How Can You Import Functions From Another JavaScript File?
### Introduction
In the world of JavaScript development, modularity is key to creating maintainable and scalable applications. As projects grow in complexity, the ability to organize code into separate files becomes essential. One of the most powerful features of JavaScript is its ability to import and export functions between different files, allowing developers to reuse code and keep their projects tidy. Whether you’re working on a small script or a large web application, understanding how to effectively import functions from another JavaScript file can significantly enhance your coding efficiency and collaboration with others.
As you delve into the intricacies of JavaScript modules, you’ll discover that importing functions not only streamlines your code but also promotes a cleaner architecture. By leveraging the `import` and `export` syntax, you can easily share functionality across various parts of your application. This practice not only reduces redundancy but also makes your codebase easier to navigate and understand.
In this article, we’ll explore the various methods of importing functions from one JavaScript file to another, highlighting best practices and common pitfalls to avoid. Whether you’re using ES6 modules or CommonJS, you’ll gain insights into how to structure your files for optimal performance and clarity. Get ready to unlock the full potential of modular programming in JavaScript!
Using ES6 Modules
To import functions from another JavaScript file using ES6 modules, you must first ensure that your JavaScript files are organized correctly. ES6 modules utilize the `export` and `import` keywords to facilitate the sharing of functions, objects, or variables between files.
To export a function from a JavaScript file, you can use either named exports or default exports:
- Named Exports: Allows you to export multiple functions or variables from a single file.
- Default Exports: Allows you to export a single function, object, or variable as the default export for a module.
Here’s an example illustrating both methods.
Example of Named Exports:
In `math.js`:
javascript
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a – b;
}
In `app.js`:
javascript
import { add, subtract } from ‘./math.js’;
console.log(add(5, 3)); // Outputs: 8
console.log(subtract(5, 3)); // Outputs: 2
Example of Default Export:
In `math.js`:
javascript
export default function multiply(a, b) {
return a * b;
}
In `app.js`:
javascript
import multiply from ‘./math.js’;
console.log(multiply(5, 3)); // Outputs: 15
Using CommonJS Modules
In environments like Node.js, CommonJS is the standard module system. It employs the `require()` function to import modules and `module.exports` to export them. This method is also compatible with older JavaScript environments.
Here’s how to implement CommonJS modules:
Example of Exporting and Importing:
In `math.js`:
javascript
function divide(a, b) {
return a / b;
}
module.exports = divide;
In `app.js`:
javascript
const divide = require(‘./math.js’);
console.log(divide(10, 2)); // Outputs: 5
Using CommonJS with Multiple Exports:
In `math.js`:
javascript
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a – b;
}
module.exports = {
add,
subtract
};
In `app.js`:
javascript
const math = require(‘./math.js’);
console.log(math.add(5, 3)); // Outputs: 8
console.log(math.subtract(5, 3)); // Outputs: 2
Choosing Between ES6 and CommonJS
When deciding between ES6 modules and CommonJS, consider the following factors:
Feature | ES6 Modules | CommonJS |
---|---|---|
Syntax | import/export | require/module.exports |
Asynchronous Loading | No | |
Browser Compatibility | Modern browsers | Node.js and older browsers |
Default Exports | Yes | No |
By understanding how to import functions from another JavaScript file using either ES6 modules or CommonJS, you can effectively structure your codebase for better maintainability and reusability.
Using ES6 Modules
To import functions from another JavaScript file, the ES6 module syntax is the most modern and widely adopted method. This approach allows you to organize your code into separate files for better maintainability and clarity.
Exporting Functions:
In the source file, you need to export the functions you want to make available. This can be done using the `export` keyword.
Example of exporting functions:
javascript
// mathFunctions.js
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a – b;
}
You can also use a default export if you want to export a single function or value:
javascript
// mathFunctions.js
export default function multiply(a, b) {
return a * b;
}
Importing Functions:
To use the exported functions in another file, you will use the `import` statement. The syntax varies depending on whether you are importing named exports or a default export.
Example of importing named exports:
javascript
// app.js
import { add, subtract } from ‘./mathFunctions.js’;
console.log(add(5, 3)); // Outputs: 8
console.log(subtract(5, 3)); // Outputs: 2
Example of importing a default export:
javascript
// app.js
import multiply from ‘./mathFunctions.js’;
console.log(multiply(5, 3)); // Outputs: 15
CommonJS Modules
For environments such as Node.js, the CommonJS module syntax is prevalent. This method uses `require` for importing and `module.exports` for exporting.
