How Can You Declare a Variable in an SQL Query?
In the world of databases and data manipulation, SQL (Structured Query Language) stands as a powerful tool that enables users to interact with their data efficiently. Whether you’re a seasoned developer or a curious beginner, mastering the art of SQL queries is essential for effective data management. One of the foundational aspects of writing dynamic and flexible SQL queries is the ability to declare variables. This seemingly simple technique can unlock a new level of functionality in your database interactions, allowing you to store temporary values, enhance readability, and streamline complex operations.
Declaring variables in SQL queries is a crucial skill that empowers you to create more sophisticated scripts and procedures. By using variables, you can hold data temporarily, making it easier to manipulate and reference throughout your SQL statements. This not only enhances the clarity of your code but also allows for more efficient execution, especially when dealing with repetitive tasks or complex calculations.
In this article, we will delve into the various methods and best practices for declaring variables in SQL queries. We will explore how different SQL dialects, such as T-SQL for Microsoft SQL Server and PL/SQL for Oracle, handle variable declaration, giving you a comprehensive understanding of how to leverage this feature effectively. Whether you’re looking to improve your query performance or simply want to write cleaner code, understanding how
Declaring Variables in SQL
Declaring variables in SQL is a fundamental aspect of programming within database management systems. Variables are used to store temporary data that can be referenced and manipulated during the execution of SQL queries.
To declare a variable in SQL, you typically use the `DECLARE` statement followed by the variable name and its data type. This allows you to specify the kind of data the variable will hold, ensuring type safety and preventing errors during execution.
Syntax for Declaring Variables
The general syntax for declaring a variable in SQL is as follows:
“`sql
DECLARE @VariableName DataType;
“`
For example:
“`sql
DECLARE @EmployeeCount INT;
“`
In this case, `@EmployeeCount` is a variable of type `INT`, which can hold integer values.
Assigning Values to Variables
After declaring a variable, you can assign a value to it using the `SET` statement or during the declaration itself. Here’s how you can do it:
- Using the `SET` statement:
“`sql
SET @EmployeeCount = 10;
“`
- Assigning a value at declaration:
“`sql
DECLARE @EmployeeCount INT = 10;
“`
This assignment allows you to initialize the variable with a value immediately upon declaration.
Using Variables in SQL Queries
Once a variable is declared and assigned a value, it can be used in SQL queries. This is particularly useful for dynamic queries, calculations, and conditional logic.
Here’s an example of using a variable in a query:
“`sql
DECLARE @DepartmentId INT;
SET @DepartmentId = 5;
SELECT * FROM Employees WHERE DepartmentId = @DepartmentId;
“`
In this case, the variable `@DepartmentId` is used to filter results from the `Employees` table.
Table of Common Data Types
Understanding the appropriate data types is critical for effective variable declaration. Below is a table summarizing common SQL data types used for declaring variables:
Data Type | Description |
---|---|
INT | Integer value, typically used for whole numbers. |
VARCHAR(n) | Variable-length string with a maximum length of n characters. |
DATETIME | Stores date and time values. |
DECIMAL(p,s) | Fixed-point number with precision p and scale s. |
BIT | Stores a boolean value (0 or 1). |
Best Practices for Variable Declaration
When declaring variables, consider the following best practices:
- Use meaningful names: Choose variable names that reflect their purpose, making code easier to understand.
- Limit scope: Declare variables in the smallest scope possible to avoid conflicts and maintain clarity.
- Initialize variables: Always initialize your variables to avoid unexpected behavior due to null values.
- Use appropriate data types: Select data types that suit the data being stored, optimizing performance and storage.
By following these guidelines, you can effectively manage variables within your SQL queries, enhancing the clarity and maintainability of your code.
Declaring Variables in SQL
In SQL, declaring variables allows for the storage of temporary data that can be used in queries. The syntax for declaring variables varies slightly among different SQL dialects, such as T-SQL (used in Microsoft SQL Server) and PL/SQL (used in Oracle). Below are the common methods for declaring variables in these environments.
Declaring Variables in T-SQL
In T-SQL, variables are declared using the `DECLARE` statement. The basic syntax is as follows:
“`sql
DECLARE @VariableName DataType;
“`
- @VariableName: The name of the variable, prefixed with an `@`.
- DataType: The data type of the variable (e.g., `INT`, `VARCHAR`, `DATETIME`).
