How Can You Display a Word Document in HTML Using JavaScript?
In the digital age, the seamless integration of various file formats into web applications has become a necessity for enhancing user experience. Among these formats, Microsoft Word documents hold a significant place due to their widespread use in professional and educational settings. However, displaying Word documents directly within HTML pages can pose challenges for developers and content creators alike. If you’re looking to present your Word documents in a user-friendly manner on your website, this guide will walk you through the essential methods of achieving this using JavaScript.
Displaying Word documents in HTML is not just about converting files; it’s about ensuring that the content remains accessible and visually coherent. With the right techniques, you can embed Word documents that retain their formatting and are easy for users to interact with. This process often involves leveraging JavaScript libraries or APIs that facilitate the conversion and rendering of Word files directly within a web browser. By understanding the various approaches available, you can choose the one that best fits your project’s needs and technical requirements.
As we delve deeper into this topic, we will explore the different methods available for displaying Word documents in HTML, including the use of third-party libraries, conversion tools, and iframe embedding. Whether you’re a seasoned developer or a novice looking to enhance your website’s functionality, this article will equip you with the knowledge to effectively integrate
Using an Iframe to Display Word Documents
One of the simplest methods to display a Word document in HTML using JavaScript is through the use of an iframe. This technique allows you to embed the document directly into the webpage, making it viewable without requiring the user to download it. However, the compatibility of this method largely depends on the browser and the document format.
To implement this, follow these steps:
- Ensure the Word document is hosted on a server or accessible via a URL.
- Use the following HTML structure to create an iframe:
“`html
“`
Replace `URL_TO_YOUR_DOCUMENT` with the actual link to your Word file.
Converting Word Documents to HTML
Another effective approach is to convert the Word document into HTML format. This can be done using various libraries or online services that provide conversion capabilities. Once converted, the HTML content can be easily integrated into your webpage.
- Libraries for Conversion:
- Mammoth.js: A JavaScript library for converting .docx documents to HTML.
- docx.js: A library that allows reading and creating .docx files in JavaScript.
To utilize Mammoth.js, you can include it in your project as follows:
“`html
“`
After including the library, you can use the following JavaScript code snippet to convert and display the document:
“`javascript
var inputFile = document.getElementById(“uploadFile”);
inputFile.addEventListener(“change”, function(event) {
var reader = new FileReader();
reader.onload = function(event) {
mammoth.convertToHtml({arrayBuffer: event.target.result})
.then(displayResult)
.catch(handleError);
};
reader.readAsArrayBuffer(inputFile.files[0]);
});
function displayResult(result) {
document.getElementById(“output”).innerHTML = result.value; // The generated HTML
}
function handleError(err) {
console.log(err);
}
“`
This code reads the uploaded Word file and converts it to HTML, which is then displayed in an element with the ID `output`.
Embedding Using Google Docs Viewer
If you prefer not to host the document, using Google Docs Viewer can be an excellent alternative. This method allows you to embed the document directly from Google Drive.
To use Google Docs Viewer:
- Upload your document to Google Drive.
- Get the shareable link and modify it to be compatible with Google Docs Viewer.
Example format:
“`html
“`
Replace `URL_TO_YOUR_DOCUMENT` with the shareable link to your file.
Considerations When Displaying Word Documents
When selecting the method to display Word documents, consider the following factors:
Method | Compatibility | Ease of Use |
---|---|---|
Iframe | Depends on the browser | Simple implementation |
HTML Conversion | Browser-independent | Requires additional libraries |
Google Docs Viewer | Widely compatible | Easy for users |
Each method has its own strengths and weaknesses, and the choice may depend on your specific requirements and audience preferences.
Methods to Display a Word Document in HTML
Displaying a Word document in HTML using JavaScript can be achieved through various approaches. Here are some effective methods:
Using an iframe
One straightforward way to embed a Word document in a web page is by using an `
“`html
“`
- Pros: Easy to implement; no need for additional libraries.
- Cons: Limited customization and relies on the document being accessible via a URL.
Converting to HTML Format
Another approach is to convert the Word document into HTML format before displaying it. This can be done using libraries or online services.
- Convert the document to HTML using a tool like Pandoc or an online converter.
- Embed the converted HTML in your web page.
“`html
“`
- Pros: Full control over styling and content.
- Cons: Requires conversion step; formatting may not always be perfect.
Using JavaScript Libraries
Several JavaScript libraries facilitate displaying Word documents in web applications. Notable options include:
- Mammoth.js
- Converts .docx documents to HTML.
