How Do You Initialize an Array in Java? A Step-by-Step Guide
In the world of programming, arrays serve as a fundamental building block, allowing developers to store and manipulate collections of data efficiently. Java, one of the most widely used programming languages, offers robust support for arrays, making it essential for both beginners and seasoned developers to understand how to initialize them properly. Whether you’re building a simple application or a complex system, knowing how to effectively create and manage arrays can significantly enhance your coding prowess.
Initializing an array in Java is not just about declaring it; it involves understanding the various methods available to populate it with data. From static initialization, where values are defined at the moment of declaration, to dynamic initialization, which allows for more flexibility as data can be assigned during runtime, Java provides multiple approaches to suit different programming needs. Each method has its own advantages and use cases, making it crucial to grasp these concepts to write efficient and effective code.
As you delve deeper into the intricacies of array initialization in Java, you’ll uncover best practices, potential pitfalls, and the performance implications of each technique. This knowledge will empower you to harness the full potential of arrays, streamline your data management, and ultimately elevate your programming skills to new heights. So, let’s embark on this journey to master the art of array initialization in Java!
Array Initialization Syntax
In Java, arrays can be initialized in multiple ways. The choice of method often depends on the specific requirements of your application. Below are the most common techniques:
- Static Initialization: This method involves declaring an array and initializing it in a single line. It is useful when you know the values that should be stored in the array at the time of creation.
“`java
int[] numbers = {1, 2, 3, 4, 5};
“`
- Dynamic Initialization: You can declare an array and then allocate memory for it separately. This is particularly useful when the size of the array is not known at compile time.
“`java
int[] numbers = new int[5]; // Creates an array of size 5
“`
- Initialization with a Loop: This method allows you to fill an array with values dynamically, often using a loop.
“`java
int[] numbers = new int[5];
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i * 2; // Initialize with even numbers
}
```
Multi-Dimensional Array Initialization
Multi-dimensional arrays can be initialized in a similar fashion, allowing for more complex data structures. Here are some ways to initialize two-dimensional arrays:
- Static Initialization:
“`java
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
“`
- Dynamic Initialization:
“`java
int[][] matrix = new int[3][3]; // Creates a 3×3 matrix
“`
- Using Nested Loops:
“`java
int[][] matrix = new int[3][3];
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
matrix[i][j] = i + j; // Initialize with the sum of indices
}
}
```
Common Practices for Array Initialization
When initializing arrays, it is essential to follow best practices to ensure code clarity and maintainability. Here are some recommendations:
- Use Meaningful Names: Choose descriptive names for your arrays to convey their purpose.
- Avoid Hardcoding Values: If possible, avoid hardcoding values directly in array initialization. Consider using constants or configuration values.
- Consider Immutable Collections: For fixed data sets, consider using immutable collections like `List.of()` in Java 9 and later instead of arrays for better safety.
Method | Usage | Example |
---|---|---|
Static Initialization | When values are known at compile time | int[] arr = {1, 2, 3}; |
Dynamic Initialization | When size is known but values are not | int[] arr = new int[5]; |
Loop Initialization | When values depend on computations | for(int i = 0; i < arr.length; i++) { arr[i] = i; } |
Utilizing the appropriate method for array initialization can enhance your Java programming efficiency and code quality.
Array Initialization Syntax in Java
In Java, arrays can be initialized in several ways depending on the needs of the application. The most common methods include:
- Static Initialization: This method initializes an array at the time of declaration.
“`java
int[] numbers = {1, 2, 3, 4, 5};
“`
- Dynamic Initialization: This involves creating an array and then populating it with values later in the code.
“`java
int[] numbers = new int[5]; // Creates an array of 5 integers
numbers[0] = 1;
numbers[1] = 2;
numbers[2] = 3;
numbers[3] = 4;
numbers[4] = 5;
“`
- Using Arrays Utility Class: The `Arrays` class provides utility methods for array manipulation.
“`java
int[] numbers = new int[5];
Arrays.fill(numbers, 0); // Initializes all elements to 0
“`
Multi-dimensional Array Initialization
Java supports multi-dimensional arrays, which can be initialized similarly to single-dimensional arrays. The syntax for initializing two-dimensional arrays is as follows:
- Static Initialization:
“`java
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
“`
- Dynamic Initialization:
“`java
int[][] matrix = new int[3][3]; // Creates a 3×3 matrix
matrix[0][0] = 1;
matrix[0][1] = 2;
matrix[0][2] = 3;
// Additional assignments for other rows…
“`
Array Initialization with Objects
When working with arrays of objects, the initialization syntax is similar to that of primitive types. For example, if you have a class named `Book`, the array can be initialized as follows:
- Static Initialization:
“`java
Book[] books = {
new Book(“1984”, “George Orwell”),
new Book(“To Kill a Mockingbird”, “Harper Lee”)
};
“`
- Dynamic Initialization:
“`java
Book[] books = new Book[2]; // Creates an array for 2 Book objects
books[0] = new Book(“1984”, “George Orwell”);
books[1] = new Book(“To Kill a Mockingbird”, “Harper Lee”);
“`
Array Length and Accessing Elements
To access the length of an array, the `.length` property is used. This property returns the number of elements in the array.