Example:
“`sql
DECLARE @EmployeeCount INT;
SET @EmployeeCount = 10;
“`
Multiple variables can be declared in a single statement:
“`sql
DECLARE @StartDate DATETIME, @EndDate DATETIME;
SET @StartDate = ‘2023-01-01’;
SET @EndDate = ‘2023-12-31’;
“`
Using Variables in T-SQL
Once declared, variables can be used in SQL statements:
“`sql
SELECT * FROM Employees WHERE HireDate BETWEEN @StartDate AND @EndDate;
“`
Declaring Variables in PL/SQL
In PL/SQL, variable declaration occurs within a block structure. The syntax includes a `DECLARE` section, followed by the executable section. Here’s how to do it:
“`sql
DECLARE
VariableName DataType;
BEGIN
— Executable statements
END;
“`
Example:
“`sql
DECLARE
v_EmployeeCount NUMBER;
BEGIN
v_EmployeeCount := 10;
DBMS_OUTPUT.PUT_LINE(‘Employee Count: ‘ || v_EmployeeCount);
END;
“`
Using Variables in PL/SQL
Variables in PL/SQL can be used to store results or control flow:
“`sql
DECLARE
v_TotalSales NUMBER;
BEGIN
SELECT SUM(SalesAmount) INTO v_TotalSales FROM Sales WHERE SaleDate = SYSDATE;
DBMS_OUTPUT.PUT_LINE(‘Total Sales Today: ‘ || v_TotalSales);
END;
“`
Variable Scope
The scope of declared variables can vary:
- Local Variables: Declared within a block, accessible only within that block.
- Global Variables: May be declared in a broader scope, accessible across various sessions or procedures, depending on the database system.
Best Practices
- Use meaningful variable names that enhance code readability.
- Always initialize variables to avoid unexpected behavior.
- Limit the scope of variables to reduce memory usage and improve performance.
Summary of Variable Declaration Syntax
SQL Dialect | Declaration Syntax | Example |
---|---|---|
T-SQL | `DECLARE @VariableName DataType;` | `DECLARE @EmployeeCount INT;` |
PL/SQL | `DECLARE VariableName DataType;` | `DECLARE v_EmployeeCount NUMBER;` |
Following the outlined methods for declaring and using variables enhances the efficiency and clarity of SQL queries, making it easier to manage data within database operations.
Expert Insights on Declaring Variables in SQL Queries
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “Declaring variables in SQL queries is essential for managing temporary data within a session. Using the `DECLARE` statement allows developers to define variables that can enhance the readability and maintainability of complex queries.”
Michael Chen (Senior SQL Developer, Data Solutions Corp.). “When declaring variables in SQL, it is crucial to choose appropriate data types. This ensures efficient memory usage and prevents runtime errors. For instance, using `VARCHAR` for string data and `INT` for numerical values is a best practice that should be adhered to.”
Lisa Patel (SQL Trainer and Consultant, Query Masters). “Understanding the scope of declared variables in SQL is vital. Variables declared within a stored procedure are not accessible outside of it, which can lead to confusion. Clear documentation and naming conventions can mitigate these issues.”
Frequently Asked Questions (FAQs)
How do you declare a variable in SQL Server?
In SQL Server, you declare a variable using the `DECLARE` statement followed by the variable name and data type. For example: `DECLARE @MyVariable INT;`.
Can you assign a value to a variable at the time of declaration in SQL?
Yes, you can assign a value to a variable during declaration using the `SET` statement or the `SELECT` statement. For example: `DECLARE @MyVariable INT = 10;` or `SET @MyVariable = 10;`.
What is the scope of a variable declared in a SQL query?
The scope of a variable declared in a SQL query is limited to the batch, stored procedure, or function in which it is declared. It cannot be accessed outside of that context.
Can you use variables in SQL queries for conditional logic?
Yes, variables can be used in SQL queries for conditional logic, such as in `IF` statements or `CASE` expressions, allowing for dynamic query behavior based on variable values.
Are there different ways to declare variables in other SQL databases?
Yes, different SQL databases have varying syntax for declaring variables. For instance, MySQL uses `SET` or `SELECT` for user-defined variables, while PostgreSQL uses `DO` blocks or PL/pgSQL functions.
Can you declare multiple variables in a single statement in SQL?
Yes, you can declare multiple variables in a single statement by separating each declaration with a comma. For example: `DECLARE @Var1 INT, @Var2 VARCHAR(50);`.
Declaring variables in SQL queries is a fundamental skill that enhances the flexibility and efficiency of database operations. SQL allows for the declaration of variables using the `DECLARE` statement, which is typically followed by the variable name and its data type. This process is crucial for storing temporary data that can be used throughout the session or within a specific block of code, such as stored procedures or functions.
Once a variable is declared, it can be assigned a value using the `SET` statement or during the declaration itself. This enables developers to manipulate data dynamically, perform calculations, and store results for later use. It is important to note that the scope of the variable is limited to the batch, procedure, or function in which it is declared, ensuring that it does not interfere with other operations in the database.
Additionally, understanding the differences in variable declaration across various SQL dialects, such as T-SQL, PL/SQL, and MySQL, is essential for effective database management. Each dialect may have its own syntax and rules regarding variable usage, which can impact how queries are structured and executed. Therefore, familiarity with the specific SQL version being used is vital for optimal performance and accuracy in data manipulation.
Author Profile
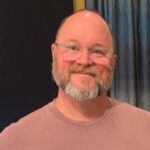
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?