How Can You Create a Copy of a List in Python?
In the world of programming, efficiency and precision are paramount, especially when it comes to managing data structures like lists in Python. Whether you’re a seasoned developer or a curious beginner, understanding how to manipulate lists effectively can significantly enhance your coding prowess. One fundamental operation that often arises is the need to create a copy of a list. This seemingly simple task can have profound implications for how your code behaves, particularly in terms of memory management and data integrity. In this article, we’ll delve into the various methods of duplicating lists in Python, ensuring you have the tools you need to avoid common pitfalls and optimize your programs.
When you create a copy of a list, it’s crucial to grasp the difference between shallow and deep copies. A shallow copy creates a new list that references the original elements, while a deep copy generates a completely independent clone of the list and its contents. This distinction can lead to unexpected behavior if not understood properly, especially when dealing with nested lists or mutable objects. As we explore the different techniques available, you’ll learn how to choose the right method based on your specific use case, ensuring that your data remains intact and your code runs smoothly.
In addition to the traditional approaches, Python offers a variety of built-in functions and libraries that can simplify the copying process.
Methods to Copy a List in Python
Copying a list in Python can be achieved through several methods, each with its own characteristics and use cases. Below are some of the most common techniques for duplicating lists.
Using the `copy()` Method
The `copy()` method is a straightforward way to create a shallow copy of a list. This method returns a new list containing the same elements as the original.
“`python
original_list = [1, 2, 3, 4, 5]
copied_list = original_list.copy()
“`
Using List Slicing
List slicing is another popular method for copying a list. By specifying the entire list in the slice, Python creates a new list with the same elements.
“`python
original_list = [1, 2, 3, 4, 5]
copied_list = original_list[:]
“`
Using the `list()` Constructor
The `list()` constructor can also be used to create a copy of a list. This method is particularly useful when converting other iterable types into a list format.
“`python
original_list = [1, 2, 3, 4, 5]
copied_list = list(original_list)
“`
Using the `copy` Module for Deep Copies
For lists containing nested objects, a shallow copy may not suffice. In such cases, the `copy` module’s `deepcopy()` function is necessary to ensure that all objects within the list are copied recursively.
“`python
import copy
original_list = [[1, 2], [3, 4]]
copied_list = copy.deepcopy(original_list)
“`
Comparing Different Copying Methods
The following table summarizes the various methods of copying a list, their types, and their use cases:
Method | Type | Use Case |
---|---|---|
copy() | Shallow Copy | Basic duplication of a list |
Slicing | Shallow Copy | Quick and concise copying |
list() | Shallow Copy | Converting iterables to lists |
copy.deepcopy() | Deep Copy | Handling nested lists |
Performance Considerations
When choosing a method to copy a list, performance may vary. For small lists, differences are negligible, but for larger lists, the efficiency of the method can impact your program’s performance. Generally, list slicing and the `copy()` method are faster than using the `list()` constructor or the `copy.deepcopy()` method. Always consider the structure of your data and the depth of copying required when selecting an approach.
Methods to Copy a List in Python
To create a copy of a list in Python, several methods can be employed, each with its advantages depending on the specific use case. Below are the most common techniques.
Using the `list()` Constructor
The `list()` constructor is a straightforward way to create a copy of an existing list. It creates a new list that contains the same elements as the original.
“`python
original_list = [1, 2, 3, 4]
copied_list = list(original_list)
“`
- Pros: Simple and easy to understand.
- Cons: May not be as efficient as other methods for very large lists.
Using List Slicing
List slicing is a Pythonic way to copy a list. By slicing the entire list, you create a new one that includes all elements.
“`python
original_list = [1, 2, 3, 4]
copied_list = original_list[:]
“`
- Pros: Elegant syntax, commonly used in Python.
- Cons: Still copies all elements, which may be inefficient for large lists.
Using the `copy()` Method
The `copy()` method is available on list objects and provides a clear intention of copying the list.
“`python
original_list = [1, 2, 3, 4]
copied_list = original_list.copy()
“`
- Pros: Explicit and readable.
- Cons: Requires Python 3.3 or higher.
