How Can You Decompile a Compiled Python File Effectively?
In the world of programming, Python stands out as a versatile and powerful language, widely used for everything from web development to data analysis. However, when it comes to sharing or distributing Python applications, developers often compile their code into bytecode files, typically with a `.pyc` extension. While this process enhances performance and provides a layer of obfuscation, it can also lead to challenges for those who need to access the original source code. Whether you’re a developer looking to recover lost code, a security researcher analyzing software, or simply a curious programmer, understanding how to decompile a compiled Python file can be an invaluable skill.
Decompiling a Python file involves reversing the compilation process, allowing you to extract human-readable code from the bytecode. This process can be essential for various reasons, such as debugging, understanding third-party libraries, or even recovering code that was accidentally lost. However, it’s important to recognize that decompiling code raises ethical and legal considerations, particularly regarding intellectual property rights. As you delve into the methods and tools available for decompiling Python files, you’ll gain insights into both the technical aspects and the broader implications of this practice.
In this article, we will explore the various techniques and tools used to decompile Python bytecode
Understanding Python Bytecode
Python files with a `.pyc` extension are compiled bytecode files generated by the Python interpreter. These files contain a lower-level representation of the source code, which is executed by the Python virtual machine. Understanding the structure of bytecode is essential for decompilation, as it allows you to trace back to the original source code.
The bytecode consists of several components:
- Magic Number: Identifies the version of Python that created the file.
- Timestamp: Indicates when the bytecode was compiled.
- Code Objects: Contains the actual bytecode instructions.
- Constants: A list of constants used in the code, such as strings and numbers.
- Names and Variables: References to variable names used in the original source code.
Tools for Decompiling Python Files
Various tools are available for decompiling Python bytecode into readable source code. Some of the most popular tools include:
- uncompyle6: A widely used decompiler that supports several Python versions.
- pycdc: A Python bytecode decompiler that works with Python 2 and 3.
- decompyle3: Specifically designed for Python 3.7 and later versions.
Each of these tools has its unique features and capabilities. Below is a comparison table highlighting their main attributes:
Tool | Supported Versions | Output Format | Installation |
---|---|---|---|
uncompyle6 | Python 2.7, 3.2-3.11 | Python Source Code | pip install uncompyle6 |
pycdc | Python 2, 3.0-3.9 | Python Source Code | pip install pycdc |
decompyle3 | Python 3.7+ | Python Source Code | pip install decompyle3 |
Step-by-Step Decompilation Process
To decompile a compiled Python file, follow these steps:
- **Install the Decompiler**: Choose a decompiler that fits your needs and install it using pip or the appropriate method.
- **Locate the Compiled File**: Ensure you have the `.pyc` or `.pyo` file you wish to decompile.
- **Run the Decompiler**: Use the command line to execute the decompiler with the target file as an argument. For example, using `uncompyle6`:
“`bash
uncompyle6 myfile.pyc > myfile.py
“`
- Review the Output: Open the resulting Python file to review and edit the decompiled code as necessary.
Best Practices and Legal Considerations
When decompiling Python files, it is essential to adhere to ethical and legal guidelines. Here are some best practices:
- Obtain Permission: Always ensure you have the necessary permissions to decompile the code. Decompiling proprietary software without consent may violate copyright laws.
- Use for Educational Purposes: If you are decompiling for educational purposes, such as understanding a library or framework, ensure you are compliant with its licensing.
- Respect Privacy: Do not decompile files that may contain sensitive or personal information.
By adhering to these guidelines, you can responsibly engage in the practice of decompiling Python files.
Understanding the Python Compilation Process
Python files are typically compiled into bytecode, which is a lower-level representation of the source code that the Python interpreter can execute. This compilation happens automatically when you run a Python program, resulting in files with the `.pyc` extension stored in the `__pycache__` directory.
- Source Code: The original Python code, `.py` files.
- Bytecode: Compiled code, `.pyc` files, stored in the `__pycache__`.
- Execution: The Python interpreter runs the bytecode on the Python Virtual Machine (PVM).
Tools Required for Decompilation
To decompile a compiled Python file effectively, you will need specific tools designed for this purpose. Here are some popular options:
Tool Name | Description | Compatibility |
---|---|---|
`uncompyle6` | Decompiles Python 2.7 and 3.x bytecode. | Python 2.7, 3.x |
`decompyle3` | Specifically for Python 3.x files. | Python 3.x |
`pycdc` | A command-line decompiler for Python bytecode. | Python 2.7, 3.x |
`pyinstxtractor` | Extracts Python files packaged with PyInstaller. | Python 2.7, 3.x |
Step-by-Step Guide to Decompiling
Follow these steps to decompile a Python compiled file (.pyc):
- Install a Decompiler Tool: Choose one of the tools listed above and install it. For example, to install `uncompyle6`, run:
“`bash
pip install uncompyle6
“`
- Locate the Compiled File: Identify the `.pyc` file you want to decompile. You can typically find it in the `__pycache__` directory.