- Retains formatting and styles where possible.
“`javascript
// Example using Mammoth.js
mammoth.convertToHtml({arrayBuffer: fileBuffer})
.then(displayResult)
.catch(handleError);
function displayResult(result) {
document.getElementById(‘word-content’).innerHTML = result.value;
}
“`
- Pros: Maintains much of the original formatting and structure.
- Cons: Dependency on third-party libraries.
Using Microsoft Office Online
If the document is hosted on OneDrive or SharePoint, you can embed it using Microsoft Office Online. This provides an interactive viewer.
“`html
“`
- Pros: Interactive and maintains original formatting; easy to use.
- Cons: Requires the document to be hosted on Microsoft services.
Security Considerations
When implementing these methods, consider the following:
- Ensure that documents do not contain sensitive information.
- Use HTTPS to load documents securely.
- Be cautious of Cross-Origin Resource Sharing (CORS) issues when loading external documents.
Browser Compatibility
Different browsers may handle embedded documents differently. Ensure thorough testing across:
Browser | Compatibility Level |
---|---|
Chrome | High |
Firefox | High |
Safari | Medium |
Internet Explorer | Low |
Utilizing these methods effectively can enhance your web application’s functionality by allowing users to interact with Word documents seamlessly.
Expert Insights on Displaying Word Documents in HTML with JavaScript
Dr. Emily Chen (Senior Software Engineer, WebTech Innovations). “To effectively display Word documents in HTML using JavaScript, one must consider utilizing libraries such as Mammoth.js, which convert .docx files into HTML format seamlessly. This approach preserves the document’s structure while ensuring compatibility across various web platforms.”
Michael Thompson (Lead Front-End Developer, Digital Solutions Group). “Incorporating JavaScript to display Word documents can enhance user experience significantly. I recommend using a combination of FileReader API and HTML5 to read the document content and render it dynamically on the page, allowing for real-time interaction with the document.”
Sarah Patel (Web Development Consultant, TechSavvy Advisors). “When displaying Word documents in HTML, it is crucial to focus on accessibility and responsiveness. Using frameworks like React or Angular can streamline the process, as they provide robust tools for managing document rendering and ensuring that the content is accessible across devices.”
Frequently Asked Questions (FAQs)
How can I display a Word document in HTML using JavaScript?
To display a Word document in HTML using JavaScript, you can use libraries such as `Mammoth.js` to convert the document to HTML. Load the Word file, read its contents, and then render it in a designated HTML element.
What libraries are available for converting Word documents to HTML?
Several libraries can convert Word documents to HTML, including `Mammoth.js`, `docx.js`, and `PizZip`. Each library has unique features and capabilities, so choose one based on your specific needs.
Can I directly embed a Word document in an HTML page?
Direct embedding of a Word document in an HTML page is not supported. Instead, you can convert the document to HTML format or use an iframe to display it if hosted online.
What are the limitations of displaying Word documents in HTML?
Limitations include potential loss of formatting, unsupported features (like macros), and compatibility issues across different browsers. The conversion process may not perfectly replicate the original document’s layout.
Is it necessary to use a server to display Word documents in HTML?
It is not strictly necessary to use a server; however, if the Word document is large or requires processing, hosting it on a server can enhance performance and allow for easier access.
How do I handle user uploads of Word documents for display in HTML?
You can use an HTML file input element to allow users to upload Word documents. Once uploaded, use JavaScript to read the file and convert it to HTML for display on the page.
In summary, displaying a Word document in HTML using JavaScript involves several key techniques and considerations. The primary methods include converting the Word document into a web-friendly format, such as HTML or PDF, and then utilizing JavaScript to embed or render this content on a webpage. This process often requires the use of libraries or APIs that facilitate the conversion and display of the document, ensuring compatibility across different browsers and devices.
One significant insight is the importance of choosing the right library or tool for the task. Options like `Mammoth.js`, `docx.js`, and various PDF libraries can streamline the conversion process and enhance the user experience. Additionally, understanding the limitations of each method, such as potential formatting issues or the need for additional resources, is crucial for effective implementation.
Moreover, it is essential to consider user accessibility and performance when displaying documents. Ensuring that the content is easily navigable and loads efficiently can significantly improve user engagement. By following best practices in web development and keeping the end-user experience in mind, developers can successfully integrate Word documents into their web applications using JavaScript.
Author Profile
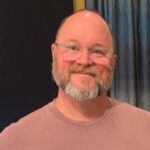
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?