How Can You Effectively Declare a Global Variable in Python?
In the realm of programming, the ability to manage variable scope effectively is crucial for building robust and maintainable code. Among the many languages that developers utilize, Python stands out for its simplicity and readability. However, as projects grow in complexity, understanding how to declare and manipulate global variables becomes essential. Whether you’re a seasoned programmer or a newcomer to the world of Python, mastering global variables can significantly enhance your coding prowess and streamline your development process.
Global variables in Python are accessible from any function or block of code, making them a powerful tool for sharing data across different parts of your program. However, with great power comes great responsibility. The misuse of global variables can lead to code that is difficult to debug and maintain, as it can introduce unexpected behavior and dependencies. Therefore, knowing when and how to declare a global variable is not just a matter of syntax; it’s about making informed decisions that will affect the overall architecture of your application.
In this article, we will explore the nuances of declaring global variables in Python, including the proper syntax and best practices. We will also discuss scenarios where global variables can be beneficial, as well as potential pitfalls to avoid. By the end, you’ll have a clearer understanding of how to leverage global variables effectively, ensuring your Python projects remain clean, efficient
Understanding Global Variables
Global variables in Python are variables that are declared outside of any function or class. This means they can be accessed and modified from any part of the code, including inside functions. Utilizing global variables can simplify data sharing across different functions but also introduces potential issues regarding code maintainability and unexpected side effects.
Declaring a Global Variable
To declare a global variable in Python, simply define it outside of any function. Here is an example:
“`python
my_global_var = 10 This is a global variable
“`
Once declared, this variable can be accessed from any function. However, if you want to modify the global variable from within a function, you must use the `global` keyword. Here’s how to do that:
“`python
def modify_global():
global my_global_var Declare the intention to use the global variable
my_global_var = 20 Modify the global variable
modify_global()
print(my_global_var) Outputs: 20
“`
Using the Global Keyword
The `global` keyword is essential when you need to change the value of a global variable inside a function. Without it, the assignment statement would create a new local variable instead of modifying the global one. Here are key points to remember:
- Use the `global` keyword for variables that need to be updated within a function.
- If a variable is only being read (i.e., not modified), it can be used without the `global` declaration.
Common Use Cases for Global Variables
While global variables can be beneficial, their use should be considered carefully. Common scenarios include:
- Configuration settings that need to be accessed by multiple functions.
- Shared counters or accumulators that are modified by different parts of the program.
Potential Issues with Global Variables
Although global variables can be convenient, they come with risks:
- Code Complexity: Tracking the changes to global variables can make debugging difficult.
- Namespace Pollution: Introducing too many global variables can lead to naming conflicts.
- Testing Challenges: Functions relying on global state can become harder to test in isolation.
Best Practices
To mitigate the drawbacks of using global variables, consider the following best practices:
- Limit their use to constants or configuration parameters.
- Encapsulate global variables within classes or modules when possible.
- Use clear and descriptive names to avoid confusion.
Example: Global Variables in a Table
Here is a table summarizing global variable usage:
Aspect | Description |
---|---|
Declaration | Outside any function or class |
Modification | Use the global keyword inside functions |
Accessibility | Accessible from any function or class |
Best Practice | Minimize usage; prefer local variables when possible |
By adhering to these guidelines, you can effectively manage global variables in Python while minimizing the associated risks.
Understanding Global Variables in Python
In Python, a global variable is defined outside any function or class and can be accessed from any function or method within the same module. These variables maintain their state throughout the program’s lifetime, allowing for consistent data sharing across different functions.
Declaring a Global Variable
To declare a global variable in Python, you simply assign a value to a variable at the top level of your script or module. The syntax is straightforward:
“`python
global_variable = “I am global”
“`
This variable can then be accessed by any function within the same module.
Using Global Variables in Functions
To modify a global variable inside a function, you must use the `global` keyword. This tells Python that you intend to use the global variable rather than create a local one. Here’s how you can do it:
“`python
global_variable = “I am global”
def modify_global():
global global_variable
global_variable = “I have been modified”
modify_global()
print(global_variable) Output: I have been modified
“`
Best Practices for Global Variables
While global variables can be useful, they should be used sparingly due to potential side effects and difficulties in debugging. Consider the following best practices:
- Limit Scope: Use global variables only when necessary. Prefer passing parameters to functions.
