How Can You Easily Import an Excel File into Python?
In today’s data-driven world, the ability to manipulate and analyze data efficiently is a vital skill for professionals across various fields. Excel files are ubiquitous, serving as a primary format for data storage and sharing. However, to unlock the full potential of this data, especially in the realm of data analysis and automation, one must learn how to seamlessly import Excel files into Python. This powerful programming language, known for its versatility and robust libraries, offers a myriad of tools that can transform raw data into actionable insights.
Importing Excel files into Python opens the door to a wealth of possibilities, whether you’re looking to perform complex data analyses, automate repetitive tasks, or visualize trends. With libraries like Pandas and openpyxl at your disposal, the process becomes not only straightforward but also efficient, allowing you to handle large datasets with ease. As we delve deeper into this topic, you’ll discover the various methods and best practices for importing Excel files, along with tips to streamline your workflow and enhance your data manipulation capabilities.
By mastering the art of importing Excel files into Python, you position yourself to harness the power of programming for data analysis. Whether you are a data scientist, analyst, or simply someone looking to enhance your skill set, understanding how to bridge the gap between Excel and
Using Pandas to Read Excel Files
Pandas is one of the most popular libraries for data manipulation and analysis in Python. It provides a straightforward method to read Excel files using the `read_excel()` function. This function supports both `.xls` and `.xlsx` formats.
To get started, ensure that you have Pandas and openpyxl installed. If not, you can install them using pip:
“`bash
pip install pandas openpyxl
“`
Once you have the necessary packages, you can use the following code snippet to read an Excel file:
“`python
import pandas as pd
Read an Excel file
df = pd.read_excel(‘path_to_file.xlsx’, sheet_name=’Sheet1′)
“`
In this example, replace `’path_to_file.xlsx’` with the actual path to your Excel file and `’Sheet1’` with the name of the sheet you want to import. If you omit the `sheet_name` parameter, Pandas will read the first sheet by default.
Understanding the Parameters of read_excel
The `read_excel()` function comes with several parameters that allow you to customize how the Excel file is read. Here are some key parameters:
- `io`: The path to the Excel file.
- `sheet_name`: The name or index of the sheet to read.
- `header`: Row number(s) to use as the column names. Default is 0 (the first row).
- `index_col`: Column(s) to set as the index. Default is None.
- `usecols`: Specifies which columns to read. It can be a range (e.g., ‘A:C’) or a list of column names.
- `dtype`: Data type for data or columns.
For instance, if you want to read specific columns and set a particular column as the index, you can do so as follows:
“`python
df = pd.read_excel(‘path_to_file.xlsx’, sheet_name=’Sheet1′, usecols=’A:C’, index_col=0)
“`
Example: Importing an Excel File
Here’s a practical example demonstrating how to import an Excel file with specific configurations:
“`python
import pandas as pd
Define the file path
file_path = ‘sales_data.xlsx’
Read the Excel file
sales_df = pd.read_excel(file_path, sheet_name=’Sales’, header=0, index_col=’OrderID’, usecols=’A:F’)
Display the first few rows of the DataFrame
print(sales_df.head())
“`
In this example, we read the ‘Sales’ sheet from the `sales_data.xlsx` file, using the ‘OrderID’ column as the index and selecting columns A to F.
Handling Multiple Sheets
If your Excel file contains multiple sheets, you can load them all into a dictionary of DataFrames by specifying `sheet_name=None`:
“`python
all_sheets = pd.read_excel(‘path_to_file.xlsx’, sheet_name=None)
“`
This will create a dictionary where the keys are the sheet names and the values are the corresponding DataFrames. For instance, to access a specific sheet, you would use:
“`python
specific_sheet_df = all_sheets[‘Sheet1’]
“`
Table of Common Parameters in read_excel
Below is a summary table of common parameters used with the `read_excel()` function:
Parameter | Description | Default Value |
---|---|---|
io | File path or object | N/A |
sheet_name | Sheet name or index | 0 (first sheet) |
header | Row number(s) to use as the header | 0 |
index_col | Column(s) to set as index | None |
usecols | Columns to read | All columns |
dtype | Data type for data or columns | None |
This table provides a quick reference to help you configure the import process effectively.
Choosing the Right Library
When importing Excel files in Python, several libraries are available, each with its strengths. The most commonly used libraries include:
- Pandas: A powerful data manipulation library that provides easy-to-use functions for importing Excel files.
- OpenPyXL: Primarily for reading and writing Excel 2010 xlsx/xlsm/xltx/xltm files.
- xlrd: Suitable for reading old Excel files (.xls) but not compatible with .xlsx files.
- pyexcel: Offers a simple interface to read, manipulate, and write Excel files.
Using Pandas to Import Excel Files
Pandas is the most popular choice for data analysis in Python due to its extensive functionality and ease of use. The following steps outline how to import an Excel file using Pandas:
- Install Pandas (if not already installed):
“`bash
pip install pandas
“`
- Import the Pandas library in your Python script:
“`python
import pandas as pd
“`
- Use the `read_excel()` function to import the Excel file:
“`python
df = pd.read_excel(‘file_path.xlsx’, sheet_name=’Sheet1′)
“`
Key parameters:
- `file_path`: Path to your Excel file.
- `sheet_name`: Specifies which sheet to load; can be a string or an integer.
