How Can You Easily Create an Empty List in Python?
In the world of programming, lists are one of the most versatile and widely used data structures, especially in Python. Whether you’re managing a collection of items, organizing data, or preparing to process information dynamically, knowing how to create and manipulate lists is essential for any aspiring programmer. One of the fundamental skills in Python is the ability to create an empty list, a blank canvas that allows you to build and customize your data collection as your program evolves. In this article, we will explore the various methods to create an empty list in Python, along with the significance of this simple yet powerful operation.
Creating an empty list is often the first step in numerous programming tasks, from data collection to iterative processing. In Python, this can be accomplished in several straightforward ways, each offering its own advantages depending on the context in which you are working. Understanding these methods not only enhances your coding skills but also lays the groundwork for more complex operations involving lists.
As we delve deeper into the topic, we will uncover the nuances of list creation, including best practices and common pitfalls to avoid. Whether you’re a beginner looking to grasp the basics or an experienced developer seeking to refine your skills, this guide will provide you with the knowledge you need to effectively create and utilize empty lists in Python. Prepare
Creating an Empty List
In Python, creating an empty list is straightforward and can be done in two primary ways. Understanding these methods is essential for initializing lists that you may want to populate later in your program.
The first method is to use a pair of square brackets. This is the most common approach and is often favored for its simplicity and clarity. For example:
“`python
empty_list = []
“`
The second method involves using the `list()` constructor. This method can be particularly useful when you want to emphasize that you are creating a list. Here’s how you can do it:
“`python
empty_list = list()
“`
Both methods are functionally equivalent, and the choice between them often comes down to personal preference or specific coding standards within a project.
Advantages of Using Empty Lists
Empty lists are versatile and serve multiple purposes in Python programming. Here are some advantages of initializing an empty list:
- Dynamic Growth: You can append elements to the list as needed, allowing for dynamic data handling.
- Placeholder: An empty list can act as a placeholder in your code, making it clear that you intend to add items later.
- Iteration: You can loop through an empty list without errors, as Python handles empty iterables gracefully.
Working with Empty Lists
Once you have created an empty list, you can perform various operations. Below are some common methods and operations you might use:
Operation | Syntax | Description |
---|---|---|
Append Element | `list.append(item)` | Adds an item to the end of the list. |
Extend List | `list.extend(iterable)` | Adds elements from an iterable to the list. |
Insert Element | `list.insert(index, item)` | Inserts an item at the specified index. |
Remove Element | `list.remove(item)` | Removes the first occurrence of an item. |
Pop Element | `list.pop(index)` | Removes and returns the item at the specified index. |
Clear List | `list.clear()` | Removes all items from the list. |
Example Usage
Here is a simple example demonstrating how to create an empty list and use it in a program:
“`python
Creating an empty list
numbers = []
Appending items
numbers.append(1)
numbers.append(2)
numbers.append(3)
Displaying the list
print(numbers) Output: [1, 2, 3]
Removing an item
numbers.remove(2)
Displaying the updated list
print(numbers) Output: [1, 3]
“`
This example showcases how easy it is to manage an empty list by adding and removing elements, emphasizing the flexibility that lists offer in Python.
Creating an Empty List
In Python, creating an empty list can be accomplished in several straightforward ways. Below are the most commonly used methods:
Using Square Brackets
The simplest way to create an empty list is by using square brackets. This method is both concise and widely adopted among Python developers.
“`python
empty_list = []
“`
This line of code initializes `empty_list` as an empty list.
Using the list() Constructor
Another method to create an empty list is by using the `list()` constructor. This approach may be preferred in situations where clarity is essential.
“`python
empty_list = list()
“`
This also results in `empty_list` being an empty list.
Comparison of Methods
While both methods yield the same result, there are subtle differences in usage and readability. The following table summarizes these differences:
Method | Syntax | Readability | Use Cases |
---|---|---|---|
Square Brackets | `empty_list = []` | Very high | General use |
list() Constructor | `empty_list = list()` | High | When emphasizing list creation |
Adding Elements to the List
After creating an empty list, you may wish to add elements to it. This can be done using the `append()` method or the `extend()` method, depending on your needs.