- Naming Conventions: Use uppercase letters for global variables (e.g., `GLOBAL_VAR`) to distinguish them from local variables.
- Documentation: Clearly document the purpose of global variables to aid readability and maintainability.
When to Avoid Global Variables
There are certain situations where using global variables is discouraged:
- Complexity: When a program becomes too complex, global variables can lead to unpredictable behavior.
- Testing: Global variables can make unit testing difficult, as they introduce hidden dependencies between functions.
- Concurrency Issues: In multi-threaded applications, global variables can lead to race conditions if not handled carefully.
Example: Using Global Variables Wisely
Here’s an example demonstrating a global variable used appropriately:
“`python
counter = 0
def increment():
global counter
counter += 1
def reset():
global counter
counter = 0
increment()
increment()
print(counter) Output: 2
reset()
print(counter) Output: 0
“`
In this example, the `counter` variable is modified through dedicated functions, showcasing controlled access to the global state.
Understanding how to declare and use global variables in Python is essential for managing data across functions effectively. However, mindful application of global variables is key to maintaining clean and maintainable code.
Expert Insights on Declaring Global Variables in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Declaring a global variable in Python requires the use of the `global` keyword within a function to modify its value. This ensures that the function accesses the variable defined at the module level rather than creating a local instance.”
Michael Chen (Lead Software Engineer, CodeMasters). “It is crucial to understand the scope of global variables in Python. They can be accessed from any function within the module, but if you intend to change their value inside a function, you must declare them as global to avoid scope-related errors.”
Sarah Thompson (Python Programming Instructor, LearnPython Academy). “When teaching Python, I emphasize the importance of using global variables judiciously. While they can simplify data sharing between functions, over-reliance on them can lead to code that is difficult to maintain and debug.”
Frequently Asked Questions (FAQs)
How do I declare a global variable in Python?
To declare a global variable in Python, define the variable outside of any function. You can then access it within functions using the `global` keyword to indicate that you are referring to the global variable rather than creating a local one.
Can I modify a global variable inside a function?
Yes, you can modify a global variable inside a function. To do so, use the `global` keyword before the variable name within the function to indicate that you are modifying the global variable.
What happens if I don’t use the global keyword?
If you do not use the `global` keyword when trying to modify a global variable inside a function, Python will treat the variable as a local variable, leading to an UnboundLocalError if you attempt to assign a value to it.
Are global variables a good practice in Python?
Global variables can lead to code that is difficult to understand and maintain. It is generally recommended to limit their use and consider alternatives such as passing parameters to functions or using class attributes.
How can I access a global variable from a nested function?
To access a global variable from a nested function, you can simply refer to the variable by its name. If you need to modify it, you must declare it as global within the nested function.
What are the alternatives to using global variables in Python?
Alternatives to using global variables include using function parameters, returning values from functions, utilizing classes and instance variables, or employing data structures like dictionaries to manage state more effectively.
In Python, declaring a global variable is essential for sharing data across different functions within a module. To declare a global variable, you must define it outside of any function. This allows the variable to be accessible throughout the module. When you want to modify a global variable inside a function, you need to use the `global` keyword to inform Python that you are referring to the global instance of that variable rather than creating a new local one.
Understanding the scope of variables is crucial when working with global variables. The scope determines where a variable can be accessed or modified. Global variables are accessible from any function within the module, but care must be taken to avoid unintended side effects, especially in larger programs. Overusing global variables can lead to code that is difficult to maintain and debug, so they should be used judiciously.
declaring global variables in Python involves defining them outside of functions and using the `global` keyword when modification is necessary. While global variables can facilitate data sharing across functions, it is important to manage their use carefully to maintain code clarity and integrity. By understanding the implications of variable scope, developers can write more effective and maintainable Python code.
Author Profile
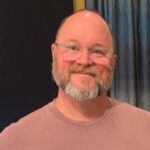
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?