How Can You Retrieve Input Values Using JavaScript?
### Introduction
In the world of web development, user interaction is at the heart of creating dynamic and engaging applications. One of the fundamental aspects of this interaction is capturing user input, whether it’s through forms, search bars, or any other input fields. Understanding how to get the input value in JavaScript is a crucial skill for developers looking to enhance their websites and applications. This knowledge not only empowers you to collect data but also allows you to respond to user actions in real-time, creating a more interactive experience.
As we delve into the intricacies of retrieving input values in JavaScript, we’ll explore various methods and techniques that can be employed to access and manipulate user input effectively. From simple text fields to more complex elements like checkboxes and radio buttons, JavaScript provides a versatile toolkit for handling different types of input. Whether you’re a novice looking to grasp the basics or an experienced developer seeking to refine your skills, understanding how to work with input values is essential for building responsive web applications.
In this article, we’ll cover the foundational concepts and best practices for getting input values in JavaScript. By the end, you’ll be equipped with the knowledge to seamlessly integrate user input into your projects, enhancing both functionality and user experience. So, let’s embark on this journey to unlock the potential of Java
Accessing Input Values with JavaScript
To get the input value in JavaScript, you typically interact with HTML input elements through the Document Object Model (DOM). This can be achieved using various methods, but the most common approach is to use `document.getElementById()` or `document.querySelector()`.
When retrieving values from input fields, it is essential to ensure that the input element is accessible in the DOM and that you are using the correct method to reference it.
Using `document.getElementById`
The `getElementById()` method allows you to select an element by its unique ID. Here’s how to use it:
In this example, when the button is clicked, the function `getInputValue()` retrieves the value of the input field with the ID `myInput` and logs it to the console.
Using `document.querySelector`
Another approach is to use `querySelector()`, which allows for more complex selections using CSS selectors. This method is particularly useful for selecting multiple types of elements or when dealing with classes.
This code snippet demonstrates how to select an input field using its class name.
Handling Different Input Types
It is important to consider that different input types may require specific handling methods. Below are some common input types:
- Text
- Number
- Checkbox
- Radio
- Select (Dropdown)
Input Type | Example | Retrieval Method |
---|---|---|
Text | `` | `input.value` |
Number | `` | `input.valueAsNumber` |
Checkbox | `` | `input.checked` |
Radio | `` | `document.querySelector(‘input[name=”radioName”]:checked’).value` |
Select | ` | `select.value` |
Event Listeners for Dynamic Retrieval
To dynamically retrieve input values as users interact with the form, you can attach event listeners. This allows you to respond to changes in real time:
In this example, the displayed value updates as the user types in the input field. This technique enhances user experience by providing immediate feedback.
By understanding these fundamental methods and practices for accessing input values in JavaScript, you can effectively manage user input in your web applications.
Accessing Input Values Using JavaScript
To retrieve the value from an input element in JavaScript, several methods can be employed depending on the context of your application and the structure of your HTML. Below are the most common methods.
Using `document.getElementById()`
This method is straightforward for accessing an input element by its unique ID.
- The `getElementById()` function returns the element with the specified ID.
- The `.value` property is used to access the current value of the input.
Using `document.querySelector()`
`querySelector()` allows for more flexible selection using CSS-like selectors.
- This method can select elements by class, attribute, or other selectors.
- It returns the first matching element from the document.
Using Event Listeners
Event listeners can be used to retrieve the input value dynamically as the user interacts with the input field.
- The `input` event fires whenever the value of the input changes.
- This method provides real-time feedback, displaying the value in a paragraph element.
Working with Forms
When dealing with multiple inputs within a form, it’s often useful to access all input values collectively.
- `FormData` captures all form elements and their values.
- The `forEach` method allows iterating over key-value pairs, making it easy to handle multiple inputs.
Accessing Values from Radio Buttons and Checkboxes
Retrieving values from radio buttons and checkboxes requires specific handling.
Red
Blue
- This approach iterates through radio button elements to check which one is selected.
- The first `checked` radio’s value is stored and logged.
Retrieving Values in Modern JavaScript
For modern applications, using arrow functions and the spread operator can simplify code.
- Arrow functions provide a concise syntax for event handlers.
- This approach enhances readability and maintains function scope naturally.
Expert Insights on Retrieving Input Values in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively retrieve input values in JavaScript, one should utilize the Document Object Model (DOM) methods such as `getElementById` or `querySelector`. These methods allow developers to access input elements directly and retrieve their values using the `.value` property, ensuring a seamless user experience.”
Mark Thompson (Front-End Developer, Creative Solutions). “Understanding event handling is crucial when getting input values in JavaScript. By attaching event listeners to input fields, developers can capture user input in real-time, which enhances interactivity and responsiveness of web applications.”
Linda Garcia (JavaScript Educator, Code Academy). “For beginners, I recommend starting with simple forms and practicing how to access input values using both traditional methods and modern frameworks like React. This foundational knowledge is essential for building dynamic and interactive web applications.”
Frequently Asked Questions (FAQs)
How can I retrieve the value of an input field in JavaScript?
You can retrieve the value of an input field by using the `value` property of the input element. For example, if your input has an ID of `myInput`, you can use `document.getElementById(‘myInput’).value` to get its value.
What is the difference between `getElementById` and `querySelector` for getting input values?
`getElementById` selects an element by its unique ID, while `querySelector` allows you to select elements using CSS selectors. Both can be used to retrieve input values, but `querySelector` offers more flexibility in selecting elements.
How do I get the value of a checkbox in JavaScript?
To get the value of a checkbox, use the `checked` property. For example, `document.getElementById(‘myCheckbox’).checked` returns `true` if the checkbox is checked and “ otherwise.
Can I get the value of multiple input fields at once?
Yes, you can use a loop to iterate through a collection of input fields. For example, using `document.querySelectorAll(‘input’)` allows you to select all input elements and then access their values in a loop.
How do I handle input value changes in JavaScript?
You can handle input value changes by adding an event listener for the `input` or `change` event. For example, `document.getElementById(‘myInput’).addEventListener(‘input’, function() { console.log(this.value); });` logs the current value whenever it changes.
Is it possible to get input values from a form in JavaScript?
Yes, you can retrieve input values from a form by using the `elements` property of the form. For example, `document.forms[‘myForm’].elements[‘myInput’].value` retrieves the value of an input field named `myInput` within the form.
In summary, obtaining the input value in JavaScript is a fundamental skill for web developers. The most common method involves using the Document Object Model (DOM) to access input elements. By utilizing methods such as `getElementById`, `querySelector`, or `getElementsByClassName`, developers can effectively target specific input fields and retrieve their values. It is important to understand the difference between these methods, as they offer varying levels of specificity and flexibility when selecting elements.
Another key aspect to consider is the timing of when to retrieve the input value. It is often essential to capture the value during specific events, such as form submission or user input. Event listeners can be employed to trigger functions that collect input values at the appropriate moments, ensuring that the data is accurate and relevant. Additionally, handling input values correctly can enhance user experience by providing real-time feedback or validation.
Moreover, developers should be aware of the different types of input elements, such as text fields, checkboxes, and radio buttons, as each type may require unique handling methods. Understanding how to manage these various input types is crucial for creating interactive and user-friendly web applications. Overall, mastering the techniques for retrieving input values in JavaScript is vital for effective client
Author Profile
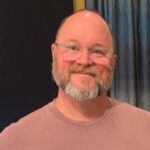
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?