How Can You Easily Fetch Guilds Using Discord.js?
In the vibrant world of Discord, guilds—more commonly known as servers—serve as the backbone of community interaction. Whether you’re a developer looking to enhance your bot’s capabilities or a community manager aiming to streamline server interactions, understanding how to fetch guilds using Discord.js is an essential skill. This powerful JavaScript library simplifies the process of interacting with the Discord API, enabling you to create dynamic and engaging experiences for users. In this article, we will explore the intricacies of fetching guilds, providing you with the knowledge to tap into the full potential of your Discord bot.
Fetching guilds in Discord.js is more than just a technical task; it opens the door to a myriad of possibilities for bot functionality and community engagement. By accessing guild data, developers can retrieve essential information such as member counts, roles, channels, and more, allowing for tailored interactions that enhance user experience. Whether you’re building a bot for game nights, community announcements, or moderation tasks, knowing how to effectively fetch and utilize guild information is crucial.
As we delve deeper into the mechanics of fetching guilds, we will cover the fundamental concepts, best practices, and common pitfalls to avoid. With a solid understanding of these principles, you’ll be well-equipped to create bots that not only meet the
Fetching Guilds with Discord.js
To fetch guilds using Discord.js, you can utilize the `GuildManager` class, which provides various methods to interact with guilds. This functionality is particularly useful when you want to retrieve a list of guilds that the bot is a member of.
To start, ensure you have your bot client set up and that you are logged in. The following code snippet demonstrates how to fetch all guilds:
“`javascript
const { Client, GatewayIntentBits } = require(‘discord.js’);
const client = new Client({ intents: [GatewayIntentBits.Guilds] });
client.once(‘ready’, () => {
console.log(`Logged in as ${client.user.tag}`);
const guilds = client.guilds.cache.map(guild => ({
id: guild.id,
name: guild.name,
memberCount: guild.memberCount
}));
console.table(guilds);
});
client.login(‘YOUR_BOT_TOKEN’);
“`
In this example, the bot logs in and, once ready, retrieves the guilds from the cache. The `map` function is used to create an array of objects containing essential information about each guild, including its ID, name, and member count.
Understanding Guild Properties
When working with guilds, it is crucial to understand the various properties that the `Guild` object exposes. Here are some key properties you may find useful:
- id: The unique identifier for the guild.
- name: The name of the guild.
- icon: The URL of the guild’s icon, if it has one.
- memberCount: The total number of members in the guild.
- channels: The collection of channels in the guild.
The following table summarizes these properties:
Property | Description |
---|---|
id | Unique identifier for the guild |
name | Name of the guild |
icon | URL of the guild’s icon |
memberCount | Total number of members in the guild |
channels | Collection of channels in the guild |
Fetching Specific Guild Information
If you need to fetch a specific guild’s information, you can use the `fetch` method provided by the `GuildManager`. Here’s how you can do it:
“`javascript
client.guilds.fetch(‘GUILD_ID’)
.then(guild => {
console.log(`Fetched Guild: ${guild.name} with ID: ${guild.id}`);
})
.catch(console.error);
“`
Replace `’GUILD_ID’` with the actual ID of the guild you want to fetch. The `fetch` method returns a promise that resolves to the `Guild` object, allowing you to access its properties directly.
In situations where you may need to handle multiple guilds or perform asynchronous operations, consider using `Promise.all` for concurrent fetching. This approach improves efficiency and reduces the waiting time for multiple requests:
“`javascript
const guildIds = [‘GUILD_ID_1’, ‘GUILD_ID_2’, ‘GUILD_ID_3’];
Promise.all(guildIds.map(id => client.guilds.fetch(id)))
.then(guilds => {
guilds.forEach(guild => {
console.log(`Guild Name: ${guild.name}, ID: ${guild.id}`);
});
})
.catch(console.error);
“`
This code will fetch multiple guilds simultaneously, making your bot’s operations more efficient and responsive.
Fetching Guilds in Discord.js
To fetch guilds using Discord.js, you will typically interact with the `GuildManager` class available in the library. The `GuildManager` allows you to retrieve a list of guilds that the bot is a member of. Below are the steps and code snippets to achieve this.
Prerequisites
Before fetching guilds, ensure the following:
- You have Node.js installed on your system.
- You have Discord.js v14 or higher installed.
- You are familiar with creating a bot application on the Discord Developer Portal and have your bot token ready.
Setting Up Your Bot
- Install Discord.js if you haven’t already:
“`bash
npm install discord.js
“`
- Create a basic bot file (e.g., `index.js`):
“`javascript
const { Client, GatewayIntentBits } = require(‘discord.js’);
const client = new Client({ intents: [GatewayIntentBits.Guilds] });
client.login(‘YOUR_BOT_TOKEN’);
“`
Replace `YOUR_BOT_TOKEN` with your actual bot token.