Exporting Functions:
You can export functions by assigning them to `module.exports`:
javascript
// mathFunctions.js
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a – b;
}
module.exports = { add, subtract };
Importing Functions:
To import the functions in another file, you use the `require` function:
javascript
// app.js
const mathFunctions = require(‘./mathFunctions’);
console.log(mathFunctions.add(5, 3)); // Outputs: 8
console.log(mathFunctions.subtract(5, 3)); // Outputs: 2
Using TypeScript with Modules
If you are working with TypeScript, the process of importing and exporting functions is similar to ES6 modules, but with type annotations.
Exporting Functions in TypeScript:
typescript
// mathFunctions.ts
export function add(a: number, b: number): number {
return a + b;
}
Importing Functions in TypeScript:
typescript
// app.ts
import { add } from ‘./mathFunctions’;
console.log(add(5, 3)); // Outputs: 8
Module Bundlers
When using module bundlers like Webpack or Parcel, the same import/export syntax applies. However, you should ensure that your bundler is configured to handle the type of modules you are using (ES6 or CommonJS).
Configuration Example:
- For ES6, ensure your `webpack.config.js` includes the following:
javascript
module.exports = {
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: ‘babel-loader’,
},
},
],
},
};
This configuration allows you to utilize ES6 syntax across your application seamlessly.
Expert Insights on Importing Functions in JavaScript
Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). “When importing functions from another JavaScript file, it is essential to use the correct syntax. Utilizing ES6 modules with the ‘import’ statement allows for a clean and efficient way to manage dependencies across files. This practice not only enhances code organization but also improves maintainability.”
James O’Connor (Lead Software Engineer, CodeCraft Solutions). “Understanding the difference between default and named exports is crucial when importing functions. Default exports allow for a single export per file, while named exports can include multiple functions. This distinction can significantly impact how you structure your code and how you import these functions in other files.”
Linda Zhang (JavaScript Educator, Web Development Academy). “It is important to ensure that the file paths are correctly specified when importing functions. Relative paths can be tricky, especially in larger projects. Using absolute paths or configuring module resolution can help prevent common import errors and streamline the development process.”
Frequently Asked Questions (FAQs)
How do I export a function from a JavaScript file?
To export a function from a JavaScript file, use the `export` keyword before the function declaration. For example, `export function myFunction() { … }` allows the function to be imported in other files.
What is the syntax to import a function from another JavaScript file?
The syntax to import a function is `import { myFunction } from ‘./myFile.js’;`. Ensure that the path to the file is correct and includes the file extension.
Can I import multiple functions from the same file?
Yes, you can import multiple functions by listing them within curly braces, separated by commas. For example, `import { functionOne, functionTwo } from ‘./myFile.js’;`.
What is the difference between default and named exports?
Default exports allow you to export a single value or function from a file without using curly braces during import. Named exports require you to use the exact name of the exported function or variable and curly braces during import.
Do I need to use a specific module system to import functions?
Yes, you need to use a module system like ES6 modules or CommonJS. ES6 modules use `import` and `export`, while CommonJS uses `require()` and `module.exports`.
What happens if I try to import a function that does not exist?
If you attempt to import a function that does not exist, JavaScript will throw a `ReferenceError` indicating that the function is not defined. Ensure that the function name matches the exported name exactly.
In summary, importing functions from another JavaScript file is a fundamental practice that enhances code organization and modularity. By utilizing the ES6 module syntax, developers can easily export functions from one file and import them into another. This approach not only promotes reusability but also allows for better maintenance of code by separating concerns across different files.
Key takeaways include the importance of using the `export` keyword to make functions available for import and the `import` keyword to bring them into the desired scope. Developers should also be aware of the two types of exports: named exports and default exports, each serving distinct purposes in structuring code. Understanding these concepts is crucial for effectively managing dependencies and ensuring smooth collaboration in larger projects.
Moreover, leveraging module imports can significantly improve the readability and scalability of JavaScript applications. By breaking down complex functionalities into smaller, manageable modules, developers can streamline their workflow and enhance the overall quality of their code. As JavaScript continues to evolve, mastering the import/export paradigm will remain an essential skill for developers aiming to create efficient and maintainable applications.
Author Profile
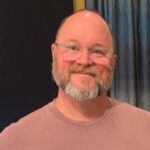
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?