient data organization and manipulation, especially when dealing with multi-dimensional datasets. Utilizing nested lists can significantly enhance the performance of algorithms that require structured data input.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “When implementing a list of lists in Python, it’s crucial to understand the implications of mutable data types. Each sublist can be modified independently, which is beneficial for dynamic data structures. However, developers must be cautious about unintended side effects when sharing references among lists.”
Sarah Patel (Python Instructor, LearnPython Academy). “Teaching how to create and manipulate lists of lists in Python is essential for beginners. It lays the groundwork for understanding more complex data structures, such as dictionaries and sets. I encourage students to practice by creating matrices or grids, which can solidify their grasp of nested lists.”
Frequently Asked Questions (FAQs)
What is a list of lists in Python?
A list of lists in Python is a nested data structure where each element of the main list is itself a list. This allows for the organization of data in a multi-dimensional format, similar to a table or matrix.
How do I create a list of lists in Python?
You can create a list of lists by initializing a main list and appending sublists to it. For example: `list_of_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]`.
How can I access elements in a list of lists?
You can access elements using double indexing. For instance, `list_of_lists[0][1]` retrieves the second element of the first sublist, which is `2` in the example above.
What are some common use cases for a list of lists?
Common use cases include representing matrices, storing tabular data, and organizing complex data structures such as graphs or grids.
How can I iterate through a list of lists?
You can use nested loops to iterate through a list of lists. For example:
“`python
for sublist in list_of_lists:
for item in sublist:
print(item)
“`
Can I modify elements in a list of lists?
Yes, you can modify elements by accessing them through their indices. For example, `list_of_lists[1][2] = 10` changes the third element of the second sublist to `10`.
Creating a list of lists in Python is a straightforward process that leverages the versatility of the list data structure. A list of lists can be used to represent complex data sets, such as matrices or tables, where each sub-list can be thought of as a row containing multiple elements. This structure allows for easy access and manipulation of nested data, making it a powerful tool for various programming tasks.
To construct a list of lists, one can simply define a list and include other lists as its elements. This can be done through direct assignment or by using loops to populate the sub-lists dynamically. Accessing elements within a list of lists requires indexing, where the first index refers to the outer list and the second index refers to the inner list. This hierarchical structure enables efficient organization and retrieval of data.
In addition to basic creation and access, Python offers numerous built-in functions and methods that facilitate operations on lists of lists. For instance, list comprehensions can be employed to generate new lists based on existing ones, while functions like `append()` and `extend()` can be used to modify the structure. Understanding these capabilities enhances a programmer’s ability to work with complex data structures effectively.
Author Profile
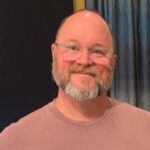
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?