How Can You Import MPD Files into Jest Test Files Effectively?
In the ever-evolving world of software development, testing frameworks play a crucial role in ensuring code quality and reliability. Jest, a popular JavaScript testing framework, is widely embraced for its simplicity and powerful features. However, as projects grow in complexity, developers often find themselves needing to integrate various file formats into their testing environments. One such format is the MPD (Media Presentation Description) file, commonly used in adaptive streaming scenarios. If you’ve ever wondered how to seamlessly import MPD files into your Jest test files, you’re in the right place! This article will guide you through the process, empowering you to enhance your testing capabilities and streamline your workflow.
When working with MPD files in Jest, understanding the structure and purpose of these files is essential. MPD files serve as a blueprint for streaming media, providing critical information about available content and its delivery. By incorporating these files into your Jest tests, you can simulate and validate media playback scenarios, ensuring that your applications handle streaming content effectively. This integration not only enhances the robustness of your tests but also helps you identify potential issues early in the development cycle.
As we delve deeper into this topic, we’ll explore the practical steps required to import MPD files into your Jest test files. From setting up your testing environment to
Understanding MPD Files
MPD files, or Media Presentation Description files, are essential in adaptive streaming technologies such as MPEG-DASH. They contain metadata about the media content, including information about available bitrates, codecs, and segment URLs. In the context of testing with Jest, importing MPD files allows developers to simulate various media scenarios and ensure that their applications can handle adaptive streaming correctly.
Setting Up Jest for MPD Files
To import MPD files into Jest test files, you must ensure your testing environment is configured correctly. This involves setting up Jest to handle non-JavaScript files, such as MPD files, using module transformers or mock files.
- Install necessary dependencies:
“`bash
npm install –save-dev jest
“`
- Create a mock file transformer for MPD files. This can be done by adding a transformer to your Jest configuration, allowing Jest to understand how to handle these files during tests.
Configuring Jest to Handle MPD Files
You can configure Jest to handle MPD files by updating your `jest.config.js` file. Below is an example configuration:
“`javascript
module.exports = {
moduleNameMapper: {
‘\\.(mpd)$’: ‘
},
};
“`
In this configuration, any import of an MPD file will be redirected to a mock file (`fileMock.js`), which can simply return an empty object or a string representation of an MPD file.
Creating a Mock File
Next, create the mock file in the `__mocks__` directory. This file will provide a simple response when an MPD file is imported in your tests.
Example of `fileMock.js`:
“`javascript
module.exports = ‘mocked-mpd-file’;
“`
This allows you to simulate the presence of MPD files without needing the actual files during testing.
Importing MPD Files in Tests
Once your Jest configuration is set up, you can import MPD files directly into your test files. Here’s an example of how to do this:
“`javascript
import mpdFile from ‘./path/to/your.mpd’;
test(‘should handle MPD file correctly’, () => {
expect(mpdFile).toBe(‘mocked-mpd-file’);
});
“`
In this example, the test checks that the imported MPD file returns the expected mocked value. This ensures that your application can correctly handle the MPD data structure.
Best Practices for Testing with MPD Files
When working with MPD files in Jest, consider the following best practices:
- Use Mock Data: Always use mock MPD data to avoid dependencies on external files during tests.
- Test Edge Cases: Simulate various scenarios, such as missing segments or different codecs, to ensure your application can handle unexpected situations.
- Keep Tests Isolated: Ensure that each test is independent and does not rely on the state of other tests.
Best Practice | Description |
---|---|
Use Mock Data | Avoid using actual MPD files to ensure tests are fast and reliable. |
Test Edge Cases | Handle various scenarios in tests to improve robustness. |
Keep Tests Isolated | Ensure tests do not affect each other, improving reliability. |
Understanding MPD Files
MPD (Media Presentation Description) files are XML files used primarily in adaptive streaming protocols like MPEG-DASH. These files describe the media segments, their codecs, and other related metadata necessary for streaming applications. To import MPD files into Jest test files, it’s important to understand their structure and how they can be integrated into JavaScript testing environments.
Setting Up Your Environment
Before importing MPD files into Jest tests, ensure that your project has the necessary dependencies. You will need:
- Node.js: Install the latest version of Node.js.
- Jest: Ensure Jest is installed in your project. You can install it using npm:
“`bash
npm install –save-dev jest
“`
- XML Parser: Since MPD files are XML, you’ll need a library to parse XML in JavaScript. A popular choice is `xml2js`:
“`bash
npm install xml2js
“`
Importing MPD Files
To import MPD files into Jest test files, follow these steps:
- **Create a Test File**: Create a new test file in your project, e.g., `mpd.test.js`.
