How Can You Effectively Execute a Stored Procedure?
In the realm of database management, stored procedures stand out as powerful tools that streamline complex operations and enhance efficiency. Whether you’re a seasoned developer or a curious newcomer, understanding how to execute stored procedures can significantly elevate your data handling capabilities. These precompiled collections of SQL statements not only simplify repetitive tasks but also improve performance by reducing the need for multiple database calls. As we delve into the intricacies of executing stored procedures, you’ll discover how they can transform your approach to database interactions.
Executing a stored procedure may seem daunting at first, but it is a fundamental skill that can unlock a plethora of advantages. Stored procedures encapsulate business logic, allowing you to execute complex operations with a single command. This not only reduces the risk of errors but also enhances security by limiting direct access to the underlying data. In this article, we will explore the various methods to execute stored procedures across different database systems, shedding light on the syntax, parameters, and best practices that ensure optimal performance.
As we navigate through the nuances of executing stored procedures, you’ll gain insights into their practical applications and the scenarios in which they shine. From improving data integrity to facilitating batch processing, stored procedures are an essential component of modern database management. Prepare to embark on a journey that will equip you with the knowledge and skills to harness
Executing a Stored Procedure in SQL Server
To execute a stored procedure in SQL Server, you can use the `EXEC` or `EXECUTE` command, followed by the name of the stored procedure. If the stored procedure requires parameters, you must provide them in the correct order and type. Here’s the basic syntax for executing a stored procedure:
“`sql
EXEC procedure_name [parameter1, parameter2, …]
“`
If your stored procedure does not require parameters, you can simply use the following:
“`sql
EXEC procedure_name
“`
Example of Executing a Stored Procedure
Consider a stored procedure named `GetEmployeeDetails` that requires an employee ID as a parameter. The execution would look like this:
“`sql
EXEC GetEmployeeDetails @EmployeeID = 123;
“`
Using Output Parameters
Stored procedures can also return values via output parameters. To handle these, you must declare a variable to hold the output value. Here’s how you can execute a stored procedure with an output parameter:
“`sql
DECLARE @OutputValue INT;
EXEC GetEmployeeCount @DepartmentID = 1, @Count = @OutputValue OUTPUT;
SELECT @OutputValue AS EmployeeCount;
“`
Executing Stored Procedures in Other Database Systems
The syntax for executing stored procedures can vary across different database management systems. Below is a comparison of executing stored procedures in some popular systems:
Database System | Syntax |
---|---|
SQL Server | EXEC procedure_name [parameters] |
MySQL | CALL procedure_name([parameters]); |
Oracle | BEGIN procedure_name; END; |
PostgreSQL | SELECT procedure_name([parameters]); |
Considerations for Executing Stored Procedures
When executing stored procedures, keep the following considerations in mind:
- Permissions: Ensure that the user account executing the stored procedure has the necessary permissions.
- Transaction Handling: Depending on the logic within the procedure, you may need to manage transactions explicitly using `BEGIN TRANSACTION`, `COMMIT`, and `ROLLBACK`.
- Error Handling: Implement error handling within your stored procedures using `TRY…CATCH` blocks to gracefully manage exceptions.
By understanding these principles, you can effectively execute stored procedures and leverage their capabilities to optimize database operations.
Executing a Stored Procedure in SQL Server
To execute a stored procedure in SQL Server, you can use the `EXEC` command or the `EXECUTE` keyword. The general syntax is as follows:
“`sql
EXEC procedure_name [ @parameter1 = value1, @parameter2 = value2, … ]
“`
Example of Execution
If you have a stored procedure named `GetEmployeeDetails`, which accepts an employee ID as a parameter, you would execute it like this:
“`sql
EXEC GetEmployeeDetails @EmployeeID = 123;
“`
Using OUTPUT Parameters
Stored procedures can also return values through output parameters. To define an output parameter, you can use the `OUTPUT` keyword in the procedure definition. Here’s an example of how to execute such a procedure:
“`sql
DECLARE @TotalSalary INT;
EXEC GetTotalSalary @DepartmentID = 5, @TotalSalary = @TotalSalary OUTPUT;
SELECT @TotalSalary AS TotalSalary;
“`
Executing with Different Methods
Stored procedures can be executed using various methods, depending on the context:
- From SQL Server Management Studio (SSMS):
- Open a new query window and write the `EXEC` statement.
- Highlight the statement and click on the execute button.
- From an Application:
- Use ADO.NET, JDBC, or other database connectivity libraries to call the stored procedure.
