How Do You Properly Initialize a Variable in Python?
In the world of programming, the ability to effectively manage and manipulate data is crucial, and at the heart of this capability lies the concept of variables. In Python, a versatile and widely-used programming language, initializing a variable is one of the first steps you’ll encounter on your coding journey. Whether you are a novice eager to grasp the fundamentals or an experienced developer brushing up on best practices, understanding how to initialize variables in Python is essential for writing clean, efficient, and functional code.
As you delve into the intricacies of Python, you’ll discover that initializing a variable is not just about assigning a value; it’s about setting the stage for your program’s logic and flow. Variables serve as containers for data, allowing you to store, modify, and retrieve information seamlessly. This process is foundational, influencing everything from simple calculations to complex algorithms. By mastering variable initialization, you lay the groundwork for more advanced programming concepts, enabling you to harness the full power of Python.
In this article, we will explore the various ways to initialize variables in Python, highlighting the syntax, conventions, and common practices that will help you write code that is both effective and easy to understand. You’ll learn how to choose appropriate data types, manage scope, and avoid common pitfalls, all of
Understanding Variable Initialization
Initializing a variable in Python involves assigning a value to it at the time of its creation. This process not only defines the variable but also allocates memory for it. In Python, this is typically done using the assignment operator `=`.
Basic Variable Initialization
To initialize a variable, simply choose a name that follows Python’s naming conventions and assign a value. For example:
“`python
x = 5
name = “Alice”
is_active = True
“`
In the above examples:
- `x` is initialized with an integer value.
- `name` is initialized with a string value.
- `is_active` is initialized with a boolean value.
Variable Naming Conventions
When naming variables, adhere to the following guidelines to ensure code readability and maintainability:
- Variable names must begin with a letter (a-z, A-Z) or an underscore (_).
- Subsequent characters can include letters, digits (0-9), or underscores.
- Avoid using Python reserved keywords such as `def`, `class`, `if`, etc.
- Variable names are case-sensitive, meaning `variable`, `Variable`, and `VARIABLE` are three distinct identifiers.
Rule | Example |
---|---|
Starts with a letter | `myVariable` |
Can include digits | `variable1` |
Can include underscores | `my_var` |
Cannot be a keyword | `class` (invalid) |
Multiple Variable Initialization
Python allows the initialization of multiple variables in a single line. This can be done through either separate assignments or tuple unpacking:
“`python
a, b, c = 1, 2, 3 Tuple unpacking
“`
Alternatively, you can assign the same value to multiple variables simultaneously:
“`python
x = y = z = 0 All variables initialized to 0
“`
Dynamic Typing in Python
Python’s dynamic typing feature means that you do not need to declare a variable’s type before assigning a value. The type is inferred from the value assigned. For example:
“`python
number = 10 Initially an integer
number = “Ten” Now a string
“`
This flexibility allows for rapid prototyping but necessitates careful management of variable types to avoid runtime errors.
Best Practices for Variable Initialization
To ensure your code is clean and efficient, consider the following best practices:
- Use meaningful names: Choose descriptive variable names that convey their purpose.
- Initialize variables before use: Avoid using variables before they have been assigned a value.
- Limit scope: Keep variable scope as narrow as possible to reduce complexity and potential bugs.
- Group related variables: When multiple variables are related, group them logically to enhance readability.
By adhering to these guidelines, you can enhance the clarity and functionality of your code, making it easier to maintain and debug.
Understanding Variable Initialization in Python
In Python, initializing a variable is a straightforward process. A variable is created the moment you assign a value to it. This can be done with a simple assignment statement.
Basic Syntax for Variable Initialization
The general syntax for initializing a variable in Python is:
“`python
variable_name = value
“`
Here, `variable_name` is the identifier you choose for the variable, and `value` can be any data type, including integers, strings, lists, or custom objects.
Examples of Variable Initialization
- Integer Initialization:
“`python
age = 30
“`
- Float Initialization:
“`python
height = 5.9
“`
- String Initialization:
“`python
name = “Alice”
“`
- List Initialization:
“`python
numbers = [1, 2, 3, 4, 5]
“`
- Dictionary Initialization:
“`python
student = {“name”: “John”, “age”: 20}
“`
Multiple Variable Initialization
Python allows the initialization of multiple variables in a single line, which can improve code readability. The syntax is as follows:
“`python
var1, var2, var3 = value1, value2, value3
“`
For example:
“`python
x, y, z = 1, 2.5, “Hello”
“`
This initializes `x` with `1`, `y` with `2.5`, and `z` with `”Hello”`.
