How Can You Effectively End a Program in Python?
In the world of programming, knowing how to effectively manage the lifecycle of your applications is crucial. Whether you’re building a simple script or a complex software system, understanding how to end a program in Python can save you time, prevent errors, and enhance the overall user experience. This seemingly straightforward task can involve various considerations, from graceful exits to handling exceptions. In this article, we’ll explore the different methods and best practices for terminating a Python program, ensuring that you have the tools you need to control your code’s execution flow with confidence.
Ending a program in Python isn’t just about stopping the execution; it’s about doing so in a way that maintains data integrity and provides feedback to the user. There are several techniques available, each suited for different scenarios. For instance, you may encounter situations where a program needs to exit due to an error, or perhaps you want to end it based on user input. Understanding these scenarios can help you choose the most appropriate method for your specific needs.
Moreover, Python offers built-in functions and exception handling mechanisms that can facilitate a smooth termination process. By mastering these techniques, you can enhance your programming skills and ensure that your applications run efficiently. As we delve deeper into the various approaches to ending a Python program, you’ll discover not only the
Using the `exit()` Function
The `exit()` function is a built-in function in Python that allows you to terminate a program. It is often used in scripts where an immediate exit is necessary. This function is part of the `sys` module, so you need to import the module before using it.
Here’s how you can use it:
“`python
import sys
Some code
if condition:
sys.exit(“Exiting the program due to condition”)
“`
The `exit()` function can take an optional argument. If you provide a numeric argument, it will use that as the exit status code, where `0` typically indicates successful completion, and any non-zero value indicates an error.
Using the `quit()` Function
Similar to `exit()`, the `quit()` function can also be employed to terminate a running script. It is primarily intended for use in the interactive interpreter, but it can be used in scripts as well.
Example usage:
“`python
if some_error_condition:
quit(“Terminating the program due to an error.”)
“`
The `quit()` function works the same way as `exit()`, with the option to provide a status code.
Using the `os._exit()` Function
For situations where you want to exit the program immediately without calling cleanup handlers, you can use `os._exit()`. This function is more abrupt than `exit()` or `quit()`, and it terminates the program directly, bypassing the normal shutdown procedures.
Example:
“`python
import os
Some condition
if critical_error_occurred:
os._exit(1) Exiting with a status code indicating an error
“`
Using `break` and `return` Statements
In the context of loops or functions, you can use `break` and `return` statements to terminate execution.
- `break`: Exits the nearest enclosing loop.
- `return`: Exits the current function and optionally returns a value.
Example of `break`:
“`python
for i in range(10):
if i == 5:
break Exits the loop when i equals 5
“`
Example of `return`:
“`python
def my_function():
if some_condition:
return Exits the function
“`
Comparison Table of Exit Methods
Method | Module | Exit Type | Exit Code |
---|---|---|---|
exit() | sys | Normal | Customizable |
quit() | sys | Normal | Customizable |
os._exit() | os | Immediate | Customizable |
break | – | Loop | – |
return | – | Function | – |
Using the `exit()` Function
The `exit()` function from the `sys` module is a straightforward way to terminate a Python program. When invoked, it raises the `SystemExit` exception, which can be caught in outer levels of the program.
“`python
import sys
Terminate the program
sys.exit()
“`
This function can also take an optional argument that specifies the exit status:
- `sys.exit(0)` indicates a successful termination.
- `sys.exit(1)` or any non-zero value indicates an error.
Utilizing `quit()` and `exit()`
The `quit()` and `exit()` functions are built-in in Python and provide a user-friendly way to terminate a program, particularly in the interactive interpreter. Both functions effectively call `sys.exit()` under the hood.
“`python
Use quit() to exit the program
quit()
“`
- Note: While `quit()` and `exit()` are convenient for interactive sessions, they are not recommended for use in scripts meant for deployment.
Leveraging Keyboard Interrupts
You can also terminate a running Python program using keyboard interrupts. This is particularly useful during development or when a script is running in an interactive shell. Pressing `Ctrl + C` sends a `KeyboardInterrupt` signal to the program, causing it to stop execution.
“`python
try:
while True:
pass Simulate a long-running process
except KeyboardInterrupt:
print(“Program terminated by user.”)
“`
Returning from the Main Function
For scripts structured with a main function, returning from this function is another way to end execution. When the main function returns, the program will exit gracefully.
