How Can You Determine the Database Size in SQL Server?
In the fast-paced world of data management, understanding the size of your SQL Server databases is crucial for optimizing performance, managing resources, and planning for future growth. Whether you’re a database administrator, a developer, or a business analyst, knowing how to assess the size of your databases can empower you to make informed decisions that enhance efficiency and scalability. As data continues to proliferate, the ability to monitor and manage database size becomes not just a technical necessity, but a strategic advantage.
Determining the size of a database in SQL Server involves more than just a simple query; it encompasses a range of considerations, from storage allocation to data growth patterns. By grasping the various components that contribute to database size, you can better understand how your data is structured and how it evolves over time. This knowledge is essential for effective capacity planning and for ensuring that your database remains responsive and reliable under increasing loads.
In this article, we will explore the methods and tools available for assessing database size in SQL Server. From built-in functions to dynamic management views, you will learn how to gather insights into your database’s footprint and leverage this information for improved database management. Get ready to dive into the world of SQL Server database size analysis and unlock the potential of your data infrastructure!
Using SQL Server Management Studio (SSMS)
To find the database size in SQL Server using SQL Server Management Studio, you can utilize a straightforward method through the graphical user interface. Follow these steps:
- Open SQL Server Management Studio and connect to your database instance.
- In the Object Explorer, locate and right-click on the database you want to check.
- Select “Properties” from the context menu.
- In the Database Properties window, navigate to the “General” page.
- You will find the database size listed under the “Size” field.
This method provides a quick overview of the database size, including both the data and log files.
Querying Database Size with T-SQL
For a more programmatic approach, you can execute a Transact-SQL (T-SQL) query to retrieve the database size. Here’s a commonly used query:
“`sql
EXEC sp_spaceused;
“`
This stored procedure returns the following information:
- database_name: Name of the database.
- database_size: Total size of the database.
- unallocated space: Amount of space that is not allocated to any object.
- reserved space: Total space reserved for the database.
- data_size: Size of the data files.
- index_size: Size of the index files.
- log_size: Size of the log files.
You can also use this query to get more granular details about each file in the database:
“`sql
SELECT
name AS [File Name],
size/128 AS [Total Size (MB)],
size/128 – CAST(FILEPROPERTY(name, ‘SpaceUsed’) AS int)/128 AS [Available Space (MB)]
FROM sys.master_files
WHERE database_id = DB_ID(‘YourDatabaseName’);
“`
Replace `’YourDatabaseName’` with the actual name of your database.
Understanding Database File Types
When assessing database size, it’s essential to understand the types of files involved:
- Data Files (.mdf and .ndf): These files store the actual data.
- Log Files (.ldf): These files store transaction logs, which are essential for database recovery.
Below is a table summarizing the file types and their purposes:
File Type | File Extension | Description |
---|---|---|
Primary Data File | .mdf | Stores data and objects, including tables and indexes. |
Secondary Data File | .ndf | Used to store data, can be added if the primary file is full. |
Transaction Log File | .ldf | Records all transactions and database modifications. |
Understanding these file types is crucial when managing and optimizing database size.
Using T-SQL to Determine Database Size
To find the size of a database in SQL Server, you can utilize Transact-SQL (T-SQL) commands. The following query provides details about the database size, including the total size, used space, and free space.
“`sql
USE [YourDatabaseName];
GO
EXEC sp_spaceused;
“`
This stored procedure returns the following columns:
- database_name: Name of the database.
- database_size: Total size of the database.
- unallocated space: Amount of free space within the database.
For a more detailed view, including sizes of individual database files, the following query can be executed:
“`sql
SELECT
name AS [File Name],
size/128.0 AS [Total Size (MB)],
size/128.0 – CAST(FILEPROPERTY(name, ‘SpaceUsed’) AS INT)/128.0 AS [Used Space (MB)],
CAST(FILEPROPERTY(name, ‘SpaceUsed’) AS INT)/128.0 AS [Free Space (MB)]
FROM
sys.master_files
WHERE
database_id = DB_ID(‘YourDatabaseName’);
“`
This query retrieves information about each file associated with the specified database.
Using SQL Server Management Studio (SSMS)
In SQL Server Management Studio, you can visually check the database size through the following steps:
- Connect to the SQL Server instance.
- In the Object Explorer, right-click on the database you want to check.
- Select Properties.
- Navigate to the General tab.
Here, you’ll find the Size and Space Available metrics.
