How Can You Effectively Join Three Tables in SQL?
Joining tables in SQL is a fundamental skill for anyone looking to harness the full power of relational databases. As data becomes increasingly interconnected, the ability to combine information from multiple sources is essential for generating meaningful insights and making informed decisions. Whether you’re a seasoned database administrator or a newcomer to the world of SQL, understanding how to join three tables effectively can elevate your data manipulation capabilities and enhance your analytical prowess.
In the realm of SQL, joining tables allows you to create a unified view of your data by linking related information across different tables. This process is not merely about merging rows; it involves understanding the relationships between tables and the significance of each join type. By mastering the techniques for joining three tables, you can unlock complex queries that reveal deeper insights and patterns hidden within your data.
As we delve into the intricacies of multi-table joins, we will explore the various types of joins available in SQL, including inner joins, outer joins, and self-joins. Each type serves a unique purpose and can be strategically employed to achieve specific results. With practical examples and clear explanations, you’ll soon be equipped to tackle even the most challenging data scenarios, transforming how you interact with your databases.
Understanding Joins in SQL
Joining three tables in SQL requires a clear understanding of the types of joins available and how they relate to the tables in question. The most common types of joins are INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN.
- INNER JOIN: Returns records that have matching values in both tables.
- LEFT JOIN: Returns all records from the left table and the matched records from the right table. If there is no match, NULL values are returned for columns from the right table.
- RIGHT JOIN: Returns all records from the right table and the matched records from the left table. If there is no match, NULL values are returned for columns from the left table.
- FULL JOIN: Returns all records when there is a match in either left or right table records.
When joining three tables, you can combine these joins to fetch the desired results based on the relationships among the tables.
Example of Joining Three Tables
Consider three tables: Customers, Orders, and Products.
- Customers: Contains customer details.
- `CustomerID`
- `CustomerName`
- Orders: Contains order details.
- `OrderID`
- `CustomerID`
- `ProductID`
- Products: Contains product details.
- `ProductID`
- `ProductName`
The relationships are as follows:
- Each order is associated with one customer (CustomerID).
- Each order is linked to one product (ProductID).
To join these three tables, you can use the following SQL query:
“`sql
SELECT
Customers.CustomerName,
Orders.OrderID,
Products.ProductName
FROM
Customers
INNER JOIN
Orders ON Customers.CustomerID = Orders.CustomerID
INNER JOIN
Products ON Orders.ProductID = Products.ProductID;
“`
This query will return a result set that includes customer names, order IDs, and product names for all matching entries across the three tables.
Resulting Data Structure
The result of the above SQL query will yield a structured dataset as shown in the table below:
Customer Name | Order ID | Product Name |
---|---|---|
John Doe | 101 | Laptop |
Jane Smith | 102 | Smartphone |
Emily Johnson | 103 | Tablet |
This table illustrates how the information from the three tables is combined based on the specified joins. Adjusting the type of join used can significantly alter the resulting dataset, allowing for flexibility in querying relational databases.
Understanding Joins in SQL
In SQL, joins are used to combine rows from two or more tables based on related columns. The primary types of joins include:
- INNER JOIN: Returns records that have matching values in both tables.
- LEFT JOIN (or LEFT OUTER JOIN): Returns all records from the left table, and the matched records from the right table. If no match is found, NULL values are returned for columns from the right table.
- RIGHT JOIN (or RIGHT OUTER JOIN): Returns all records from the right table, and the matched records from the left table. If no match is found, NULL values are returned for columns from the left table.
- FULL JOIN (or FULL OUTER JOIN): Returns all records when there is a match in either left or right table records. Non-matching rows will return NULL values for the columns of the other table.
Joining Three Tables
To join three tables, you can use multiple JOIN clauses in a single SQL query. Below is a general syntax structure for joining three tables:
“`sql
SELECT columns
FROM table1
JOIN table2 ON table1.common_column = table2.common_column
JOIN table3 ON table2.common_column = table3.common_column
WHERE condition;
“`
Example
Assuming we have three tables: `employees`, `departments`, and `projects`, and we want to retrieve employee names, their department names, and project titles.
