How Can You Resolve Compiler Error CS0433 in Your C# Code?
Encountering compiler errors can be a frustrating experience for developers, especially when they interrupt the flow of coding and hinder progress. One such error that many Cprogrammers may face is CS0433, which indicates a name conflict between types. This error can arise unexpectedly, leaving developers puzzled as they try to decipher the root cause. Understanding how to effectively troubleshoot and resolve CS0433 is essential for maintaining a smooth development process and ensuring that your applications run as intended. In this article, we will explore the nuances of this compiler error, providing you with the insights needed to tackle it head-on.
In the world of Cprogramming, clarity and precision in naming conventions are crucial. The CS0433 error typically occurs when two or more types share the same name within the same scope, leading to ambiguity that the compiler cannot resolve. This situation can arise from various scenarios, such as importing namespaces that contain overlapping type names or defining classes with identical identifiers. By recognizing the common triggers for this error, developers can take proactive steps to prevent it from occurring in the first place.
Moreover, resolving CS0433 is not just about fixing the immediate issue; it also involves understanding best practices in code organization and naming conventions. By adopting strategies to avoid naming conflicts, you can enhance the
Understanding Compiler Error CS0433
Compiler error CS0433 occurs in Cwhen there are ambiguous references to types. This typically arises when two or more types in different namespaces share the same name, leading to confusion in type resolution. The compiler cannot determine which type you are referring to, resulting in this error.
To resolve CS0433, it is essential to identify the conflicting types and specify which one you intend to use. This may involve using fully qualified names or importing the correct namespaces.
Common Causes of CS0433
Several scenarios can trigger the CS0433 error:
- Namespace Conflicts: When two namespaces contain types with the same name.
- Ambiguous References in Using Directives: Multiple `using` statements can lead to conflicts if they import the same type from different assemblies.
- Type Aliases: If you create a type alias that conflicts with another type in the project.
- Incorrect Assembly References: Referencing assemblies that contain types with the same name but different implementations.
Steps to Fix CS0433
To effectively resolve CS0433, you can follow these steps:
- Identify the Conflicting Types: Check the error message details to see which types are conflicting.
- Use Fully Qualified Names: Instead of just using the type name, include the namespace. For example:
“`csharp
var myObject = new NamespaceA.MyClass();
“`
- Remove Unnecessary Using Directives: If certain namespaces are not required, removing them can resolve conflicts.
- Create Type Aliases: If you need both types, create an alias for one of them:
“`csharp
using MyClassAlias = NamespaceA.MyClass;
“`
- Review Project References: Ensure that you are referencing the correct assemblies and that no unnecessary assemblies are included.
Action | Example |
---|---|
Fully Qualified Name | NamespaceA.MyClass |
Type Alias | using MyClassAlias = NamespaceA.MyClass; |
Removing Using Directive | Remove `using NamespaceB;` |
Best Practices to Avoid CS0433
To minimize the chances of encountering CS0433 in the future, consider the following best practices:
- Namespace Planning: Organize your code with unique namespaces to avoid conflicts.
- Limit Using Statements: Only include `using` directives that are necessary for the current file.
- Consistent Naming Conventions: Adopt consistent naming conventions for types to reduce ambiguity.
- Regular Code Reviews: Conduct regular reviews to identify potential conflicts early.
By adhering to these practices, you can create a more maintainable codebase and reduce the likelihood of encountering compiler errors such as CS0433.
Understanding Compiler Error CS0433
Compiler Error CS0433 occurs when the Ccompiler finds more than one definition for a type or namespace that it is trying to resolve. This is often due to naming conflicts between assemblies or files within the same project. To effectively address this issue, it is essential to identify the source of the conflict.
Common Causes of CS0433
Several factors can lead to this compiler error. Understanding these causes is crucial for quick resolution:
- Duplicate Class Names: Two or more classes with the same name in different namespaces or assemblies.
- Assembly References: Multiple references to different versions of the same assembly.
- File Naming Conflicts: Conflicts arising from files with the same names in the same or different folders.
- Namespace Clashes: Classes in different namespaces that are being used without proper qualification.
How to Identify the Conflict
To resolve CS0433, follow these steps to pinpoint the conflict:
- Examine Error Messages: Review the full error message in the output window. It usually specifies which types are conflicting.
- Check References: Go to the project references in your solution and identify any duplicate or conflicting assemblies.
- Namespace Qualification: Use fully qualified names to disambiguate between classes or namespaces when needed.
- Search for Duplicates: Use your IDE’s search functionality to look for duplicate class names across your project.