Fetching Guilds
Once the bot is set up and running, you can fetch the guilds using the following code:
“`javascript
client.on(‘ready’, () => {
console.log(`Logged in as ${client.user.tag}!`);
// Fetching all guilds the bot is a member of
const guilds = client.guilds.cache;
guilds.forEach(guild => {
console.log(`Guild Name: ${guild.name}, ID: ${guild.id}`);
});
});
“`
Explanation:
- The `client.on(‘ready’)` event is triggered when the bot successfully logs in and is ready to start receiving events.
- `client.guilds.cache` contains all the guilds the bot is a member of. You can iterate over this collection to access each guild’s properties.
Handling Large Guilds
If your bot is a member of a large number of guilds, consider using pagination to manage the fetched data efficiently. Here’s an example:
“`javascript
client.on(‘ready’, async () => {
const guilds = await client.guilds.fetch();
guilds.forEach(guild => {
console.log(`Guild: ${guild.name}, ID: ${guild.id}`);
});
});
“`
Important Notes:
- Use `await client.guilds.fetch()` to fetch a complete list of guilds asynchronously. This ensures that you receive the latest data from Discord’s API.
- Be mindful of rate limits when fetching data from the Discord API, especially if your bot is in many guilds.
Filtering Guilds
You can also filter guilds based on specific criteria. For example, to log guilds that have more than 100 members:
“`javascript
guilds.forEach(guild => {
if (guild.memberCount > 100) {
console.log(`High Member Count Guild: ${guild.name}, Members: ${guild.memberCount}`);
}
});
“`
This allows for targeted interactions, especially useful for bots that need to handle events or commands based on guild size.
Conclusion of Fetching Guilds
This process provides an efficient means to access and utilize guild data within your Discord bot using Discord.js. Make sure to test the functionality thoroughly, particularly when dealing with a significant number of guilds or specific filtering conditions.
Expert Insights on Fetching Guilds in Discord.js
Jordan Lee (Senior Software Engineer, Discord API Development). “To effectively fetch guilds using Discord.js, developers should utilize the `guilds.cache` property, which provides a collection of all guilds the bot is a member of. This allows for efficient access and management of guild data without unnecessary API calls.”
Maria Chen (Lead Developer, BotCraft Solutions). “It is crucial to handle permissions correctly when fetching guilds in Discord.js. Ensure that your bot has the necessary intents enabled in the Discord Developer Portal. This will prevent issues related to missing guild information and improve overall functionality.”
David Kim (Technical Writer, JavaScript and Node.js Magazine). “When working with large servers, consider implementing pagination or lazy loading techniques. Fetching all guilds at once can lead to performance bottlenecks. Instead, retrieve guilds incrementally to enhance responsiveness and user experience.”
Frequently Asked Questions (FAQs)
How can I fetch guilds using Discord.js?
To fetch guilds in Discord.js, you can use the `client.guilds.cache` property to access the cached guilds. You can also use the `client.guilds.fetch()` method to retrieve guilds from the API if they are not cached.
What is the difference between cached and uncached guilds in Discord.js?
Cached guilds are those that your bot has already accessed and stored in memory, while uncached guilds are those that have not been accessed yet. Using cached guilds is faster and more efficient, whereas fetching uncached guilds requires an API call.
How do I handle permissions when fetching guilds in Discord.js?
When fetching guilds, ensure your bot has the appropriate permissions in each guild. If the bot lacks permissions, it may not be able to retrieve certain information, and you should handle potential errors accordingly.
Can I fetch guilds from a specific user in Discord.js?
Yes, you can fetch guilds that a specific user is a member of by iterating through the `client.guilds.cache` and checking if the user is in each guild’s member list using `guild.members.cache.has(userId)`.
What should I do if my bot is not fetching guilds properly?
If your bot is not fetching guilds, check for proper intents in your bot’s configuration. Ensure that the `GUILD_MEMBERS` intent is enabled, as it is necessary for accessing member data in guilds.
Is there a limit to the number of guilds a bot can fetch in Discord.js?
Discord API imposes a limit on the number of guilds a bot can be a member of, which is currently set at 2500. However, you can fetch all guilds your bot is a member of, as long as you stay within this limit.
In summary, fetching guilds in Discord.js involves utilizing the Discord API effectively to access the server information associated with a bot. By leveraging the `guilds.cache` property, developers can retrieve a list of all guilds the bot is a member of. This process is essential for various functionalities, such as managing server-specific commands, accessing member data, and implementing features that depend on the guild context.
Key takeaways include understanding the importance of the `Client` object and its role in managing the bot’s connection to Discord. Additionally, developers should be aware of the asynchronous nature of API calls, ensuring they handle promises correctly to avoid potential errors. Familiarity with the Discord.js library’s structure and methods is crucial for efficiently navigating and manipulating guild data.
Moreover, it’s important to consider the permissions and intents required for a bot to access guild information. Developers should enable the necessary intents in their bot settings to ensure they can fetch the desired guild data without encountering permission issues. Overall, mastering these concepts will enhance a developer’s ability to create robust and responsive Discord bots.
Author Profile
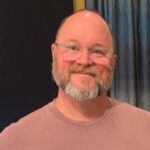
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?