How Can You Effectively Increment Values in JavaScript?
How To Increment In JavaScript: A Comprehensive Guide
In the world of programming, the ability to manipulate numbers is fundamental, and JavaScript offers a plethora of ways to achieve this. Whether you’re building interactive web applications, developing games, or simply automating tasks, understanding how to increment values is a crucial skill that can enhance your coding efficiency and effectiveness. Incrementing is not just about adding one to a number; it encompasses a range of techniques that can be applied in various contexts, from loops to event handling.
At its core, incrementing in JavaScript involves modifying a variable’s value, typically by increasing it by a specified amount. This seemingly simple operation can serve as the backbone for more complex algorithms and functionalities, such as counting iterations in a loop, adjusting scores in a game, or dynamically updating user interfaces. JavaScript provides several methods to perform increments, each with its own use cases and nuances. Understanding these methods will empower you to write cleaner, more effective code.
As we delve deeper into this topic, we will explore the various ways to increment values in JavaScript, examining both the traditional and modern approaches. From the classic increment operator to more advanced techniques involving functions and objects, this guide will equip you with the knowledge you need to master this essential programming concept
Using the Increment Operator
In JavaScript, the increment operator `++` is a convenient shorthand for increasing a numeric value by one. This operator can be used in two forms: prefix and postfix.
- Prefix Increment: When `++` is placed before the variable (e.g., `++x`), the variable is incremented first, and then the new value is returned.
- Postfix Increment: When `++` is placed after the variable (e.g., `x++`), the current value is returned first, and then the variable is incremented.
The following example illustrates the difference between the two:
“`javascript
let x = 5;
let y = ++x; // y is 6, x is 6
let z = x++; // z is 6, x is 7
“`
In this example, `y` gets the incremented value immediately, while `z` receives the value before the increment.
Incrementing in Loops
Incrementing is a common operation in loops, particularly when iterating over arrays or performing repetitive tasks. The most common loop structures include `for`, `while`, and `do…while`.
In a `for` loop, incrementing is typically done in the loop’s initialization:
“`javascript
for (let i = 0; i < 10; i++) {
console.log(i); // Outputs numbers 0 to 9
}
```
In a `while` loop, you may need to increment the counter variable inside the loop body:
```javascript
let i = 0;
while (i < 10) {
console.log(i); // Outputs numbers 0 to 9
i++;
}
```
Incrementing Values in Arrays
When working with arrays, incrementing can be utilized to manipulate elements directly. Below is an example of incrementing each element in an array by one:
“`javascript
let numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
numbers[i]++;
}
console.log(numbers); // Outputs: [2, 3, 4, 5, 6]
```
Table of Incrementing Methods
The following table summarizes various methods for incrementing values in JavaScript:
Method | Description | Example |
---|---|---|
Prefix Increment | Increments the value before returning it. | let a = 5; let b = ++a; // a is 6, b is 6 |
Postfix Increment | Returns the value before incrementing it. | let a = 5; let b = a++; // a is 6, b is 5 |
Loop Increment | Commonly used in loop structures. | for (let i = 0; i < 5; i++) { /* loop */ } |
Array Element Increment | Increments each element in an array. | let arr = [1, 2, 3]; arr[i]++; |
Using these methods, developers can efficiently manipulate numerical values in various programming contexts, enhancing code readability and performance.
Incrementing Numbers in JavaScript
In JavaScript, incrementing a number can be achieved using various methods. The most common operators for incrementing a value are the increment operator (`++`) and the addition assignment operator (`+=`).
Using the Increment Operator
The increment operator can be used in two forms: prefix and postfix.
- Prefix Increment (`++variable`): Increments the variable’s value by one and returns the new value.
- Postfix Increment (`variable++`): Increments the variable’s value by one but returns the original value before the increment.
Example:
“`javascript
let a = 5;
let b = ++a; // b is 6, a is 6
let c = a++; // c is 6, a is 7
“`
Using the Addition Assignment Operator
The addition assignment operator is another effective way to increment a variable’s value. It explicitly adds a value (usually one) to the variable.
Example:
“`javascript
let x = 10;
x += 1; // x is now 11
“`
Incrementing in Loops
Incrementing is often used in loops to iterate through a set of values. The `for` loop is one of the most common structures where incrementing is utilized.
