How Can You Insert a Rule to Make Code Stop in Python?
In the world of programming, the ability to control the flow of your code is paramount. Whether you’re debugging an issue or simply trying to create a more efficient program, knowing how to insert rules that effectively halt execution can save you time and frustration. Python, with its clean syntax and powerful capabilities, offers several methods to manage your code’s behavior. In this article, we will explore the various techniques you can employ to make your Python code stop at specific points, allowing for greater control and precision in your programming endeavors.
When working with Python, understanding how to insert rules that dictate when your code should pause or stop is essential for both novice and experienced developers. This process can involve the use of conditional statements, exception handling, or even debugging tools that allow you to set breakpoints. Each method serves a unique purpose, and knowing when to apply them can significantly enhance your coding efficiency and effectiveness.
Moreover, the ability to halt execution can be particularly beneficial during the testing phase of development. By strategically placing stopping points in your code, you can better analyze its behavior, identify bugs, and ensure that your program runs as intended. As we delve deeper into this topic, you’ll discover practical examples and best practices that will empower you to master the art of code control in
Understanding Exception Handling in Python
In Python, managing how and when your code stops executing can be effectively handled using exceptions. Exception handling allows your program to respond to errors gracefully rather than crashing unexpectedly. By utilizing try, except, and finally blocks, you can insert rules that dictate how your code should behave under various circumstances.
- try block: The code that might cause an exception is placed inside this block.
- except block: Code that runs if an exception occurs in the try block.
- finally block: Code that will run regardless of whether an exception occurred, often used for cleanup actions.
Inserting Rules to Control Execution Flow
To insert rules that dictate how your code should stop or handle errors, you can implement specific exceptions. Below is an example demonstrating how to stop code execution based on user-defined conditions:
“`python
def check_value(value):
if value < 0:
raise ValueError("Negative value not allowed!")
return value
try:
result = check_value(-10)
except ValueError as e:
print(f"Error: {e}")
```
In this example, if the `check_value` function receives a negative input, it raises a `ValueError`, which is caught in the except block. This prevents the program from crashing and allows you to define a clear message for the user.
Using Assertions to Stop Execution
Assertions in Python are a convenient way to insert rules that can stop execution if certain conditions are not met. An assertion checks a condition and raises an `AssertionError` if the condition evaluates to .
“`python
def divide(a, b):
assert b != 0, “The denominator cannot be zero!”
return a / b
try:
result = divide(10, 0)
except AssertionError as e:
print(f”Assertion Error: {e}”)
“`
In this code snippet, the assertion checks that the denominator is not zero before performing the division. If `b` is zero, the assertion will raise an error, halting further execution and allowing for appropriate error handling.
Creating Custom Exceptions
Custom exceptions allow you to define more specific error types that can provide additional context for debugging. Here’s how to create and use a custom exception in Python:
“`python
class CustomError(Exception):
pass
def risky_function():
raise CustomError(“This is a custom error message.”)
try:
risky_function()
except CustomError as e:
print(f”Caught an error: {e}”)
“`
By creating a `CustomError` class, you can raise and catch exceptions that are specific to your application, making your error handling more robust.
Exception Type | When to Use |
---|---|
ValueError | When a function receives an argument of the right type but inappropriate value. |
TypeError | When an operation or function is applied to an object of inappropriate type. |
CustomError | When you need to define specific error conditions unique to your application. |
Inserting Rules to Control Code Execution in Python
To manage the flow of execution in Python effectively, particularly in scenarios where certain conditions dictate whether the code should continue running or halt, various strategies can be employed. The most common methods include using conditional statements, exception handling, and control flow mechanisms.
Conditional Statements
Conditional statements allow you to execute specific blocks of code based on defined conditions. The primary structures include `if`, `elif`, and `else`.
“`python
if condition:
Code to execute if condition is true
elif another_condition:
Code to execute if another_condition is true
else:
Code to execute if none of the conditions are true
“`
– **Example**: This example checks if a variable exceeds a certain value, stopping execution if it does.
“`python
value = 10
if value > 10:
print(“Value exceeds 10, stopping execution.”)
exit() Terminates the program
“`
Using Exceptions to Control Flow
Exceptions in Python can also be used to halt execution. Utilizing `try` and `except` blocks, you can define rules that terminate or alter the flow based on error conditions.
“`python
try:
Code that may cause an exception
risky_operation()
except SpecificException as e:
print(f”An error occurred: {e}”)
exit() Stops execution on encountering the specified exception
“`
- When to Use:
- To catch and handle unexpected errors gracefully.
- To enforce rules that, when violated, require the program to stop.
