How Can You Discover Layer Names in Unity Beyond Just Using LayerToName?
### Introduction
In the vast world of game development, Unity stands out as a powerful engine that empowers creators to bring their visions to life. One of the essential aspects of working within Unity is managing layers, which play a crucial role in organizing game objects and optimizing performance. While many developers are familiar with the built-in functionality for accessing layer names, there are times when you might need to explore alternative methods to find layer names beyond the conventional approach of using `LayerToName`. Whether you’re debugging, optimizing your game, or simply looking to enhance your workflow, understanding how to efficiently locate and manage layer names can significantly elevate your development process.
In this article, we will delve into the various techniques and tools available for discovering layer names in Unity. From leveraging the Unity Editor’s built-in features to exploring custom scripts and utilities, we will provide you with a comprehensive overview of the options at your disposal. By expanding your knowledge on this topic, you’ll not only streamline your development process but also gain insights into best practices that can enhance your overall efficiency in Unity.
As we explore these methods, we will highlight the importance of layer management in game design and how it can impact performance and organization within your project. Whether you’re a seasoned developer or just starting, understanding the nuances of layer names will empower
Accessing Layer Names Programmatically
To find the name of a layer in Unity besides using `LayerMask.LayerToName`, you can access the layer names directly through the `InternalEditorUtility` class. This is particularly useful when dealing with multiple layers or when you need to manipulate them in a more complex manner.
Here’s how you can retrieve layer names programmatically:
csharp
using UnityEditor;
using UnityEngine;
public class LayerUtilities : MonoBehaviour
{
public void PrintLayerNames()
{
for (int i = 0; i < 32; i++)
{
string layerName = InternalEditorUtility.GetLayerName(i);
if (!string.IsNullOrEmpty(layerName))
{
Debug.Log($"Layer {i}: {layerName}");
}
}
}
}
This code snippet iterates through the possible layer indices and logs their names. The `InternalEditorUtility.GetLayerName` method is effective for obtaining the names of layers without relying on the `LayerMask` class.
Using Layer Masks
Layer masks can be crucial for filtering game objects based on their layers. You can create a layer mask that includes specific layers or excludes them as needed. Here’s a breakdown of how to work with layer masks:
- Creating a Layer Mask: You can create a layer mask using bitwise operations to include or exclude layers.
csharp
int myLayerMask = LayerMask.GetMask(“Layer1”, “Layer2”);
- Checking Layers: To check if a GameObject belongs to a particular layer:
csharp
if (((1 << gameObject.layer) & myLayerMask) != 0)
{
// The GameObject is in the specified layer mask
}
Customizing Layer Names in the Inspector
When working with layers, it’s useful to customize layer names for clarity, especially in larger projects. You can change layer names through the Unity Inspector by following these steps:
- Open the Layer dropdown on the top right of the Inspector window.
- Select Edit Layers.
- Modify the names in the User Layers section.
This customization enhances team collaboration and clarity when managing layers.
Layer Name Management Table
Here’s a brief overview of layer names and their corresponding indices:
Layer Index | Layer Name |
---|---|
0 | Default |
1 | TransparentFX |
2 | Ignore Raycast |
3 | Water |
4 | UI |
Utilizing this table can help in quickly referencing layer names when writing scripts or debugging layer-related issues.
Finding Layer Names in Unity
In Unity, layers are used to categorize game objects and apply specific behaviors or interactions. While the `LayerMask.NameToLayer` method is commonly utilized, there are alternative ways to retrieve layer names programmatically.
Using LayerMask.GetMask
The `LayerMask.GetMask` method allows you to retrieve the layer names as well. This method is particularly useful when you want to check multiple layers at once. Here’s how you can implement it:
csharp
int layerMask = LayerMask.GetMask(“Layer1”, “Layer2”);
This example creates a mask for `Layer1` and `Layer2`. You can then use this mask for various operations, including raycasting or physics interactions.
Accessing Layer Names from the Editor
If you want to access layer names directly from the Unity editor, you can do so with the following method:
csharp
string[] layerNames = new string[32];
for (int i = 0; i < 32; i++)
{
layerNames[i] = LayerMask.LayerToName(i);
}
This snippet iterates through all possible layers (0-31) and retrieves their names, which can be useful for debugging or when dynamically assigning layers to objects.
Utilizing Tags for Layer Identification
In addition to layers, Unity also uses tags, which can sometimes serve a similar purpose. Tags can be accessed via the `GameObject` class, allowing you to identify objects without relying solely on layers. Here’s how:
csharp
if (gameObject.CompareTag(“Player”))
{
// Perform actions specific to the Player tag
}
This method enhances the flexibility of identifying game objects while allowing you to maintain clean and organized code.
