How Can You Insert a Null Value in SQL Effectively?
In the world of databases, the ability to manage and manipulate data effectively is paramount. One of the key concepts that every SQL user must grasp is the handling of null values. Whether you’re designing a new database, updating existing records, or performing complex queries, understanding how to insert a null value in SQL can significantly impact the integrity and functionality of your data. This seemingly simple task can sometimes lead to confusion, especially for those new to SQL or database management. In this article, we will demystify the process of inserting null values, ensuring you have the knowledge to maintain the accuracy of your datasets.
At its core, a null value in SQL signifies the absence of data. It is not the same as an empty string or a zero; rather, it represents an unknown or missing value. This distinction is crucial for database integrity and can affect how queries are executed and how results are interpreted. When inserting data into a table, knowing when and how to use null values can help you avoid errors and ensure that your database reflects the real-world scenarios it is meant to represent.
Inserting null values is a fundamental skill that can enhance your database management capabilities. It involves understanding the syntax and context in which nulls can be appropriately applied. By mastering this aspect of SQL, you will not
Understanding Null Values in SQL
In SQL, a null value represents the absence of a value or an unknown value. It is important to distinguish null from an empty string or a zero value, as these represent different concepts. Null values can be used in various scenarios, such as when data is not applicable, missing, or yet to be collected.
To effectively manage null values, it is essential to understand the implications of inserting them into a database. For instance, null values can affect the outcome of aggregate functions, comparisons, and conditional statements.
Inserting Null Values in SQL
When inserting data into a SQL table, it is possible to explicitly insert null values. This can be done in various ways, depending on the context of the insert operation. Below are common methods for inserting null values:
- Explicitly specifying null: When inserting a row, you can explicitly set a column to null.
- Omitting the column: If you do not specify a value for a column that allows nulls, SQL automatically assigns a null value.
Here is a basic syntax for inserting null values:
“`sql
INSERT INTO table_name (column1, column2, column3) VALUES (value1, NULL, value3);
“`
Alternatively, if `column2` is designed to accept nulls, you can skip it altogether:
“`sql
INSERT INTO table_name (column1, column3) VALUES (value1, value3);
“`
This automatically assigns a null value to `column2`.
Example of Inserting Null Values
Consider the following table structure:
“`sql
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
MiddleName VARCHAR(50) NULL
);
“`
To insert a new employee without a middle name, you can use either of the following approaches:
“`sql
INSERT INTO Employees (EmployeeID, FirstName, LastName, MiddleName) VALUES (1, ‘John’, ‘Doe’, NULL);
“`
or omit the `MiddleName` column:
“`sql
INSERT INTO Employees (EmployeeID, FirstName, LastName) VALUES (2, ‘Jane’, ‘Smith’);
“`
In both cases, `MiddleName` will contain a null value for the new records.
Considerations When Using Null Values
While null values can be useful, they also come with certain considerations:
- Search behavior: Null values are not equal to any other value, including another null. Therefore, comparisons using `=` or `!=` will not yield true when nulls are involved. Use `IS NULL` or `IS NOT NULL` for checks.
- Aggregate functions: Functions like `COUNT()`, `SUM()`, and `AVG()` ignore null values. This can impact results when performing calculations.
Here’s a simple table illustrating null behavior:
Column | Value | Result of Comparison (`= NULL`) |
---|---|---|
Column with NULL | NULL | |
Column with 5 | 5 | |
Column with 0 | 0 |
Understanding how to manage null values in SQL is crucial for maintaining data integrity and accurately interpreting query results.
Understanding NULL Values in SQL
In SQL, a NULL value represents the absence of a value or an unknown value. It is important to differentiate NULL from an empty string or a zero; NULL signifies that no data is present. When designing databases, understanding how to handle NULL values is crucial for maintaining data integrity.
Inserting NULL Values
To insert a NULL value into a database table, you can use the SQL `INSERT` statement. The syntax allows you to specify NULL explicitly in place of a value. Below are the key points to consider:
- Use the keyword `NULL` without quotes.
- Ensure the column allows NULL values; otherwise, the operation will fail.