- Example in Cwith ADO.NET:
“`csharp
using (SqlConnection connection = new SqlConnection(connectionString))
{
SqlCommand command = new SqlCommand(“GetEmployeeDetails”, connection);
command.CommandType = CommandType.StoredProcedure;
command.Parameters.AddWithValue(“@EmployeeID”, 123);
connection.Open();
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
// Process results
}
}
“`
Handling Errors
When executing stored procedures, it is vital to handle potential errors. Use `TRY…CATCH` blocks in T-SQL to manage exceptions effectively:
“`sql
BEGIN TRY
EXEC GetEmployeeDetails @EmployeeID = 123;
END TRY
BEGIN CATCH
SELECT ERROR_MESSAGE() AS ErrorMessage;
END CATCH;
“`
Transaction Control
When performing operations that modify data, consider wrapping the execution in a transaction to ensure data integrity:
“`sql
BEGIN TRANSACTION;
BEGIN TRY
EXEC UpdateEmployeeSalary @EmployeeID = 123, @NewSalary = 50000;
COMMIT TRANSACTION;
END TRY
BEGIN CATCH
ROLLBACK TRANSACTION;
SELECT ERROR_MESSAGE() AS ErrorMessage;
END CATCH;
“`
Performance Considerations
Optimizing the execution of stored procedures can lead to improved performance. Here are key factors to consider:
- Parameter Sniffing: SQL Server caches the execution plan based on the first set of parameters it encounters. To mitigate issues:
- Use `OPTION (RECOMPILE)` to force a new plan.
- Consider using `sp_executesql` for dynamic SQL execution.
- Execution Plans: Analyze execution plans using SQL Server Management Studio to identify bottlenecks.
- Statistics and Indexes: Ensure statistics are up-to-date and consider indexing the tables involved in the procedure for faster access.
Best Practices | Description |
---|---|
Use Appropriate Data Types | Match the parameter data types with the corresponding column types. |
Minimize the Use of Cursors | Opt for set-based operations instead of row-by-row processing. |
Avoid Using SELECT * | Specify only the columns needed to reduce data transfer and processing overhead. |
By following these guidelines, you can effectively execute stored procedures and optimize their performance within SQL Server environments.
Executing Stored Procedures: Perspectives from Database Experts
Maria Gonzalez (Senior Database Administrator, Tech Solutions Inc.). “To effectively execute a stored procedure, one must first ensure that the procedure is correctly defined in the database. Utilizing the EXEC command followed by the procedure name is the standard approach, but it is crucial to handle parameters appropriately to avoid runtime errors.”
James O’Connor (Lead Data Analyst, Data Insights Group). “When executing stored procedures, especially in a production environment, it is essential to monitor performance metrics. Using tools like SQL Server Profiler can help identify bottlenecks and optimize execution plans for better efficiency.”
Linda Chen (Database Architect, Innovative Tech Solutions). “Best practices for executing stored procedures include maintaining proper version control and documentation. This ensures that any changes made to the procedure are tracked, and the execution context is clear, which is vital for debugging and future modifications.”
Frequently Asked Questions (FAQs)
How do I execute a stored procedure in SQL Server?
To execute a stored procedure in SQL Server, use the `EXEC` command followed by the procedure name. For example: `EXEC dbo.YourStoredProcedureName;`. You can also pass parameters if required, like this: `EXEC dbo.YourStoredProcedureName @Parameter1 = Value1, @Parameter2 = Value2;`.
Can I execute a stored procedure from within another stored procedure?
Yes, you can execute a stored procedure from within another stored procedure using the same `EXEC` command. This allows for modular programming and code reuse.
What permissions are needed to execute a stored procedure?
To execute a stored procedure, a user must have the `EXECUTE` permission on that procedure. This permission can be granted by a database administrator or through a role that includes execute permissions.
How do I handle output parameters when executing a stored procedure?
To handle output parameters, declare a variable to store the output value, and specify the `OUTPUT` keyword in the procedure call. For example:
“`sql
DECLARE @OutputValue INT;
EXEC dbo.YourStoredProcedureName @InputParam = Value, @OutputParam = @OutputValue OUTPUT;
“`
What is the difference between `EXEC` and `EXECUTE` in SQL?
There is no difference between `EXEC` and `EXECUTE`; both commands can be used interchangeably to run stored procedures in SQL Server.
Can I execute a stored procedure in a different database?
Yes, you can execute a stored procedure in a different database by qualifying the procedure name with the database name and schema. For example: `EXEC DatabaseName.dbo.YourStoredProcedureName;`.
Executing a stored procedure is a fundamental task in database management that allows users to encapsulate complex SQL statements into a single callable routine. This process not only enhances code reusability but also improves performance by reducing the amount of information sent between the application and the database server. Understanding how to properly execute a stored procedure is essential for developers and database administrators alike, as it can significantly streamline database operations.
When executing a stored procedure, it is crucial to consider the specific syntax and requirements of the database management system in use, whether it be SQL Server, MySQL, Oracle, or another platform. Each system has its own conventions for calling stored procedures, including how to pass parameters and handle return values. Mastery of these details ensures that procedures run efficiently and produce the desired outcomes.
Moreover, leveraging stored procedures can lead to better security practices by limiting direct access to the underlying database tables. By encapsulating business logic within stored procedures, organizations can enforce stricter access controls and reduce the risk of SQL injection attacks. Overall, understanding how to execute stored procedures effectively is a vital skill that contributes to robust database management and application performance.
Author Profile
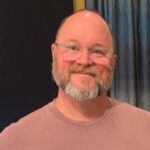
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?