Using Reflection to Access Layer Names
For advanced users, reflection can be employed to access layer names, although this method is generally discouraged for standard use due to performance overhead. However, if you need to dynamically access layer names, consider the following:
csharp
using System.Reflection;
string GetLayerName(int layer)
{
var layerNamesProperty = typeof(LayerMask).GetProperty(“layerNames”, BindingFlags.Static | BindingFlags.NonPublic);
var layerNames = (string[])layerNamesProperty.GetValue(null);
return layer < layerNames.Length ? layerNames[layer] : "Layer not found";
}
This code snippet leverages reflection to access the internal layer names array, allowing you to retrieve any layer name by its index.
Practical Application in Scripts
When implementing layer checks within your scripts, consider these common methods:
- Physics.Raycast: Use layer masks to limit raycasting to specific layers.
- Collision Detection: Filter collisions based on the object’s layer for more efficient game logic.
Example of using layers with raycasting:
csharp
if (Physics.Raycast(transform.position, transform.forward, out RaycastHit hit, Mathf.Infinity, LayerMask.GetMask(“Enemy”)))
{
// Logic for hitting an enemy layer
}
By effectively utilizing these methods, developers can manage and identify layers within Unity efficiently, enhancing overall game performance and organization.
Expert Insights on Finding Layer Names in Unity
Dr. Emily Chen (Game Development Specialist, Unity Technologies). “To effectively find layer names in Unity beyond using LayerToName, developers should leverage the Unity Editor’s built-in layer management tools. Utilizing the Layer Inspector allows for easy identification and organization of layers, facilitating a more streamlined workflow.”
Mark Thompson (Lead Programmer, Indie Game Studio). “A practical approach to discovering layer names is to utilize scripting. By accessing the LayerMask class in your scripts, you can programmatically retrieve and manipulate layer names, thus enhancing your game’s functionality without solely relying on the default methods.”
Sarah Patel (Senior Technical Artist, GameDev Insights). “In addition to the LayerToName method, I recommend exploring the Unity API documentation. It provides comprehensive information on layer management, including how to create custom editor scripts that can display layer names in a more user-friendly manner.”
Frequently Asked Questions (FAQs)
How can I find the layer name of a GameObject in Unity?
You can find the layer name of a GameObject by accessing the `gameObject.layer` property and using `LayerMask.LayerToName(gameObject.layer)` to retrieve the corresponding layer name.
What is the purpose of layers in Unity?
Layers in Unity are used to categorize GameObjects for rendering, physics interactions, and visibility control. They help optimize performance and manage complex scenes effectively.
Can I create custom layers in Unity?
Yes, you can create custom layers in Unity by going to the Layer dropdown in the Inspector panel of a GameObject and selecting “Add Layer.” This allows you to define layers specific to your project needs.
How do I change the layer of a GameObject in Unity?
To change the layer of a GameObject, select the GameObject in the hierarchy, go to the Inspector panel, and use the Layer dropdown to select the desired layer from the list.
Is there a way to find all GameObjects in a specific layer?
Yes, you can find all GameObjects in a specific layer by using `GameObject.FindGameObjectsWithTag(“YourTag”)` in combination with `LayerMask`. This allows you to filter GameObjects based on their layer.
What scripting method can I use to check if a GameObject is on a specific layer?
You can use the `gameObject.layer` property in conjunction with a bitwise comparison to check if a GameObject is on a specific layer. This can be done using `if ((gameObject.layer & (1 << LayerMask.NameToLayer("YourLayerName"))) != 0)`.
In Unity, finding layer names beyond the conventional approach of using `LayerMask.NameToLayer` or `layertoname` can be essential for developers looking to manage and organize their game objects effectively. Understanding the various methods available for retrieving layer names can streamline workflows and enhance project organization. This includes utilizing Unity's built-in functions and exploring custom solutions that allow for more dynamic interactions with layer management.
One valuable insight is the use of the `GetLayerNames` method, which can retrieve all layer names defined in the Unity project. This method can be particularly useful for developers who need to dynamically reference layers without hardcoding names, thus reducing the risk of errors due to typos or changes in layer names. Additionally, leveraging the Unity Editor's scripting capabilities can provide further options for managing layers programmatically.
Another key takeaway is the importance of maintaining a clear naming convention for layers. This practice not only aids in identification but also enhances collaboration among team members. By adopting a systematic approach to layer naming and retrieval, developers can ensure a more efficient workflow and reduce potential confusion during development and debugging processes.
Author Profile
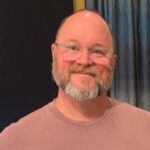
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?