“`sql
SELECT e.name AS EmployeeName, d.name AS DepartmentName, p.title AS ProjectTitle
FROM employees e
JOIN departments d ON e.department_id = d.id
JOIN projects p ON e.project_id = p.id;
“`
Considerations When Joining Tables
When joining multiple tables, consider the following best practices:
- Use Aliases: As shown in the example, using aliases (e.g., `e`, `d`, `p`) helps make the query more readable.
- Specify Columns: Instead of using `SELECT *`, specify the columns needed to enhance performance.
- Filter Results: Use the `WHERE` clause to filter results and improve efficiency.
- Check for Duplicates: Be aware of potential duplicate rows when joining tables, especially with many-to-many relationships.
Common Issues with Multi-Table Joins
Joining three tables can sometimes lead to complications. Here are common issues to watch for:
Issue | Description |
---|---|
Cartesian Product | Occurs when there are no matching conditions in joins, leading to a large result set. |
Performance Concerns | More joins can slow down query performance; index optimization is crucial. |
Ambiguous Column Names | If different tables have columns with the same name, specify the table alias. |
By adhering to these guidelines and understanding the join types, one can effectively retrieve and manipulate data from multiple tables in SQL.
Expert Insights on Joining Three Tables in SQL
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “Joining three tables in SQL requires a clear understanding of the relationships between the tables. It is essential to use the appropriate join types—INNER JOIN, LEFT JOIN, or RIGHT JOIN—based on the data retrieval needs. Proper indexing can also significantly enhance performance when executing complex queries involving multiple tables.”
Michael Thompson (Senior Data Analyst, Insight Analytics). “When joining three tables, ensure that the join conditions are correctly specified to avoid Cartesian products, which can lead to inflated result sets. Utilizing aliases for table names can improve readability and maintainability of the SQL queries, especially in complex joins.”
Lisa Patel (SQL Trainer and Consultant, DataWise Solutions). “A common practice when joining multiple tables is to start with the most restrictive join first, which can help in reducing the dataset size early on. Additionally, using Common Table Expressions (CTEs) can simplify the structure of your queries and make them easier to understand and debug.”
Frequently Asked Questions (FAQs)
How do I join three tables in SQL?
To join three tables in SQL, use the JOIN clause for each table. For example:
“`sql
SELECT columns
FROM table1
JOIN table2 ON table1.id = table2.foreign_id
JOIN table3 ON table2.id = table3.foreign_id;
“`
What types of joins can be used when joining three tables?
You can use INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN when joining three tables, depending on the results you want to achieve.
Can I join three tables without a common key?
Yes, you can join three tables without a common key using CROSS JOIN, which produces a Cartesian product of the tables, but this is generally not recommended due to the large number of resulting rows.
What is the syntax for joining three tables using LEFT JOIN?
The syntax for joining three tables using LEFT JOIN is as follows:
“`sql
SELECT columns
FROM table1
LEFT JOIN table2 ON table1.id = table2.foreign_id
LEFT JOIN table3 ON table2.id = table3.foreign_id;
“`
Is it possible to join more than three tables in SQL?
Yes, it is possible to join more than three tables in SQL by continuing to add additional JOIN clauses for each subsequent table.
What should I consider when joining multiple tables?
Consider the relationships between the tables, the type of join needed, the performance impact of the query, and the specific columns you wish to retrieve to ensure efficient data retrieval.
Joining three tables in SQL is a fundamental operation that enables users to combine data from multiple sources into a single query result. This process typically involves using the JOIN clause, which allows for various types of joins such as INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN. Understanding the relationships between the tables is crucial, as it dictates the type of join to be used and how the data will be combined. Properly structuring the SQL query ensures that the desired data is accurately retrieved while maintaining data integrity.
When performing joins, it is essential to specify the correct join conditions, typically using the ON clause to define how the tables relate to one another. This involves identifying the primary and foreign keys that establish the relationships between the tables. Additionally, using aliases can enhance readability and simplify complex queries, especially when dealing with multiple tables. By effectively using these techniques, users can extract meaningful insights from their datasets.
Moreover, performance considerations should not be overlooked when joining multiple tables. The size of the tables and the efficiency of the join operations can significantly impact query performance. It is advisable to analyze the execution plan and optimize queries as necessary, utilizing indexes where appropriate. By mastering the art of joining three tables, users can unlock the
Author Profile
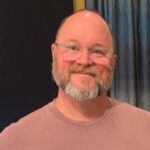
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?