- Run the Decompiler: Use the command line to run the decompiler tool. Here’s an example using `uncompyle6`:
“`bash
uncompyle6 -o . __pycache__/your_file.cpython-38.pyc
“`
- Review the Output: The decompiled code will be printed to the terminal or saved to the specified directory. Check the output for readability and correctness.
Common Issues and Troubleshooting
While decompiling, you might encounter some issues. Here are common problems and solutions:
- Incompatible Python Version: Ensure that the decompiler tool matches the Python version used to compile the bytecode.
- Obfuscated Bytecode: If the bytecode has been obfuscated or optimized, decompilation may yield incomplete or unreadable code.
- Missing Dependencies: The original code may rely on external libraries or modules that need to be installed separately.
Legal Considerations
Decompiling software can raise legal and ethical concerns. Consider the following points:
- Copyright Issues: Ensure that you have the right to decompile the software. Unauthorized decompilation can violate copyright laws.
- License Agreements: Review the software’s license agreement for any clauses related to reverse engineering.
- Intellectual Property: Respect the intellectual property rights of the original authors and developers.
Expert Insights on Decompiling Compiled Python Files
Dr. Emily Carter (Senior Software Engineer, CodeSecure Labs). Decompiling a compiled Python file, typically a .pyc or .pyo file, requires understanding the bytecode generated by the Python interpreter. Tools like `uncompyle6` and `decompyle3` are essential for this task, as they can translate bytecode back into readable Python source code, allowing developers to analyze or recover lost scripts.
Marcus Liu (Cybersecurity Consultant, Digital Defense Group). It is crucial to consider the legal and ethical implications when decompiling Python files. While it can be a useful technique for debugging or recovering lost code, unauthorized decompilation could violate software licenses or copyright laws. Always ensure you have permission before proceeding.
Sarah Thompson (Open Source Advocate, Python Community Forum). The Python community has developed various tools and libraries to facilitate decompilation, but users should be aware of the limitations. The decompiled code may not perfectly match the original source due to optimizations and transformations done during compilation. Therefore, it is advisable to use decompilation as a last resort when source code recovery is necessary.
Frequently Asked Questions (FAQs)
What is a compiled Python file?
A compiled Python file is typically a `.pyc` or `.pyo` file, which contains bytecode generated from the original Python source code. This bytecode is optimized for execution by the Python interpreter.
Why would someone want to decompile a compiled Python file?
Decompiling a compiled Python file allows developers to recover the original source code for purposes such as debugging, understanding third-party code, or recovering lost scripts.
What tools are available for decompiling Python files?
Several tools can be used for decompiling Python files, including `uncompyle6`, `decompyle3`, and `pycdc`. These tools can convert bytecode back into readable Python source code.
Is it legal to decompile a compiled Python file?
The legality of decompiling a compiled Python file depends on the copyright status of the code and the intent behind decompilation. Always ensure compliance with applicable laws and respect software licenses.
What are the limitations of decompiling Python files?
Decompiled code may not perfectly match the original source code, especially in terms of comments and variable names. Additionally, certain optimizations in the compilation process can lead to loss of information.
How can I decompile a .pyc file using uncompyle6?
To decompile a `.pyc` file using `uncompyle6`, install the tool via pip, then run the command `uncompyle6
Decompiling a compiled Python file, typically in the form of a .pyc or .pyo file, involves converting the bytecode back into a more human-readable format. This process is essential for developers who may need to recover lost source code or analyze the behavior of a compiled program. The primary tool for this task is the `uncompyle6` package, which is specifically designed to handle Python bytecode from versions 2.7 to 3.7. Additionally, understanding the structure of Python bytecode and the differences between various Python versions can significantly aid in the decompilation process.
It is crucial to note that while decompiling can be a useful technique, it raises ethical and legal considerations. Users should ensure they have the right to decompile the code, as doing so without permission may violate copyright laws or licensing agreements. Furthermore, decompiled code may not perfectly replicate the original source code, as comments and certain formatting details are often lost during compilation.
In summary, decompiling Python files can be a valuable skill for developers facing challenges with compiled code. By leveraging tools like `uncompyle6`, understanding the bytecode structure, and being mindful of legal implications, developers can effectively navigate the
Author Profile
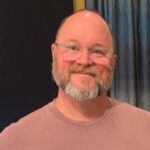
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?