“`python
def main():
print(“Program is running…”)
return Ends the main function
if __name__ == “__main__”:
main()
“`
Using `os._exit()` for Immediate Exit
In certain cases, you may need to terminate a program immediately without cleanup. The `os._exit()` function can be used for this purpose. It exits the program immediately with the specified status code and does not call cleanup handlers.
“`python
import os
Immediate exit with status code
os._exit(0)
“`
- Caution: Using `os._exit()` should be reserved for extreme cases, as it bypasses the normal shutdown process.
Handling Exceptions for Clean Exits
It is often beneficial to handle exceptions to ensure that your program exits cleanly. Using a try-except block allows you to catch exceptions and perform necessary cleanup before exiting.
“`python
try:
Code that may raise exceptions
risky_operation()
except Exception as e:
print(f”An error occurred: {e}”)
finally:
print(“Cleaning up before exit.”)
sys.exit(1) Exit with error status
“`
Using `signal` Module for Graceful Termination
For more complex applications, especially those running on Unix-like systems, the `signal` module allows you to define handlers for termination signals. This provides a mechanism to clean up resources before exiting.
“`python
import signal
def signal_handler(sig, frame):
print(“Signal received, exiting gracefully.”)
sys.exit(0)
signal.signal(signal.SIGINT, signal_handler)
Long-running process
while True:
pass
“`
By implementing any of these methods, you can effectively manage the termination of your Python programs based on your specific needs and scenarios.
Expert Insights on Properly Ending Python Programs
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “To effectively end a Python program, one should utilize the `sys.exit()` function, which allows for a clean termination of the script and can also return a status code to the operating system. This is particularly useful for signaling success or failure to other processes.”
Michael Thompson (Lead Python Instructor, Code Academy). “Understanding the context of your program’s termination is crucial. For instance, using `return` statements in functions can help manage exits within specific scopes, while `break` can be used to exit loops gracefully. Each method serves a purpose based on the program’s structure.”
Sarah Lee (Software Architect, Tech Innovations Inc.). “In scenarios where you need to ensure that cleanup code is executed before a program ends, utilizing `try…finally` blocks is essential. This ensures that resources are released properly, preventing potential memory leaks or file corruption.”
Frequently Asked Questions (FAQs)
How can I end a Python program from within the code?
You can end a Python program by using the `sys.exit()` function from the `sys` module. Import the module with `import sys` and call `sys.exit()` to terminate the program.
What is the purpose of the `exit()` function in Python?
The `exit()` function is a built-in function that raises a `SystemExit` exception, allowing you to exit the program. It can be used in scripts and interactive sessions to stop execution.
Can I use a keyboard interrupt to stop a running Python program?
Yes, you can stop a running Python program by pressing `Ctrl + C` in the terminal or command prompt. This raises a `KeyboardInterrupt` exception, which terminates the program.
Is there a way to end a Python program based on a condition?
Yes, you can use conditional statements to check for specific criteria and call `sys.exit()` or `exit()` when the condition is met, effectively terminating the program.
What happens if I don’t handle exceptions in my Python program?
If exceptions are not handled, the program will terminate abruptly, displaying an error message. This can be avoided by using try-except blocks to manage exceptions gracefully.
Can I use a return statement to exit a Python script?
No, a return statement can only exit from a function. To exit a script entirely, you should use `sys.exit()` or `exit()`.
In summary, ending a program in Python can be accomplished through various methods, each suited to different scenarios. The most common approach is to use the built-in `exit()` or `sys.exit()` functions, which terminate the program gracefully. Additionally, the `break` statement can be employed to exit loops, while the `return` statement can be used to exit functions and return control to the caller. Understanding these methods allows developers to manage program flow effectively and ensure that resources are released appropriately.
Key takeaways include the importance of choosing the right method based on the context of the program. For instance, using `sys.exit()` is particularly useful when you need to terminate a program due to an error or a specific condition. In contrast, `break` and `return` are more appropriate for controlling flow within loops and functions, respectively. By mastering these techniques, developers can write cleaner, more efficient code that behaves as intended.
Ultimately, knowing how to end a program in Python is a fundamental skill that enhances a programmer’s ability to manage execution and improve user experience. Whether handling exceptions, controlling loops, or returning from functions, a solid grasp of these concepts is essential for effective programming in Python.
Author Profile
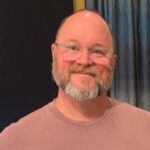
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?