Using SQL Server Management Views
SQL Server provides management views that can be queried to obtain database size information. The following example uses the `sys.dm_db_partition_stats` view:
“`sql
SELECT
DB_NAME(database_id) AS [Database Name],
SUM(reserved_page_count) * 8 / 1024 AS [Size (MB)]
FROM
sys.dm_db_partition_stats
GROUP BY
database_id;
“`
This query calculates the size of all databases in megabytes by summing the reserved pages.
Monitoring Database Size Growth
To monitor how a database size changes over time, consider enabling database growth tracking. You can set up alerts or log this information regularly using a scheduled job. An example query to check the size over time can be:
“`sql
SELECT
name AS [Database Name],
CAST(size * 8 / 1024 AS VARCHAR) + ‘ MB’ AS [Size]
FROM
sys.master_files
WHERE
database_id = DB_ID(‘YourDatabaseName’);
“`
This can be run at intervals to log changes in size.
Considerations and Best Practices
When managing database size, consider the following best practices:
- Regularly monitor database size to prevent unexpected growth.
- Set appropriate growth settings for database files to optimize space usage.
- Archive or purge old data to maintain manageable database sizes.
- Use compression techniques where applicable to save space.
By following these methods and practices, you can effectively manage and monitor the size of your SQL Server databases.
Expert Insights on Determining Database Size in SQL Server
Dr. Emily Chen (Database Architect, Tech Solutions Inc.). “To accurately find the database size in SQL Server, one should utilize the system stored procedure sp_spaceused. This method provides a comprehensive breakdown of the database’s space usage, including the total size, unallocated space, and the size of data and indexes, which is crucial for effective database management.”
Mark Thompson (Senior SQL Server Consultant, Data Dynamics). “In addition to sp_spaceused, I recommend using the sys.master_files catalog view for a more granular analysis. This allows you to see the size of each file associated with the database, which can be particularly useful when managing multiple files or filegroups within SQL Server.”
Linda Patel (SQL Server Database Administrator, Cloud Data Experts). “For ongoing monitoring of database size, implementing a SQL Server Agent job that runs a script to log the size at regular intervals can be invaluable. This proactive approach not only helps in tracking growth trends but also aids in capacity planning and resource allocation.”
Frequently Asked Questions (FAQs)
How can I check the size of a specific database in SQL Server?
You can check the size of a specific database by executing the following SQL query:
“`sql
USE [YourDatabaseName];
EXEC sp_spaceused;
“`
This will return the total size of the database along with the space used and unallocated space.
What SQL command provides the size of all databases in SQL Server?
To retrieve the size of all databases, you can run the following query:
“`sql
SELECT DB_NAME(database_id) AS DatabaseName,
SUM(size * 8 / 1024) AS SizeMB
FROM sys.master_files
GROUP BY database_id;
“`
This will display the size of each database in megabytes.
How do I find the size of database files in SQL Server?
To find the size of individual database files, you can execute:
“`sql
SELECT name AS FileName,
size * 8 / 1024 AS SizeMB
FROM sys.master_files
WHERE database_id = DB_ID(‘YourDatabaseName’);
“`
This provides the size of each file associated with the specified database.
Is there a way to get the size of a database including unallocated space?
Yes, you can use the following query to get the total size, including unallocated space:
“`sql
EXEC sp_spaceused @updateusage = N’TRUE’;
“`
This will give you a comprehensive view of both used and unused space in the database.
Can I find the database size using SQL Server Management Studio (SSMS)?
Yes, in SSMS, you can right-click on the database, select Properties, and navigate to the General page. Here, you will see the database size displayed in the Size field.
What factors can affect the size of a database in SQL Server?
Several factors can affect the size of a database, including the amount of data stored, the number of indexes, the use of large objects (LOBs), transaction logs, and database growth settings. Regular maintenance, such as index rebuilding and data archiving, can help manage database size effectively.
Finding the database size in SQL Server is a crucial task for database administrators and developers alike. Understanding the size of a database helps in efficient resource management, capacity planning, and performance optimization. SQL Server provides various methods to determine the size of a database, including using system stored procedures, querying system views, and utilizing SQL Server Management Studio (SSMS) graphical interface.
One of the most common methods involves using the `sp_spaceused` stored procedure, which provides detailed information about the total size of the database, including data and log file sizes. Additionally, querying the `sys.master_files` system view allows for a more granular approach by retrieving the size of individual database files. These methods can be executed through T-SQL commands, making them accessible for automation and scripting purposes.
In summary, regularly monitoring the database size is essential for maintaining optimal performance and ensuring that storage resources are effectively utilized. By leveraging the built-in tools and procedures provided by SQL Server, users can easily obtain the necessary information to make informed decisions regarding database management and maintenance.
Author Profile
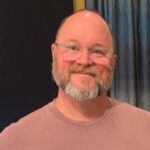
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?