Steps to Resolve CS0433
Once the source of the conflict is identified, you can take the following actions:
- Rename Classes: If feasible, rename one of the conflicting classes to a unique name.
- Adjust Namespace: Place the conflicting class in a different namespace to avoid naming collisions.
- Remove Redundant References: Eliminate any unnecessary or duplicate assembly references in your project.
- Check for Updates: Ensure that all referenced assemblies are up to date and compatible with each other.
Example Scenarios
Here are some common scenarios that illustrate how CS0433 might occur:
Scenario | Description |
---|---|
Duplicate Class in Same Namespace | Class `User` defined in both `ProjectA.Models` and `ProjectB.Models`. |
Conflicting Assemblies | Referencing `LibraryV1.dll` and `LibraryV2.dll`, both containing a class `Service`. |
File Naming Conflict | Two files named `Utilities.cs` within the same project folder. |
Best Practices to Avoid CS0433
Implementing best practices can significantly reduce the likelihood of encountering CS0433:
- Use Unique Naming Conventions: Adopt a clear and consistent naming convention for classes and files.
- Organize Code Effectively: Structure your code into well-defined namespaces and folders.
- Limit Assembly References: Avoid unnecessary references to assemblies, particularly those that may contain conflicting types.
- Regular Code Reviews: Conduct code reviews to catch potential naming conflicts early in the development process.
By following these guidelines, you can effectively manage and prevent Compiler Error CS0433 in your Cprojects.
Expert Insights on Resolving Compiler Error CS0433
Dr. Emily Carter (Senior Software Engineer, Code Solutions Inc.). “Compiler error CS0433 typically indicates that there is a name conflict due to multiple definitions of a type in the same namespace. To resolve this, I recommend checking your project references and ensuring that you do not have duplicate classes or conflicting namespaces.”
Michael Thompson (Lead Developer, Tech Innovations Group). “When facing CS0433, it’s crucial to examine your using directives and the scope of your classes. Often, this error arises from ambiguous references. A thorough review of your code structure can help clarify these conflicts.”
Linda Garcia (Software Architect, FutureTech Labs). “In my experience, CS0433 can also occur when working with third-party libraries that may define types with the same name as your own. Always ensure that your project is using the correct version of these libraries and consider employing aliasing to avoid such conflicts.”
Frequently Asked Questions (FAQs)
What does compiler error CS0433 indicate?
Compiler error CS0433 indicates that there is a type name conflict in your code. This error occurs when two or more types with the same name are found in the same scope, leading to ambiguity.
How can I resolve compiler error CS0433?
To resolve compiler error CS0433, you should ensure that all type names in your code are unique within the same namespace or scope. You can either rename one of the conflicting types or use fully qualified names to specify which type you intend to use.
What causes CS0433 in a Cproject?
CS0433 is typically caused by importing multiple namespaces that contain types with the same name. This often occurs when using third-party libraries alongside your own code or when there are duplicate class definitions.
Can using aliases help fix CS0433?
Yes, using aliases can help fix CS0433. By creating an alias for one of the conflicting namespaces, you can differentiate between the types and avoid ambiguity in your code.
Is CS0433 specific to certain versions of C?
No, CS0433 is not specific to any version of C. This error can occur in any version of Cwhere there are naming conflicts in the code.
Are there any tools to help identify CS0433 errors?
Yes, many integrated development environments (IDEs) like Visual Studio provide built-in tools to identify and highlight compiler errors, including CS0433. Additionally, static analysis tools can help detect naming conflicts before compilation.
In summary, the compiler error CS0433 in Cindicates that there is a type name ambiguity due to multiple definitions of the same type in different namespaces or assemblies. This error commonly arises when two or more references in a project contain types with the same name, leading to confusion for the compiler regarding which type to use. Understanding the context of the error is essential for effectively resolving it.
To fix CS0433, developers should first identify the conflicting types by examining the error message, which typically includes the names of the types and their respective namespaces. Once the source of the ambiguity is determined, developers can choose to either fully qualify the type names with their namespaces, remove unnecessary references, or rename the conflicting types if possible. These strategies help clarify which type should be utilized and eliminate the ambiguity.
Key takeaways include the importance of maintaining organized namespaces and carefully managing project references to avoid conflicts. Regularly reviewing dependencies and ensuring that type names are unique within a given context can significantly reduce the likelihood of encountering CS0433. By following best practices in naming conventions and project structure, developers can enhance code clarity and prevent similar compiler errors in the future.
Author Profile
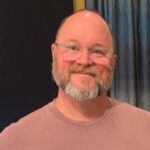
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?