How Can You Import Python Files From Another Directory Easily?
In the world of Python programming, organizing your code efficiently is crucial for maintaining readability and scalability. As projects grow in complexity, developers often find themselves needing to manage multiple files spread across various directories. This can lead to challenges when it comes to importing modules and functions from one file to another. If you’ve ever grappled with `ImportError` messages or spent too much time adjusting your file structure, you’re not alone. Understanding how to import Python files from another directory can streamline your workflow and enhance your coding experience.
Navigating Python’s import system is essential for any developer looking to harness the full potential of the language. By mastering the art of importing files from different directories, you can create modular code that promotes reusability and collaboration. This knowledge not only helps in keeping your projects organized but also allows for easier debugging and testing. Whether you’re working on a small script or a large application, knowing how to effectively manage your imports will save you time and headaches down the line.
In this article, we will explore the various methods available for importing Python files from different directories. From adjusting your Python path to using relative imports, we’ll cover the essential techniques that will empower you to structure your projects with confidence. Get ready to dive into the intricacies of Python imports and
Understanding Python Module Search Path
When importing Python files from another directory, it is crucial to comprehend the module search path, which is a list of directories that Python scans to locate modules. This search path is stored in the `sys.path` variable. By default, this includes the directory containing the input script, the current directory, and the directories specified in the `PYTHONPATH` environment variable.
To view the current Python module search path, you can use the following code snippet:
“`python
import sys
print(sys.path)
“`
Using sys.path to Import Modules
One of the most straightforward methods to import a Python file from another directory is to modify the `sys.path` list at runtime. This allows you to append the directory containing your target module dynamically. Here’s how you can do it:
“`python
import sys
sys.path.append(‘/path/to/your/directory’)
import your_module
“`
This approach is particularly useful for scripts that need to access modules located in various directories without altering the environment permanently.
Using __init__.py for Package Management
If you are organizing your Python files into packages, ensure that each directory contains an `__init__.py` file. This file can be empty or contain initialization code for the package. The presence of `__init__.py` allows Python to recognize the directory as a package, facilitating easier imports.
For example, consider the following directory structure:
“`
/project
/package
__init__.py
module_a.py
main.py
“`
In `main.py`, you can import `module_a` like this:
“`python
from package import module_a
“`
Relative Imports
Relative imports are also an option when working with packages. They allow you to import modules relative to the current module’s location. This is particularly useful in large projects. Here’s how you can implement a relative import:
“`python
from .module_a import function_name
“`
This syntax indicates that `module_a` is in the same package as the current module.
Table of Import Methods
Method | Description | Example |
---|---|---|
sys.path | Modify the module search path at runtime. | sys.path.append(‘/path/to/dir’) |
__init__.py | Define a directory as a package. | from package import module |
Relative Imports | Import modules relative to the current module. | from .module import function |
Using Virtual Environments
In scenarios where managing dependencies and paths becomes complex, utilizing virtual environments can simplify the process. Virtual environments allow you to create isolated spaces for your projects, ensuring that dependencies and package versions do not interfere with one another. You can create a virtual environment using the following command:
“`bash
python -m venv myenv
“`
Activate the virtual environment and install necessary packages within it. This keeps your project organized and ensures that all imports function as expected without conflicts.
Understanding Python’s Module System
Python uses a module system that allows developers to organize code into reusable components. Each Python file is a module that can be imported into other files. Understanding how this system works is crucial for effectively importing files from different directories.
- Modules: Any Python file with a `.py` extension.
- Packages: A directory that contains an `__init__.py` file, allowing it to be treated as a package.
Using sys.path to Import from Another Directory
One of the simplest ways to import a Python file from another directory is by modifying the `sys.path`. This is a list of directories that Python searches for modules.
“`python
import sys
sys.path.append(‘/path/to/your/directory’)
import your_module
“`
- Advantages:
- Quick to implement.
- No need to restructure your project.
- Disadvantages:
- Can lead to confusion if the module is imported multiple times.
- Risk of name clashes with modules in the standard library.
Creating a Package Structure
Organizing Python files into a package structure is a more sustainable method for managing imports. To create a package, follow these steps:
- Directory Structure:
“`
my_project/
├── main.py
└── my_package/
├── __init__.py
└── my_module.py
“`
- Importing:
In `main.py`, you can import `my_module` using:
“`python
from my_package import my_module
“`
- Benefits:
- Promotes better organization of code.
- Avoids potential conflicts in module names.
Relative Imports
When working within a package, you can use relative imports to access modules in sibling directories. This is achieved using the dot notation.
