How Can You Increment an Enum Variable in C?
Enums, or enumerations, are a powerful feature in C programming that allow developers to define a variable that can hold a set of predefined constants. This not only enhances code readability but also minimizes the risk of errors associated with using arbitrary numbers. However, when it comes to manipulating these enum variables—specifically, incrementing their values—many programmers find themselves at a crossroads. How do you effectively manage and utilize enum variables to streamline your code? In this article, we will delve into the nuances of incrementing enum variables in C, unraveling the intricacies of their underlying mechanics and providing practical insights to elevate your coding skills.
Understanding how to increment enum variables is essential for any C programmer looking to harness the full potential of this feature. Enums are typically used to represent a collection of related constants, and incrementing these values can facilitate smoother control flow in your applications. Whether you are developing a simple game or a complex application, knowing how to manipulate these constants can lead to cleaner, more efficient code.
As we explore this topic, we will cover the fundamental principles of enums in C, how they can be incremented, and the best practices for implementing this functionality in your projects. By the end of this article, you will not only grasp the technical aspects
Understanding Enum Variables in C
In C, an enumeration (enum) is a user-defined data type consisting of integral constants. An enum variable can be incremented in a way similar to other integral types. Understanding how to manipulate these variables is essential for effectively utilizing enums in programming.
Declaring an Enum
To use an enum in C, you need to declare it first. The syntax is straightforward:
“`c
enum Color { RED, GREEN, BLUE };
“`
In this example, `RED` is assigned the value `0`, `GREEN` the value `1`, and `BLUE` the value `2` by default. You can also assign specific values manually:
“`c
enum Color { RED = 1, GREEN = 2, BLUE = 4 };
“`
Incrementing Enum Variables
Incrementing an enum variable is akin to incrementing an integer variable, as enums are essentially integer values. You can increment an enum variable using the increment operator `++`. Here’s a simple example:
“`c
enum Color { RED, GREEN, BLUE };
enum Color myColor = RED;
myColor++; // myColor is now GREEN
“`
This operation will automatically advance the variable to the next defined value in the enum sequence. If you reach the last value and try to increment again, the variable will wrap around to the first value.
Example of Enum Incrementing
Here’s an example that illustrates incrementing an enum variable:
“`c
include
enum Days { MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY };
int main() {
enum Days today = MONDAY;
printf(“Today is: %d\n”, today); // Output: Today is: 0
today++; // Incrementing to the next day
printf(“Tomorrow is: %d\n”, today); // Output: Tomorrow is: 1
return 0;
}
“`
In this code, the enum `Days` is defined with days of the week. The variable `today` starts at `MONDAY` and is incremented to `TUESDAY`.
Handling Enum Values Beyond Defined Limits
When incrementing enum variables, it’s crucial to manage values that exceed the defined enumeration range. Here is how you can handle it:
- Wrap Around: If you increment an enum variable beyond its maximum defined value, it will wrap to the beginning.
- Validation: Implement checks to ensure that operations do not lead to unintended behavior.
Here’s a simple validation mechanism:
“`c
if (today < SUNDAY) {
today++;
} else {
today = MONDAY; // Wrap around to MONDAY
}
```
Table of Enum Values
To give a better understanding of enum values and their increments, consider the following table:
Enum Name | Value |
---|---|
MONDAY | 0 |
TUESDAY | 1 |
WEDNESDAY | 2 |
THURSDAY | 3 |
FRIDAY | 4 |
SATURDAY | 5 |
SUNDAY | 6 |
This table presents the values corresponding to each day of the week, illustrating how incrementing an enum variable progresses through these values.
Understanding Enums in C
Enums, short for enumerations, are a user-defined data type in C that consists of integral constants. They provide a way to assign meaningful names to a set of related values, enhancing code readability and maintainability. An enum can be defined using the `enum` keyword followed by a name and a list of enumerators.
Example of defining an enum:
“`c
enum Color {
RED,
GREEN,
BLUE
};
“`
In this example, `RED` is assigned the value `0`, `GREEN` is `1`, and `BLUE` is `2` by default.
Incrementing an Enum Variable
Incrementing an enum variable in C is straightforward, as enums are essentially integers. However, it is essential to ensure that the incremented value remains within the defined range of the enum.