Example of a `for` loop:
“`javascript
for (let i = 0; i < 5; i++) {
console.log(i); // Outputs 0 to 4
}
```
Incrementing Objects and Arrays
When working with objects and arrays, incrementing can also be applied to properties or indices.
- Incrementing Array Elements:
“`javascript
let numbers = [1, 2, 3];
for (let i = 0; i < numbers.length; i++) {
numbers[i] += 1; // Each element is incremented by 1
}
```
- Incrementing Object Properties:
“`javascript
let count = { value: 0 };
count.value++; // count.value is now 1
“`
Best Practices for Incrementing
When incrementing values in JavaScript, consider the following best practices:
- Use Clear Syntax: Choose between prefix and postfix increment based on the desired output and clarity of code.
- Avoid Mutating State in Loops: When modifying values in loops, ensure that the mutations do not lead to unintended side effects.
- Maintain Readability: Use explicit addition (`+=`) for clarity, especially in complex expressions.
Common Use Cases
Incrementing is widely used in various scenarios, including:
- Counting iterations in loops.
- Managing scores or counters in applications.
- Updating indices in data structures.
By understanding and utilizing these incrementing techniques, developers can effectively manage numeric values and control flow in their JavaScript applications.
Expert Insights on Incrementing in JavaScript
Julia Thompson (Senior JavaScript Developer, Tech Innovations Inc.). “Using the increment operator (++) in JavaScript is a fundamental technique that can streamline your code. It is essential to understand the difference between pre-increment and post-increment to avoid unexpected results in your applications.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “When incrementing values in JavaScript, it is crucial to consider the context of your operations. Utilizing the += operator can improve readability and maintainability, especially when dealing with complex calculations or loops.”
Sarah Patel (JavaScript Educator, Code Academy). “Incrementing in JavaScript is not just about adding one to a number. It is an opportunity to teach best practices, such as immutability and functional programming principles, which can lead to more robust and error-free code.”
Frequently Asked Questions (FAQs)
How do I increment a variable in JavaScript?
You can increment a variable in JavaScript using the increment operator `++`. For example, if you have a variable `let count = 0;`, you can increment it by using `count++;` or `count += 1;`.
What is the difference between pre-increment and post-increment in JavaScript?
Pre-increment (`++variable`) increases the variable’s value before it is used in an expression, while post-increment (`variable++`) increases the variable’s value after its current value is used in the expression.
Can I increment a variable by a specific number in JavaScript?
Yes, you can increment a variable by a specific number using the addition assignment operator `+=`. For example, `count += 5;` will increase the value of `count` by 5.
What happens if I increment a variable that is not a number?
If you increment a variable that is not a number, JavaScript attempts to convert it to a number. For instance, incrementing a string like `let str = “5”; str++;` will convert it to a number and result in `6`.
Is it possible to increment an array element in JavaScript?
Yes, you can increment an element in an array by accessing it through its index and using the increment operator. For example, `array[0]++;` will increment the first element of the array.
Can I use the increment operator in a loop in JavaScript?
Yes, the increment operator is commonly used in loops, such as `for` loops, to control the iteration. For example, `for (let i = 0; i < 10; i++) { /* loop body */ }` increments `i` with each iteration.
incrementing values in JavaScript is a fundamental operation that can be performed using various methods. The most common approach is utilizing the increment operator, which can be either the prefix (++) or postfix (++) form. Understanding the difference between these two forms is crucial, as they can lead to different outcomes when used in expressions. Additionally, incrementing can also be achieved through traditional arithmetic operations, such as using the addition assignment operator (+=), which provides flexibility in modifying variable values.
Another important aspect to consider is the context in which incrementing occurs. When working with arrays or objects, developers often need to increment indices or properties. This requires a solid grasp of JavaScript's data structures and the implications of incrementing values within loops or conditional statements. Furthermore, maintaining code readability and preventing side effects is essential when incrementing values, particularly in complex applications.
Ultimately, mastering the increment operation in JavaScript is vital for effective programming. It not only enhances the efficiency of code but also contributes to clearer logic and better performance. As developers continue to explore JavaScript's capabilities, understanding how to properly increment values will remain a key skill in their toolkit.
Author Profile
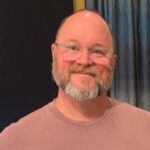
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?