Handling Multiple Sheets
If your Excel file contains multiple sheets, you can import them all or specify a subset. Here’s how to handle multiple sheets:
- Import all sheets into a dictionary of DataFrames:
“`python
all_sheets = pd.read_excel(‘file_path.xlsx’, sheet_name=None)
“`
- Accessing a specific sheet from the dictionary:
“`python
specific_sheet = all_sheets[‘Sheet1’]
“`
DataFrame Operations After Import
Once the data is imported into a DataFrame, various operations can be performed:
– **Displaying the first few rows** of the DataFrame:
“`python
print(df.head())
“`
– **Getting summary statistics** of the DataFrame:
“`python
print(df.describe())
“`
– **Filtering data** based on conditions:
“`python
filtered_data = df[df[‘Column_Name’] > threshold]
“`
Using OpenPyXL for Advanced Features
For advanced operations, such as formatting or modifying existing Excel files, OpenPyXL is preferred. Here’s how to use it:
- Install OpenPyXL:
“`bash
pip install openpyxl
“`
- Import OpenPyXL:
“`python
from openpyxl import load_workbook
“`
- Load the workbook and select a sheet:
“`python
workbook = load_workbook(‘file_path.xlsx’)
sheet = workbook[‘Sheet1’]
“`
- Accessing cell values:
“`python
value = sheet[‘A1’].value
“`
Exporting Data to Excel
After processing data, you might want to export your DataFrame back to an Excel file. With Pandas, this can be done easily:
“`python
df.to_excel(‘output_file.xlsx’, sheet_name=’ProcessedData’, index=)
“`
Key parameters:
- `output_file`: The destination file name.
- `sheet_name`: Name of the sheet in the output file.
- `index`: Whether to write row names (index).
Troubleshooting Common Issues
When working with Excel files, you may encounter some common issues:
Issue | Solution |
---|---|
FileNotFoundError | Ensure the file path is correct. |
ValueError on sheet names | Check the sheet names for typos or mismatches. |
MemoryError | Reduce the size of the file or increase system memory. |
Ensure that you have the necessary libraries installed and that your Excel files are formatted correctly to avoid these issues.
Expert Insights on Importing Excel Files in Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “Importing Excel files in Python can be efficiently handled using libraries like Pandas and OpenPyXL. These libraries provide robust functionalities that not only simplify the import process but also allow for extensive data manipulation and analysis.”
Michael Thompson (Software Engineer, Data Solutions Group). “When working with Excel files in Python, it is crucial to choose the right library based on your needs. For instance, while Pandas is excellent for data analysis, OpenPyXL is better suited for tasks involving Excel file formatting and writing.”
Sarah Patel (Python Developer, CodeCraft Labs). “I recommend using the ‘read_excel’ function from Pandas for its simplicity and efficiency. However, users should be aware of potential issues with file formats and ensure that they have the necessary dependencies installed to avoid runtime errors.”
Frequently Asked Questions (FAQs)
How can I import an Excel file in Python?
You can import an Excel file in Python using libraries such as `pandas` or `openpyxl`. The `pandas` library provides a convenient function called `read_excel()` that allows you to read Excel files directly into a DataFrame.
What libraries are commonly used to read Excel files in Python?
The most commonly used libraries for reading Excel files in Python are `pandas`, `openpyxl`, and `xlrd`. `pandas` is preferred for data manipulation, while `openpyxl` and `xlrd` are useful for reading and writing Excel files.
Do I need to install any packages to import Excel files in Python?
Yes, you need to install the `pandas` library and, depending on the Excel file format, either `openpyxl` or `xlrd`. You can install them using pip with the command `pip install pandas openpyxl xlrd`.
Can I read specific sheets from an Excel file using Python?
Yes, you can read specific sheets from an Excel file by specifying the `sheet_name` parameter in the `read_excel()` function. You can provide the sheet name or the sheet index (0 for the first sheet).
Is it possible to write data to an Excel file using Python?
Yes, you can write data to an Excel file using the `to_excel()` method provided by the `pandas` library. This allows you to export DataFrames to Excel files easily.
What file formats can I import using Python?
You can import `.xls` and `.xlsx` file formats using Python. The `pandas` library supports both formats, while `openpyxl` is specifically designed for `.xlsx` files.
In summary, importing Excel files in Python is a straightforward process that can be accomplished using various libraries, with the most popular being Pandas and openpyxl. These libraries provide robust functionalities that allow users to read, manipulate, and analyze data stored in Excel formats (.xls and .xlsx). The choice of library may depend on specific requirements, such as the need for advanced data manipulation or compatibility with older Excel formats.
Utilizing Pandas, for instance, enables users to easily read Excel files into DataFrames, facilitating data analysis and visualization. The `read_excel()` function is particularly useful, as it allows for the specification of sheet names, data types, and other parameters. On the other hand, openpyxl is preferred for tasks that require more control over the Excel file structure, such as writing to Excel files or modifying existing ones.
Key takeaways include the importance of installing the necessary libraries using package managers like pip and understanding the various parameters that can be passed to the functions for optimal performance. Additionally, users should be aware of the potential need for additional dependencies, such as xlrd for reading older Excel files, to ensure compatibility and functionality.
Author Profile
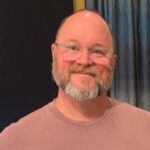
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?