How Can You Effectively Delay Execution in Python?
In the world of programming, timing can be everything. Whether you’re building a game that requires a pause between actions, developing a web scraper that needs to avoid hitting a server too quickly, or creating a user interface that feels smooth and responsive, knowing how to effectively implement delays in Python is a crucial skill. Python, with its straightforward syntax and powerful libraries, offers several methods to introduce delays in your code, allowing you to control the flow of execution with ease. This article will guide you through the various techniques for implementing delays in Python, ensuring that your programs run exactly as you intend.
When it comes to delaying execution in Python, there are multiple approaches to consider, each suited for different scenarios. The most common method involves using the built-in `time` module, which provides a simple way to pause your program for a specified duration. However, for more complex applications, such as those involving asynchronous programming or GUI development, you may need to explore additional libraries and techniques that can handle delays without freezing your application.
Understanding how to implement delays not only enhances the functionality of your programs but also improves user experience. By mastering these techniques, you can create applications that are not only efficient but also intuitive and engaging. In the following sections, we will delve deeper into
Using the time Module
The simplest way to introduce delays in a Python program is by utilizing the built-in `time` module. This module provides various time-related functions, including `sleep()`, which is specifically designed to pause the execution of a program for a specified number of seconds.
To implement a delay using the `time.sleep()` function, the syntax is straightforward:
“`python
import time
time.sleep(seconds)
“`
Where `seconds` can be a floating-point number to indicate fractions of a second. For example:
“`python
import time
print(“Wait for 2 seconds…”)
time.sleep(2)
print(“2 seconds have passed.”)
“`
This code snippet pauses the program for 2 seconds before continuing.
Using the threading Module
For more complex applications, particularly those involving concurrency, the `threading` module can be employed. This module allows you to create threads that can run concurrently with other parts of the program. The `Event` class from the `threading` module can be used to create delays without blocking the main thread.
Here’s an example demonstrating the use of `threading` with a delay:
“`python
import threading
def delayed_function():
print(“This function runs after a delay.”)
Create a timer that runs the function after 3 seconds
timer = threading.Timer(3.0, delayed_function)
timer.start()
“`
In this example, the `delayed_function` will execute after a 3-second delay without freezing the main program.
Using asyncio for Asynchronous Delays
When working with asynchronous programming in Python, the `asyncio` module provides a robust framework for managing delays. It allows for non-blocking delays in a way that is suitable for I/O-bound tasks. The `await asyncio.sleep()` function can be used to pause execution within an asynchronous function.
Here’s an example:
“`python
import asyncio
async def main():
print(“Waiting for 1 second…”)
await asyncio.sleep(1)
print(“1 second has passed.”)
asyncio.run(main())
“`
In this snippet, `asyncio.sleep()` is used to create a non-blocking delay, allowing other tasks to run concurrently during the wait time.
Comparison of Delay Methods
The following table summarizes the key differences among the methods discussed for introducing delays in Python:
Method | Blocking | Use Case |
---|---|---|
time.sleep() | Yes | Simple scripts, linear execution |
threading.Timer | No | Concurrent execution, non-blocking delays |
asyncio.sleep() | No | Asynchronous tasks, I/O-bound operations |
Each method has its specific scenarios where it is most effective, depending on the requirements for blocking behavior and concurrency. When choosing a method, consider the nature of the tasks your program will be performing and how they interact during execution.
Using the time Module
The `time` module in Python provides a straightforward method to introduce delays in your code. The `time.sleep(seconds)` function pauses the execution of the program for a specified number of seconds.
Example usage:
“`python
import time
print(“Start”)
time.sleep(2) Delays for 2 seconds
print(“End”)
“`
Key points about `time.sleep()`:
- Accepts a float value, allowing for delays in fractions of a second.
- It can be used in both scripts and interactive sessions.
- Useful for creating timed pauses in loops or between operations.
Using the threading Module
For more complex applications, especially in multi-threaded environments, the `threading` module can be utilized. The `Thread` class allows you to run functions in a separate thread, which can include delays.
Example usage:
“`python
import threading
def delayed_function():
print(“This runs after a delay”)
Create a thread that runs the delayed_function after a 2-second delay
thread = threading.Timer(2.0, delayed_function)
thread.start()
“`
Considerations when using `threading.Timer`:
- The delay is non-blocking; the main program continues while the timer runs.
- You can cancel the timer if needed using `thread.cancel()`.
Using the asyncio Module
For asynchronous programming, Python’s `asyncio` module provides an efficient way to introduce delays. The `asyncio.sleep(seconds)` function can be used within asynchronous functions to pause execution without blocking the entire program.