- Example:
“`java
int[] numbers = {1, 2, 3, 4, 5};
int length = numbers.length; // length will be 5
“`
Accessing elements in an array is done using the index, where the first element is at index 0.
- Example:
“`java
int firstElement = numbers[0]; // Accessing the first element
“`
Best Practices for Array Initialization
When initializing arrays in Java, consider the following best practices:
- Always define the size of the array if using dynamic initialization.
- Use static initialization for known values to enhance readability.
- Be cautious of the index bounds; accessing an index outside the array size results in an `ArrayIndexOutOfBoundsException`.
- For multi-dimensional arrays, ensure that each row is initialized properly to avoid `NullPointerException`.
Utilizing these practices will lead to cleaner, more maintainable code.
Expert Insights on Initializing Arrays in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When initializing an array in Java, it is crucial to understand the different methods available, such as using array literals or the `new` keyword. Each method has its own use cases, and selecting the right one can enhance code readability and performance.”
Michael Chen (Java Developer Advocate, CodeCraft). “A common mistake among beginners is failing to specify the size of the array when using the `new` keyword. This can lead to runtime exceptions. Always ensure that the array is properly initialized to avoid such pitfalls.”
Sarah Thompson (Lead Instructor, Java Programming Bootcamp). “Understanding the difference between one-dimensional and multi-dimensional arrays is essential for effective initialization. Multi-dimensional arrays can be initialized in a nested manner, which is often overlooked by newcomers to Java.”
Frequently Asked Questions (FAQs)
How do I initialize an array in Java?
You can initialize an array in Java by using the syntax `dataType[] arrayName = new dataType[size];` or by providing values directly using curly braces, such as `dataType[] arrayName = {value1, value2, value3};`.
What are the different ways to initialize an array in Java?
Arrays in Java can be initialized in several ways: using the `new` keyword with a specified size, using an initializer list, or by using array literals. For example, `int[] numbers = new int[5];` or `int[] numbers = {1, 2, 3, 4, 5};`.
Can I initialize an array without specifying its size?
Yes, you can initialize an array without specifying its size by using an initializer list. For instance, `String[] fruits = {“Apple”, “Banana”, “Cherry”};` automatically determines the size based on the number of elements provided.
What happens if I try to access an index outside the bounds of an array?
Accessing an index outside the bounds of an array will result in an `ArrayIndexOutOfBoundsException`. This exception occurs when you attempt to access an index that is either negative or greater than or equal to the length of the array.
Can I initialize a multidimensional array in Java?
Yes, you can initialize a multidimensional array in Java using nested curly braces. For example, `int[][] matrix = {{1, 2, 3}, {4, 5, 6}};` initializes a 2D array with two rows and three columns.
What is the default value of elements in an initialized array?
The default values of elements in an initialized array depend on the data type. For numeric types, the default is `0`, for boolean it is “, and for object references, it is `null`.
In Java, initializing an array is a fundamental concept that allows developers to create and manage collections of data efficiently. There are several methods to initialize an array, including using the array initializer syntax, the `new` keyword, and the `Arrays` utility class. Each method serves different use cases and provides flexibility depending on the specific requirements of the program. Understanding these various initialization techniques is essential for effective Java programming.
One of the most common methods is the array initializer syntax, which allows for a concise declaration and initialization in a single line. For instance, declaring an array of integers can be done as follows: `int[] numbers = {1, 2, 3, 4};`. This method is particularly useful for small arrays where values are known at compile time. Alternatively, using the `new` keyword provides more control, especially when the size of the array is determined at runtime or when the array needs to be filled with default values.
Additionally, the `Arrays` utility class offers static methods that facilitate array manipulation, including initialization. For example, `Arrays.fill()` can be used to set all elements of an array to a specific value. This is particularly useful for larger arrays where manual initialization would be cumbersome. Overall
Author Profile
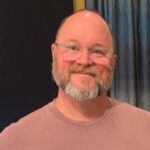
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?