Using the `copy` Module
For more complex copying needs, especially when dealing with nested lists, the `copy` module’s `deepcopy()` function can be used. This method creates a new list with recursively copied elements.
“`python
import copy
original_list = [[1, 2], [3, 4]]
copied_list = copy.deepcopy(original_list)
“`
- Pros: Handles nested structures effectively.
- Cons: Slower than other methods due to recursive copying.
Performance Considerations
When choosing a method to copy a list, consider the following performance aspects:
Method | Time Complexity | Space Complexity | Use Case |
---|---|---|---|
`list()` Constructor | O(n) | O(n) | Basic list copying |
List Slicing | O(n) | O(n) | Pythonic and concise |
`copy()` Method | O(n) | O(n) | Clearer intention for copying |
`deepcopy()` | O(n) | O(n) | Nested lists and complex objects |
Selecting the appropriate method will depend on factors such as readability, performance requirements, and the complexity of the data structures involved.
Expert Insights on Copying Lists in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Creating a copy of a list in Python is fundamental for maintaining data integrity, especially when working with mutable objects. Utilizing the built-in list methods like `copy()` or slicing with `[:]` can prevent unintended side effects, ensuring that the original list remains unchanged during operations.”
Mark Thompson (Python Developer Advocate, CodeMasters). “When copying lists in Python, it is crucial to understand the difference between shallow and deep copies. The `copy` module provides a `deepcopy()` function that is essential when your list contains nested objects. This ensures that all levels of data are duplicated, avoiding potential pitfalls related to references.”
Linda Garcia (Data Scientist, Analytics Solutions). “In data manipulation, making a copy of a list is often necessary to avoid altering the original dataset. Techniques such as list comprehension or the `list()` constructor can be effective for creating copies. Choosing the right method depends on the specific requirements of your data processing task.”
Frequently Asked Questions (FAQs)
How do I create a shallow copy of a list in Python?
You can create a shallow copy of a list using the `list()` constructor or by slicing. For example, `copy_list = list(original_list)` or `copy_list = original_list[:]` will both create a shallow copy.
What is the difference between a shallow copy and a deep copy?
A shallow copy creates a new list containing references to the original objects, while a deep copy creates a new list and recursively copies all objects found in the original list, resulting in completely independent objects.
How can I make a deep copy of a list in Python?
To make a deep copy of a list, use the `copy` module’s `deepcopy()` function. For example, `import copy; deep_copy_list = copy.deepcopy(original_list)` ensures that all nested objects are also copied.
Is using the `copy()` method a good way to copy a list?
Yes, using the `copy()` method is a valid way to create a shallow copy of a list. For example, `copy_list = original_list.copy()` achieves this effectively.
What happens if I modify a shallow copied list?
If you modify a shallow copied list, changes to the mutable objects within the list will affect the original list, as both lists reference the same objects. However, adding or removing elements from the copied list will not affect the original list.
Can I copy a list of lists in Python?
Yes, you can copy a list of lists. To create a shallow copy, use slicing or the `copy()` method. For a deep copy, utilize `copy.deepcopy()`, which ensures that all inner lists are also copied independently.
In Python, creating a copy of a list is a fundamental operation that can be accomplished through various methods. The most common techniques include using the list slicing method, the `list()` constructor, and the `copy()` method. Each of these methods serves the purpose of duplicating the original list while ensuring that modifications to the new list do not affect the original list.
It is essential to understand the distinction between shallow and deep copies when working with lists containing nested objects. A shallow copy creates a new list containing references to the original list’s elements, while a deep copy creates a completely independent copy of the entire list, including all nested objects. Utilizing the `copy` module’s `deepcopy()` function is crucial when dealing with complex data structures that require a full duplication.
In summary, mastering the various techniques for copying lists in Python is vital for effective programming. Understanding when to use shallow versus deep copies can prevent unintended side effects and ensure data integrity. By employing these methods appropriately, developers can enhance their code’s reliability and maintainability.
Author Profile
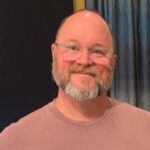
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?