Example usage:
“`python
import asyncio
async def main():
print(“Start”)
await asyncio.sleep(2) Delays for 2 seconds
print(“End”)
asyncio.run(main())
“`
Benefits of `asyncio.sleep()`:
- Allows other tasks to run concurrently during the delay.
- Best suited for applications involving I/O operations or network requests.
Using a Custom Delay Function
Creating a custom delay function can enhance flexibility, especially if you require specific logging or additional functionality during the delay.
Example:
“`python
def custom_delay(seconds):
print(f”Delaying for {seconds} seconds…”)
time.sleep(seconds)
print(“Delay complete.”)
custom_delay(3)
“`
This function can be modified to include:
- Progress indicators.
- Logging messages.
- Conditional delays based on program states.
Comparative Overview of Delay Methods
Method | Blocking | Asynchronous | Use Case |
---|---|---|---|
`time.sleep()` | Yes | No | Simple scripts and operations |
`threading.Timer` | No | No | Running functions in the background |
`asyncio.sleep()` | No | Yes | I/O-bound and concurrent tasks |
Custom function | Depends on implementation | Depends on implementation | Flexible and tailored delays |
Understanding the context and requirements of your Python application will determine which delay method is most suitable for your needs.
Expert Insights on Delaying Execution in Python
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “In Python, the most straightforward way to introduce a delay in execution is by utilizing the `time.sleep()` function. This method is particularly effective for simple scripts where precise timing is not critical. However, developers should be cautious as it blocks the entire thread, which may not be ideal for applications requiring responsiveness.”
Mark Thompson (Lead Developer, Python Development Group). “For asynchronous applications, I recommend using `asyncio.sleep()`. This function allows for non-blocking delays, enabling other tasks to run concurrently. It is essential for maintaining responsiveness in applications that rely on I/O operations or user interactions while waiting.”
Sarah Patel (Python Instructor, Code Academy). “When teaching beginners about delaying execution, I emphasize the importance of understanding the context in which a delay is applied. Whether using `time.sleep()` for simple scripts or `asyncio.sleep()` for more complex asynchronous programming, knowing when and how to implement these functions can greatly enhance the efficiency and performance of Python applications.”
Frequently Asked Questions (FAQs)
How can I introduce a delay in a Python program?
You can introduce a delay in a Python program using the `time.sleep(seconds)` function from the `time` module, where `seconds` is the duration of the delay in seconds.
What is the purpose of using time.sleep() in Python?
The `time.sleep()` function is used to pause the execution of a program for a specified amount of time, which can be useful for creating delays between actions, managing timing in loops, or throttling requests to external services.
Can I use time.sleep() with fractions of a second?
Yes, `time.sleep()` accepts floating-point numbers, allowing you to specify delays in fractions of a second, such as `time.sleep(0.5)` for a half-second delay.
Are there alternatives to time.sleep() for creating delays in Python?
Yes, alternatives include using asynchronous programming with `asyncio.sleep()` for non-blocking delays or implementing timers with threading for more complex timing needs.
Does time.sleep() block the entire program?
Yes, `time.sleep()` blocks the execution of the current thread, meaning no other code in that thread will run until the sleep duration has elapsed. For non-blocking delays, consider using asynchronous methods.
How can I create a countdown timer in Python?
You can create a countdown timer by using a loop that decrements a value and calls `time.sleep()` for each iteration to create the desired delay between updates.
In Python, delaying execution can be achieved through various methods, with the most common being the use of the `time` module. The `time.sleep(seconds)` function allows developers to pause the execution of a program for a specified number of seconds. This is particularly useful in scenarios such as rate limiting, managing API requests, or simply adding a pause in a user interface to enhance user experience. Additionally, other libraries like `asyncio` offer more advanced techniques for creating delays in asynchronous programming environments.
Another important aspect to consider is the context in which delays are implemented. For instance, in GUI applications, using `time.sleep()` can freeze the interface, leading to a poor user experience. Instead, using threading or asynchronous programming can provide a non-blocking way to introduce delays. Understanding the implications of blocking versus non-blocking operations is crucial for writing efficient and responsive applications.
In summary, the ability to introduce delays in Python is a fundamental skill that can enhance the functionality of applications. By leveraging the appropriate methods and understanding the context of their use, developers can create more effective and user-friendly programs. The choice of delay mechanism should align with the specific requirements of the application to ensure optimal performance and user satisfaction.
Author Profile
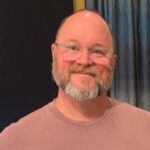
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?