Example of Incrementing an Enum
“`c
include
enum Color {
RED,
GREEN,
BLUE,
COLOR_COUNT // This can be useful for looping
};
int main() {
enum Color myColor = RED;
// Incrementing the enum variable
myColor++;
// Check if the incremented value is valid
if (myColor < COLOR_COUNT) {
printf("The color is now: %d\n", myColor); // Outputs: 1 (GREEN)
} else {
printf("Invalid color increment\n");
}
return 0;
}
```
Important Considerations
- Bounds Checking: Always ensure that the incremented value does not exceed the defined range of the enum. This can be managed through conditional checks, as shown in the example.
- Wraparound Behavior: If you increment an enum variable beyond its maximum value, it will continue to increment, potentially leading to unexpected values. To prevent this, implement logic to wrap the value back to a valid state.
Alternative Incrementing Methods
Instead of direct incrementing, use a function to handle the increment operation, allowing for more complex logic:
“`c
enum Color incrementColor(enum Color color) {
if (color < COLOR_COUNT - 1) {
return color + 1;
} else {
return 0; // Wrap around to RED
}
}
```
Practical Use Cases
- State Machines: Enums are often used in state machines to represent the current state clearly.
- Switch Statements: They can be beneficial in switch-case constructs for better readability.
“`c
switch (myColor) {
case RED:
// Handle red case
break;
case GREEN:
// Handle green case
break;
case BLUE:
// Handle blue case
break;
default:
// Handle unexpected cases
break;
}
“`
By following these guidelines, you can effectively manage and increment enum variables in C, ensuring your code remains clear and robust.
Expert Insights on Incrementing Enum Variables in C
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When incrementing an enum variable in C, it is crucial to ensure that the enum values are defined in a contiguous manner. This allows for straightforward arithmetic operations, such as incrementing the enum variable using the standard increment operator.”
Michael Chen (C Programming Specialist, CodeMaster Academy). “Using enums can enhance code readability, but developers must be cautious when incrementing enum values. It is advisable to cast the enum to an integer type before performing arithmetic operations to avoid unexpected behavior, especially when the enum is not defined as a sequential series.”
Sarah Thompson (Lead Developer, Open Source Projects). “In C, while you can increment an enum variable directly, it is best practice to create a function that encapsulates this logic. This approach not only improves code maintainability but also allows for error handling, ensuring that the incremented value remains within the defined range of the enum.”
Frequently Asked Questions (FAQs)
What is an enum in C?
An enum, or enumeration, in C is a user-defined data type that consists of integral constants. It allows for the creation of named integer constants, improving code readability and maintainability.
How do you define an enum in C?
An enum is defined using the `enum` keyword followed by a name and a list of identifiers. For example:
“`c
enum Color { RED, GREEN, BLUE };
“`
Can you increment an enum variable in C?
Yes, you can increment an enum variable in C as it is essentially an integer type. For example:
“`c
enum Color { RED, GREEN, BLUE };
enum Color color = RED;
color++; // Now color will be GREEN
“`
What happens if you increment an enum variable beyond its defined range?
If you increment an enum variable beyond its defined range, it will wrap around to the beginning of the enumeration. For instance, incrementing `BLUE` in the previous example would result in `color` being set to `0`, which corresponds to `RED`.
Is it possible to assign non-sequential values to enum constants?
Yes, enum constants can be assigned non-sequential values. For example:
“`c
enum Status { SUCCESS = 1, FAILURE = 5, PENDING = 10 };
“`
What are the best practices for using enums in C?
Best practices include using meaningful names for enum constants, keeping enumerations grouped logically, and avoiding implicit integer values to enhance code clarity and prevent potential errors.
In C programming, enumerations, or enums, provide a way to define a variable that can hold a set of predefined constants. Incrementing an enum variable is not as straightforward as incrementing a standard integer variable, but it can be accomplished by casting the enum to an integer type, performing the increment, and then casting it back to the enum type. This method allows developers to leverage the benefits of enums while still being able to manipulate their values programmatically.
One important consideration when incrementing an enum variable is to ensure that the incremented value does not exceed the defined range of the enum. This can lead to behavior if the value goes beyond the maximum defined constant. Therefore, it is crucial to implement checks to prevent such scenarios, maintaining the integrity of the enum’s intended use.
Additionally, using enums can enhance code readability and maintainability. They provide meaningful names for sets of related constants, making the code more understandable. When incrementing enum variables, developers should be mindful of the underlying integer values to avoid confusion and ensure that the logic remains clear. Overall, incrementing enum variables in C can be effectively managed with careful coding practices and a solid understanding of enum behavior.
Author Profile
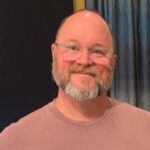
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?