- Using append(): Adds a single element to the end of the list.
“`python
empty_list.append(‘item1’)
“`
- Using extend(): Adds multiple elements from an iterable to the end of the list.
“`python
empty_list.extend([‘item2’, ‘item3’])
“`
Examples of Creating and Modifying an Empty List
Here are practical examples demonstrating the creation of an empty list and subsequent modifications:
“`python
Creating an empty list
my_list = []
Adding elements
my_list.append(‘apple’)
my_list.append(‘banana’)
Extending with multiple items
my_list.extend([‘orange’, ‘grape’])
print(my_list) Output: [‘apple’, ‘banana’, ‘orange’, ‘grape’]
“`
This snippet shows how to create an empty list and then populate it with fruit names.
Conclusion on List Creation
Understanding how to create and manipulate empty lists is fundamental in Python programming. The choice between using square brackets and the `list()` constructor often comes down to personal or team coding style preferences.
Expert Insights on Creating Empty Lists in Python
Dr. Emily Carter (Senior Software Engineer, Python Innovations Inc.). Creating an empty list in Python is a fundamental skill for any developer. It allows for dynamic data handling, enabling programmers to append items as needed. The syntax is straightforward: simply use `empty_list = []` or `empty_list = list()`. Understanding this concept is crucial for effective data manipulation.
Michael Chen (Lead Python Developer, CodeCraft Solutions). The ability to create an empty list is essential for initializing collections that will be populated later in your code. This practice not only enhances code readability but also optimizes performance, as it allows for efficient memory management when dealing with large datasets.
Sarah Patel (Data Scientist, Analytics Hub). In data science, initializing an empty list is often the first step in data collection processes. By using `my_list = []`, you can iteratively add elements based on conditions or results from computations. This flexibility is one of the many reasons Python remains a preferred language for data analysis tasks.
Frequently Asked Questions (FAQs)
How do I create an empty list in Python?
To create an empty list in Python, you can use either of the following methods: `empty_list = []` or `empty_list = list()`. Both will initialize an empty list.
Can I add elements to an empty list after creating it?
Yes, you can add elements to an empty list using methods such as `append()`, `extend()`, or `insert()`. For example, `empty_list.append(1)` will add the element `1` to the list.
What are some common use cases for an empty list in Python?
Empty lists are often used as placeholders for data collection, to store results from computations, or to initialize variables before populating them with data during loops or functions.
Is there a difference between using `[]` and `list()` to create an empty list?
No, there is no functional difference between using `[]` and `list()` to create an empty list. Both methods yield an empty list, and the choice often comes down to personal or stylistic preference.
Can I create a list with a specific size initialized to empty values?
Yes, you can create a list of a specific size initialized to empty values using list comprehension or multiplication. For example, `empty_list = [None] * 5` creates a list of five `None` values.
What happens if I try to access an index in an empty list?
Attempting to access an index in an empty list will raise an `IndexError`, as there are no elements in the list to access. Always ensure the list contains elements before accessing an index.
Creating an empty list in Python is a fundamental skill that serves as the foundation for utilizing lists effectively in programming. Lists are versatile data structures that can hold an ordered collection of items, and initializing them as empty is often the first step in various applications, such as data collection, manipulation, and storage. In Python, there are two primary methods to create an empty list: using the list constructor `list()` or by using square brackets `[]`. Both approaches are equally valid and can be chosen based on personal or stylistic preference.
Understanding how to create an empty list is essential for beginners and experienced programmers alike. An empty list can be populated later in the code, allowing for dynamic data handling. This flexibility is particularly useful in scenarios where the size or content of the list is not predetermined. Furthermore, mastering the creation of empty lists lays the groundwork for more advanced operations, such as list comprehensions, slicing, and iteration.
In summary, the ability to create an empty list in Python is a straightforward yet crucial aspect of programming in this language. It opens up opportunities for effective data management and manipulation. By familiarizing oneself with the syntax and use cases of empty lists, programmers can enhance their coding practices and build more robust applications.
Author Profile
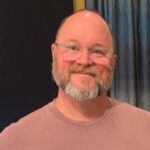
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?