Control Flow with Functions
Functions can encapsulate rules that control execution. Using `return` statements, you can stop the execution of a function and, by extension, the program if certain criteria are not met.
“`python
def check_value(value):
if value < 0:
print("Negative value detected, halting execution.")
return Stops function execution
Continue with further processing
print("Processing value...")
```
- Best Practices:
- Ensure functions return clear indicators of failure or success.
- Use meaningful messages to clarify the reason for halting execution.
Using Flags for Control Flow
Flags are boolean variables that can be used to control the execution of code blocks. Setting a flag to `True` or “ can dictate whether certain operations are performed.
“`python
stop_execution =
if some_condition:
stop_execution = True
if stop_execution:
print(“Execution stopped based on flag.”)
else:
Continue with the rest of the code
“`
- Advantages:
- Provides flexibility in managing code execution.
- Enhances readability by clearly showing the conditions under which execution halts.
Summary of Control Mechanisms
Mechanism | Description | Use Case |
---|---|---|
Conditional Statements | Execute code based on conditions | Basic flow control |
Exception Handling | Stop execution on errors | Handling unexpected situations |
Functions | Use return statements to control execution | Modular code with clear exit points |
Flags | Boolean variables to dictate flow | Simplified control over execution |
Implementing these strategies will provide robust mechanisms to insert rules and control the execution of your Python code effectively.
Strategies for Implementing Rule-Based Code Control in Python
Dr. Emily Carter (Senior Software Engineer, Code Solutions Inc.). “Inserting rules to control the flow of code execution in Python can significantly enhance your program’s robustness. Utilizing exception handling and conditional statements allows developers to create clear pathways for code execution, effectively stopping or altering the flow based on predefined rules.”
Michael Chen (Lead Developer, Python Innovations). “To effectively implement rules that stop code execution in Python, consider using decorators or context managers. These tools provide a flexible way to enforce rules dynamically, allowing for cleaner and more maintainable code structures that can adapt to changing requirements.”
Sarah Thompson (Technical Consultant, Agile Development Group). “Incorporating rule-based logic into your Python applications can be achieved through the use of libraries such as Pydantic for data validation or custom exception classes. This approach not only stops code execution when rules are violated but also provides informative feedback to developers and users alike.”
Frequently Asked Questions (FAQs)
How can I insert a rule to make my Python code stop executing?
You can use the `raise` statement to trigger an exception, which will stop the execution of your code. For example, `raise Exception(“Stopping execution”)` will halt the program when this line is reached.
What is the purpose of using `sys.exit()` in Python?
The `sys.exit()` function is used to exit from Python. It raises a `SystemExit` exception, which can be caught in outer layers of the program. This function allows for a clean exit from the program, optionally providing an exit status.
Can I use conditions to stop code execution in Python?
Yes, you can use conditional statements to control the flow of your code. For instance, using an `if` statement to check a condition and then calling `sys.exit()` or raising an exception will stop execution based on that condition.
How do I implement a timeout to stop code execution after a certain period?
You can use the `signal` module to set an alarm that raises an exception after a specified time. For example, `signal.alarm(seconds)` can be used to trigger a `SIGALRM` after the given number of seconds, allowing you to handle the timeout gracefully.
Is there a way to stop a loop in Python based on a specific rule?
Yes, you can use the `break` statement within a loop to exit it when a specific condition is met. For example, `if condition: break` will terminate the loop when the condition evaluates to true.
What are best practices for stopping code execution in Python?
Best practices include using exceptions for error handling, employing `sys.exit()` for graceful exits, and ensuring that your code is structured to handle unexpected terminations without leaving resources open or in an inconsistent state.
Inserting rules to make code stop in Python is an essential skill for developers who wish to control the flow of their programs effectively. The primary methods to achieve this include using conditional statements, exceptions, and specific control flow statements such as ‘break’ and ‘return’. Each of these techniques allows developers to dictate when and how their code should terminate or skip certain sections, enhancing both functionality and user experience.
Understanding the context in which to apply these rules is crucial. For instance, using ‘break’ within loops can help exit the loop prematurely based on certain conditions, while ‘return’ is vital for exiting functions and returning values. Additionally, employing exceptions provides a robust way to handle errors and unexpected conditions, allowing for graceful degradation of functionality without crashing the program. Mastery of these concepts leads to cleaner, more maintainable code.
Moreover, it is important to consider the implications of stopping code execution. Developers should ensure that any rules implemented do not inadvertently lead to incomplete processes or data loss. Proper testing and validation of the implemented rules can help mitigate such risks. By strategically inserting rules to control code execution, developers can create more reliable and efficient Python applications.
Author Profile
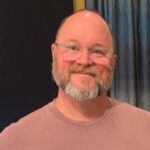
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?