Basic Syntax for Inserting NULL
The basic syntax to insert a NULL value is as follows:
“`sql
INSERT INTO table_name (column1, column2, column3)
VALUES (value1, NULL, value3);
“`
For example, if you have a table named `employees` with columns `id`, `name`, and `department`, you can insert a NULL value into the `department` column as shown below:
“`sql
INSERT INTO employees (id, name, department)
VALUES (1, ‘John Doe’, NULL);
“`
Using NULL in UPDATE Statements
You can also set a column to NULL using an `UPDATE` statement. This is useful when you want to clear an existing value. The syntax is:
“`sql
UPDATE table_name
SET column_name = NULL
WHERE condition;
“`
For instance, to update the department of an employee to NULL:
“`sql
UPDATE employees
SET department = NULL
WHERE id = 1;
“`
Considerations When Using NULL
When working with NULL values, keep the following considerations in mind:
- NULL Comparisons: Use `IS NULL` or `IS NOT NULL` for comparisons, as NULL cannot be compared using standard equality operators (`=`, `!=`).
- Aggregate Functions: Most aggregate functions (e.g., `COUNT`, `SUM`, `AVG`) ignore NULL values. This can affect query results.
- Default Values: If a column has a default value and you insert NULL, the default is not applied.
Examples of NULL Handling
Operation | SQL Statement |
---|---|
Insert NULL | `INSERT INTO table_name (column1) VALUES (NULL);` |
Update to NULL | `UPDATE table_name SET column1 = NULL WHERE id = 1;` |
Select NULL Values | `SELECT * FROM table_name WHERE column1 IS NULL;` |
Conclusion on NULL Value Management
Handling NULL values in SQL is essential for accurate data representation and retrieval. By understanding how to insert and manage NULL values, you can ensure that your database operations are robust and reliable.
Expert Insights on Inserting Null Values in SQL
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). Inserting a null value in SQL is essential for accurately representing missing or unknown data. It is crucial to understand that a null value is not the same as an empty string or zero; it signifies the absence of a value. Using the `INSERT` statement, you can specify a null value by either omitting the column from the statement or explicitly using the keyword NULL.
Marcus Lee (Senior SQL Developer, Data Insights Corp.). When handling null values in SQL, it is important to consider the implications for data integrity and query results. For instance, using `INSERT INTO table_name (column1, column2) VALUES (value1, NULL)` is a straightforward method. However, be aware that null values can affect aggregate functions and comparisons, leading to unexpected results if not handled properly.
Linda Tran (Data Analyst, Analytics Group). Understanding how to insert null values in SQL is fundamental for effective data management. When designing your database schema, consider how nulls will interact with constraints. For instance, if a column is defined as NOT NULL, attempting to insert a null value will result in an error. Therefore, always ensure that your database design aligns with your data requirements.
Frequently Asked Questions (FAQs)
What is a null value in SQL?
A null value in SQL represents the absence of a value or an unknown value. It is not the same as zero or an empty string; it signifies that the data is missing.
How do you insert a null value into a SQL table?
To insert a null value into a SQL table, use the keyword `NULL` in your `INSERT` statement. For example: `INSERT INTO table_name (column_name) VALUES (NULL);`.
Can you insert a null value into a non-nullable column?
No, you cannot insert a null value into a non-nullable column. Attempting to do so will result in an error, as non-nullable columns require a valid value.
What happens if you omit a column in an insert statement?
If you omit a column in an insert statement and that column allows null values, SQL will automatically insert a null value for that column. If the column is non-nullable, an error will occur.
Is it possible to update a column to null after it has a value?
Yes, you can update a column to null after it has a value, provided that the column is defined to accept null values. Use the `UPDATE` statement, such as: `UPDATE table_name SET column_name = NULL WHERE condition;`.
How can you check for null values in a SQL query?
To check for null values in a SQL query, use the `IS NULL` condition. For example: `SELECT * FROM table_name WHERE column_name IS NULL;` This will return all rows where the specified column is null.
Inserting a null value in SQL is a fundamental operation that allows for the representation of missing or unknown data within a database. Understanding how to properly implement null values is essential for maintaining data integrity and accurately reflecting real-world scenarios where information may be absent. SQL provides specific syntax and methods for inserting null values, which can vary slightly depending on the database management system being used, such as MySQL, PostgreSQL, or SQL Server.
To insert a null value, the standard approach involves using the keyword ‘NULL’ in the SQL statement. For example, when using the INSERT command, one can specify a column to receive a null value by omitting it from the list of columns or explicitly stating ‘NULL’ in the values section. It is crucial to ensure that the target column is defined to accept null values; otherwise, an error will occur. Additionally, understanding the implications of null values in queries, such as comparisons and aggregations, is vital for effective data manipulation and retrieval.
Key takeaways from the discussion include the importance of recognizing the difference between null values and empty strings or zeroes, as they represent distinct concepts in SQL. Furthermore, when designing database schemas, careful consideration should be given to which columns should allow null values
Author Profile
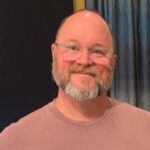
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?