- **Read the MPD File**: Use Node.js’s `fs` module to read the MPD file. The code snippet below demonstrates how to read and parse the file:
“`javascript
const fs = require(‘fs’);
const xml2js = require(‘xml2js’);
const mpdFilePath = ‘path/to/your/file.mpd’; // Update this path
fs.readFile(mpdFilePath, ‘utf8’, (err, data) => {
if (err) throw err;
xml2js.parseString(data, (err, result) => {
if (err) throw err;
// Access the parsed MPD data
console.log(result);
});
});
“`
- **Writing Tests**: Once you have the MPD data parsed, you can write Jest tests to validate the structure or content of the MPD file. For example:
“`javascript
test(‘MPD file should contain required elements’, (done) => {
fs.readFile(mpdFilePath, ‘utf8’, (err, data) => {
if (err) return done(err);
xml2js.parseString(data, (err, result) => {
if (err) return done(err);
expect(result).toHaveProperty(‘MPD’);
expect(result.MPD).toHaveProperty(‘Period’);
done();
});
});
});
“`
Best Practices
When working with MPD files in Jest tests, consider the following best practices:
- Use Mock Data: Instead of relying on actual MPD files, create mock MPD files for testing purposes. This approach ensures that tests are independent and can run in isolation.
- Error Handling: Implement robust error handling to manage potential issues when reading or parsing MPD files.
- Keep Tests Fast: Limit the size of the MPD files used in tests to keep the test suite running quickly.
Sample MPD Structure
Understanding the MPD file structure can be beneficial when writing tests. Below is a simplified representation of an MPD file:
“`xml
“`
By adhering to these guidelines, importing MPD files into Jest test files can be streamlined, ensuring effective testing of media streaming applications.
Expert Insights on Importing MPD Files into Jest Test Files
Dr. Emily Carter (Software Engineer, Test Automation Specialist). “To effectively import MPD files into Jest test files, it is crucial to ensure that the file structure aligns with Jest’s module resolution. Utilizing the correct import syntax and ensuring that the MPD files are properly formatted will facilitate seamless integration into your testing framework.”
Michael Chen (Senior Developer, JavaScript Testing Advocate). “One common approach to importing MPD files is to leverage Jest’s built-in module mocking capabilities. By creating a mock module for the MPD files, developers can simulate their behavior in tests, allowing for more controlled and predictable testing scenarios.”
Sarah Thompson (Lead QA Engineer, Agile Testing Group). “When importing MPD files into Jest, it is essential to consider the dependencies and how they interact with the Jest environment. Utilizing tools like Babel can help transpile the MPD files, making them compatible with Jest’s testing ecosystem and ensuring that all features are properly tested.”
Frequently Asked Questions (FAQs)
What are MPD files and how are they used in testing?
MPD files, or Model Project Descriptor files, are typically used to define and manage configurations for testing environments. They provide structured information about the test setup, dependencies, and execution parameters.
Can Jest directly import MPD files?
Jest does not natively support importing MPD files. You will need to convert or adapt the contents of the MPD file into a format that Jest can understand, such as JavaScript or JSON.
What is the process to convert MPD files for Jest?
To convert MPD files for Jest, you can create a script that reads the MPD file, extracts the necessary configurations, and outputs them as a JavaScript object or JSON file that Jest can import.
Are there any specific libraries to help with MPD file conversion?
While there are no dedicated libraries specifically for MPD file conversion, general-purpose XML or JSON parsing libraries can be used to read and transform MPD files into a format compatible with Jest.
How can I test the imported configurations in Jest?
After importing the converted configurations into your Jest test files, you can write test cases that utilize these configurations, ensuring that the expected behavior aligns with the defined parameters.
What should I do if I encounter issues while importing MPD files?
If you encounter issues, check for syntax errors in your converted files, ensure that the Jest configuration is correctly set up, and verify that all dependencies are properly installed and accessible within your testing environment.
Importing MPD files into Jest test files is a process that requires a clear understanding of both the MPD file format and the Jest testing framework. MPD files, commonly associated with MPEG-DASH, contain metadata for media streaming, and integrating them into Jest tests can enhance the testing of media-related functionalities. The approach typically involves using appropriate libraries to parse MPD files and then utilizing Jest’s capabilities to assert expected outcomes based on the parsed data.
Key insights from this discussion highlight the importance of setting up the correct environment for testing. This includes ensuring that all necessary dependencies are installed and configured properly. Additionally, leveraging Jest’s mocking features can significantly streamline the testing process by simulating the behavior of MPD files without needing to rely on actual file access during tests.
Moreover, understanding how to structure your Jest tests to accommodate the specific data and scenarios represented in MPD files is crucial. By doing so, developers can create more robust tests that accurately reflect the functionality of their applications. This not only improves code quality but also enhances maintainability and the overall development workflow.
Author Profile
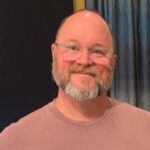
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?