Dynamic Typing in Python
Python is dynamically typed, meaning that the type of a variable is determined at runtime and can change as needed. This flexibility can be demonstrated as follows:
“`python
var = 10 Initially an integer
var = “Ten” Now a string
“`
Variable Naming Conventions
When initializing variables, adhere to the following naming conventions:
- Use descriptive names to indicate the purpose of the variable.
- Start with a letter or underscore, followed by letters, digits, or underscores.
- Avoid using reserved keywords (e.g., `if`, `else`, `for`, etc.).
- Follow the snake_case style for multi-word variable names (e.g., `user_age`).
Common Mistakes in Variable Initialization
Be aware of common pitfalls when initializing variables:
Mistake | Description |
---|---|
Using reserved keywords | Attempting to name a variable with a keyword. |
Starting with a number | Variable names cannot begin with a digit. |
Special characters | Using symbols like @, , or spaces in names. |
Best Practices for Variable Initialization
- Always initialize variables before using them to avoid `NameError`.
- Use meaningful names to enhance code clarity.
- Group related variables together for better organization.
- When applicable, use constants for values that should not change, typically defined using uppercase letters (e.g., `PI = 3.14`).
These guidelines and practices ensure efficient and effective variable initialization in Python, contributing to cleaner and more maintainable code.
Expert Insights on Initializing Variables in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “When initializing a variable in Python, it is essential to understand that Python is dynamically typed. This means that you do not need to declare the variable type explicitly. Simply assigning a value to a variable name initializes it, making the process straightforward and efficient for developers.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “A common practice in Python is to initialize variables with descriptive names that reflect their purpose. This not only enhances code readability but also helps in maintaining the codebase as projects scale. For instance, using ‘user_age’ instead of a generic ‘x’ can significantly improve understanding.”
Sarah Thompson (Python Educator, LearnPython Academy). “It is crucial to initialize variables before using them in your code. In Python, failing to initialize a variable can lead to a ‘NameError’ at runtime. Therefore, establishing a habit of initializing variables with a default value, such as ‘None’, can prevent potential errors and improve code robustness.”
Frequently Asked Questions (FAQs)
How do I initialize a variable in Python?
To initialize a variable in Python, simply assign a value to it using the equals sign (`=`). For example, `x = 10` initializes the variable `x` with the integer value `10`.
Can I initialize multiple variables in one line?
Yes, you can initialize multiple variables in one line by separating them with commas. For example, `a, b, c = 1, 2, 3` initializes `a`, `b`, and `c` with the values `1`, `2`, and `3`, respectively.
What types of values can I assign to a variable?
You can assign various types of values to a variable, including integers, floats, strings, lists, tuples, dictionaries, and more. For instance, `name = “Alice”` assigns a string value to the variable `name`.
Is it necessary to declare a variable type in Python?
No, Python is dynamically typed, meaning you do not need to declare a variable’s type explicitly. The type is inferred from the value assigned to the variable at runtime.
What happens if I try to use a variable before initializing it?
If you attempt to use a variable before it has been initialized, Python will raise a `NameError`, indicating that the variable is not defined.
Can I change the value of a variable after initialization?
Yes, you can change the value of a variable at any time by reassigning it. For example, after initializing `x = 10`, you can later set `x = 20`, updating its value to `20`.
In Python, initializing a variable is a straightforward process that involves assigning a value to a variable name. This can be done using the assignment operator ‘=’. Python is dynamically typed, meaning that you do not need to declare the variable type explicitly; the interpreter infers the type based on the assigned value. For example, you can initialize an integer variable with ‘x = 10’, a string with ‘name = “Alice”‘, or a list with ‘numbers = [1, 2, 3]’. This flexibility allows for rapid development and ease of use.
It is important to note that variable names in Python must adhere to specific naming conventions. They should start with a letter or an underscore, followed by letters, numbers, or underscores. Additionally, Python is case-sensitive, so ‘Variable’ and ‘variable’ would be considered two distinct identifiers. Using descriptive variable names enhances code readability and maintainability, which is crucial for collaborative projects and long-term code management.
Furthermore, Python supports multiple assignments, allowing you to initialize several variables simultaneously. For instance, ‘a, b, c = 1, 2, 3’ assigns the values 1, 2, and 3 to variables ‘a’, ‘
Author Profile
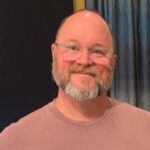
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?