- Example Structure:
“`
my_project/
├── main.py
└── my_package/
├── __init__.py
├── module_a.py
└── module_b.py
“`
- Importing in module_b.py:
“`python
from .module_a import some_function
“`
- Caveats:
- Relative imports only work within packages.
- Can lead to confusion if not well-documented.
Using the PYTHONPATH Environment Variable
Another approach to import files from a different directory is to set the `PYTHONPATH` environment variable. This variable allows you to specify additional directories for Python to search for modules.
- Setting PYTHONPATH:
- On Unix/Linux:
“`bash
export PYTHONPATH=”/path/to/your/directory:$PYTHONPATH”
“`
- On Windows:
“`cmd
set PYTHONPATH=”C:\path\to\your\directory;%PYTHONPATH%”
“`
- Usage:
After setting the `PYTHONPATH`, you can import modules as if they were in the current directory:
“`python
import your_module
“`
Considerations for Best Practices
When importing Python files from another directory, consider the following best practices:
- Keep your directory structure clean: Avoid deep nesting of directories.
- Use absolute imports: They are more readable and less error-prone than relative imports.
- Document your imports: Clearly comment on any non-standard imports to aid future maintenance.
- Testing: Ensure that your imports work correctly across different environments and setups.
By adhering to these practices, you can maintain a robust and maintainable codebase while effectively managing module imports across directories.
Expert Insights on Importing Python Files from Different Directories
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “To effectively import Python files from another directory, it is crucial to understand the Python module search path. Utilizing the `sys.path.append()` method allows you to dynamically add the target directory to the list of directories Python checks for modules, ensuring seamless access to your files.”
Michael Chen (Python Developer, Tech Innovations Inc.). “When organizing your Python projects, it is essential to maintain a clear directory structure. Using relative imports within packages can simplify the process of importing files from sibling directories, but one must be cautious with the `__init__.py` files to ensure proper package recognition.”
Sarah Patel (Lead Data Scientist, Data Insights Group). “In data science projects, managing dependencies across directories can be challenging. I recommend using virtual environments in conjunction with the `PYTHONPATH` environment variable to streamline the import process and avoid conflicts with system-wide packages.”
Frequently Asked Questions (FAQs)
How can I import a Python file from a different directory?
To import a Python file from another directory, you can modify the `sys.path` list to include the directory containing the file. Use the following code snippet:
“`python
import sys
sys.path.append(‘/path/to/your/directory’)
import your_module
“`
What is the purpose of `sys.path`?
`sys.path` is a list in Python that contains the directories Python searches for modules when an import statement is executed. By adding a directory to `sys.path`, you can make Python aware of additional locations to find modules.
Can I use relative imports to access files in other directories?
Relative imports can be used within a package structure. Use a dot notation to indicate the relative path, such as `from ..module import function`, but this only works if the script is executed as part of a package.
What is the `PYTHONPATH` environment variable?
`PYTHONPATH` is an environment variable that you can set to specify additional directories for Python to search for modules. You can set it in your operating system to include the paths to your custom modules.
Are there any limitations to importing files from other directories?
Yes, limitations include potential conflicts with module names, difficulties in maintaining code organization, and issues with circular imports. It’s advisable to follow a structured project layout to mitigate these issues.
How can I avoid namespace conflicts when importing modules?
To avoid namespace conflicts, consider using aliasing during the import, such as `import your_module as ym`. This allows you to reference the module with a unique name, reducing the risk of conflicts with other modules.
In summary, importing Python files from another directory is a common requirement for developers looking to organize their code effectively. The primary methods for achieving this include modifying the Python path, using relative imports, and utilizing the `sys` module to append the target directory to the system path. Each of these methods has its own use cases and advantages, depending on the project’s structure and the specific requirements of the import operation.
It is crucial to understand the implications of each method. For instance, modifying the Python path can lead to potential conflicts if not managed properly, while relative imports can simplify code organization within packages. Additionally, using the `sys` module provides a flexible approach, allowing developers to dynamically adjust the import paths at runtime. Understanding these methods enables developers to maintain clean, modular, and reusable code across different directories.
Ultimately, the choice of method should be guided by the project’s architecture and the need for maintainability. By carefully considering the structure of your directories and the relationships between your modules, you can effectively manage imports and enhance the overall quality of your Python projects. This strategic approach not only facilitates collaboration but also improves the scalability of your codebase.
Author Profile
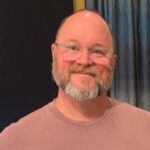
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?