How Do You Properly End a Function in Python?
How To End Function In Python
In the world of programming, functions are the building blocks that allow us to encapsulate logic and reuse code efficiently. Python, with its elegant syntax and dynamic capabilities, makes defining and using functions a breeze. However, understanding how to properly end a function is crucial for ensuring that your code runs smoothly and achieves the desired outcomes. Whether you’re a novice coder or a seasoned developer, mastering the nuances of function termination in Python can significantly enhance your coding proficiency.
At its core, ending a function in Python involves more than just using the return statement. It encompasses various aspects, including how to gracefully exit a function, handle exceptions, and manage the flow of control. The return statement plays a vital role in conveying results back to the caller, but there are other techniques and best practices that can help you write cleaner, more efficient code. Moreover, understanding the implications of function termination on variable scope and memory management is essential for writing robust applications.
As we delve deeper into the intricacies of ending functions in Python, we will explore the different methods available, common pitfalls to avoid, and how to leverage these techniques to write better code. By the end of this article, you’ll not only know how to end a function effectively but also appreciate the broader context of
Understanding Function Termination in Python
In Python, functions are defined using the `def` keyword, and they can be concluded using various statements depending on the desired outcome. The most common ways to end a function are through the use of the `return` statement, reaching the end of the function body, or by raising an exception.
Using the Return Statement
The `return` statement is the primary method to end a function and send a value back to the caller. When the `return` statement is executed, the function terminates immediately, and control is passed back to the point where the function was called. If no value is specified, `None` is returned by default.
- Example:
“`python
def add(a, b):
return a + b
“`
In the example above, the function `add` will terminate upon reaching the `return` statement, providing the sum of `a` and `b`.
Ending a Function Without Returning a Value
If a function reaches the end of its body without encountering a `return` statement, it will terminate and return `None`. This is useful when the function performs an action without needing to provide a result.
- Example:
“`python
def print_message():
print(“Hello, World!”)
“`
In this case, `print_message` concludes when the print statement has been executed. No value is returned, and Python defaults to returning `None`.
Raising Exceptions to End Functions
Functions can also be terminated by raising exceptions. When an exception is raised, the normal flow of execution is interrupted, and control is passed to the nearest exception handler. This is particularly useful for error handling.
- Example:
“`python
def divide(a, b):
if b == 0:
raise ValueError(“Cannot divide by zero.”)
return a / b
“`
In this example, if `b` is zero, the function will terminate and raise a `ValueError`, indicating that the operation cannot be performed.
Function Termination Behavior
To provide a clearer understanding of how functions can terminate, the following table summarizes the different methods of ending a function:
Method | Description | Return Value |
---|---|---|
Return Statement | Immediately ends the function and returns a value to the caller. | Specified value or None |
End of Function Body | Function ends naturally when the last line of code is executed. | None |
Raise Exception | Interrupts the normal flow and raises an error. | No return, control is passed to an exception handler |
Best Practices for Ending Functions
When defining functions, it is essential to consider how and when they will terminate. Here are some best practices:
- Always use `return` statements to provide clear outputs.
- Use exceptions to handle errors gracefully instead of terminating abruptly.
- Maintain readability by ensuring that function termination is straightforward and understandable.
By adhering to these practices, you can create functions that not only perform their intended tasks but also handle termination in a clean and predictable manner.
Understanding Function Termination in Python
In Python, functions are terminated using the `return` statement. This statement not only ends the function but also optionally sends a value back to the caller. When the `return` statement is executed, the control flow of the program returns to the point where the function was called. If no value is specified, the function returns `None` by default.
Using the `return` Statement
To end a function effectively, you can use the `return` statement as follows:
“`python
def add(a, b):
return a + b Function ends here and returns the sum
“`
Key Points about `return`:
- Single Use: A function can have multiple `return` statements, but once one is executed, the function exits immediately.
- Value Returning: You can return any type of data, including numbers, strings, lists, or even other functions.
- Implicit Return: If a function does not have a `return` statement, it will return `None` when called.
Examples of Function Termination
Here are several examples demonstrating how to terminate functions in different scenarios:
“`python
def multiply(a, b):
return a * b Ends the function and returns the product
def greet(name):
if name:
return f”Hello, {name}!” Returns a greeting
return “Hello, Guest!” Returns a default greeting
def check_even(num):
if num % 2 == 0:
return True Returns True for even numbers
return Returns for odd numbers
“`
Multiple Return Statements
Functions can contain multiple `return` statements based on conditions. This is often used in functions that need to evaluate different scenarios:
“`python
def classify_number(num):
if num > 0:
return “Positive”
elif num < 0:
return "Negative"
return "Zero" Ends the function for zero
```
Flow of Control:
- The control exits the function as soon as a `return` statement is executed.
- Subsequent code after a `return` statement within the same function is not executed.
Returning Multiple Values
Python allows returning multiple values as a tuple. Here’s how it works:
“`python
def min_max(numbers):
return min(numbers), max(numbers) Returns both min and max values
result = min_max([10, 20, 30])
print(result) Output: (10, 30)
“`
Best Practices for Function Termination
- Clear Return Statements: Always ensure that your return statements are clear and return meaningful values.
- Avoid Unreachable Code: Code placed after a return statement is unreachable and should be removed to maintain code clarity.
- Consistent Returns: Strive to return values consistently across different execution paths to avoid confusion.
Aspect | Description |
---|---|
Function End | Use `return` to exit the function. |
Implicit Return | Returns `None` if no return is specified. |
Multiple Return | Can have multiple return statements based on conditions. |
Returning Tuples | Multiple values can be returned as a tuple. |
Conclusion on Function Termination
Understanding how to effectively end functions using the `return` statement is crucial for controlling the flow of your Python programs. Utilizing return statements appropriately not only enhances code readability but also ensures that functions operate as intended.
Expert Insights on Ending Functions in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Ending a function in Python is straightforward, as Python automatically returns control to the caller once the function’s block is completed. However, it is crucial to understand the use of the return statement for returning values explicitly, which enhances code clarity and functionality.”
Michael Tran (Python Developer, CodeCraft Solutions). “In Python, functions can be ended using the return statement, which can also return a value. If no return statement is provided, the function will return None by default. This behavior is essential for developers to grasp, as it affects how data is handled in larger applications.”
Sarah Patel (Lead Instructor, Python Programming Academy). “Understanding how to properly end functions in Python is vital for effective programming. The return statement not only terminates the function but also allows for the passing of data back to the caller, which is fundamental for building modular and reusable code.”
Frequently Asked Questions (FAQs)
How do I end a function in Python?
To end a function in Python, you simply use the `return` statement. This statement can return a value to the caller and terminate the function’s execution. If no return statement is provided, the function will end when the last line of code is executed, returning `None` by default.
Can I end a function early in Python?
Yes, you can end a function early by using the `return` statement at any point within the function. This allows you to exit the function and return a value immediately, bypassing any subsequent code within that function.
What happens if I don’t use return in a Python function?
If you do not use a `return` statement in a Python function, the function will execute all its statements until completion and return `None` by default. This means that no value will be explicitly returned to the caller.
Is it possible to have multiple return statements in a Python function?
Yes, a Python function can have multiple `return` statements. The function will exit and return the value from the first `return` statement that is executed, allowing for conditional exits based on different scenarios.
Can a function in Python end without a return statement?
Yes, a function can end without a return statement. In such cases, the function will complete its execution and return `None` implicitly. This is common for functions that perform actions but do not need to send a value back to the caller.
What is the purpose of the return statement in Python?
The `return` statement in Python serves to exit a function and optionally pass back a value to the caller. It allows for the output of a function to be captured and used elsewhere in the code, facilitating modular and reusable programming.
In Python, functions are essential building blocks that allow for the organization and reuse of code. To properly conclude a function, the `return` statement is utilized, which not only exits the function but also optionally sends a value back to the caller. If a function reaches its end without encountering a `return` statement, it implicitly returns `None`. Understanding how to effectively end functions is crucial for maintaining clarity and control over the flow of a program.
Another important aspect to consider is the use of exception handling within functions. By incorporating `try` and `except` blocks, developers can manage errors gracefully, ensuring that functions terminate correctly even in the presence of unexpected inputs or conditions. This practice enhances the robustness of the code and prevents abrupt terminations that could lead to undesirable behavior in applications.
Moreover, the concept of scope plays a significant role in function termination. Local variables defined within a function cease to exist once the function has ended, which underscores the importance of returning values if they need to be accessed outside the function. This encapsulation not only aids in preventing variable name conflicts but also promotes cleaner, more maintainable code.
In summary, effectively ending a function in Python involves using the `return` statement, managing
Author Profile
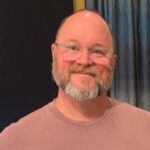
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?